title
stringlengths 1
100
| titleSlug
stringlengths 3
77
| Java
int64 0
1
| Python3
int64 1
1
| content
stringlengths 28
44.4k
| voteCount
int64 0
3.67k
| question_content
stringlengths 65
5k
| question_hints
stringclasses 970
values |
---|---|---|---|---|---|---|---|
Python Heap | Explained | find-score-of-an-array-after-marking-all-elements | 0 | 1 | # Intuition\nWe have to mark the smallest index as well as its left and right element too if they exist.\nTo find the smallest integer present use a heap pushing (ele, index)\nnow pop the smallest element and add it to seen and score if we havent seen this index before and then also add its left and right index if they exist.\n\n# Code\n```\nclass Solution:\n def findScore(self, nums: List[int]) -> int:\n score = 0\n bag = []\n for i, num in enumerate(nums):\n bag.append((num, i))\n heapq.heapify(bag)\n seen = set()\n while bag:\n smallestNum, idx = heapq.heappop(bag)\n if idx not in seen:\n score += smallestNum\n seen.add(idx)\n if idx - 1 >= 0:\n seen.add(idx-1)\n if idx + 1 < len(nums):\n seen.add(idx+1)\n return(score)\n\n\n\n``` | 1 | You are given an array `nums` consisting of positive integers.
Starting with `score = 0`, apply the following algorithm:
* Choose the smallest integer of the array that is not marked. If there is a tie, choose the one with the smallest index.
* Add the value of the chosen integer to `score`.
* Mark **the chosen element and its two adjacent elements if they exist**.
* Repeat until all the array elements are marked.
Return _the score you get after applying the above algorithm_.
**Example 1:**
**Input:** nums = \[2,1,3,4,5,2\]
**Output:** 7
**Explanation:** We mark the elements as follows:
- 1 is the smallest unmarked element, so we mark it and its two adjacent elements: \[2,1,3,4,5,2\].
- 2 is the smallest unmarked element, so we mark it and its left adjacent element: \[2,1,3,4,5,2\].
- 4 is the only remaining unmarked element, so we mark it: \[2,1,3,4,5,2\].
Our score is 1 + 2 + 4 = 7.
**Example 2:**
**Input:** nums = \[2,3,5,1,3,2\]
**Output:** 5
**Explanation:** We mark the elements as follows:
- 1 is the smallest unmarked element, so we mark it and its two adjacent elements: \[2,3,5,1,3,2\].
- 2 is the smallest unmarked element, since there are two of them, we choose the left-most one, so we mark the one at index 0 and its right adjacent element: \[2,3,5,1,3,2\].
- 2 is the only remaining unmarked element, so we mark it: \[2,3,5,1,3,2\].
Our score is 1 + 2 + 2 = 5.
**Constraints:**
* `1 <= nums.length <= 105`
* `1 <= nums[i] <= 106` | null |
Python 3 || 3 lines, bisect_left || T/M: 1712 ms / 17.7 MB | minimum-time-to-repair-cars | 0 | 1 | Note that we can use integer division and integer square root `isqrt` because of the nature of the problem. \n```\nclass Solution:\n def repairCars(self, ranks: List[int], cars: int) -> int:\n \n arr = range(cars*cars * max(ranks))\n\n f = lambda t: sum(isqrt(t//r) for r in ranks)\n\n return bisect_left(arr,cars, key = lambda t: f(t))\n```\n[https://leetcode.com/problems/minimum-time-to-repair-cars/submissions/918948065/](http://)\n\nI could be wrong, but I think that time complexity is *O*(*N*) and space complexity is *O*(*N*). | 3 | You are given an integer array `ranks` representing the **ranks** of some mechanics. ranksi is the rank of the ith mechanic. A mechanic with a rank `r` can repair n cars in `r * n2` minutes.
You are also given an integer `cars` representing the total number of cars waiting in the garage to be repaired.
Return _the **minimum** time taken to repair all the cars._
**Note:** All the mechanics can repair the cars simultaneously.
**Example 1:**
**Input:** ranks = \[4,2,3,1\], cars = 10
**Output:** 16
**Explanation:**
- The first mechanic will repair two cars. The time required is 4 \* 2 \* 2 = 16 minutes.
- The second mechanic will repair two cars. The time required is 2 \* 2 \* 2 = 8 minutes.
- The third mechanic will repair two cars. The time required is 3 \* 2 \* 2 = 12 minutes.
- The fourth mechanic will repair four cars. The time required is 1 \* 4 \* 4 = 16 minutes.
It can be proved that the cars cannot be repaired in less than 16 minutes.
**Example 2:**
**Input:** ranks = \[5,1,8\], cars = 6
**Output:** 16
**Explanation:**
- The first mechanic will repair one car. The time required is 5 \* 1 \* 1 = 5 minutes.
- The second mechanic will repair four cars. The time required is 1 \* 4 \* 4 = 16 minutes.
- The third mechanic will repair one car. The time required is 8 \* 1 \* 1 = 8 minutes.
It can be proved that the cars cannot be repaired in less than 16 minutes.
**Constraints:**
* `1 <= ranks.length <= 105`
* `1 <= ranks[i] <= 100`
* `1 <= cars <= 106` | null |
Python Easy solution with Easy explanation | minimum-time-to-repair-cars | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\nApply binary search on time.\nImportant things:\n1) Setting the limits\n left limit: 0(if no cars)\n right limit: cars*(maximum rating)<sup>2</sup>\n2) Check function.\n finding number of cars can be repaired with given time(countcars).\n if(totalcars>countcars):\n not possible with that time \n move left pointer to mid+1\n else:\n store the mid val\n move right pointer to mid-1\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n# o(nlogn)\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n# o(1)\n\n# Code\n```\nfrom math import sqrt\n\nclass Solution:\n def repairCars(self, ranks: List[int], cars: int) -> int:\n def check(maxtime):\n total=0\n for i in range(len(ranks)):\n total+=int(sqrt(maxtime//ranks[i]))\n if(total>=cars):\n return True\n return False\n left=0\n right=cars*cars*max(ranks)\n ans=-1\n while(left<=right):\n mid=left+(right-left)//2\n if(check(mid)):\n ans=mid\n right=mid-1\n else:\n left=mid+1\n return ans\n\n\n\n \n\n \n``` | 1 | You are given an integer array `ranks` representing the **ranks** of some mechanics. ranksi is the rank of the ith mechanic. A mechanic with a rank `r` can repair n cars in `r * n2` minutes.
You are also given an integer `cars` representing the total number of cars waiting in the garage to be repaired.
Return _the **minimum** time taken to repair all the cars._
**Note:** All the mechanics can repair the cars simultaneously.
**Example 1:**
**Input:** ranks = \[4,2,3,1\], cars = 10
**Output:** 16
**Explanation:**
- The first mechanic will repair two cars. The time required is 4 \* 2 \* 2 = 16 minutes.
- The second mechanic will repair two cars. The time required is 2 \* 2 \* 2 = 8 minutes.
- The third mechanic will repair two cars. The time required is 3 \* 2 \* 2 = 12 minutes.
- The fourth mechanic will repair four cars. The time required is 1 \* 4 \* 4 = 16 minutes.
It can be proved that the cars cannot be repaired in less than 16 minutes.
**Example 2:**
**Input:** ranks = \[5,1,8\], cars = 6
**Output:** 16
**Explanation:**
- The first mechanic will repair one car. The time required is 5 \* 1 \* 1 = 5 minutes.
- The second mechanic will repair four cars. The time required is 1 \* 4 \* 4 = 16 minutes.
- The third mechanic will repair one car. The time required is 8 \* 1 \* 1 = 8 minutes.
It can be proved that the cars cannot be repaired in less than 16 minutes.
**Constraints:**
* `1 <= ranks.length <= 105`
* `1 <= ranks[i] <= 100`
* `1 <= cars <= 106` | null |
✅98.44%🔥3 line code🔥 | minimum-time-to-repair-cars | 1 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def repairCars(self, ranks: List[int], cars: int) -> int:\n \n arr = range(cars*cars * max(ranks))\n\n f = lambda t: sum(isqrt(t//r) for r in ranks)\n\n return bisect_left(arr,cars, key = lambda t: f(t))\n``` | 1 | You are given an integer array `ranks` representing the **ranks** of some mechanics. ranksi is the rank of the ith mechanic. A mechanic with a rank `r` can repair n cars in `r * n2` minutes.
You are also given an integer `cars` representing the total number of cars waiting in the garage to be repaired.
Return _the **minimum** time taken to repair all the cars._
**Note:** All the mechanics can repair the cars simultaneously.
**Example 1:**
**Input:** ranks = \[4,2,3,1\], cars = 10
**Output:** 16
**Explanation:**
- The first mechanic will repair two cars. The time required is 4 \* 2 \* 2 = 16 minutes.
- The second mechanic will repair two cars. The time required is 2 \* 2 \* 2 = 8 minutes.
- The third mechanic will repair two cars. The time required is 3 \* 2 \* 2 = 12 minutes.
- The fourth mechanic will repair four cars. The time required is 1 \* 4 \* 4 = 16 minutes.
It can be proved that the cars cannot be repaired in less than 16 minutes.
**Example 2:**
**Input:** ranks = \[5,1,8\], cars = 6
**Output:** 16
**Explanation:**
- The first mechanic will repair one car. The time required is 5 \* 1 \* 1 = 5 minutes.
- The second mechanic will repair four cars. The time required is 1 \* 4 \* 4 = 16 minutes.
- The third mechanic will repair one car. The time required is 8 \* 1 \* 1 = 8 minutes.
It can be proved that the cars cannot be repaired in less than 16 minutes.
**Constraints:**
* `1 <= ranks.length <= 105`
* `1 <= ranks[i] <= 100`
* `1 <= cars <= 106` | null |
python3 Solution | minimum-time-to-repair-cars | 0 | 1 | \n```\nclass Solution:\n def repairCars(self, ranks: List[int], cars: int) -> int:\n count=Counter(ranks)\n left=1\n right=min(count)*cars*cars\n while left<right:\n mid=(left+right)//2\n if sum(isqrt(mid//a)*count[a] for a in count)<cars:\n left=mid+1\n\n else:\n right=mid\n\n return left \n``` | 1 | You are given an integer array `ranks` representing the **ranks** of some mechanics. ranksi is the rank of the ith mechanic. A mechanic with a rank `r` can repair n cars in `r * n2` minutes.
You are also given an integer `cars` representing the total number of cars waiting in the garage to be repaired.
Return _the **minimum** time taken to repair all the cars._
**Note:** All the mechanics can repair the cars simultaneously.
**Example 1:**
**Input:** ranks = \[4,2,3,1\], cars = 10
**Output:** 16
**Explanation:**
- The first mechanic will repair two cars. The time required is 4 \* 2 \* 2 = 16 minutes.
- The second mechanic will repair two cars. The time required is 2 \* 2 \* 2 = 8 minutes.
- The third mechanic will repair two cars. The time required is 3 \* 2 \* 2 = 12 minutes.
- The fourth mechanic will repair four cars. The time required is 1 \* 4 \* 4 = 16 minutes.
It can be proved that the cars cannot be repaired in less than 16 minutes.
**Example 2:**
**Input:** ranks = \[5,1,8\], cars = 6
**Output:** 16
**Explanation:**
- The first mechanic will repair one car. The time required is 5 \* 1 \* 1 = 5 minutes.
- The second mechanic will repair four cars. The time required is 1 \* 4 \* 4 = 16 minutes.
- The third mechanic will repair one car. The time required is 8 \* 1 \* 1 = 8 minutes.
It can be proved that the cars cannot be repaired in less than 16 minutes.
**Constraints:**
* `1 <= ranks.length <= 105`
* `1 <= ranks[i] <= 100`
* `1 <= cars <= 106` | null |
[Python 3] Binary Search | minimum-time-to-repair-cars | 0 | 1 | The helper function *repair(time)* determines if *cars* can be repaired within *time*.\n\n# Code\n```\nclass Solution:\n def repairCars(self, ranks: List[int], cars: int) -> int:\n lo,hi = 0,100*cars*cars\n def repair(time):\n n = cars\n for r in ranks:\n n -= floor(sqrt(time/r))\n if n<=0:\n break\n return n<=0\n while lo<=hi:\n m = (lo+hi)//2\n if repair(m):\n ans = m\n hi = m-1\n else:\n lo = m+1\n return ans\n``` | 2 | You are given an integer array `ranks` representing the **ranks** of some mechanics. ranksi is the rank of the ith mechanic. A mechanic with a rank `r` can repair n cars in `r * n2` minutes.
You are also given an integer `cars` representing the total number of cars waiting in the garage to be repaired.
Return _the **minimum** time taken to repair all the cars._
**Note:** All the mechanics can repair the cars simultaneously.
**Example 1:**
**Input:** ranks = \[4,2,3,1\], cars = 10
**Output:** 16
**Explanation:**
- The first mechanic will repair two cars. The time required is 4 \* 2 \* 2 = 16 minutes.
- The second mechanic will repair two cars. The time required is 2 \* 2 \* 2 = 8 minutes.
- The third mechanic will repair two cars. The time required is 3 \* 2 \* 2 = 12 minutes.
- The fourth mechanic will repair four cars. The time required is 1 \* 4 \* 4 = 16 minutes.
It can be proved that the cars cannot be repaired in less than 16 minutes.
**Example 2:**
**Input:** ranks = \[5,1,8\], cars = 6
**Output:** 16
**Explanation:**
- The first mechanic will repair one car. The time required is 5 \* 1 \* 1 = 5 minutes.
- The second mechanic will repair four cars. The time required is 1 \* 4 \* 4 = 16 minutes.
- The third mechanic will repair one car. The time required is 8 \* 1 \* 1 = 8 minutes.
It can be proved that the cars cannot be repaired in less than 16 minutes.
**Constraints:**
* `1 <= ranks.length <= 105`
* `1 <= ranks[i] <= 100`
* `1 <= cars <= 106` | null |
[Java/Python 3] Binary Search. | minimum-time-to-repair-cars | 1 | 1 | **Q & A:**\n\n*Q1:* What is the logic behind setting higher bound for binary search as `hi = 1L * maxRank * cars * cars`?\n \n *A1:* The one with highest rank repairing all cars will cost longest time, and there is no way that we could spend more time to finish all jobs. Therefore, we set the worst case of `L * maxRank * cars * cars` as the higher bound of our search space.\n\n**Q & A:**\n\n----\n\n1. The worst case is that the max rank repairs all cars, and the corresponding time cost is the higher bound of our search space;\n2. Set the lower bound as `0`;\n3. Binary search the minimum time need to repair all cars.\n\n```java\n public long repairCars(int[] ranks, int cars) {\n int maxRank = IntStream.of(ranks).max().getAsInt();\n long lo = 0, hi = 1L * maxRank * cars * cars;\n while (lo < hi) {\n long time = lo + (hi - lo) / 2;\n if (canFinish(ranks, cars, time)) {\n hi = time;\n }else {\n lo = time + 1;\n }\n }\n return lo;\n }\n private boolean canFinish(int[] ranks, int cars, long time) {\n long finish = 0;\n for (int r : ranks) {\n finish += (int)Math.floor(Math.sqrt(time / r));\n if (finish >= cars) {\n return true;\n }\n }\n return false;\n }\n```\n\n```python\n def repairCars(self, ranks: List[int], cars: int) -> int:\n \n def canFinish(time: int) -> bool:\n finish = 0\n for r in ranks:\n finish += floor(math.sqrt(time / r))\n if finish >= cars:\n return True\n return False\n\n lo, hi = 0, max(ranks) * cars * cars\n while lo < hi:\n time = lo + (hi - lo) // 2\n if canFinish(time):\n hi = time\n else:\n lo = time + 1\n return lo\n```\nSince Python 3.10 we can simplify the above code as follows:\n```python\n def repairCars(self, ranks: List[int], cars: int) -> int:\n return bisect_left(range(max(ranks) * cars * cars), cars, key=lambda x: sum(floor(math.sqrt(x // r)) for r in ranks))\n```\n\n**Analysis:**\n\nTime: `O(nlog(max(ranks) * m * m))`, space: `O(1)`, where `n = ranks.length, m = cars`. | 12 | You are given an integer array `ranks` representing the **ranks** of some mechanics. ranksi is the rank of the ith mechanic. A mechanic with a rank `r` can repair n cars in `r * n2` minutes.
You are also given an integer `cars` representing the total number of cars waiting in the garage to be repaired.
Return _the **minimum** time taken to repair all the cars._
**Note:** All the mechanics can repair the cars simultaneously.
**Example 1:**
**Input:** ranks = \[4,2,3,1\], cars = 10
**Output:** 16
**Explanation:**
- The first mechanic will repair two cars. The time required is 4 \* 2 \* 2 = 16 minutes.
- The second mechanic will repair two cars. The time required is 2 \* 2 \* 2 = 8 minutes.
- The third mechanic will repair two cars. The time required is 3 \* 2 \* 2 = 12 minutes.
- The fourth mechanic will repair four cars. The time required is 1 \* 4 \* 4 = 16 minutes.
It can be proved that the cars cannot be repaired in less than 16 minutes.
**Example 2:**
**Input:** ranks = \[5,1,8\], cars = 6
**Output:** 16
**Explanation:**
- The first mechanic will repair one car. The time required is 5 \* 1 \* 1 = 5 minutes.
- The second mechanic will repair four cars. The time required is 1 \* 4 \* 4 = 16 minutes.
- The third mechanic will repair one car. The time required is 8 \* 1 \* 1 = 8 minutes.
It can be proved that the cars cannot be repaired in less than 16 minutes.
**Constraints:**
* `1 <= ranks.length <= 105`
* `1 <= ranks[i] <= 100`
* `1 <= cars <= 106` | null |
[C++, Java, Python3] Binary Search + 1 liner | minimum-time-to-repair-cars | 1 | 1 | \n# Intuition\nA mechanic can repair `n` cars in `r * n * n` minutes. So in `mid` minutes a mechanic can repair `n` cars where, `n = sqrt(mid / r)` \nLet\'s assume that the correct answer is `mid` minutes, we can find the number of cars each mechanic can repair in `mid` minutes, then find the find the total number of cars fixed. If total number of cars fixed is greater than or equal to the cars we have to repair, `mid` minutes is a valid answer, else we have to increase `mid`. We can not linearly calculate total cars fixed for each mid in (0 to 10^14), we must use binary search to lower the time complexity.\n\n# Approach\n* Total cars repaired by all workers in `mid` minutes must be greater than or equal to total cars that have to be repaired\n* Binary search over the range `0 to 10^14` to find what is the minimum time required to repair all cars\n* We use high as `10^14` because `max(rank) = 100` and `max(cars) = 10^6` so, `r * n * n = 100 * 10^6 * 10^6 = 10^14`\n\n# Complexity\n- Time complexity: `O(nlog(10^14))`\n\n- Space complexity: `O(1)`\n\n# Code\n**C++**:\n```\nlong long repairCars(vector<int>& ranks, int cars) {\n long long low = 0, high = 1e14;\n while (low < high) {\n long long mid = low + (high - low) / 2, repaired_cars = 0;\n for (int& r: ranks)\n repaired_cars += sqrt(mid / r);\n if (repaired_cars < cars) low = mid + 1;\n else high = mid;\n }\n return low;\n}\n```\n\n**Java**:\n```\npublic long repairCars(int[] ranks, int cars) {\n long low = 0, high = (long)1e14;\n while (low < high) {\n long mid = low + (high - low) / 2, repaired_cars = 0;\n for (int r: ranks)\n repaired_cars += Math.sqrt(mid / r);\n if (repaired_cars < cars) low = mid + 1;\n else high = mid;\n }\n return low;\n}\n```\n\n**Python3**:\n```\ndef repairCars(self, A: List[int], cars: int) -> int:\n return bisect_left(range(10**14), cars, key=lambda x: sum(int(sqrt(x / a)) for a in A))\n```\n\nSimilar Questions:\n[Minimum Time to Complete Trips](https://leetcode.com/problems/minimum-time-to-complete-trips/solutions/1802415/python3-java-c-binary-search-1-liner/)\n[Kth Missing Positive Number](https://leetcode.com/problems/kth-missing-positive-number/solutions/3262163/c-java-python3-1-line-ologn/)\n[Maximum Tastiness of Candy Basket](https://leetcode.com/problems/maximum-tastiness-of-candy-basket/solutions/2947983/c-java-python-binary-search-and-sorting/)\n[Number of Flowers in Full Bloom](https://leetcode.com/problems/number-of-flowers-in-full-bloom/solutions/1976804/python3-2-lines-sort-and-binary-search/) | 62 | You are given an integer array `ranks` representing the **ranks** of some mechanics. ranksi is the rank of the ith mechanic. A mechanic with a rank `r` can repair n cars in `r * n2` minutes.
You are also given an integer `cars` representing the total number of cars waiting in the garage to be repaired.
Return _the **minimum** time taken to repair all the cars._
**Note:** All the mechanics can repair the cars simultaneously.
**Example 1:**
**Input:** ranks = \[4,2,3,1\], cars = 10
**Output:** 16
**Explanation:**
- The first mechanic will repair two cars. The time required is 4 \* 2 \* 2 = 16 minutes.
- The second mechanic will repair two cars. The time required is 2 \* 2 \* 2 = 8 minutes.
- The third mechanic will repair two cars. The time required is 3 \* 2 \* 2 = 12 minutes.
- The fourth mechanic will repair four cars. The time required is 1 \* 4 \* 4 = 16 minutes.
It can be proved that the cars cannot be repaired in less than 16 minutes.
**Example 2:**
**Input:** ranks = \[5,1,8\], cars = 6
**Output:** 16
**Explanation:**
- The first mechanic will repair one car. The time required is 5 \* 1 \* 1 = 5 minutes.
- The second mechanic will repair four cars. The time required is 1 \* 4 \* 4 = 16 minutes.
- The third mechanic will repair one car. The time required is 8 \* 1 \* 1 = 8 minutes.
It can be proved that the cars cannot be repaired in less than 16 minutes.
**Constraints:**
* `1 <= ranks.length <= 105`
* `1 <= ranks[i] <= 100`
* `1 <= cars <= 106` | null |
Python Binary search with explanation | minimum-time-to-repair-cars | 0 | 1 | # Intuition\n1. Find minimum time within which given number of cars could be repaired.\n2. Count cars that can be repaired by each person within x time. If more cars can be repaired during x time than given number of cars, search for a lesser time.\n3. If lesser cars can be repaired during x time than given number of cars, search for higher time.\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\nO(nlogn)\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def repairCars(self, ranks: List[int], cars: int) -> int:\n ans,low,high = -1,1,10**14\n while low <= high:\n mid = (low+high)//2\n i=0\n cars_temp=0\n while i < len(ranks):\n cars_temp += math.floor((mid/ranks[i])**0.5)\n#count cars for given rank and selected time\n\n i+=1\n if cars_temp >= cars:\n ans=mid\n high = mid-1 #If more cars can be repaired during x time than given number of cars, search for a lesser time.\n\n else:\n low=mid+1 #If lesser cars can be repaired during x time than given number of cars, search for higher time.\n return ans\n``` | 1 | You are given an integer array `ranks` representing the **ranks** of some mechanics. ranksi is the rank of the ith mechanic. A mechanic with a rank `r` can repair n cars in `r * n2` minutes.
You are also given an integer `cars` representing the total number of cars waiting in the garage to be repaired.
Return _the **minimum** time taken to repair all the cars._
**Note:** All the mechanics can repair the cars simultaneously.
**Example 1:**
**Input:** ranks = \[4,2,3,1\], cars = 10
**Output:** 16
**Explanation:**
- The first mechanic will repair two cars. The time required is 4 \* 2 \* 2 = 16 minutes.
- The second mechanic will repair two cars. The time required is 2 \* 2 \* 2 = 8 minutes.
- The third mechanic will repair two cars. The time required is 3 \* 2 \* 2 = 12 minutes.
- The fourth mechanic will repair four cars. The time required is 1 \* 4 \* 4 = 16 minutes.
It can be proved that the cars cannot be repaired in less than 16 minutes.
**Example 2:**
**Input:** ranks = \[5,1,8\], cars = 6
**Output:** 16
**Explanation:**
- The first mechanic will repair one car. The time required is 5 \* 1 \* 1 = 5 minutes.
- The second mechanic will repair four cars. The time required is 1 \* 4 \* 4 = 16 minutes.
- The third mechanic will repair one car. The time required is 8 \* 1 \* 1 = 8 minutes.
It can be proved that the cars cannot be repaired in less than 16 minutes.
**Constraints:**
* `1 <= ranks.length <= 105`
* `1 <= ranks[i] <= 100`
* `1 <= cars <= 106` | null |
Python, binary seach solution | minimum-time-to-repair-cars | 0 | 1 | Question is similar to koko eating bananas, binary search is used to find optimal answer.\n\n# Complexity\n- Time complexity:\n$$O(nlog(r*c^2))$$\n\n- Space complexity:\n$$O(1)$$\n\n# Code\n```\nclass Solution:\n def repairCars(self, ranks: List[int], cars: int) -> int:\n left, right = 0, min(ranks) * cars * cars\n while left < right:\n mid = (left + right) // 2\n carsRepaired = sum(floor(sqrt(mid/rank)) for rank in ranks)\n if carsRepaired >= cars:\n right = mid\n else:\n left = mid + 1\n \n return left\n \n\n``` | 0 | You are given an integer array `ranks` representing the **ranks** of some mechanics. ranksi is the rank of the ith mechanic. A mechanic with a rank `r` can repair n cars in `r * n2` minutes.
You are also given an integer `cars` representing the total number of cars waiting in the garage to be repaired.
Return _the **minimum** time taken to repair all the cars._
**Note:** All the mechanics can repair the cars simultaneously.
**Example 1:**
**Input:** ranks = \[4,2,3,1\], cars = 10
**Output:** 16
**Explanation:**
- The first mechanic will repair two cars. The time required is 4 \* 2 \* 2 = 16 minutes.
- The second mechanic will repair two cars. The time required is 2 \* 2 \* 2 = 8 minutes.
- The third mechanic will repair two cars. The time required is 3 \* 2 \* 2 = 12 minutes.
- The fourth mechanic will repair four cars. The time required is 1 \* 4 \* 4 = 16 minutes.
It can be proved that the cars cannot be repaired in less than 16 minutes.
**Example 2:**
**Input:** ranks = \[5,1,8\], cars = 6
**Output:** 16
**Explanation:**
- The first mechanic will repair one car. The time required is 5 \* 1 \* 1 = 5 minutes.
- The second mechanic will repair four cars. The time required is 1 \* 4 \* 4 = 16 minutes.
- The third mechanic will repair one car. The time required is 8 \* 1 \* 1 = 8 minutes.
It can be proved that the cars cannot be repaired in less than 16 minutes.
**Constraints:**
* `1 <= ranks.length <= 105`
* `1 <= ranks[i] <= 100`
* `1 <= cars <= 106` | null |
Easy Python Solution | number-of-even-and-odd-bits | 0 | 1 | \n# Code\n```\nclass Solution:\n def evenOddBit(self, n: int) -> List[int]:\n x=(bin(n)[2:])[::-1]\n even=0\n odd=0\n for i in range(len(x)):\n if i%2==0:\n if x[i]=="1":\n even+=1\n else:\n if x[i]=="1":\n odd+=1\n return [even, odd]\n``` | 2 | You are given a **positive** integer `n`.
Let `even` denote the number of even indices in the binary representation of `n` (**0-indexed**) with value `1`.
Let `odd` denote the number of odd indices in the binary representation of `n` (**0-indexed**) with value `1`.
Return _an integer array_ `answer` _where_ `answer = [even, odd]`.
**Example 1:**
**Input:** n = 17
**Output:** \[2,0\]
**Explanation:** The binary representation of 17 is 10001.
It contains 1 on the 0th and 4th indices.
There are 2 even and 0 odd indices.
**Example 2:**
**Input:** n = 2
**Output:** \[0,1\]
**Explanation:** The binary representation of 2 is 10.
It contains 1 on the 1st index.
There are 0 even and 1 odd indices.
**Constraints:**
* `1 <= n <= 1000` | null |
Easy Binary | number-of-even-and-odd-bits | 0 | 1 | \n\n# Code\n```\nclass Solution:\n def evenOddBit(self, n: int) -> List[int]:\n x=bin(n)\n s=x[2:]\n s=s[::-1]\n # s=str(n)\n l=list(s)\n ot=[0,0]\n for i in range(len(s)) :\n if(l[i]==\'1\' and (i)%2==1) :\n ot[1]+=1\n elif(l[i]==\'1\' and i%2==0) :\n ot[0]+=1\n return ot\n``` | 1 | You are given a **positive** integer `n`.
Let `even` denote the number of even indices in the binary representation of `n` (**0-indexed**) with value `1`.
Let `odd` denote the number of odd indices in the binary representation of `n` (**0-indexed**) with value `1`.
Return _an integer array_ `answer` _where_ `answer = [even, odd]`.
**Example 1:**
**Input:** n = 17
**Output:** \[2,0\]
**Explanation:** The binary representation of 17 is 10001.
It contains 1 on the 0th and 4th indices.
There are 2 even and 0 odd indices.
**Example 2:**
**Input:** n = 2
**Output:** \[0,1\]
**Explanation:** The binary representation of 2 is 10.
It contains 1 on the 1st index.
There are 0 even and 1 odd indices.
**Constraints:**
* `1 <= n <= 1000` | null |
[c++ & Python] O(n) soution | number-of-even-and-odd-bits | 0 | 1 | \n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity: $$O(n)$$\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: $$O(1)$$\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# C++\n```\nclass Solution {\npublic:\n vector<int> evenOddBit(int n) {\n int even = 0, odd = 0, ind = 0;\n while(n) {\n if((ind & 1) && (n & 1)) odd++;\n else if(n & 1) even++;\n ind++;\n n = n >> 1;\n }\n return {even, odd};\n }\n};\n```\n\n# Python / Python3\n```\nclass Solution:\n def evenOddBit(self, n: int) -> List[int]:\n even = odd = ind = 0\n while n:\n if (ind & 1) and (n & 1):\n odd += 1\n elif n & 1:\n even += 1\n ind += 1\n n = n >> 1\n return [even, odd]\n``` | 1 | You are given a **positive** integer `n`.
Let `even` denote the number of even indices in the binary representation of `n` (**0-indexed**) with value `1`.
Let `odd` denote the number of odd indices in the binary representation of `n` (**0-indexed**) with value `1`.
Return _an integer array_ `answer` _where_ `answer = [even, odd]`.
**Example 1:**
**Input:** n = 17
**Output:** \[2,0\]
**Explanation:** The binary representation of 17 is 10001.
It contains 1 on the 0th and 4th indices.
There are 2 even and 0 odd indices.
**Example 2:**
**Input:** n = 2
**Output:** \[0,1\]
**Explanation:** The binary representation of 2 is 10.
It contains 1 on the 1st index.
There are 0 even and 1 odd indices.
**Constraints:**
* `1 <= n <= 1000` | null |
Python easy 100% beats - 2595. Number of Even and Odd Bits | number-of-even-and-odd-bits | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def evenOddBit(self, n: int) -> List[int]:\n val = bin(n)[2::][::-1]\n l = [0 , 0]\n for i in range(0 , len(val)):\n if val[i]==\'1\':\n if i%2:l[1]+=1\n else:l[0]+=1\n return l\n``` | 1 | You are given a **positive** integer `n`.
Let `even` denote the number of even indices in the binary representation of `n` (**0-indexed**) with value `1`.
Let `odd` denote the number of odd indices in the binary representation of `n` (**0-indexed**) with value `1`.
Return _an integer array_ `answer` _where_ `answer = [even, odd]`.
**Example 1:**
**Input:** n = 17
**Output:** \[2,0\]
**Explanation:** The binary representation of 17 is 10001.
It contains 1 on the 0th and 4th indices.
There are 2 even and 0 odd indices.
**Example 2:**
**Input:** n = 2
**Output:** \[0,1\]
**Explanation:** The binary representation of 2 is 10.
It contains 1 on the 1st index.
There are 0 even and 1 odd indices.
**Constraints:**
* `1 <= n <= 1000` | null |
Python 3 || 2 lines, w/ example || T/M: 31 ms / 13.8 MB | number-of-even-and-odd-bits | 0 | 1 | Here\'s the code:\n```\nclass Solution:\n def evenOddBit(self, n: int) -> List[int]: \n evens, odds = int(\'0101010101\',2), int(\'1010101010\',2)\n\n return [(evens&n).bit_count(),(odds&n).bit_count()]\n```\nHere\'s an example:\n```\n Example: n = 545 --> \'0b1000100001\'\n\n n = 1000100001 n = 1000100001\n evens = 0101010101 odds = 1010101010\n \u2013\u2013\u2013\u2013\u2013\u2013\u2013\u2013\u2013\u2013 \u2013\u2013\u2013\u2013\u2013\u2013\u2013\u2013\u2013\u2013\n evens&n = 0000000001 odds&n = 1000100000\n\n return [1,2]\n```\n[https://leetcode.com/problems/number-of-even-and-odd-bits/submissions/918209401/](http://)\n\n\n\nI could be wrong, but I think that time complexity is *O*(1) and space complexity is *O*(1). | 6 | You are given a **positive** integer `n`.
Let `even` denote the number of even indices in the binary representation of `n` (**0-indexed**) with value `1`.
Let `odd` denote the number of odd indices in the binary representation of `n` (**0-indexed**) with value `1`.
Return _an integer array_ `answer` _where_ `answer = [even, odd]`.
**Example 1:**
**Input:** n = 17
**Output:** \[2,0\]
**Explanation:** The binary representation of 17 is 10001.
It contains 1 on the 0th and 4th indices.
There are 2 even and 0 odd indices.
**Example 2:**
**Input:** n = 2
**Output:** \[0,1\]
**Explanation:** The binary representation of 2 is 10.
It contains 1 on the 1st index.
There are 0 even and 1 odd indices.
**Constraints:**
* `1 <= n <= 1000` | null |
[Python3] Simple solutions with explanation (string, bit manipulation) | number-of-even-and-odd-bits | 0 | 1 | The logic behind is based on the observation that the least significant group of ones can be determined by checking whether the least significant bit of n is a \'1\' or a \'0\'. Similarly, the next group of ones can be determined by checking the second least significant bit, and so on.\nWe count adjacent groups of ones, starting from the most significant bit to the least significant bit. If the current index is even and the value is \'1\', increment the first element of the list, otherwise, if the current index is odd and the value is also \'1\', increment the second element of the list.\n```\nclass Solution:\n def evenOddBit(self, n: int) -> List[int]:\n ans = [0, 0]\n \n for idx, val in enumerate(bin(n)[2:][::-1]):\n if idx % 2 == 0 and val == \'1\':\n ans[0] += 1\n elif idx % 2 == 1 and val == \'1\':\n ans[1] += 1\n \n return ans\n```\nSame story, but now with bitwise operations. Inside the while loop, we add the least significant bit of **n** to the appropriate element in the list based on the value of **i**: if i == 0, we add the least significant bit to the first element of the list, otherwise, we add it to the second element of the list. Next, we **right shift** n by one bit, removes the least significant bit of n. Finally, we use **XOR** i with 1 to flips the value of i between 0 and 1.\n\n```\nclass Solution:\n def evenOddBit(self, n: int) -> List[int]:\n ans = [0, 0]\n i = 0\n \n while n:\n ans[i] += n & 1\n n >>= 1\n i ^= 1\n\n return ans\n``` | 3 | You are given a **positive** integer `n`.
Let `even` denote the number of even indices in the binary representation of `n` (**0-indexed**) with value `1`.
Let `odd` denote the number of odd indices in the binary representation of `n` (**0-indexed**) with value `1`.
Return _an integer array_ `answer` _where_ `answer = [even, odd]`.
**Example 1:**
**Input:** n = 17
**Output:** \[2,0\]
**Explanation:** The binary representation of 17 is 10001.
It contains 1 on the 0th and 4th indices.
There are 2 even and 0 odd indices.
**Example 2:**
**Input:** n = 2
**Output:** \[0,1\]
**Explanation:** The binary representation of 2 is 10.
It contains 1 on the 1st index.
There are 0 even and 1 odd indices.
**Constraints:**
* `1 <= n <= 1000` | null |
Python 3 || 12 lines, iteration and dict || T/M: 100% / 89% | check-knight-tour-configuration | 0 | 1 | ```\nclass Solution:\n def checkValidGrid(self, grid: List[List[int]]) -> bool:\n\n n, d = len(grid), defaultdict(tuple)\n if n < 5: return n == 1\n\n notLegal = lambda x, y : {abs(x[0]-y[0]),\n abs(x[1]-y[1])} != {1,2}\n\n for row, col in product(range(n),range(n)):\n d[grid[row][col]] = (row,col)\n \n prev, cnt = (0,0), 1\n\n while cnt < n*n:\n curr = d[cnt]\n\n if notLegal(prev,curr): return False\n\n cnt+=1\n prev = curr\n\n return True\n```\n[https://leetcode.com/problems/check-knight-tour-configuration/submissions/1006491076/](http://)\n\n\nI could be wrong, but I think that time complexity is *O*(*N*^2) and space complexity is *O*(*N*^2). | 4 | There is a knight on an `n x n` chessboard. In a valid configuration, the knight starts **at the top-left cell** of the board and visits every cell on the board **exactly once**.
You are given an `n x n` integer matrix `grid` consisting of distinct integers from the range `[0, n * n - 1]` where `grid[row][col]` indicates that the cell `(row, col)` is the `grid[row][col]th` cell that the knight visited. The moves are **0-indexed**.
Return `true` _if_ `grid` _represents a valid configuration of the knight's movements or_ `false` _otherwise_.
**Note** that a valid knight move consists of moving two squares vertically and one square horizontally, or two squares horizontally and one square vertically. The figure below illustrates all the possible eight moves of a knight from some cell.
**Example 1:**
**Input:** grid = \[\[0,11,16,5,20\],\[17,4,19,10,15\],\[12,1,8,21,6\],\[3,18,23,14,9\],\[24,13,2,7,22\]\]
**Output:** true
**Explanation:** The above diagram represents the grid. It can be shown that it is a valid configuration.
**Example 2:**
**Input:** grid = \[\[0,3,6\],\[5,8,1\],\[2,7,4\]\]
**Output:** false
**Explanation:** The above diagram represents the grid. The 8th move of the knight is not valid considering its position after the 7th move.
**Constraints:**
* `n == grid.length == grid[i].length`
* `3 <= n <= 7`
* `0 <= grid[row][col] < n * n`
* All integers in `grid` are **unique**. | null |
Python easy solution | check-knight-tour-configuration | 0 | 1 | \n\n# Code\n```\nclass Solution:\n def checkValidGrid(self, grid: List[List[int]]) -> bool:\n n = len(grid)\n size = n*n\n directions = [(2, 1), (2, -1), (-2, 1), (-2, -1), (1, 2), (1, -2), (-1, -2), (-1, 2)]\n\n def dfs(i, j, curr_size):\n if curr_size == size-1:\n return True\n \n next_position = grid[i][j]+1\n\n for d in directions:\n x, y = d\n r = x + i\n c = y + j\n if r < 0 or r >= n or c < 0 or c >= n or grid[r][c] != next_position:\n continue\n\n ans = dfs(r, c, curr_size + 1)\n if ans:\n return ans\n return False\n return dfs(0, 0, 0)\n \n``` | 2 | There is a knight on an `n x n` chessboard. In a valid configuration, the knight starts **at the top-left cell** of the board and visits every cell on the board **exactly once**.
You are given an `n x n` integer matrix `grid` consisting of distinct integers from the range `[0, n * n - 1]` where `grid[row][col]` indicates that the cell `(row, col)` is the `grid[row][col]th` cell that the knight visited. The moves are **0-indexed**.
Return `true` _if_ `grid` _represents a valid configuration of the knight's movements or_ `false` _otherwise_.
**Note** that a valid knight move consists of moving two squares vertically and one square horizontally, or two squares horizontally and one square vertically. The figure below illustrates all the possible eight moves of a knight from some cell.
**Example 1:**
**Input:** grid = \[\[0,11,16,5,20\],\[17,4,19,10,15\],\[12,1,8,21,6\],\[3,18,23,14,9\],\[24,13,2,7,22\]\]
**Output:** true
**Explanation:** The above diagram represents the grid. It can be shown that it is a valid configuration.
**Example 2:**
**Input:** grid = \[\[0,3,6\],\[5,8,1\],\[2,7,4\]\]
**Output:** false
**Explanation:** The above diagram represents the grid. The 8th move of the knight is not valid considering its position after the 7th move.
**Constraints:**
* `n == grid.length == grid[i].length`
* `3 <= n <= 7`
* `0 <= grid[row][col] < n * n`
* All integers in `grid` are **unique**. | null |
Python 2 solutions | Move Thourgh Grid | Save the steps | check-knight-tour-configuration | 0 | 1 | # Method 1\nWe save the index of steps, in our moves array, \nindex0: pos of first step in grid\nindex1: pos of second step in grid\nand so on\n\nThen we check if the moves are valid or not the absolute value of change in direction can be either (1,2) or (2,1)\notherwise its an illegal move \n```\nclass Solution:\n def checkValidGrid(self, grid: List[List[int]]) -> bool:\n if(grid[0][0] != 0 ):return False\n n = len(grid);\n moves = [ 0 for _ in range(0,n*n)]\n for i in range(0,n):\n for j in range(0,n) :\n moves[grid[i][j]] = [i,j]\n \n prev = [0,0]\n for i in range(1,n*n):\n if(abs(moves[i][0]-prev[0]) == 1 and abs(moves[i][1]-prev[1]) == 2 ):\n prev = moves[i] ;\n continue;\n elif(abs(moves[i][0]-prev[0]) == 2 and abs(moves[i][1]-prev[1]) == 1 ):\n prev = moves[i]\n continue;\n else:\n return False\n\n return True \n```\n\n\n# Method 2\n\nWe try to check if the direction of the next move is legit, and then we have to store that point and check the next for(n*n-1) times\n```\n def checkValidGrid(self, grid: List[List[int]]) -> bool:\n n = len(grid);\n #build directions\n direc=[]\n a,b = [-2,2],[-1,1]\n direc = [(x,y) for x in a for y in b]\n direc += [(x,y) for x in b for y in a]\n \n if(grid[0][0] != 0):return False\n\n prev = [0,0]\n step = 0\n for i in range(1,n*n):\n present = False\n for dir in direc:\n x = prev[0] + dir[0]\n y = prev[1] + dir[1]\n if( x>=0 and y>=0 and x<n and y<n and grid[x][y] == step+1 ):\n prev = [x,y]\n step += 1\n present = True\n break;\n if(not present ):\n return False\n return True \n \n \n\n``` | 1 | There is a knight on an `n x n` chessboard. In a valid configuration, the knight starts **at the top-left cell** of the board and visits every cell on the board **exactly once**.
You are given an `n x n` integer matrix `grid` consisting of distinct integers from the range `[0, n * n - 1]` where `grid[row][col]` indicates that the cell `(row, col)` is the `grid[row][col]th` cell that the knight visited. The moves are **0-indexed**.
Return `true` _if_ `grid` _represents a valid configuration of the knight's movements or_ `false` _otherwise_.
**Note** that a valid knight move consists of moving two squares vertically and one square horizontally, or two squares horizontally and one square vertically. The figure below illustrates all the possible eight moves of a knight from some cell.
**Example 1:**
**Input:** grid = \[\[0,11,16,5,20\],\[17,4,19,10,15\],\[12,1,8,21,6\],\[3,18,23,14,9\],\[24,13,2,7,22\]\]
**Output:** true
**Explanation:** The above diagram represents the grid. It can be shown that it is a valid configuration.
**Example 2:**
**Input:** grid = \[\[0,3,6\],\[5,8,1\],\[2,7,4\]\]
**Output:** false
**Explanation:** The above diagram represents the grid. The 8th move of the knight is not valid considering its position after the 7th move.
**Constraints:**
* `n == grid.length == grid[i].length`
* `3 <= n <= 7`
* `0 <= grid[row][col] < n * n`
* All integers in `grid` are **unique**. | null |
Simply put chessbord into an array and simulate knight's movement | check-knight-tour-configuration | 0 | 1 | # Code\n```\ndef checkValidGrid(self, grid: List[List[int]]) -> bool:\n n = len(grid)\n arr = [None] * (n*n)\n for row in range(n):\n for col in range(n):\n arr[grid[row][col]] = (row, col)\n pos = arr[0]\n if pos != (0,0):\n return False\n for i in range(1, n*n):\n a = abs(pos[0] - arr[i][0])\n b = abs(pos[1] - arr[i][1])\n if a == 1 and b == 2 or a == 2 and b == 1:\n pos = arr[i]\n else:\n return False\n return True\n``` | 1 | There is a knight on an `n x n` chessboard. In a valid configuration, the knight starts **at the top-left cell** of the board and visits every cell on the board **exactly once**.
You are given an `n x n` integer matrix `grid` consisting of distinct integers from the range `[0, n * n - 1]` where `grid[row][col]` indicates that the cell `(row, col)` is the `grid[row][col]th` cell that the knight visited. The moves are **0-indexed**.
Return `true` _if_ `grid` _represents a valid configuration of the knight's movements or_ `false` _otherwise_.
**Note** that a valid knight move consists of moving two squares vertically and one square horizontally, or two squares horizontally and one square vertically. The figure below illustrates all the possible eight moves of a knight from some cell.
**Example 1:**
**Input:** grid = \[\[0,11,16,5,20\],\[17,4,19,10,15\],\[12,1,8,21,6\],\[3,18,23,14,9\],\[24,13,2,7,22\]\]
**Output:** true
**Explanation:** The above diagram represents the grid. It can be shown that it is a valid configuration.
**Example 2:**
**Input:** grid = \[\[0,3,6\],\[5,8,1\],\[2,7,4\]\]
**Output:** false
**Explanation:** The above diagram represents the grid. The 8th move of the knight is not valid considering its position after the 7th move.
**Constraints:**
* `n == grid.length == grid[i].length`
* `3 <= n <= 7`
* `0 <= grid[row][col] < n * n`
* All integers in `grid` are **unique**. | null |
Simple BFS Traversal (Python) | check-knight-tour-configuration | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution: \n def checkValidGrid(self, grid: List[List[int]]) -> bool:\n r1,c1=0,0\n src=0\n dest=(len(grid)*len(grid))-1\n queue=collections.deque([(r1,c1,src)])\n vis=[[0 for i in range(len(grid))] for j in range(len(grid))]\n while queue:\n row,col,src=queue.popleft()\n vis[row][col]=1\n if src==dest:\n return True\n dx=[1,2,1,2,-1,-2,-1,-2]\n dy=[2,1,-2,-1,2,1,-2,-1]\n for i in range(8):\n newr=row+dx[i]\n newc=col+dy[i]\n #print(newr,newc)\n if 0<=newr<len(grid) and 0<=newc<len(grid) and vis[newr][newc]!=1:\n if grid[newr][newc]==(src+1):\n queue.append((newr,newc,src+1))\n \n return False\n``` | 1 | There is a knight on an `n x n` chessboard. In a valid configuration, the knight starts **at the top-left cell** of the board and visits every cell on the board **exactly once**.
You are given an `n x n` integer matrix `grid` consisting of distinct integers from the range `[0, n * n - 1]` where `grid[row][col]` indicates that the cell `(row, col)` is the `grid[row][col]th` cell that the knight visited. The moves are **0-indexed**.
Return `true` _if_ `grid` _represents a valid configuration of the knight's movements or_ `false` _otherwise_.
**Note** that a valid knight move consists of moving two squares vertically and one square horizontally, or two squares horizontally and one square vertically. The figure below illustrates all the possible eight moves of a knight from some cell.
**Example 1:**
**Input:** grid = \[\[0,11,16,5,20\],\[17,4,19,10,15\],\[12,1,8,21,6\],\[3,18,23,14,9\],\[24,13,2,7,22\]\]
**Output:** true
**Explanation:** The above diagram represents the grid. It can be shown that it is a valid configuration.
**Example 2:**
**Input:** grid = \[\[0,3,6\],\[5,8,1\],\[2,7,4\]\]
**Output:** false
**Explanation:** The above diagram represents the grid. The 8th move of the knight is not valid considering its position after the 7th move.
**Constraints:**
* `n == grid.length == grid[i].length`
* `3 <= n <= 7`
* `0 <= grid[row][col] < n * n`
* All integers in `grid` are **unique**. | null |
[Python 3] BFS | Iterative | check-knight-tour-configuration | 0 | 1 | ```\nclass Solution:\n def checkValidGrid(self, grid: List[List[int]]) -> bool:\n n = len(grid)\n \n q = deque( [(0, 0)] )\n res = 0\n \n while q:\n res += 1\n x, y = q.popleft()\n\n for i, j in [ (x + 1, y + 2), (x + 1, y - 2), (x - 1, y + 2), (x - 1, y - 2), (x + 2, y + 1), (x + 2, y - 1), (x - 2, y + 1), (x - 2, y - 1) ]:\n if 0 <= i < n and 0 <= j < n and grid[i][j] == grid[x][y] + 1:\n q.append( (i, j) )\n\n return res == pow(n, 2)\n``` | 4 | There is a knight on an `n x n` chessboard. In a valid configuration, the knight starts **at the top-left cell** of the board and visits every cell on the board **exactly once**.
You are given an `n x n` integer matrix `grid` consisting of distinct integers from the range `[0, n * n - 1]` where `grid[row][col]` indicates that the cell `(row, col)` is the `grid[row][col]th` cell that the knight visited. The moves are **0-indexed**.
Return `true` _if_ `grid` _represents a valid configuration of the knight's movements or_ `false` _otherwise_.
**Note** that a valid knight move consists of moving two squares vertically and one square horizontally, or two squares horizontally and one square vertically. The figure below illustrates all the possible eight moves of a knight from some cell.
**Example 1:**
**Input:** grid = \[\[0,11,16,5,20\],\[17,4,19,10,15\],\[12,1,8,21,6\],\[3,18,23,14,9\],\[24,13,2,7,22\]\]
**Output:** true
**Explanation:** The above diagram represents the grid. It can be shown that it is a valid configuration.
**Example 2:**
**Input:** grid = \[\[0,3,6\],\[5,8,1\],\[2,7,4\]\]
**Output:** false
**Explanation:** The above diagram represents the grid. The 8th move of the knight is not valid considering its position after the 7th move.
**Constraints:**
* `n == grid.length == grid[i].length`
* `3 <= n <= 7`
* `0 <= grid[row][col] < n * n`
* All integers in `grid` are **unique**. | null |
Simple Python3 Solution || =~100% Faster || Commented & Explained || Using simple {Dictionary} | check-knight-tour-configuration | 0 | 1 | \n# Approach\n1. First, create a list of all possible valid knight moves using the given "diff" list.\n2. Then, create a dictionary "new" that maps each cell number to its row and column indices.\n3. Iterate through the cell numbers from 0 to (n*n)-1, and check if the difference between the row and column indices of the current cell and the next cell is a valid knight move using the "diff" list.\n4. If the difference is not a valid knight move, return False. Otherwise, continue checking for the remaining cells.\n5. If all cells are valid knight moves, return True.\n\n# Complexity\n- Time complexity:\nO(n^2)\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def checkValidGrid(self, grid: List[List[int]]) -> bool:\n diff = [\n [2, 1],\n [1, 2],\n ]\n # based on the grid value saving the Row,Col in dictionary\n # key : [Value] ::: Grid_Number : [row,column]\n new = defaultdict(list)\n n = len(grid)\n for i in range(n):\n for j in range(n):\n new[grid[i][j]].append(i)\n new[grid[i][j]].append(j)\n\n # Must check condition Grid need to start at left top\n # A specific testcase is given to check this. \n if new[0] != [0,0]:\n return False\n \n # Remaining just check if the absolute diff of Row & Col\n # is within the range, i.e, [1,2] or [2,1]\n # If not Return False\n for i in range((n*n)-1):\n row = abs(new[i][0] - new[i+1][0])\n col = abs(new[i][1] - new[i+1][1])\n #print(row,col)\n if [row,col] not in diff:\n return False\n \n # If Everything is perfect return True\n return True\n \n \n``` | 1 | There is a knight on an `n x n` chessboard. In a valid configuration, the knight starts **at the top-left cell** of the board and visits every cell on the board **exactly once**.
You are given an `n x n` integer matrix `grid` consisting of distinct integers from the range `[0, n * n - 1]` where `grid[row][col]` indicates that the cell `(row, col)` is the `grid[row][col]th` cell that the knight visited. The moves are **0-indexed**.
Return `true` _if_ `grid` _represents a valid configuration of the knight's movements or_ `false` _otherwise_.
**Note** that a valid knight move consists of moving two squares vertically and one square horizontally, or two squares horizontally and one square vertically. The figure below illustrates all the possible eight moves of a knight from some cell.
**Example 1:**
**Input:** grid = \[\[0,11,16,5,20\],\[17,4,19,10,15\],\[12,1,8,21,6\],\[3,18,23,14,9\],\[24,13,2,7,22\]\]
**Output:** true
**Explanation:** The above diagram represents the grid. It can be shown that it is a valid configuration.
**Example 2:**
**Input:** grid = \[\[0,3,6\],\[5,8,1\],\[2,7,4\]\]
**Output:** false
**Explanation:** The above diagram represents the grid. The 8th move of the knight is not valid considering its position after the 7th move.
**Constraints:**
* `n == grid.length == grid[i].length`
* `3 <= n <= 7`
* `0 <= grid[row][col] < n * n`
* All integers in `grid` are **unique**. | null |
Sorting + Preprocessing + DFS Speed up DFS to squeeze by weird time cutoff | the-number-of-beautiful-subsets | 0 | 1 | Given n <= 20, iterating all possible subsets and checking for violations seem reasonable, but checking each pair for every subset results in a complexity of O(2^n * n ^ 2). Which TLE\'s when n == 19.\n\nSubsequently, we might instead try DFS, which not only prunes many incorrect subsets, when adding an element to existing valid subset, we only need to check up to n other elements. Which has complexity O(2^n * n), which TLE\'s when n == 20.\n\nLastly, we can take advantage of the property of difference, using sorting and preprocessing to achieve near constant time when adding new elements to a valid subset. O(2^n * max banned range). \nThe given testsets seem to have all unique values which means that max banned range == 1. O(2^n) passes all test cases\n# Code\n```\nclass Solution:\n def beautifulSubsets(self, nums: List[int], k: int) -> int:\n nums.sort()\n banned_range = []\n n = len(nums)\n for i in range(len(nums)):\n l = 100\n r = -1\n for j in range(0,i):\n if nums[i] == nums[j] + k:\n l = min(l,j)\n r = max(r,j)\n banned_range.append([l,r])\n \n tot = [0]\n def dfs(l):\n if n == len(l):\n return\n else:\n left,right = banned_range[len(l)]\n if not any(l[left:right + 1]):\n tot[0] += 1\n dfs(l + [True]) \n dfs(l + [False])\n dfs([])\n return tot[0] \n \n``` | 1 | You are given an array `nums` of positive integers and a **positive** integer `k`.
A subset of `nums` is **beautiful** if it does not contain two integers with an absolute difference equal to `k`.
Return _the number of **non-empty beautiful** subsets of the array_ `nums`.
A **subset** of `nums` is an array that can be obtained by deleting some (possibly none) elements from `nums`. Two subsets are different if and only if the chosen indices to delete are different.
**Example 1:**
**Input:** nums = \[2,4,6\], k = 2
**Output:** 4
**Explanation:** The beautiful subsets of the array nums are: \[2\], \[4\], \[6\], \[2, 6\].
It can be proved that there are only 4 beautiful subsets in the array \[2,4,6\].
**Example 2:**
**Input:** nums = \[1\], k = 1
**Output:** 1
**Explanation:** The beautiful subset of the array nums is \[1\].
It can be proved that there is only 1 beautiful subset in the array \[1\].
**Constraints:**
* `1 <= nums.length <= 20`
* `1 <= nums[i], k <= 1000` | null |
Why is this giving wrong answer Python | the-number-of-beautiful-subsets | 0 | 1 | I am testing whether the set is valid for all the subset. I know that it will give TLE But instead it is giving wrong answer what is the problem in the code.\n\n```\nclass Solution:\n def beautifulSubsets(self, nums: List[int], k: int) -> int:\n n = len(nums)\n val = pow(2,n)-1\n res = 0\n invalidset = defaultdict(int)\n while(val):\n x = val ;\n invalidset.clear()\n i = 0\n while(i<n):\n if(x&1 ):\n if(invalidset[ nums[i]-k ] == 0 and invalidset[ k-nums[i] ] == 0 ) : \n invalidset[nums[i]] += 1;\n else:break;\n x >>=1\n i+=1\n if(i==n): res += 1\n val -=1\n \n return res;\n```\n\n```\nWrong Answer\nRuntime: 5385 ms\nInput\n nums = [15,6,3,25,14,29,21,16,28,23,11,9,4,30,24,12,26,1,27,18]\n k = 7\nOutput \n 172799\nExpected \n 163839\n``` | 1 | You are given an array `nums` of positive integers and a **positive** integer `k`.
A subset of `nums` is **beautiful** if it does not contain two integers with an absolute difference equal to `k`.
Return _the number of **non-empty beautiful** subsets of the array_ `nums`.
A **subset** of `nums` is an array that can be obtained by deleting some (possibly none) elements from `nums`. Two subsets are different if and only if the chosen indices to delete are different.
**Example 1:**
**Input:** nums = \[2,4,6\], k = 2
**Output:** 4
**Explanation:** The beautiful subsets of the array nums are: \[2\], \[4\], \[6\], \[2, 6\].
It can be proved that there are only 4 beautiful subsets in the array \[2,4,6\].
**Example 2:**
**Input:** nums = \[1\], k = 1
**Output:** 1
**Explanation:** The beautiful subset of the array nums is \[1\].
It can be proved that there is only 1 beautiful subset in the array \[1\].
**Constraints:**
* `1 <= nums.length <= 20`
* `1 <= nums[i], k <= 1000` | null |
[C++, Java, Python] Evolve Brute Force to DP | Explained 7 Approaches | the-number-of-beautiful-subsets | 1 | 1 | For every element $x$, we have a choice to take or not take it to make the subset. So, maximum number of possible subsets can be $2^n$.\n\n---\n\n***\u2714\uFE0F Solution - I (Recursion + Backtracking) [Accepted]***\n\nLet\'s say we have to decide to take or not take the element at index $i$. We can recursively make two calls, one for not taking it and another for taking it. Since, question says subset should be non-empty, $1$ is subtracted from final result.\n\nTo take the element at index $i$, the elements $nums[i] \\pm k$ should not be taken in the subset before. Frequency map can be made to keep the track of elements taken till now.\n\n```C++ []\nclass Solution {\nprivate:\n int _beautifulSubsets(vector<int>& nums, int k, unordered_map<int, int>& freq, int i) {\n if (i == nums.size()) { // base case\n return 1;\n }\n int result = _beautifulSubsets(nums, k, freq, i + 1); // nums[i] not taken\n if (!freq[nums[i] - k] && !freq[nums[i] + k]) { // check if we can take nums[i]\n freq[nums[i]]++;\n result += _beautifulSubsets(nums, k, freq, i + 1); // nums[i] taken\n freq[nums[i]]--;\n }\n return result;\n }\n \npublic:\n int beautifulSubsets(vector<int>& nums, int k) {\n unordered_map<int, int> freq;\n return _beautifulSubsets(nums, k, freq, 0) - 1; // -1 for empty subset\n }\n};\n```\n```Java []\nclass Solution {\n private HashMap<Integer, Integer> freq;\n\n private int beautifulSubsets(int[] nums, int k, int i) {\n if (i == nums.length) { // base case\n return 1;\n }\n int result = beautifulSubsets(nums, k, i + 1); // nums[i] not taken\n if (!freq.containsKey(nums[i] - k) && !freq.containsKey(nums[i] + k)) { // check if we can take nums[i]\n freq.put(nums[i], freq.getOrDefault(nums[i], 0) + 1);\n result += beautifulSubsets(nums, k, i + 1); // nums[i] taken\n freq.put(nums[i], freq.get(nums[i]) - 1);\n if (freq.get(nums[i]) == 0) {\n freq.remove(nums[i]);\n }\n }\n return result;\n }\n\n public int beautifulSubsets(int[] nums, int k) {\n freq = new HashMap<Integer, Integer>();\n return beautifulSubsets(nums, k, 0) - 1; // -1 for empty subset\n }\n}\n```\n```Python []\nclass Solution:\n def beautifulSubsets(self, nums: List[int], k: int) -> int:\n freq = {}\n def f(i: int) -> int:\n if i == len(nums): # base case\n return 1\n result = f(i + 1) # nums[i] not taken\n if not nums[i] - k in freq and not nums[i] + k in freq: # check if we can take nums[i]\n freq[nums[i]] = freq[nums[i]] + 1 if nums[i] in freq else 1\n result += f(i + 1) # nums[i] taken\n freq[nums[i]] -= 1\n if freq[nums[i]] == 0:\n del freq[nums[i]]\n return result\n return f(0) - 1 # -1 for empty subset\n```\n\nSince, maximum no. of subsets can be $2^n$, time complexity will be $O(2^n)$.\nRecursion stack space and frequency map will make space complexity $O(n)$.\n\n- Time Complexity: $$O(2^n)$$\n- Space Complexity: $$O(n)$$\n\n---\n\n***\u2714\uFE0F Solution - II (Recursion + Backtracking) [Accepted]***\n\nSince time complexity is already $O(2^n)$, sorting the array beforehand, for $O(n \\log n)$, will not change it. Also, if we know that before the current index $i$, there were no larger elements, then we have to check just for the existence of $nums[i] - k$, and not for $nums[i] + k$. Therefore, we sort the array.\n\nTime/Space Complexities will be same as before but LeetCode\'s Online Judge shows difference of $1200 ms$ and $800 ms$, so I thought to mention it too, as number of operations will be less.\n\n```C++ []\nclass Solution {\nprivate:\n int _beautifulSubsets(vector<int>& nums, int k, unordered_map<int, int>& freq, int i) {\n if (i == nums.size()) { // base case\n return 1;\n }\n int result = _beautifulSubsets(nums, k, freq, i + 1); // nums[i] not taken\n if (!freq[nums[i] - k]) { // check if we can take nums[i]\n freq[nums[i]]++;\n result += _beautifulSubsets(nums, k, freq, i + 1); // nums[i] taken\n freq[nums[i]]--;\n }\n return result;\n }\n \npublic:\n int beautifulSubsets(vector<int>& nums, int k) {\n unordered_map<int, int> freq;\n sort(nums.begin(), nums.end());\n return _beautifulSubsets(nums, k, freq, 0) - 1; // -1 for empty subset\n }\n};\n```\n```Java []\nclass Solution {\n private HashMap<Integer, Integer> freq;\n\n private int beautifulSubsets(int[] nums, int k, int i) {\n if (i == nums.length) { // base case\n return 1;\n }\n int result = beautifulSubsets(nums, k, i + 1); // nums[i] not taken\n if (!freq.containsKey(nums[i] - k)) { // check if we can take nums[i]\n freq.put(nums[i], freq.getOrDefault(nums[i], 0) + 1);\n result += beautifulSubsets(nums, k, i + 1); // nums[i] taken\n freq.put(nums[i], freq.get(nums[i]) - 1);\n if (freq.get(nums[i]) == 0) {\n freq.remove(nums[i]);\n }\n }\n return result;\n }\n\n public int beautifulSubsets(int[] nums, int k) {\n freq = new HashMap<Integer, Integer>();\n Arrays.sort(nums);\n return beautifulSubsets(nums, k, 0) - 1; // -1 for empty subset\n }\n}\n```\n```Python []\nclass Solution:\n def beautifulSubsets(self, nums: List[int], k: int) -> int:\n freq = {}\n nums.sort()\n def f(i: int) -> int:\n if i == len(nums): # base case\n return 1\n result = f(i + 1) # nums[i] not taken\n if not nums[i] - k in freq: # check if we can take nums[i]\n freq[nums[i]] = freq[nums[i]] + 1 if nums[i] in freq else 1\n result += f(i + 1) # nums[i] taken\n freq[nums[i]] -= 1\n if freq[nums[i]] == 0:\n del freq[nums[i]]\n return result\n return f(0) - 1 # -1 for empty subset\n```\n\n- Time Complexity: $$O(2^n)$$\n- Space Complexity: $$O(n)$$\n\n---\n\n***\u2714\uFE0F Solution - III (Recursion Optimized) [Accepted]***\n\nIn the above approach, we are trying to make every possible subset. The reason why we can\'t directly return $2^n$ without going to every subset, is that we don\'t know how many of them will satisfy the condition of *beautiful*. But what if there are no elements in the array whose difference is $k$?\n\n**Example 1**\nSuppose $nums = [1, 3, 5, 7]$, $k = 1$. We have differences of $2, 4, 6$ but never $1$. So, if we knew this before, we could have directly returned $2^n - 1 = 15$, ($-1$ for empty subset) without actually going to every subset by backtracking.\n\n**Example 2**\nNow, suppose $nums = [1, 2, 3, 4]$, $k = 2$. Difference of $2$ can be made by $4-2$, and $3-1$. That means $[2, 4]$ can\'t be in the same subset. Also, $[1, 3]$ can\'t be in the same subset. So, how can we write the answer if we knew this before?\nWe have two sets,\n$s_1 = [1, 3]$\n$s_2 = [2, 4]$\nWe can calculate number of *beautiful* subsets for $s_1$ and $s_2$ separately because the choices in $s_1$ is independent of $s_2$ and vice versa. Let the answers be $f(s_1)$ and $f(s_2)$.\nFor final answer, we can multiply both of them because there is no pair $(x_1,x_2)$ such that $x_1 \\in s_1$, $x_2 \\in s_2$ and $|x_1-x_2| = k$.\n$f(nums) = f(s_1) \\times f(s_2) - 1$ ($-1$ for empty subset)\n\n**Example 3**\nSo, one thing we can do is to separate the given array into these subsets, such that there is no pair $(x_1,x_2)$, $x_1$ and $x_2$ belonging to different subsets and $|x_1-x_2| = k$.\nIn one subset, there will be elemenets of the form: $[x, x + k, x + 2k, ..., x + \\lambda k]$.\nIf we take $x$, then we can\'t take $x+k$, but can take $x+2k$ and so on.\nAll these elements have common remainder when divided by $k$. So we can make these subsets by separating on the basis of remainder ($\\mod k$ ).\nFor example, $nums = [1, 2, 3, 4, 5, 6]$, $k = 2$.\n- $s_1$ : $nums[i] \\enspace \\% \\enspace k = 0$ : $[2,4,6]$\n- $s_2$ : $nums[i] \\enspace \\% \\enspace k = 1$ : $[1,3,5]$\n\nNote that all $x+\\lambda k$ might not be available in $s_1$. For example, there might be a possibility where $x+k$ is not present in $nums$ at all so subset will look like $[x, x+2k, ...]$.\n\n**Example 4**\nNow, suppose $nums = [5, 5, 5, 7, 7]$, $k = 2$. We can\'t take $[5, 7]$ in the same subset. Representing $s_1$ in $\\{value: frequency\\}$ form\n$s_1 = [5: 3, 7: 2]$\nLet\'s say $f(i)$ calculates number of beautiful subsets in $s_1$ starting from index $i$. We want to calculate $f(0)$.\n\n- When $i = 0$, we are at $5$, there are two options, whether we skip it or take it. There are $2^3$ ways, out of which in $2^3-1=7$ ways, we take atleast one $5$, and one way where we skip $5$.\n$take_5=7$, $skip_5=1$\n- Now, next element at $i + 1$ is $7=5+2$, so we can\'t take it if we took $5$. Therefore, number of ways of taking $5$ will be $take_5 \\times f(i + 2)$.\n- Number of ways of skipping $5$ will be $skip_5 \\times f(i + 1)$.\n- $take_{s[i]} = 2 ^ {frequency(s[i])} - 1$\n- $skip_{s[i]} = 1$\n- $f(i) = take_{s[i]} \\times f(i + 2) + skip_{s[i]} \\times f(i + 1)$\n\n```C++ []\nclass Solution {\nprivate:\n int beautifulSubsets(vector<pair<int, int>>& s, int n, int k, int i) {\n if (i == n) {\n return 1;\n }\n int skip = beautifulSubsets(s, n, k, i + 1); // 1 * f(i + 1)\n int take = (1 << s[i].second) - 1; // take s[i]\n if (i + 1 < n && s[i + 1].first - s[i].first == k) { // next number is s[i] + k\n take *= beautifulSubsets(s, n, k, i + 2);\n } else {\n take *= beautifulSubsets(s, n, k, i + 1);\n }\n return skip + take;\n }\n\npublic:\n int beautifulSubsets(vector<int>& nums, int k) {\n int result = 1;\n map<int, map<int, int>> freq;\n // {remainder : {num : frequency}}\n // map is sorted based on num to get subset of form [x, x + k, x + 2k, ...]\n for (int& x: nums) { // splitting subsets based on remainder and calculating frequency\n freq[x % k][x]++;\n }\n for (auto& fr: freq) { // loop for s1, s2, ...\n vector<pair<int, int>> s(fr.second.begin(), fr.second.end());\n result *= beautifulSubsets(s, s.size(), k, 0); // f(s1) * f(s2) ...\n }\n return result - 1; // -1 for empty subset\n }\n};\n```\n```Java []\nclass Solution {\n int[][] s;\n\n private void mapToList(Map<Integer, Integer> fr) {\n s = new int[fr.size()][2];\n int i = 0;\n for (Map.Entry<Integer, Integer> x: fr.entrySet()) {\n s[i][0] = x.getKey();\n s[i][1] = x.getValue();\n i++;\n }\n }\n\n private int beautifulSubsets(int n, int k, int i) {\n if (i == n) {\n return 1;\n }\n int skip = beautifulSubsets(n, k, i + 1); // 1 * f(i + 1)\n int take = (1 << s[i][1]) - 1; // take s[i]\n if (i + 1 < n && s[i + 1][0] - s[i][0] == k) { // next number is s[i] + k\n take *= beautifulSubsets(n, k, i + 2);\n } else {\n take *= beautifulSubsets(n, k, i + 1);\n }\n return skip + take;\n }\n\n public int beautifulSubsets(int[] nums, int k) {\n int result = 1;\n Map<Integer, Map<Integer, Integer>> freq = new TreeMap<>();\n // {remainder : {num : frequency}}\n // map is sorted based on num to get subset of form [x, x + k, x + 2k, ...]\n for (int x: nums) { // splitting subsets based on remainder and calculating frequency\n Map<Integer, Integer> fr = freq.getOrDefault(x % k, new TreeMap<>());\n fr.put(x, fr.getOrDefault(x, 0) + 1);\n freq.put(x % k, fr);\n }\n for (Map fr: freq.values()) { // loop for s1, s2, ...\n mapToList(fr);\n result *= beautifulSubsets(s.length, k, 0); // f(s1) * f(s2) ...\n }\n return result - 1; // -1 for empty subset\n }\n}\n```\n```Python []\nclass Solution:\n def beautifulSubsets(self, nums: List[int], k: int) -> int:\n result = 1\n freq = {}\n # {remainder : {num : frequency}}\n for x in nums:\n if not x % k in freq:\n freq[x % k] = {}\n if not x in freq[x % k]:\n freq[x % k][x] = 0\n freq[x % k][x] += 1\n for fr in freq.values(): # loop for s1, s2, ...\n s = sorted([[k, v] for k, v in fr.items()])\n def f(i: int) -> int:\n if i == len(s):\n return 1\n skip = f(i + 1) # 1 * f(i + 1)\n take = 2 ** s[i][1] - 1 # take s[i]\n if i + 1 < len(s) and s[i + 1][0] - s[i][0] == k: # next number is s[i] + k\n take *= f(i + 2)\n else:\n take *= f(i + 1)\n return skip + take\n result *= f(0) # f(s1) * f(s2) ...\n return result - 1 # -1 for empty subset\n```\n\nSince map is sorted, it is implemented by Self Balancing BST. Insert operation is $O(\\log n)$, it will take $O(n \\log n)$ to make map. There can be $k$ different remainders, meaning maximum $k$ subset splits.\nWorst case hits when remainder is same for all numbers (i.e., all comes in same subset $s_1$) and no number is repeated ($frequency = 1$), time is still $O(2^n)$.\n\n- Time Complexity: $$O(n \\log n + 2^n) = O(2^n)$$\n- Space Complexity: $$O(n + k)$$\n\n---\n\n***\u2714\uFE0F Solution - IV (DP: Recursion + Memoization) [Accepted]***\n\n$f(i)$ calculates number of beautiful subsets in $s$ starting from index $i$. Memoize the function $f(i)$.\n\n```C++ []\nclass Solution {\nprivate:\n int beautifulSubsets(vector<pair<int, int>>& s, int n, int k, int i, vector<int>& count) {\n if (i == n) {\n return 1;\n }\n if (count[i] != -1) {\n return count[i];\n }\n int skip = beautifulSubsets(s, n, k, i + 1, count);\n int take = (1 << s[i].second) - 1;\n if (i + 1 < n && s[i + 1].first - s[i].first == k) {\n take *= beautifulSubsets(s, n, k, i + 2, count);\n } else {\n take *= beautifulSubsets(s, n, k, i + 1, count);\n }\n return count[i] = skip + take;\n }\n\npublic:\n int beautifulSubsets(vector<int>& nums, int k) {\n int result = 1;\n map<int, map<int, int>> freq;\n for (int& x: nums) {\n freq[x % k][x]++;\n }\n for (auto& fr: freq) {\n vector<pair<int, int>> s(fr.second.begin(), fr.second.end());\n vector<int> count(s.size(), -1);\n result *= beautifulSubsets(s, s.size(), k, 0, count);\n }\n return result - 1;\n }\n};\n```\n```Java []\nclass Solution {\n int[][] s;\n int[] count;\n\n private void mapToList(Map<Integer, Integer> fr) {\n s = new int[fr.size()][2];\n int i = 0;\n for (Map.Entry<Integer, Integer> x: fr.entrySet()) {\n s[i][0] = x.getKey();\n s[i][1] = x.getValue();\n i++;\n }\n }\n\n private int beautifulSubsets(int n, int k, int i) {\n if (i == n) {\n return 1;\n }\n if (count[i] != -1) {\n return count[i];\n }\n int skip = beautifulSubsets(n, k, i + 1);\n int take = (1 << s[i][1]) - 1;\n if (i + 1 < n && s[i + 1][0] - s[i][0] == k) {\n take *= beautifulSubsets(n, k, i + 2);\n } else {\n take *= beautifulSubsets(n, k, i + 1);\n }\n return count[i] = skip + take;\n }\n\n public int beautifulSubsets(int[] nums, int k) {\n int result = 1;\n Map<Integer, Map<Integer, Integer>> freq = new TreeMap<>();\n for (int x: nums) {\n Map<Integer, Integer> fr = freq.getOrDefault(x % k, new TreeMap<>());\n fr.put(x, fr.getOrDefault(x, 0) + 1);\n freq.put(x % k, fr);\n }\n for (Map fr: freq.values()) {\n mapToList(fr);\n count = new int[fr.size()];\n Arrays.fill(count, -1);\n result *= beautifulSubsets(s.length, k, 0);\n }\n return result - 1;\n }\n}\n```\n```Python []\nclass Solution:\n def beautifulSubsets(self, nums: List[int], k: int) -> int:\n result = 1\n freq = {}\n for x in nums:\n if not x % k in freq:\n freq[x % k] = {}\n if not x in freq[x % k]:\n freq[x % k][x] = 0\n freq[x % k][x] += 1\n for fr in freq.values():\n s = sorted([[k, v] for k, v in fr.items()])\n @cache\n def f(i: int) -> int:\n if i == len(s):\n return 1\n skip = f(i + 1)\n take = 2 ** s[i][1] - 1\n if i + 1 < len(s) and s[i + 1][0] - s[i][0] == k:\n take *= f(i + 2)\n else:\n take *= f(i + 1)\n return skip + take\n result *= f(0)\n return result - 1\n```\n\nMaking map is still $O(n \\log n)$. Calculating answer for one subset will be equal to number of states in DP which makes it $O(n)$.\n\n- Time Complexity: $$O(n \\log n + n) = O(n \\log n)$$\n- Space Complexity: $$O(n + k)$$\n\n---\n\n***\u2714\uFE0F Solution - V (Iterative DP) [Accepted]***\n\nChanging recursion to iteration. To calculate $f(i)$, we need to know $f(i + 1)$ and $f(i + 2)$. So DP should be filled right to left.\n\n```C++ []\nclass Solution {\npublic:\n int beautifulSubsets(vector<int>& nums, int k) {\n int result = 1;\n map<int, map<int, int>> freq;\n for (int& x: nums) {\n freq[x % k][x]++;\n }\n for (auto& fr: freq) {\n int n = fr.second.size();\n vector<pair<int, int>> s(fr.second.begin(), fr.second.end());\n vector<int> count(n + 1);\n count[n] = 1;\n for (int i = n - 1; i >= 0; i--) {\n int skip = count[i + 1];\n int take = (1 << s[i].second) - 1;\n if (i + 1 < n && s[i + 1].first - s[i].first == k) {\n take *= count[i + 2];\n } else {\n take *= count[i + 1];\n }\n count[i] = skip + take;\n }\n result *= count[0];\n }\n return result - 1;\n }\n};\n```\n```Java []\nclass Solution {\n private int[][] mapToList(Map<Integer, Integer> fr) {\n int[][] s = new int[fr.size()][2];\n int i = 0;\n for (Map.Entry<Integer, Integer> x: fr.entrySet()) {\n s[i][0] = x.getKey();\n s[i][1] = x.getValue();\n i++;\n }\n return s;\n }\n\n public int beautifulSubsets(int[] nums, int k) {\n int result = 1;\n Map<Integer, Map<Integer, Integer>> freq = new TreeMap<>();\n for (int x: nums) {\n Map<Integer, Integer> fr = freq.getOrDefault(x % k, new TreeMap<>());\n fr.put(x, fr.getOrDefault(x, 0) + 1);\n freq.put(x % k, fr);\n }\n for (Map fr: freq.values()) {\n int n = fr.size();\n int[][] s = mapToList(fr);\n int[] count = new int[n + 1];\n count[n] = 1;\n for (int i = n - 1; i >= 0; i--) {\n int skip = count[i + 1];\n int take = (1 << s[i][1]) - 1;\n if (i + 1 < n && s[i + 1][0] - s[i][0] == k) {\n take *= count[i + 2];\n } else {\n take *= count[i + 1];\n }\n count[i] = skip + take;\n }\n result *= count[0];\n }\n return result - 1;\n }\n}\n```\n```Python []\nclass Solution:\n def beautifulSubsets(self, nums: List[int], k: int) -> int:\n result = 1\n freq = {}\n for x in nums:\n if not x % k in freq:\n freq[x % k] = {}\n if not x in freq[x % k]:\n freq[x % k][x] = 0\n freq[x % k][x] += 1\n for fr in freq.values():\n n = len(fr)\n s = sorted([[k, v] for k, v in fr.items()])\n count = [0] * (n + 1)\n count[n] = 1\n for i in range(n - 1, -1, -1):\n skip = count[i + 1]\n take = 2 ** s[i][1] - 1\n if i + 1 < n and s[i + 1][0] - s[i][0] == k:\n take *= count[i + 2]\n else:\n take *= count[i + 1]\n count[i] = skip + take\n result *= count[0]\n return result - 1\n```\n\n- Time Complexity: $$O(n \\log n)$$\n- Space Complexity: $$O(n + k)$$\n\n---\n\n***\u2714\uFE0F Solution - VI (Iterative DP Space Optimized) [Accepted]***\n\nTo calculate $f(i)$, we only need $f(i + 1)$ and $f(i + 2)$.\nStoring $f(i + 3)$ onwards is useless.\n- $curr = f(i)$\n- $next_1 = f(i + 1)$\n- $next_2 = f(i + 2)$\n\n```C++ []\nclass Solution {\npublic:\n int beautifulSubsets(vector<int>& nums, int k) {\n int result = 1;\n map<int, map<int, int>> freq;\n for (int& x: nums) {\n freq[x % k][x]++;\n }\n for (auto& fr: freq) {\n int n = fr.second.size(), curr, next1 = 1, next2;\n vector<pair<int, int>> s(fr.second.begin(), fr.second.end());\n for (int i = n - 1; i >= 0; i--) {\n int skip = next1;\n int take = (1 << s[i].second) - 1;\n if (i + 1 < n && s[i + 1].first - s[i].first == k) {\n take *= next2;\n } else {\n take *= next1;\n }\n curr = skip + take;\n next2 = next1; next1 = curr;\n }\n result *= curr;\n }\n return result - 1;\n }\n};\n```\n```Java []\nclass Solution {\n private int[][] mapToList(Map<Integer, Integer> fr) {\n int[][] s = new int[fr.size()][2];\n int i = 0;\n for (Map.Entry<Integer, Integer> x: fr.entrySet()) {\n s[i][0] = x.getKey();\n s[i][1] = x.getValue();\n i++;\n }\n return s;\n }\n\n public int beautifulSubsets(int[] nums, int k) {\n int result = 1;\n Map<Integer, Map<Integer, Integer>> freq = new TreeMap<>();\n for (int x: nums) {\n Map<Integer, Integer> fr = freq.getOrDefault(x % k, new TreeMap<>());\n fr.put(x, fr.getOrDefault(x, 0) + 1);\n freq.put(x % k, fr);\n }\n for (Map fr: freq.values()) {\n int n = fr.size(), curr = 1, next1 = 1, next2 = 0;\n int[][] s = mapToList(fr);\n for (int i = n - 1; i >= 0; i--) {\n int skip = next1;\n int take = (1 << s[i][1]) - 1;\n if (i + 1 < n && s[i + 1][0] - s[i][0] == k) {\n take *= next2;\n } else {\n take *= next1;\n }\n curr = skip + take;\n next2 = next1; next1 = curr;\n }\n result *= curr;\n }\n return result - 1;\n }\n}\n```\n```Python []\nclass Solution:\n def beautifulSubsets(self, nums: List[int], k: int) -> int:\n result = 1\n freq = {}\n for x in nums:\n if not x % k in freq:\n freq[x % k] = {}\n if not x in freq[x % k]:\n freq[x % k][x] = 0\n freq[x % k][x] += 1\n for fr in freq.values():\n n, curr, next1, next2 = len(fr), 1, 1, 0\n s = sorted([[k, v] for k, v in fr.items()])\n for i in range(n - 1, -1, -1):\n skip = next1\n take = 2 ** s[i][1] - 1\n if i + 1 < n and s[i + 1][0] - s[i][0] == k:\n take *= next2\n else:\n take *= next1\n curr = skip + take\n next2, next1 = next1, curr\n result *= curr\n return result - 1\n```\n\n- Time Complexity: $$O(n \\log n)$$\n- Space Complexity: $$O(n + k)$$\n\n---\n\n***\u2714\uFE0F Solution - VII (Iterative DP: Forward) [Accepted]***\n\nIn previous approaches, we were calculating in reverse direction (right to left) of $s$. Due to this, we needed to convert sorted map to array first. It can be avoided if we traverse left to right.\n\n```C++ []\nclass Solution {\npublic:\n int beautifulSubsets(vector<int>& nums, int k) {\n int result = 1;\n map<int, map<int, int>> freq;\n for (int& x: nums) {\n freq[x % k][x]++;\n }\n for (auto& fr: freq) {\n int prevNum = -k, prev2, prev1 = 1, curr;\n for (auto& [num, f]: fr.second) {\n int skip = prev1;\n int take = ((1 << f) - 1) * (num - prevNum == k ? prev2 : prev1);\n curr = skip + take;\n prev2 = prev1; prev1 = curr;\n prevNum = num;\n }\n result *= curr;\n }\n return result - 1;\n }\n};\n```\n```Java []\nclass Solution {\n public int beautifulSubsets(int[] nums, int k) {\n int result = 1;\n Map<Integer, Map<Integer, Integer>> freq = new TreeMap<>();\n for (int x: nums) {\n Map<Integer, Integer> fr = freq.getOrDefault(x % k, new TreeMap<>());\n fr.put(x, fr.getOrDefault(x, 0) + 1);\n freq.put(x % k, fr);\n }\n for (Map<Integer, Integer> fr: freq.values()) {\n int prevNum = -k, prev2 = 0, prev1 = 1, curr = 1;\n for (Map.Entry<Integer, Integer> entry: fr.entrySet()) {\n int num = entry.getKey(), f = entry.getValue();\n int skip = prev1;\n int take = ((1 << f) - 1) * (num - prevNum == k ? prev2 : prev1);\n curr = skip + take;\n prev2 = prev1; prev1 = curr;\n prevNum = num;\n }\n result *= curr;\n }\n return result - 1;\n }\n}\n```\n```Python []\nclass Solution:\n def beautifulSubsets(self, nums: List[int], k: int) -> int:\n result = 1\n freq = {}\n for x in nums:\n if not x % k in freq:\n freq[x % k] = {}\n if not x in freq[x % k]:\n freq[x % k][x] = 0\n freq[x % k][x] += 1\n for fr in freq.values():\n prevNum, curr, prev1, prev2 = -k, 1, 1, 0\n for num in sorted(fr):\n skip = prev1\n take = (2 ** fr[num] - 1) * (prev2 if num - prevNum == k else prev1)\n curr = skip + take\n prev2, prev1 = prev1, curr\n prevNum = num\n result *= curr\n return result - 1\n```\n\n- Time Complexity: $O(n \\log n)$\n- Space Complexity: $O(n + k)$\n\n---\n\nFor any mistakes / suggestions / questions, please do comment below \uD83D\uDC47\nUpvote if helps! | 63 | You are given an array `nums` of positive integers and a **positive** integer `k`.
A subset of `nums` is **beautiful** if it does not contain two integers with an absolute difference equal to `k`.
Return _the number of **non-empty beautiful** subsets of the array_ `nums`.
A **subset** of `nums` is an array that can be obtained by deleting some (possibly none) elements from `nums`. Two subsets are different if and only if the chosen indices to delete are different.
**Example 1:**
**Input:** nums = \[2,4,6\], k = 2
**Output:** 4
**Explanation:** The beautiful subsets of the array nums are: \[2\], \[4\], \[6\], \[2, 6\].
It can be proved that there are only 4 beautiful subsets in the array \[2,4,6\].
**Example 2:**
**Input:** nums = \[1\], k = 1
**Output:** 1
**Explanation:** The beautiful subset of the array nums is \[1\].
It can be proved that there is only 1 beautiful subset in the array \[1\].
**Constraints:**
* `1 <= nums.length <= 20`
* `1 <= nums[i], k <= 1000` | null |
✔💯 DAY 362 || [JAVA/C++/PYTHON] || 100% || EXPLAINED || INTUTION || TC 0(N) | the-number-of-beautiful-subsets | 1 | 1 | \n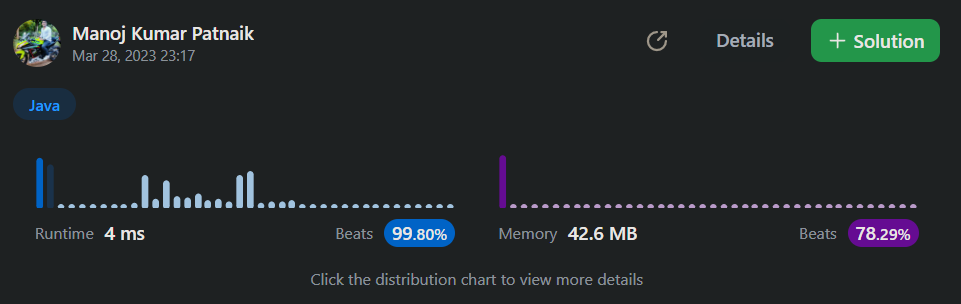\n# UPVOTE PLS\n\n# Intuition & Approach\n<!-- Describe your first thoughts on how to solve this problem. -->\n##### \u2022\tThe goal is to count the number of subsets of an integer array nums that satisfy a certain condition. The condition is that for any two distinct elements a and b in a subset, the absolute difference |a-b| should be exactly equal to k.\n##### \u2022\tThe code works by first creating a HashMap called frequencyMap that maps each number in the array to its frequency. Then it loops through each number in frequencyMap, and for each number it checks if there is another number in the map that is k less than it. If there is not, then it skips to the next number.\n##### \u2022\tIf there is another number that is k less than the current number, then the code finds all other numbers that are k apart from each other, and creates a list of their frequencies. It then uses this list to calculate the number of subsets that satisfy the condition.\n##### \u2022\tThe calculation is done using two variables, subsetWithout and subsetWith. subsetWithout keeps track of the number of subsets that do not include any of the k apart numbers, while subsetWith keeps track of the number of subsets that include at least one of the k apart numbers. The final answer is the product of all the subsetWith and subsetWithout values for each set of k apart numbers, plus 1 to account for the empty subset.\n##### \u2022\tThe code then repeats this process for each number in the array, and multiplies the results together to get the final answer. The final answer is then subtracted by 1 to account for the empty subset.\n\n\n\n# Code\n```java []\n public int beautifulSubsets(int[] nums, int k) {\n Map<Integer, Integer> frequencyMap = new HashMap<>();\n for (int num: nums) {\n frequencyMap.put(num, frequencyMap.getOrDefault(num, 0) + 1);\n }\n int result = 1;\n for (Map.Entry<Integer, Integer> entry: frequencyMap.entrySet()) {\n int key = entry.getKey();\n if (!frequencyMap.containsKey(key - k)) {\n if (!frequencyMap.containsKey(key + k)) {\n result *= (1 << frequencyMap.get(key));\n continue;\n }\n List<Integer> kDiffFrequencies = new ArrayList<>();\n kDiffFrequencies.add(frequencyMap.get(key));\n while (frequencyMap.containsKey(key + k)) {\n key += k;\n kDiffFrequencies.add(frequencyMap.get(key));\n }\n int subsetWithout = 0, subsetWith = 0;\n for (int frequency: kDiffFrequencies) {\n subsetWithout = subsetWith + subsetWithout;\n subsetWith = ((1 << frequency) - 1) * ((subsetWithout - subsetWith) + 1);\n }\n result *= (subsetWith + subsetWithout + 1);\n }\n }\n return result - 1;\n }\n```\n```c++ []\n int beautifulSubsets(vector<int>& nums, int k) {\n unordered_map<int, int> frequencyMap;\n for (int num : nums) {\n frequencyMap[num]++;\n }\n\n int result = 1;\n for (auto entry : frequencyMap) {\n int key = entry.first;\n if (!frequencyMap.count(key - k)) {\n if (!frequencyMap.count(key + k)) {\n result *= (1 << frequencyMap[key]);\n continue;\n }\n\n vector<int> kDiffFrequencies;\n kDiffFrequencies.push_back(frequencyMap[key]);\n while (frequencyMap.count(key + k)) {\n key += k;\n kDiffFrequencies.push_back(frequencyMap[key]);\n }\n\n int subsetWithout = 0, subsetWith = 0;\n for (int frequency : kDiffFrequencies) {\n subsetWithout = subsetWith + subsetWithout;\n subsetWith = ((1 << frequency) - 1) * ((subsetWithout - subsetWith) + 1);\n }\n\n result *= (subsetWith + subsetWithout + 1);\n }\n }\n\n return result - 1;\n }\n```\n```python []\n def beautifulSubsets(self, nums: List[int], k: int) -> int:\n frequencyMap = {}\n for num in nums:\n frequencyMap[num] = frequencyMap.get(num, 0) + 1\n\n result = 1\n for key, value in frequencyMap.items():\n if key - k not in frequencyMap:\n if key + k not in frequencyMap:\n result *= (1 << frequencyMap[key])\n continue\n\n kDiffFrequencies = [frequencyMap[key]]\n while key + k in frequencyMap:\n key += k\n kDiffFrequencies.append(frequencyMap[key])\n\n subsetWithout, subsetWith = 0, 0\n for frequency in kDiffFrequencies:\n subsetWithout, subsetWith = subsetWith + subsetWithout, ((1 << frequency) - 1) * ((subsetWithout - subsetWith) + 1)\n\n result *= (subsetWith + subsetWithout + 1)\n\n return result - 1\n```\nMY LINKE : https://leetcode.com/problems/the-number-of-beautiful-subsets/solutions/3352572/day-362-java-c-python-100-explained-intution-tc-0-n/?orderBy=most_votes\n\n# Complexity\n\n##### \u2022\tTime complexity: \n##### \u2022\tThe function first creates a frequency map of the input array, which takes O(n) time, where n is the length of the input array. \n##### \u2022\tThe function then loops through each entry in the frequency map, which takes O(m) time, where m is the number of unique elements in the input array.\n##### \u2022\tFor each entry in the frequency map, the function checks if the value starts a chain of k difference values. \n##### \u2022\tThis takes O(1) time. If the value does start a chain, the function creates a list of the frequencies of the values in the chain, which takes O(l) time, where l is the length of the chain. \n##### \u2022\tThe function then loops through the frequencies in the list and calculates the number of beautiful subsets that can be formed using the values in the chain. This takes O(l) time. \n##### \u2022\tFinally, the function multiplies the total number of beautiful subsets by the result accumulated so far, which takes O(1) time. Therefore, the total time complexity of the function is O(n + m * (l + l)), which simplifies to O(n + ml). \n##### \u2022\tSpace complexity: The function creates a frequency map of the input array, which takes O(m) space, where m is the number of unique elements in the input array. The function creates a list of the frequencies of the values in the chain, which takes O(l) space, where l is the length of the chain. The function uses a constant amount of extra space for variables like result , key , and value . Therefore, the total space complexity of the function is O(m + l), where m is the number of unique elements in the input array and l is the length of the chain.\n\n# 2ND WAY \n\n# Intuition & Approach\n\n##### \u2022\tThe problem asks us to find the number of beautiful subsets of the given array A , where a subset is beautiful if the absolute difference between any two elements in the subset is exactly k . \n##### \u2022\tThe intuition behind the algorithm is to use dynamic programming to count the number of beautiful subsets that end with each element of the array. \n##### \u2022\tWe can then sum up these counts to get the total number of beautiful subsets. To implement this approach, we first count the frequency of each element in the array using a HashMap . \n##### \u2022\tWe then define a recursive function dp(a) that takes an element a and returns a pair of values (dp0, dp1) , where dp0 is the number of beautiful subsets that end with a and do not include any element that is k units away from a , and dp1 is the number of beautiful subsets that end with a and include at least one element that is k units away from a . To compute dp(a) , \n##### \u2022\twe check if there is an element a-k in the count map. If there is, we recursively call dp(a-k) to get the values (dp0\', dp1\') for the element a-k . \n##### \u2022\tWe can then compute dp0 and dp1 as follows: dp0 = dp0\' + dp1\' : We can append a to any beautiful subset that ends with a-k and does not include any element that is k units away from a . dp1 = dp0\' * (2^count[a] - 1) : \n##### \u2022\tWe can append a to any beautiful subset that ends with a-k and includes at least one element that is k units away from a . \n##### \u2022\tThere are dp0\' such subsets, and for each subset, we can choose any subset of the count[a] elements that are k units away from a , except for the empty subset. If there is no element a-k in the count map, we set dp0 = 1 and dp1 = 2^count[a] - 1 , since the only beautiful subset that ends with a is the singleton set {a} . To avoid redundant calculations, we use memoization to store the values of dp(a) in a HashMap called memo . \n##### \u2022\tFinally, we sum up the values of dp0 and dp1 for all elements a in the count map that do not have an element a+k in the count map, and multiply them together to get the total number of beautiful subsets. \n##### \u2022\tWe subtract 1 from the result to exclude the empty subset. The time complexity of this algorithm is O(n log n), where n is the length of the input array, since we need to sort the array and perform O(log n) recursive calls for each element. \n##### \u2022\tThe space complexity is also O(n), since we need to store the count map and the memo map.\n\n\n```PYTHON []\nfrom collections import Counter\nfrom functools import reduce\nfrom operator import mul\n\nclass Solution:\n def beautifulSubsets(self, A: List[int], k: int) -> int:\n count = Counter(A)\n\n @lru_cache(None)\n def dp(a):\n if a - k in count:\n dp0, dp1 = dp(a - k)\n else:\n dp0, dp1 = 1, 0\n return dp0 + dp1, dp0 * (2 ** count[a] - 1)\n\n return reduce(mul, (sum(dp(a)) for a in count if a + k not in count)) - 1\n```\n```JAVA []\n\n class Solution {\n public int beautifulSubsets(int[] A, int k) {\n Map<Integer, Integer> count = new HashMap<Integer, Integer>();\n for (int a : A) {\n if (count.containsKey(a)) {\n count.put(a, count.get(a) + 1);\n } else {\n count.put(a, 1);\n }\n }\n\n final Map<Integer, Pair<Integer, Integer>> memo = new HashMap<Integer, Pair<Integer, Integer>>();\n Function<Integer, Pair<Integer, Integer>> dp = new Function<Integer, Pair<Integer, Integer>>() {\n public Pair<Integer, Integer> apply(Integer a) {\n if (count.containsKey(a - k)) {\n Pair<Integer, Integer> p = memo.containsKey(a - k) ? memo.get(a - k) : apply(a - k);\n int dp0 = p.getKey() + p.getValue();\n int dp1 = p.getKey() * ((1 << count.get(a)) - 1);\n Pair<Integer, Integer> result = new Pair<Integer, Integer>(dp0, dp1);\n memo.put(a, result);\n return result;\n } else {\n Pair<Integer, Integer> result = new Pair<Integer, Integer>(1, (1 << count.get(a)) - 1);\n memo.put(a, result);\n return result;\n }\n }\n };\n\n int result = 1;\n for (Map.Entry<Integer, Integer> entry : count.entrySet()) {\n int a = entry.getKey();\n int freq = entry.getValue();\n if (!count.containsKey(a + k)) {\n Pair<Integer, Integer> p = memo.containsKey(a) ? memo.get(a) : dp.apply(a);\n result *= p.getKey() + p.getValue();\n }\n }\n\n return result - 1;\n }\n}\n```\n```C++ []\nclass Solution {\npublic:\n int beautifulSubsets(vector<int>& A, int k) {\n unordered_map<int, int> count;\n for (int a : A) {\n count[a]++;\n }\n\n function<pair<int, int>(int)> dp = [&](int a) {\n if (count.count(a - k)) {\n auto [dp0, dp1] = dp(a - k);\n return make_pair(dp0 + dp1, dp0 * ((1 << count[a]) - 1));\n } else {\n return make_pair(1, (1 << count[a]) - 1);\n }\n };\n\n int result = 1;\n for (auto [a, freq] : count) {\n if (!count.count(a + k)) {\n auto [dp0, dp1] = dp(a);\n result *= dp0 + dp1;\n }\n }\n\n return result - 1;\n }\n};\n```\n# DRY RUN \nhere\'s a dry run of the function for the input nums = [2,4,6], k = 2:\n##### \u2022\tCreate a frequency map of the input array:\n##### \u2022\tcount = {\n##### \u2022\t 2: 1,\n##### \u2022\t 4: 1,\n##### \u2022\t 6: 1,\n##### \u2022\t}\n##### \u2022\tDefine the recursive function dp:\n##### \u2022\tdp(a) = (dp0, dp1)\n##### \u2022 where dp0 is the number of beautiful subsets that end with a and do not include any element that is k units away from a, and dp1 is the number of beautiful subsets that end with a and include at least one element that is k units away from a.\n##### \u2022 If count contains a - k, then we recursively call dp(a - k) to get the values (dp0\', dp1\') for a - k, and compute dp0 and dp1 as follows:\n##### \u2022 dp0 = dp0\' + dp1\' // append a to any beautiful subset that ends with a-k and does not include any element that is k units away from a\n##### \u2022 dp1 = dp0\' * ((1 << count[a]) - 1) // append a to any beautiful subset that ends with a-k and includes at least one element that is k units away from a\n##### \u2022 If count does not contain a - k, then we set dp0 = 1 and dp1 = (1 << count[a]) - 1, since the only beautiful subset that ends with a is the singleton set {a}.\n##### \u2022\tInitialize the result to 1, and loop through each element a in count that does not have an element a + k in count. For each such element, call dp(a) to get the values (dp0, dp1), and multiply the result by dp0 + dp1.\n##### \u2022\tresult = 1\n##### \u2022\tfor a, freq in count:\n##### \u2022\t if not count.contains(a + k):\n##### \u2022\t dp0, dp1 = dp(a)\n##### \u2022\t result *= dp0 + dp1\n##### \u2022\tSubtract 1 from the result to exclude the empty subset.\n##### \u2022\tresult -= 1\n##### \u2022\tReturn the result, which is 4 in this case, since there are 4 beautiful subsets of [2,4,6] with absolute difference k = 2: [2,4], [4,6], [2,4,6], and {} (empty subset).\n\n\n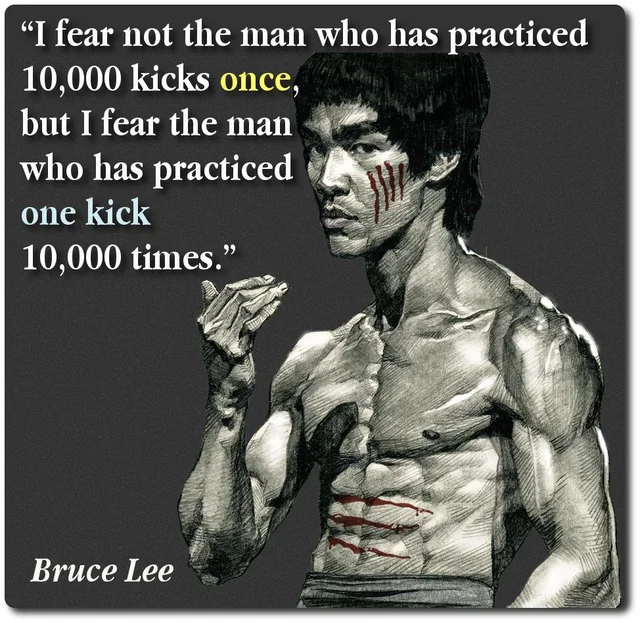\n\n\n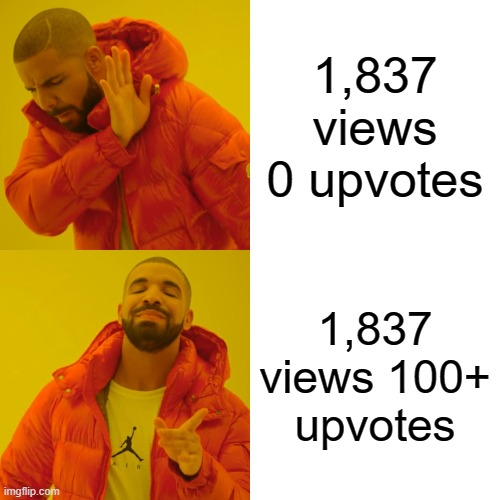\n | 3 | You are given an array `nums` of positive integers and a **positive** integer `k`.
A subset of `nums` is **beautiful** if it does not contain two integers with an absolute difference equal to `k`.
Return _the number of **non-empty beautiful** subsets of the array_ `nums`.
A **subset** of `nums` is an array that can be obtained by deleting some (possibly none) elements from `nums`. Two subsets are different if and only if the chosen indices to delete are different.
**Example 1:**
**Input:** nums = \[2,4,6\], k = 2
**Output:** 4
**Explanation:** The beautiful subsets of the array nums are: \[2\], \[4\], \[6\], \[2, 6\].
It can be proved that there are only 4 beautiful subsets in the array \[2,4,6\].
**Example 2:**
**Input:** nums = \[1\], k = 1
**Output:** 1
**Explanation:** The beautiful subset of the array nums is \[1\].
It can be proved that there is only 1 beautiful subset in the array \[1\].
**Constraints:**
* `1 <= nums.length <= 20`
* `1 <= nums[i], k <= 1000` | null |
python backtracking | the-number-of-beautiful-subsets | 0 | 1 | # Code\n```\nclass Solution:\n def beautifulSubsets(self, nums: List[int], k: int) -> int:\n n = len(nums)\n output = 0\n \n def dfs(i, ctr):\n nonlocal output\n if i == n:\n if ctr:\n output += 1\n return\n \n if nums[i] - k not in ctr and nums[i] + k not in ctr:\n ctr[nums[i]] += 1\n dfs(i + 1, ctr)\n ctr[nums[i]] -= 1\n if not ctr[nums[i]]:\n del ctr[nums[i]]\n dfs(i + 1, ctr)\n \n dfs(0, Counter())\n return output\n \n``` | 9 | You are given an array `nums` of positive integers and a **positive** integer `k`.
A subset of `nums` is **beautiful** if it does not contain two integers with an absolute difference equal to `k`.
Return _the number of **non-empty beautiful** subsets of the array_ `nums`.
A **subset** of `nums` is an array that can be obtained by deleting some (possibly none) elements from `nums`. Two subsets are different if and only if the chosen indices to delete are different.
**Example 1:**
**Input:** nums = \[2,4,6\], k = 2
**Output:** 4
**Explanation:** The beautiful subsets of the array nums are: \[2\], \[4\], \[6\], \[2, 6\].
It can be proved that there are only 4 beautiful subsets in the array \[2,4,6\].
**Example 2:**
**Input:** nums = \[1\], k = 1
**Output:** 1
**Explanation:** The beautiful subset of the array nums is \[1\].
It can be proved that there is only 1 beautiful subset in the array \[1\].
**Constraints:**
* `1 <= nums.length <= 20`
* `1 <= nums[i], k <= 1000` | null |
[Python 3] Iterative BackTracking | the-number-of-beautiful-subsets | 0 | 1 | ```\nclass Solution:\n def beautifulSubsets(self, nums: List[int], k: int) -> int:\n q = deque( [([], -1)] )\n res = 0\n \n while q:\n cur, idx = q.popleft()\n res += 1\n \n for i in range(idx + 1, len(nums)):\n if nums[i] - k in cur or nums[i] + k in cur:\n continue\n q.append( (cur + [nums[i]], i) )\n \n return res - 1\n``` | 9 | You are given an array `nums` of positive integers and a **positive** integer `k`.
A subset of `nums` is **beautiful** if it does not contain two integers with an absolute difference equal to `k`.
Return _the number of **non-empty beautiful** subsets of the array_ `nums`.
A **subset** of `nums` is an array that can be obtained by deleting some (possibly none) elements from `nums`. Two subsets are different if and only if the chosen indices to delete are different.
**Example 1:**
**Input:** nums = \[2,4,6\], k = 2
**Output:** 4
**Explanation:** The beautiful subsets of the array nums are: \[2\], \[4\], \[6\], \[2, 6\].
It can be proved that there are only 4 beautiful subsets in the array \[2,4,6\].
**Example 2:**
**Input:** nums = \[1\], k = 1
**Output:** 1
**Explanation:** The beautiful subset of the array nums is \[1\].
It can be proved that there is only 1 beautiful subset in the array \[1\].
**Constraints:**
* `1 <= nums.length <= 20`
* `1 <= nums[i], k <= 1000` | null |
[python] O(n) | the-number-of-beautiful-subsets | 0 | 1 | # Intuition\nwe have to notice that the only elements that affect one another are those that exist in a streak of numbers that differ by k.\n\n# Approach\ni group these numbers together into these streaks, and find the number of subsets of each streak separately. we can then multiply the answers together, since all streak subsets are compatible with one another.\n\n# Code\n```\nclass Solution:\n def beautifulSubsets(self, nums: List[int], k: int) -> int: \n @cache\n # ways of choosing from streaks[si][i:]\n def ways(i, si):\n if i >= len(streaks[si]):\n return 1\n # there are 2**rep-1 ways of choosing at least \n # one of streak[i].\n return ways(i+1, si) + (2**reps[streaks[si][i]]-1) * ways(i+2, si)\n \n reps = defaultdict(int)\n mark = {} # -1 for start of streak, 1 otherwise\n\n for num in nums:\n mark[num] = -1\n\n for num in nums:\n if reps[num]:\n reps[num] += 1\n else:\n if num - k in mark:\n mark[num] = 1\n reps[num] = 1\n\n streaks = []\n for num in nums:\n if num not in mark or mark[num] == 1:\n continue\n curr = num\n streaks.append([])\n while curr in mark:\n streaks[-1].append(curr)\n del mark[curr]\n curr = curr + k\n \n prod = 1\n for i in range(len(streaks)):\n prod *= ways(0, i)\n return prod - 1 # don\'t consider empty subset\n``` | 4 | You are given an array `nums` of positive integers and a **positive** integer `k`.
A subset of `nums` is **beautiful** if it does not contain two integers with an absolute difference equal to `k`.
Return _the number of **non-empty beautiful** subsets of the array_ `nums`.
A **subset** of `nums` is an array that can be obtained by deleting some (possibly none) elements from `nums`. Two subsets are different if and only if the chosen indices to delete are different.
**Example 1:**
**Input:** nums = \[2,4,6\], k = 2
**Output:** 4
**Explanation:** The beautiful subsets of the array nums are: \[2\], \[4\], \[6\], \[2, 6\].
It can be proved that there are only 4 beautiful subsets in the array \[2,4,6\].
**Example 2:**
**Input:** nums = \[1\], k = 1
**Output:** 1
**Explanation:** The beautiful subset of the array nums is \[1\].
It can be proved that there is only 1 beautiful subset in the array \[1\].
**Constraints:**
* `1 <= nums.length <= 20`
* `1 <= nums[i], k <= 1000` | null |
Python || dynamic programming || 90ms | the-number-of-beautiful-subsets | 0 | 1 | 1. First we group `nums` by `n % k`. These buckets are disjoint, we could compute the number of beautiful subsets for each bucket(including empty set), and the product of these numbers minus 1 would be the answer.\n2. For each bucket, use dynamic programming. Here we consider an example, [2,2,2,4,4,6], `k = 2`.\n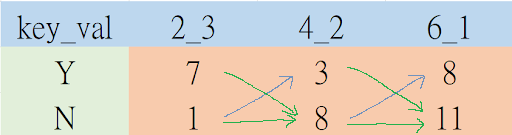\nFirst we consider only 2. There are `2**3-1 = 7` subsets containing 2, and `1` subset not containing 2(empty), i.e. `dp_y = 7, dp_n = 1`.\nNext we consider 4. If we choose to have 4 in our subsets, it cannot come from those subsets containing 2, i.e. `dp_y = 1*(2**2-1) = 3`. If we choose not to have 4, it can come from either subsets containing 2 or not, i.e. `dp_n = 7+1 = 8`.\nFinally we consider 6. Similar to above, we have `dp_y = 8*1 = 8, dp_n = 3+8 = 11`.\nSo the number of all possibilities(including empty set) is the sum of the rightmost column, `8+11 = 19`.\n```\nclass Solution:\n def beautifulSubsets(self, nums: List[int], k: int) -> int:\n buckets = [[] for _ in range(k)]\n for n in nums:\n buckets[n % k].append(n)\n \n def dp(bu):\n cnt = Counter(bu)\n arr = sorted((key, val) for key, val in cnt.items())\n dp_y, dp_n = 2**arr[0][1]-1, 1\n for i, (key, val) in enumerate(arr[1:], start=1):\n tmp = 2**val-1\n if key - arr[i-1][0] == k:\n dp_y, dp_n = dp_n*tmp, dp_y+dp_n\n else:\n dp_y, dp_n = (dp_y+dp_n)*tmp, dp_y+dp_n\n return dp_y + dp_n\n \n ans = 1\n for bu in buckets:\n if bu:\n ans *= dp(bu)\n return ans - 1 | 2 | You are given an array `nums` of positive integers and a **positive** integer `k`.
A subset of `nums` is **beautiful** if it does not contain two integers with an absolute difference equal to `k`.
Return _the number of **non-empty beautiful** subsets of the array_ `nums`.
A **subset** of `nums` is an array that can be obtained by deleting some (possibly none) elements from `nums`. Two subsets are different if and only if the chosen indices to delete are different.
**Example 1:**
**Input:** nums = \[2,4,6\], k = 2
**Output:** 4
**Explanation:** The beautiful subsets of the array nums are: \[2\], \[4\], \[6\], \[2, 6\].
It can be proved that there are only 4 beautiful subsets in the array \[2,4,6\].
**Example 2:**
**Input:** nums = \[1\], k = 1
**Output:** 1
**Explanation:** The beautiful subset of the array nums is \[1\].
It can be proved that there is only 1 beautiful subset in the array \[1\].
**Constraints:**
* `1 <= nums.length <= 20`
* `1 <= nums[i], k <= 1000` | null |
Follow Procedure || Easy || Python3 | the-number-of-beautiful-subsets | 0 | 1 | \n```\nclass Solution:\n def beautifulSubsets(self, nums: List[int], k: int) -> int: \n \n def count(n,prev):\n if n == 0:\n return 1 \n ans = 0\n if prev[nums[n-1]-k] == 0 and prev[nums[n-1]+k] == 0:\n prev[nums[n-1]] += 1\n ans += count(n-1,prev) \n prev[nums[n-1]] -= 1 \n ans += count(n-1,prev) \n \n return ans \n \n return count(len(nums),Counter())-1\n \n \n \n \n``` | 2 | You are given an array `nums` of positive integers and a **positive** integer `k`.
A subset of `nums` is **beautiful** if it does not contain two integers with an absolute difference equal to `k`.
Return _the number of **non-empty beautiful** subsets of the array_ `nums`.
A **subset** of `nums` is an array that can be obtained by deleting some (possibly none) elements from `nums`. Two subsets are different if and only if the chosen indices to delete are different.
**Example 1:**
**Input:** nums = \[2,4,6\], k = 2
**Output:** 4
**Explanation:** The beautiful subsets of the array nums are: \[2\], \[4\], \[6\], \[2, 6\].
It can be proved that there are only 4 beautiful subsets in the array \[2,4,6\].
**Example 2:**
**Input:** nums = \[1\], k = 1
**Output:** 1
**Explanation:** The beautiful subset of the array nums is \[1\].
It can be proved that there is only 1 beautiful subset in the array \[1\].
**Constraints:**
* `1 <= nums.length <= 20`
* `1 <= nums[i], k <= 1000` | null |
Python Backtracking || Explanation | the-number-of-beautiful-subsets | 0 | 1 | # Intuition\nSubset problem, think of using backtracking!\n\n***Why using dictionary instead of set or list?***\nUsing List : When checking numbers in seen, it needs O(n) to check which is inefficient. \n***Then you will think about use set***. However, when you are going yo pop out the number for current dfs level and there are serveral same numbers in the subsets, there would be a problem.\n\n\n# Code\n```\nclass Solution:\n def beautifulSubsets(self, nums: List[int], k: int) -> int:\n self.output = 0\n def dfs(start,seen) :\n if start >= len(nums) :\n return\n for i in range(start, len(nums)) :\n if len(seen) != 0 and seen[nums[i]-k] != 0 or seen[nums[i]+k] != 0 :\n continue\n seen[nums[i]] += 1\n self.output += 1\n dfs(i+1, seen)\n seen[nums[i]] -= 1\n return\n dfs(0, collections.defaultdict(int))\n return self.output\n \n \n \n``` | 3 | You are given an array `nums` of positive integers and a **positive** integer `k`.
A subset of `nums` is **beautiful** if it does not contain two integers with an absolute difference equal to `k`.
Return _the number of **non-empty beautiful** subsets of the array_ `nums`.
A **subset** of `nums` is an array that can be obtained by deleting some (possibly none) elements from `nums`. Two subsets are different if and only if the chosen indices to delete are different.
**Example 1:**
**Input:** nums = \[2,4,6\], k = 2
**Output:** 4
**Explanation:** The beautiful subsets of the array nums are: \[2\], \[4\], \[6\], \[2, 6\].
It can be proved that there are only 4 beautiful subsets in the array \[2,4,6\].
**Example 2:**
**Input:** nums = \[1\], k = 1
**Output:** 1
**Explanation:** The beautiful subset of the array nums is \[1\].
It can be proved that there is only 1 beautiful subset in the array \[1\].
**Constraints:**
* `1 <= nums.length <= 20`
* `1 <= nums[i], k <= 1000` | null |
[Python3] Modulus O(n), Clean & Concise | smallest-missing-non-negative-integer-after-operations | 0 | 1 | # Complexity\n- Time complexity: $$O(n)$$\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: $$O(value)$$\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def findSmallestInteger(self, nums: List[int], value: int) -> int:\n counter = Counter([x % value for x in nums])\n ans = 0\n while counter[ans % value] > 0:\n counter[ans % value] -= 1\n ans += 1\n return ans\n``` | 2 | You are given a **0-indexed** integer array `nums` and an integer `value`.
In one operation, you can add or subtract `value` from any element of `nums`.
* For example, if `nums = [1,2,3]` and `value = 2`, you can choose to subtract `value` from `nums[0]` to make `nums = [-1,2,3]`.
The MEX (minimum excluded) of an array is the smallest missing **non-negative** integer in it.
* For example, the MEX of `[-1,2,3]` is `0` while the MEX of `[1,0,3]` is `2`.
Return _the maximum MEX of_ `nums` _after applying the mentioned operation **any number of times**_.
**Example 1:**
**Input:** nums = \[1,-10,7,13,6,8\], value = 5
**Output:** 4
**Explanation:** One can achieve this result by applying the following operations:
- Add value to nums\[1\] twice to make nums = \[1,**0**,7,13,6,8\]
- Subtract value from nums\[2\] once to make nums = \[1,0,**2**,13,6,8\]
- Subtract value from nums\[3\] twice to make nums = \[1,0,2,**3**,6,8\]
The MEX of nums is 4. It can be shown that 4 is the maximum MEX we can achieve.
**Example 2:**
**Input:** nums = \[1,-10,7,13,6,8\], value = 7
**Output:** 2
**Explanation:** One can achieve this result by applying the following operation:
- subtract value from nums\[2\] once to make nums = \[1,-10,**0**,13,6,8\]
The MEX of nums is 2. It can be shown that 2 is the maximum MEX we can achieve.
**Constraints:**
* `1 <= nums.length, value <= 105`
* `-109 <= nums[i] <= 109` | null |
[C++ / Python] | | Easy && Concise ✅ | smallest-missing-non-negative-integer-after-operations | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nOne can observe that the elements of the array can be increased/decreased by k any number of times. We use the modular arithmetic approach to set the value to the elements to maximise the **MEX.**\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\nCompute the modulo of each element in array A with k and store the counts in a hashmap. Begin from 0 and iterate up to k. When reaching k, restart from 0. Decrease the count in the hashmap each time a mod value is found. The first mod value with a count of 0 becomes the MEX (smallest positive integer absent in the array). Modify the array internally by replacing A with the **sequence [0, 1, 2, ..., k-1] to find the maximum MEX**\n\n\n# Complexity\n- Time complexity: O(n) + O(n)\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: O(k)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n\n```C++ []\nclass Solution {\npublic:\n int findSmallestInteger(vector<int>& v, int k) {\n unordered_map<int,int> mp;\n for(auto x : v){\n int mod = x % k;\n if(mod < 0) mod = k + mod;\n mp[mod]++;\n }\n // now calculate the mex \n int mex = 0;\n while(true){\n if(mp[mex] == 0) return mex;\n int ct = mp[mex];\n\n int i = 0;\n while(ct--){\n mp[mex + (i * k)]++;\n i++;\n } \n mex++;\n }\n return -1;\n }\n};\n```\n\n\n\n```Python []\nfrom collections import defaultdict\n\nclass Solution:\n def findSmallestInteger(self, arr, k):\n hash_map = defaultdict(int)\n\n for v in arr:\n mod = (k * 10**8 + v) % k\n hash_map[mod] += 1\n\n mex = 0\n while True:\n if hash_map[mex] == 0:\n return mex\n count = hash_map[mex]\n\n i = 0\n while count > 0:\n hash_map[mex + i * k] += 1\n i += 1\n count -= 1\n\n mex += 1\n\n return -1\n``` | 2 | You are given a **0-indexed** integer array `nums` and an integer `value`.
In one operation, you can add or subtract `value` from any element of `nums`.
* For example, if `nums = [1,2,3]` and `value = 2`, you can choose to subtract `value` from `nums[0]` to make `nums = [-1,2,3]`.
The MEX (minimum excluded) of an array is the smallest missing **non-negative** integer in it.
* For example, the MEX of `[-1,2,3]` is `0` while the MEX of `[1,0,3]` is `2`.
Return _the maximum MEX of_ `nums` _after applying the mentioned operation **any number of times**_.
**Example 1:**
**Input:** nums = \[1,-10,7,13,6,8\], value = 5
**Output:** 4
**Explanation:** One can achieve this result by applying the following operations:
- Add value to nums\[1\] twice to make nums = \[1,**0**,7,13,6,8\]
- Subtract value from nums\[2\] once to make nums = \[1,0,**2**,13,6,8\]
- Subtract value from nums\[3\] twice to make nums = \[1,0,2,**3**,6,8\]
The MEX of nums is 4. It can be shown that 4 is the maximum MEX we can achieve.
**Example 2:**
**Input:** nums = \[1,-10,7,13,6,8\], value = 7
**Output:** 2
**Explanation:** One can achieve this result by applying the following operation:
- subtract value from nums\[2\] once to make nums = \[1,-10,**0**,13,6,8\]
The MEX of nums is 2. It can be shown that 2 is the maximum MEX we can achieve.
**Constraints:**
* `1 <= nums.length, value <= 105`
* `-109 <= nums[i] <= 109` | null |
Find Remainder || python3 || simple and concise | smallest-missing-non-negative-integer-after-operations | 0 | 1 | \n```\nclass Solution:\n def findSmallestInteger(self, nums: List[int], value: int) -> int:\n count = Counter() \n for i in nums:\n count[i%value] += 1 \n \n for i in range(len(nums)):\n rem = i % value \n if count[rem] <= 0:\n return i \n count[rem] -= 1 \n \n return i+1\n \n \n``` | 2 | You are given a **0-indexed** integer array `nums` and an integer `value`.
In one operation, you can add or subtract `value` from any element of `nums`.
* For example, if `nums = [1,2,3]` and `value = 2`, you can choose to subtract `value` from `nums[0]` to make `nums = [-1,2,3]`.
The MEX (minimum excluded) of an array is the smallest missing **non-negative** integer in it.
* For example, the MEX of `[-1,2,3]` is `0` while the MEX of `[1,0,3]` is `2`.
Return _the maximum MEX of_ `nums` _after applying the mentioned operation **any number of times**_.
**Example 1:**
**Input:** nums = \[1,-10,7,13,6,8\], value = 5
**Output:** 4
**Explanation:** One can achieve this result by applying the following operations:
- Add value to nums\[1\] twice to make nums = \[1,**0**,7,13,6,8\]
- Subtract value from nums\[2\] once to make nums = \[1,0,**2**,13,6,8\]
- Subtract value from nums\[3\] twice to make nums = \[1,0,2,**3**,6,8\]
The MEX of nums is 4. It can be shown that 4 is the maximum MEX we can achieve.
**Example 2:**
**Input:** nums = \[1,-10,7,13,6,8\], value = 7
**Output:** 2
**Explanation:** One can achieve this result by applying the following operation:
- subtract value from nums\[2\] once to make nums = \[1,-10,**0**,13,6,8\]
The MEX of nums is 2. It can be shown that 2 is the maximum MEX we can achieve.
**Constraints:**
* `1 <= nums.length, value <= 105`
* `-109 <= nums[i] <= 109` | null |
python3 Solution | smallest-missing-non-negative-integer-after-operations | 0 | 1 | \n```\nclass Solution:\n def findSmallestInteger(self, nums: List[int], value: int) -> int:\n count=Counter(num%value for num in nums)\n stop=0\n for i in range(value):\n if count[i]<count[stop]:\n stop=i\n\n return value*count[stop]+stop \n``` | 1 | You are given a **0-indexed** integer array `nums` and an integer `value`.
In one operation, you can add or subtract `value` from any element of `nums`.
* For example, if `nums = [1,2,3]` and `value = 2`, you can choose to subtract `value` from `nums[0]` to make `nums = [-1,2,3]`.
The MEX (minimum excluded) of an array is the smallest missing **non-negative** integer in it.
* For example, the MEX of `[-1,2,3]` is `0` while the MEX of `[1,0,3]` is `2`.
Return _the maximum MEX of_ `nums` _after applying the mentioned operation **any number of times**_.
**Example 1:**
**Input:** nums = \[1,-10,7,13,6,8\], value = 5
**Output:** 4
**Explanation:** One can achieve this result by applying the following operations:
- Add value to nums\[1\] twice to make nums = \[1,**0**,7,13,6,8\]
- Subtract value from nums\[2\] once to make nums = \[1,0,**2**,13,6,8\]
- Subtract value from nums\[3\] twice to make nums = \[1,0,2,**3**,6,8\]
The MEX of nums is 4. It can be shown that 4 is the maximum MEX we can achieve.
**Example 2:**
**Input:** nums = \[1,-10,7,13,6,8\], value = 7
**Output:** 2
**Explanation:** One can achieve this result by applying the following operation:
- subtract value from nums\[2\] once to make nums = \[1,-10,**0**,13,6,8\]
The MEX of nums is 2. It can be shown that 2 is the maximum MEX we can achieve.
**Constraints:**
* `1 <= nums.length, value <= 105`
* `-109 <= nums[i] <= 109` | null |
Python solution | smallest-missing-non-negative-integer-after-operations | 0 | 1 | \n# Code\n```\nclass Solution:\n def findSmallestInteger(self, nums: List[int], value: int) -> int:\n # here in order to find the first non negative missing number \n # first calculate the remainder (nums[i]%value) of all element and count the no of occurences of same remainders\n # the maximum value of the remainder will be (value - 1) \n\n # consider \n # [0,3,-7,1]\n # 0%3=0 , 3%3=0 , -7%3=2 , 1%3=1\n # so therefore , d = { 0 : 2 , 1 : 1 , 2 :1 }\n # if any remainder less than the value is not present in the dictionary then that is the missing number\n # else linearly traverse the dictionary and subtract the frequency of the rem by 1 \n # if you reach 0 then that is the missing value\n \n d = {} # dictionary to store the remainders and its count \n l = [-1]*(value) # list to know whether the rem is found or not if it is not found then it will be -1 and that corresponding position is the missing value\n\n for i in range(len(nums)) :\n if nums[i]%value in d :\n d[nums[i]%value] += 1\n else:\n d[nums[i]%value] = 1\n l[nums[i]%value] = 1\n if -1 in l:\n return l.index(-1) \n\n # this is for if every rem is found ie all nos from (0 to value-1) is found\n \n cnt = 0\n #storing the frequency of the remainders in a list\n lis = [d[x] for x in range(0,value)] \n i = 0\n\n #decrement the frequency each time\n #increment the count \n # if the frequency becomes 0 then that is the missing value\n while(lis[i]!=0) :\n if lis[i]!=0 :\n cnt += 1\n lis[i]-=1\n i += 1\n i%=value #done to avoid ovwerflow error\n return cnt\n``` | 2 | You are given a **0-indexed** integer array `nums` and an integer `value`.
In one operation, you can add or subtract `value` from any element of `nums`.
* For example, if `nums = [1,2,3]` and `value = 2`, you can choose to subtract `value` from `nums[0]` to make `nums = [-1,2,3]`.
The MEX (minimum excluded) of an array is the smallest missing **non-negative** integer in it.
* For example, the MEX of `[-1,2,3]` is `0` while the MEX of `[1,0,3]` is `2`.
Return _the maximum MEX of_ `nums` _after applying the mentioned operation **any number of times**_.
**Example 1:**
**Input:** nums = \[1,-10,7,13,6,8\], value = 5
**Output:** 4
**Explanation:** One can achieve this result by applying the following operations:
- Add value to nums\[1\] twice to make nums = \[1,**0**,7,13,6,8\]
- Subtract value from nums\[2\] once to make nums = \[1,0,**2**,13,6,8\]
- Subtract value from nums\[3\] twice to make nums = \[1,0,2,**3**,6,8\]
The MEX of nums is 4. It can be shown that 4 is the maximum MEX we can achieve.
**Example 2:**
**Input:** nums = \[1,-10,7,13,6,8\], value = 7
**Output:** 2
**Explanation:** One can achieve this result by applying the following operation:
- subtract value from nums\[2\] once to make nums = \[1,-10,**0**,13,6,8\]
The MEX of nums is 2. It can be shown that 2 is the maximum MEX we can achieve.
**Constraints:**
* `1 <= nums.length, value <= 105`
* `-109 <= nums[i] <= 109` | null |
[Python 3] MOD | Greedy | smallest-missing-non-negative-integer-after-operations | 0 | 1 | ```\nclass Solution:\n def findSmallestInteger(self, nums: List[int], val: int) -> int:\n nums = sorted( [i % val for i in nums] )\n seen = set(nums)\n t = 1\n \n for i in range(len(nums) - 1):\n if nums[i] == nums[i + 1]:\n seen.add(nums[i] + (t * val))\n t += 1\n else:\n t = 1\n \n for i in range(len(seen) + 1):\n if i not in seen:\n return i\n``` | 4 | You are given a **0-indexed** integer array `nums` and an integer `value`.
In one operation, you can add or subtract `value` from any element of `nums`.
* For example, if `nums = [1,2,3]` and `value = 2`, you can choose to subtract `value` from `nums[0]` to make `nums = [-1,2,3]`.
The MEX (minimum excluded) of an array is the smallest missing **non-negative** integer in it.
* For example, the MEX of `[-1,2,3]` is `0` while the MEX of `[1,0,3]` is `2`.
Return _the maximum MEX of_ `nums` _after applying the mentioned operation **any number of times**_.
**Example 1:**
**Input:** nums = \[1,-10,7,13,6,8\], value = 5
**Output:** 4
**Explanation:** One can achieve this result by applying the following operations:
- Add value to nums\[1\] twice to make nums = \[1,**0**,7,13,6,8\]
- Subtract value from nums\[2\] once to make nums = \[1,0,**2**,13,6,8\]
- Subtract value from nums\[3\] twice to make nums = \[1,0,2,**3**,6,8\]
The MEX of nums is 4. It can be shown that 4 is the maximum MEX we can achieve.
**Example 2:**
**Input:** nums = \[1,-10,7,13,6,8\], value = 7
**Output:** 2
**Explanation:** One can achieve this result by applying the following operation:
- subtract value from nums\[2\] once to make nums = \[1,-10,**0**,13,6,8\]
The MEX of nums is 2. It can be shown that 2 is the maximum MEX we can achieve.
**Constraints:**
* `1 <= nums.length, value <= 105`
* `-109 <= nums[i] <= 109` | null |
Count Moduli | smallest-missing-non-negative-integer-after-operations | 0 | 1 | For an element `n`, we can achieve the minimum non-negative value of `n % value`.\n\nWe can also transform element `n` to `n % value + k * value`. So, we first count moduli we can get from all numbers.\n\nThen, we iterate `i` from zero upwards, and check if we have a modulo (`i % value`) that we can tranform to `i`.\n\nIf so, we decrease the counter for that modulo. If the counter is zero, we cannot produce `i` and it\'s our missing value. \n\n**Python 3**\n```python\nclass Solution:\n def findSmallestInteger(self, nums: List[int], value: int) -> int:\n m = Counter([n % value for n in nums])\n for i in range(len(nums)):\n if m[i % value] == 0:\n return i\n m[i % value] -= 1\n return len(nums)\n```\n \n**C++**\n```cpp\nint findSmallestInteger(vector<int>& nums, int v) {\n int m[100001] = {}, sz = nums.size();\n for (auto n : nums)\n // C++ fix for negative remainder: (b + (a % b)) % b\n ++m[(v + n % v) % v];\n for (int i = 0; i < sz; ++i)\n if (--m[i % v] < 0)\n return i;\n return sz;\n}\n```\nA different way to look at it (suggested by [Benlegend](https://leetcode.com/Benlegend/)). \n\nWe can find a modulo with the smalest count. That modulo and count to compute the missing value.\n\nThis is useful when value is much smaller than the number of elements.\n\n**C++**\n```cpp\nint findSmallestInteger(vector<int>& nums, int v) {\n int m[100001] = {}, sz = nums.size();\n for (auto n : nums)\n // C++ fix for negative remainder: (b + (a % b)) % b\n ++m[(v + n % v) % v];\n int i = min_element(begin(m), begin(m) + v) - begin(m);\n return i + m[i] * v;\n}\n``` | 51 | You are given a **0-indexed** integer array `nums` and an integer `value`.
In one operation, you can add or subtract `value` from any element of `nums`.
* For example, if `nums = [1,2,3]` and `value = 2`, you can choose to subtract `value` from `nums[0]` to make `nums = [-1,2,3]`.
The MEX (minimum excluded) of an array is the smallest missing **non-negative** integer in it.
* For example, the MEX of `[-1,2,3]` is `0` while the MEX of `[1,0,3]` is `2`.
Return _the maximum MEX of_ `nums` _after applying the mentioned operation **any number of times**_.
**Example 1:**
**Input:** nums = \[1,-10,7,13,6,8\], value = 5
**Output:** 4
**Explanation:** One can achieve this result by applying the following operations:
- Add value to nums\[1\] twice to make nums = \[1,**0**,7,13,6,8\]
- Subtract value from nums\[2\] once to make nums = \[1,0,**2**,13,6,8\]
- Subtract value from nums\[3\] twice to make nums = \[1,0,2,**3**,6,8\]
The MEX of nums is 4. It can be shown that 4 is the maximum MEX we can achieve.
**Example 2:**
**Input:** nums = \[1,-10,7,13,6,8\], value = 7
**Output:** 2
**Explanation:** One can achieve this result by applying the following operation:
- subtract value from nums\[2\] once to make nums = \[1,-10,**0**,13,6,8\]
The MEX of nums is 2. It can be shown that 2 is the maximum MEX we can achieve.
**Constraints:**
* `1 <= nums.length, value <= 105`
* `-109 <= nums[i] <= 109` | null |
python3 :-) | smallest-missing-non-negative-integer-after-operations | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def findSmallestInteger(self, nums: List[int], value: int) -> int:\n a=[x % value for x in nums]\n A=Counter(a)\n ans=0\n while A[ans % value] > 0:\n A[ans % value]-=1\n ans+=1\n return ans\n``` | 1 | You are given a **0-indexed** integer array `nums` and an integer `value`.
In one operation, you can add or subtract `value` from any element of `nums`.
* For example, if `nums = [1,2,3]` and `value = 2`, you can choose to subtract `value` from `nums[0]` to make `nums = [-1,2,3]`.
The MEX (minimum excluded) of an array is the smallest missing **non-negative** integer in it.
* For example, the MEX of `[-1,2,3]` is `0` while the MEX of `[1,0,3]` is `2`.
Return _the maximum MEX of_ `nums` _after applying the mentioned operation **any number of times**_.
**Example 1:**
**Input:** nums = \[1,-10,7,13,6,8\], value = 5
**Output:** 4
**Explanation:** One can achieve this result by applying the following operations:
- Add value to nums\[1\] twice to make nums = \[1,**0**,7,13,6,8\]
- Subtract value from nums\[2\] once to make nums = \[1,0,**2**,13,6,8\]
- Subtract value from nums\[3\] twice to make nums = \[1,0,2,**3**,6,8\]
The MEX of nums is 4. It can be shown that 4 is the maximum MEX we can achieve.
**Example 2:**
**Input:** nums = \[1,-10,7,13,6,8\], value = 7
**Output:** 2
**Explanation:** One can achieve this result by applying the following operation:
- subtract value from nums\[2\] once to make nums = \[1,-10,**0**,13,6,8\]
The MEX of nums is 2. It can be shown that 2 is the maximum MEX we can achieve.
**Constraints:**
* `1 <= nums.length, value <= 105`
* `-109 <= nums[i] <= 109` | null |
✔️ Python3 Solution | Clean & Concise | smallest-missing-non-negative-integer-after-operations | 0 | 1 | # Approach\nSince we can `add or subtract` as many times, first we made all the elements in range(0, value) by taking its `modulus` (simply by adding or subtracting) and store its count in `dp`. \n\nNow we just need to check that `i % v` exist or not in `dp` for making a number equal to i , if it exist then by adding `(i % v) + k * v = i`, it proves that if i % v exist, i can be obtain by adding operation.\n\n# Complexity\n- Time complexity: $$O(n)$$\n- Space complexity: $$O(n)$$\n\n# Code\n```Python\nclass Solution:\n def findSmallestInteger(self, A: List[int], v: int) -> int:\n n = len(A)\n m = min(n, v)\n dp = [0] * m\n for i in A:\n if i % v < m: \n dp[i % v] += 1\n for i in range(n):\n if i % v >= n or dp[i % v] == 0: return i \n dp[i % v] -= 1\n return n\n``` | 2 | You are given a **0-indexed** integer array `nums` and an integer `value`.
In one operation, you can add or subtract `value` from any element of `nums`.
* For example, if `nums = [1,2,3]` and `value = 2`, you can choose to subtract `value` from `nums[0]` to make `nums = [-1,2,3]`.
The MEX (minimum excluded) of an array is the smallest missing **non-negative** integer in it.
* For example, the MEX of `[-1,2,3]` is `0` while the MEX of `[1,0,3]` is `2`.
Return _the maximum MEX of_ `nums` _after applying the mentioned operation **any number of times**_.
**Example 1:**
**Input:** nums = \[1,-10,7,13,6,8\], value = 5
**Output:** 4
**Explanation:** One can achieve this result by applying the following operations:
- Add value to nums\[1\] twice to make nums = \[1,**0**,7,13,6,8\]
- Subtract value from nums\[2\] once to make nums = \[1,0,**2**,13,6,8\]
- Subtract value from nums\[3\] twice to make nums = \[1,0,2,**3**,6,8\]
The MEX of nums is 4. It can be shown that 4 is the maximum MEX we can achieve.
**Example 2:**
**Input:** nums = \[1,-10,7,13,6,8\], value = 7
**Output:** 2
**Explanation:** One can achieve this result by applying the following operation:
- subtract value from nums\[2\] once to make nums = \[1,-10,**0**,13,6,8\]
The MEX of nums is 2. It can be shown that 2 is the maximum MEX we can achieve.
**Constraints:**
* `1 <= nums.length, value <= 105`
* `-109 <= nums[i] <= 109` | null |
Counting % || Linear TC and SC | smallest-missing-non-negative-integer-after-operations | 1 | 1 | # Intuition\nJust count the modulo of all the elements with K.\nnums[i]%value\nFor -ve, add +k too(To make it +ve) since MEX is >= 0.\n\n\n# Complexity\n- Time complexity:\nO(n)\n\n- Space complexity:\nO(n)\n\n# Code\n``` C++ []\nclass Solution {\npublic:\n int findSmallestInteger(vector<int>&nums, int val) {\n int n=nums.size();\n int res=0;\n unordered_map<int,int>mp;\n set<int>st;\n for(int i=0;i<n;i++){\n nums[i]=(nums[i]%val+val)%val;\n if(mp[nums[i]])nums[i]=mp[nums[i]]+val-1;\n st.insert(nums[i]);\n mp[nums[i]%val]=nums[i]+1;\n }\n int mex=0;\n for(auto x : st){\n if(x==mex)mex++;\n else return mex;\n }\n return mex;\n }\n};\n\n```\n```java []\nclass Solution {\n public int findSmallestInteger(int[] nums, int val) {\n int n = nums.length;\n int res = 0;\n Map<Integer, Integer> mp = new HashMap<>();\n Set<Integer> st = new HashSet<>();\n for (int i = 0; i < n; i++) {\n nums[i] = (nums[i] % val + val) % val;\n if (mp.containsKey(nums[i])) {\n nums[i] = mp.get(nums[i]) + val - 1;\n }\n st.add(nums[i]);\n mp.put(nums[i] % val, nums[i] + 1);\n }\n int mex = 0;\n for (int x : st) {\n if (x == mex) {\n mex++;\n } else {\n return mex;\n }\n }\n return mex;\n }\n}\n```\n```python3 []\nclass Solution:\n def findSmallestInteger(self, nums: List[int], val: int) -> int:\n n = len(nums)\n res = 0\n mp = {}\n st = set()\n for i in range(n):\n nums[i] = (nums[i] % val + val) % val\n if nums[i] in mp:\n nums[i] = mp[nums[i]] + val - 1\n st.add(nums[i])\n mp[nums[i] % val] = nums[i] + 1\n mex = 0\n for x in sorted(st):\n if x == mex:\n mex += 1\n else:\n return mex\n return mex\n``` | 4 | You are given a **0-indexed** integer array `nums` and an integer `value`.
In one operation, you can add or subtract `value` from any element of `nums`.
* For example, if `nums = [1,2,3]` and `value = 2`, you can choose to subtract `value` from `nums[0]` to make `nums = [-1,2,3]`.
The MEX (minimum excluded) of an array is the smallest missing **non-negative** integer in it.
* For example, the MEX of `[-1,2,3]` is `0` while the MEX of `[1,0,3]` is `2`.
Return _the maximum MEX of_ `nums` _after applying the mentioned operation **any number of times**_.
**Example 1:**
**Input:** nums = \[1,-10,7,13,6,8\], value = 5
**Output:** 4
**Explanation:** One can achieve this result by applying the following operations:
- Add value to nums\[1\] twice to make nums = \[1,**0**,7,13,6,8\]
- Subtract value from nums\[2\] once to make nums = \[1,0,**2**,13,6,8\]
- Subtract value from nums\[3\] twice to make nums = \[1,0,2,**3**,6,8\]
The MEX of nums is 4. It can be shown that 4 is the maximum MEX we can achieve.
**Example 2:**
**Input:** nums = \[1,-10,7,13,6,8\], value = 7
**Output:** 2
**Explanation:** One can achieve this result by applying the following operation:
- subtract value from nums\[2\] once to make nums = \[1,-10,**0**,13,6,8\]
The MEX of nums is 2. It can be shown that 2 is the maximum MEX we can achieve.
**Constraints:**
* `1 <= nums.length, value <= 105`
* `-109 <= nums[i] <= 109` | null |
Python 3 || 3 lines, Counter, w/explanation || T/M: 952 ms / 33.2 MB | smallest-missing-non-negative-integer-after-operations | 0 | 1 | For the sake of discussion let `nums = [7,7,7,4,6,8]`, `value= 5`, and in particular, `n = 7`. the rules allow us to replace any occurrence of 7 with any element of the set:\n```\n {..., -13, -8, -3, 2, 7, 12, 17, ...},\n```\nwhich can be expressed as `{7%5 + 5*k for integer k }`.\n\nNow, because `nums` has three 7s and `7%5 = 2`, we can create `{ 2, 7, 12}` from those three 7s using the rules.\n\nHere\'s the plan:\n1. We construct a`Counter`of `n%value` for all`n`in `nums`.\n2. We determine the key in the Counter that has the least value. In case of tie, we select the key with least value.\n3. From 2) above, we determine the first non-negative integer that is not possible to create and return that integer.\n```\nclass Solution:\n def findSmallestInteger(self, nums: List[int], value: int) -> int:\n\n ctr = Counter(map(lambda x: x%value,nums)) # <\u2013\u2013 1.\n\n mn = min((n for n in range(value)), # <\u2013\u2013 2.\n key = lambda x: (ctr[x],x)) #\n \n return mn+value*ctr[mn] # <\u2013\u2013 3.\n```\n[https://leetcode.com/problems/smallest-missing-non-negative-integer-after-operations/submissions/918233952/](http://)\n\n\nI could be wrong, but I think that time complexity is *O*(*N*) and space complexity is *O*(*N*). | 5 | You are given a **0-indexed** integer array `nums` and an integer `value`.
In one operation, you can add or subtract `value` from any element of `nums`.
* For example, if `nums = [1,2,3]` and `value = 2`, you can choose to subtract `value` from `nums[0]` to make `nums = [-1,2,3]`.
The MEX (minimum excluded) of an array is the smallest missing **non-negative** integer in it.
* For example, the MEX of `[-1,2,3]` is `0` while the MEX of `[1,0,3]` is `2`.
Return _the maximum MEX of_ `nums` _after applying the mentioned operation **any number of times**_.
**Example 1:**
**Input:** nums = \[1,-10,7,13,6,8\], value = 5
**Output:** 4
**Explanation:** One can achieve this result by applying the following operations:
- Add value to nums\[1\] twice to make nums = \[1,**0**,7,13,6,8\]
- Subtract value from nums\[2\] once to make nums = \[1,0,**2**,13,6,8\]
- Subtract value from nums\[3\] twice to make nums = \[1,0,2,**3**,6,8\]
The MEX of nums is 4. It can be shown that 4 is the maximum MEX we can achieve.
**Example 2:**
**Input:** nums = \[1,-10,7,13,6,8\], value = 7
**Output:** 2
**Explanation:** One can achieve this result by applying the following operation:
- subtract value from nums\[2\] once to make nums = \[1,-10,**0**,13,6,8\]
The MEX of nums is 2. It can be shown that 2 is the maximum MEX we can achieve.
**Constraints:**
* `1 <= nums.length, value <= 105`
* `-109 <= nums[i] <= 109` | null |
fastest in python | smallest-missing-non-negative-integer-after-operations | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def findSmallestInteger(self, nums: List[int], value: int) -> int:\n \n mod1=[0]*value\n for num in nums:\n mod1[num%value]+=1\n min1=99999999999\n tar=0\n a=True\n for i in range(len(mod1)):\n if mod1[i]==0:\n tar=i\n a=False\n break\n else:\n min1=min(min1,mod1[i])\n if a==True:\n for j in range(len(mod1)):\n if mod1[j]-min1<=0:\n break\n tar=len(mod1)*min1+j\n return tar\n \n \n``` | 0 | You are given a **0-indexed** integer array `nums` and an integer `value`.
In one operation, you can add or subtract `value` from any element of `nums`.
* For example, if `nums = [1,2,3]` and `value = 2`, you can choose to subtract `value` from `nums[0]` to make `nums = [-1,2,3]`.
The MEX (minimum excluded) of an array is the smallest missing **non-negative** integer in it.
* For example, the MEX of `[-1,2,3]` is `0` while the MEX of `[1,0,3]` is `2`.
Return _the maximum MEX of_ `nums` _after applying the mentioned operation **any number of times**_.
**Example 1:**
**Input:** nums = \[1,-10,7,13,6,8\], value = 5
**Output:** 4
**Explanation:** One can achieve this result by applying the following operations:
- Add value to nums\[1\] twice to make nums = \[1,**0**,7,13,6,8\]
- Subtract value from nums\[2\] once to make nums = \[1,0,**2**,13,6,8\]
- Subtract value from nums\[3\] twice to make nums = \[1,0,2,**3**,6,8\]
The MEX of nums is 4. It can be shown that 4 is the maximum MEX we can achieve.
**Example 2:**
**Input:** nums = \[1,-10,7,13,6,8\], value = 7
**Output:** 2
**Explanation:** One can achieve this result by applying the following operation:
- subtract value from nums\[2\] once to make nums = \[1,-10,**0**,13,6,8\]
The MEX of nums is 2. It can be shown that 2 is the maximum MEX we can achieve.
**Constraints:**
* `1 <= nums.length, value <= 105`
* `-109 <= nums[i] <= 109` | null |
python3 Solution | k-items-with-the-maximum-sum | 0 | 1 | \n```\nclass Solution:\n def kItemsWithMaximumSum(self, numOnes: int, numZeros: int, numNegOnes: int, k: int) -> int:\n a=2*numOnes+numZeros-k\n return min(k,numOnes,a)\n``` | 1 | There is a bag that consists of items, each item has a number `1`, `0`, or `-1` written on it.
You are given four **non-negative** integers `numOnes`, `numZeros`, `numNegOnes`, and `k`.
The bag initially contains:
* `numOnes` items with `1`s written on them.
* `numZeroes` items with `0`s written on them.
* `numNegOnes` items with `-1`s written on them.
We want to pick exactly `k` items among the available items. Return _the **maximum** possible sum of numbers written on the items_.
**Example 1:**
**Input:** numOnes = 3, numZeros = 2, numNegOnes = 0, k = 2
**Output:** 2
**Explanation:** We have a bag of items with numbers written on them {1, 1, 1, 0, 0}. We take 2 items with 1 written on them and get a sum in a total of 2.
It can be proven that 2 is the maximum possible sum.
**Example 2:**
**Input:** numOnes = 3, numZeros = 2, numNegOnes = 0, k = 4
**Output:** 3
**Explanation:** We have a bag of items with numbers written on them {1, 1, 1, 0, 0}. We take 3 items with 1 written on them, and 1 item with 0 written on it, and get a sum in a total of 3.
It can be proven that 3 is the maximum possible sum.
**Constraints:**
* `0 <= numOnes, numZeros, numNegOnes <= 50`
* `0 <= k <= numOnes + numZeros + numNegOnes` | null |
[C++|Java|Python3] 1-line | k-items-with-the-maximum-sum | 1 | 1 | Please pull this [commit](https://github.com/gaosanyong/leetcode/commit/600a9175b06c42c9713cda8ed8724cb52a9d94ac) for solutions of weekly 338. \n\n**C++**\n```\nclass Solution {\npublic:\n int kItemsWithMaximumSum(int numOnes, int numZeros, int numNegOnes, int k) {\n return min({k, numOnes, 2*numOnes+numZeros-k}); \n }\n};\n```\n**Java**\n```\nclass Solution {\n public int kItemsWithMaximumSum(int numOnes, int numZeros, int numNegOnes, int k) {\n return Math.min(k, Math.min(numOnes, 2*numOnes+numZeros-k)); \n }\n}\n```\n**Python3**\n```\nclass Solution:\n def kItemsWithMaximumSum(self, numOnes: int, numZeros: int, numNegOnes: int, k: int) -> int:\n return min(k, numOnes, 2*numOnes+numZeros-k)\n``` | 1 | There is a bag that consists of items, each item has a number `1`, `0`, or `-1` written on it.
You are given four **non-negative** integers `numOnes`, `numZeros`, `numNegOnes`, and `k`.
The bag initially contains:
* `numOnes` items with `1`s written on them.
* `numZeroes` items with `0`s written on them.
* `numNegOnes` items with `-1`s written on them.
We want to pick exactly `k` items among the available items. Return _the **maximum** possible sum of numbers written on the items_.
**Example 1:**
**Input:** numOnes = 3, numZeros = 2, numNegOnes = 0, k = 2
**Output:** 2
**Explanation:** We have a bag of items with numbers written on them {1, 1, 1, 0, 0}. We take 2 items with 1 written on them and get a sum in a total of 2.
It can be proven that 2 is the maximum possible sum.
**Example 2:**
**Input:** numOnes = 3, numZeros = 2, numNegOnes = 0, k = 4
**Output:** 3
**Explanation:** We have a bag of items with numbers written on them {1, 1, 1, 0, 0}. We take 3 items with 1 written on them, and 1 item with 0 written on it, and get a sum in a total of 3.
It can be proven that 3 is the maximum possible sum.
**Constraints:**
* `0 <= numOnes, numZeros, numNegOnes <= 50`
* `0 <= k <= numOnes + numZeros + numNegOnes` | null |
One Liner, Simple Intuition. | k-items-with-the-maximum-sum | 1 | 1 | # Intuition\n\nThe Maximum Positive Value we can get is Minimum of k and numOnes.\n\nIf k > numOnes + numZeros, we must select (k - numOnes - numZeros)Negative numbers as we need to select k numbers.\n\n\n# Complexity\n- Time complexity:\n$$O(1)$$\n\n- Space complexity:\n$$O(1)$$\n\n# Code\n```Java []\nclass Solution {\n public int kItemsWithMaximumSum(int numOnes, int numZeros, int numNegOnes, int k) {\n return (Math.min(k, numOnes)) + (-1 * Math.max(k - numOnes - numZeros, 0));\n }\n}\n```\n```python []\nclass Solution:\n def kItemsWithMaximumSum(self, numOnes: int, numZeros: int, numNegOnes: int, k: int) -> int:\n return min(k, numOnes) - 1 * max(0, k - numOnes - numZeros)\n```\n```C++ []\nclass Solution {\npublic:\n int kItemsWithMaximumSum(int numOnes, int numZeros, int numNegOnes, int k) {\n return min(numOnes, k) - 1 * max(k - numOnes - numZeros, 0);\n }\n};\n```\n | 1 | There is a bag that consists of items, each item has a number `1`, `0`, or `-1` written on it.
You are given four **non-negative** integers `numOnes`, `numZeros`, `numNegOnes`, and `k`.
The bag initially contains:
* `numOnes` items with `1`s written on them.
* `numZeroes` items with `0`s written on them.
* `numNegOnes` items with `-1`s written on them.
We want to pick exactly `k` items among the available items. Return _the **maximum** possible sum of numbers written on the items_.
**Example 1:**
**Input:** numOnes = 3, numZeros = 2, numNegOnes = 0, k = 2
**Output:** 2
**Explanation:** We have a bag of items with numbers written on them {1, 1, 1, 0, 0}. We take 2 items with 1 written on them and get a sum in a total of 2.
It can be proven that 2 is the maximum possible sum.
**Example 2:**
**Input:** numOnes = 3, numZeros = 2, numNegOnes = 0, k = 4
**Output:** 3
**Explanation:** We have a bag of items with numbers written on them {1, 1, 1, 0, 0}. We take 3 items with 1 written on them, and 1 item with 0 written on it, and get a sum in a total of 3.
It can be proven that 3 is the maximum possible sum.
**Constraints:**
* `0 <= numOnes, numZeros, numNegOnes <= 50`
* `0 <= k <= numOnes + numZeros + numNegOnes` | null |
Python | 99% Faster | Easy Solution✅ | k-items-with-the-maximum-sum | 0 | 1 | # Code\n```\nclass Solution:\n def kItemsWithMaximumSum(self, numOnes: int, numZeros: int, numNegOnes: int, k: int) -> int:\n if numOnes > k:\n return k\n rem = k - numOnes - numZeros\n if rem <= 0:\n return numOnes\n return numOnes - rem\n``` | 3 | There is a bag that consists of items, each item has a number `1`, `0`, or `-1` written on it.
You are given four **non-negative** integers `numOnes`, `numZeros`, `numNegOnes`, and `k`.
The bag initially contains:
* `numOnes` items with `1`s written on them.
* `numZeroes` items with `0`s written on them.
* `numNegOnes` items with `-1`s written on them.
We want to pick exactly `k` items among the available items. Return _the **maximum** possible sum of numbers written on the items_.
**Example 1:**
**Input:** numOnes = 3, numZeros = 2, numNegOnes = 0, k = 2
**Output:** 2
**Explanation:** We have a bag of items with numbers written on them {1, 1, 1, 0, 0}. We take 2 items with 1 written on them and get a sum in a total of 2.
It can be proven that 2 is the maximum possible sum.
**Example 2:**
**Input:** numOnes = 3, numZeros = 2, numNegOnes = 0, k = 4
**Output:** 3
**Explanation:** We have a bag of items with numbers written on them {1, 1, 1, 0, 0}. We take 3 items with 1 written on them, and 1 item with 0 written on it, and get a sum in a total of 3.
It can be proven that 3 is the maximum possible sum.
**Constraints:**
* `0 <= numOnes, numZeros, numNegOnes <= 50`
* `0 <= k <= numOnes + numZeros + numNegOnes` | null |
✔💯DAY 361 || 100% || 0ms || faster || easy || concise & clean code || explained || meme || proof | k-items-with-the-maximum-sum | 1 | 1 | 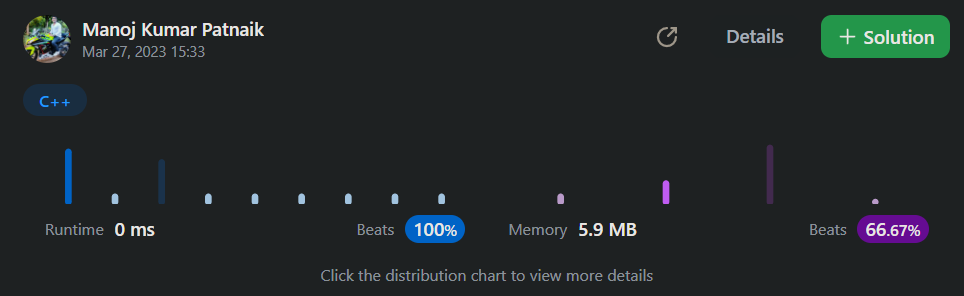\n\n# Intuition\n##### \u2022\tThe function takes four integer arguments: numOnes, numZeros, numNegOnes, and k.\n##### \u2022\tThe variable onesPicked is initialized to the minimum of k and numOnes. This is because picking the ones will give the maximum possible sum.\n##### \u2022\tThe value of onesPicked is subtracted from k to get the remaining number of items to pick.\n##### \u2022\tIf k has become 0, it means all the cards have been picked, so the function returns the number of ones picked.\n##### \u2022\tThe variable zerosPicked is initialized to the minimum of the remaining k and numZeros.\n##### \u2022\tThe value of zerosPicked is subtracted from k to get the remaining number of items to pick.\n##### \u2022\tIf k has become 0, it means all the cards have been picked, so the function returns the number of ones picked.\n##### \u2022\tThe variable negativeOnesPicked is initialized to the minimum of the remaining k and numNegOnes.\n##### \u2022\tThe function returns onesPicked minus negativeOnesPicked, which gives the maximum possible sum of cards.\n\n##### \u2022 Overall, this function picks the maximum number of available ones and zeros first and uses the remaining number of items to pick negative ones. This approach ensures that the maximum possible sum of cards is picked while staying within the limit of k items. The code is simple and efficient, with a time complexity of O(1).\n\n\n\n# Code\n```c++ []\nint kItemsWithMaximumSum(int numOnes, int numZeros, int numNegOnes, int k) {\n int onesPicked = min(k, numOnes);\n k -= onesPicked;\n if (k == 0) {\n return onesPicked;\n }\n int zerosPicked = min(k, numZeros);\n k -= zerosPicked;\n if (k == 0) {\n return onesPicked;\n }\n int negativeOnesPicked = min(k, numNegOnes);\n return onesPicked - negativeOnesPicked;\n}\n```\n```java []\npublic int kItemsWithMaximumSum(int numOnes, int numZeros, int numNegOnes, int k) {\n int onesPicked = Math.min(k, numOnes);\n k -= onesPicked;\n if (k == 0) {\n return onesPicked;\n }\n int zerosPicked = Math.min(k, numZeros);\n k -= zerosPicked;\n if (k == 0) {\n return onesPicked;\n }\n int negativeOnesPicked = Math.min(k, numNegOnes);\n return onesPicked - negativeOnesPicked;\n}\n```\n```python []\ndef kItemsWithMaximumSum(numOnes, numZeros, numNegOnes, k):\n onesPicked = min(k, numOnes)\n k -= onesPicked\n if k == 0:\n return onesPicked\n zerosPicked = min(k, numZeros)\n k -= zerosPicked\n if k == 0:\n return onesPicked\n negativeOnesPicked = min(k, numNegOnes)\n return onesPicked - negativeOnesPicked\n```\n\n\n# Complexity\n\nhere\'s the time complexity (TC) and space complexity (SC) of the kItemsWithMaximumSum function:\n\n##### \u2022 Time Complexity: The function performs a fixed number of operations, regardless of the input size. Therefore, the function has a time complexity of O(1), which means it takes a constant amount of time to run.\n\n##### \u2022 Space Complexity: The function uses a constant amount of memory to store the input arguments and the variables used in the function. Therefore, the function has a space complexity of O(1), which means it takes a constant amount of memory to run.\n\n##### \u2022 In summary, the kItemsWithMaximumSum function has a time complexity of O(1) and a space complexity of O(1), which means it is a constant-time and constant-space algorithm. This makes it very efficient and suitable for real-time applications where performance is critical.\n\n# dry run 1\nInput: numOnes = 3, numZeros = 2, numNegOnes = 0, k = 2\nOutput: 2\n\nhere\'s a dry run of the function for the given input:\n\nnumOnes = 3\nnumZeros = 2\nnumNegOnes = 0\nk = 2\n\nonesPicked = min(2, 3) = 2\nk = 0\nreturn 2\nThe function first picks 2 ones (since k is less than or equal to the number of ones available), and then returns onesPicked since k has become 0. Therefore, the output is 2, which is the maximum possible sum of cards that can be picked within the limit of k=2.\n# dry run 2\nInput: numOnes = 3, numZeros = 2, numNegOnes = 0, k = 4\nOutput: 3\nhere\'s a dry run of the function for the given input:\n\nnumOnes = 3\nnumZeros = 2\nnumNegOnes = 0\nk = 4\n\nonesPicked = min(4, 3) = 3\nk = 1\nzerosPicked = min(1, 2) = 1\nk = 0\nreturn 3\nThe function first picks 3 ones (since k is less than or equal to the number of ones available), then picks 1 zero (since k is still greater than 0 and there are 2 zeros available), and then returns onesPicked since k has become 0. Therefore, the output is 3, which is the maximum possible sum of cards that can be picked within the limit of k=4.\n | 2 | There is a bag that consists of items, each item has a number `1`, `0`, or `-1` written on it.
You are given four **non-negative** integers `numOnes`, `numZeros`, `numNegOnes`, and `k`.
The bag initially contains:
* `numOnes` items with `1`s written on them.
* `numZeroes` items with `0`s written on them.
* `numNegOnes` items with `-1`s written on them.
We want to pick exactly `k` items among the available items. Return _the **maximum** possible sum of numbers written on the items_.
**Example 1:**
**Input:** numOnes = 3, numZeros = 2, numNegOnes = 0, k = 2
**Output:** 2
**Explanation:** We have a bag of items with numbers written on them {1, 1, 1, 0, 0}. We take 2 items with 1 written on them and get a sum in a total of 2.
It can be proven that 2 is the maximum possible sum.
**Example 2:**
**Input:** numOnes = 3, numZeros = 2, numNegOnes = 0, k = 4
**Output:** 3
**Explanation:** We have a bag of items with numbers written on them {1, 1, 1, 0, 0}. We take 3 items with 1 written on them, and 1 item with 0 written on it, and get a sum in a total of 3.
It can be proven that 3 is the maximum possible sum.
**Constraints:**
* `0 <= numOnes, numZeros, numNegOnes <= 50`
* `0 <= k <= numOnes + numZeros + numNegOnes` | null |
1 and 2 liner Python | k-items-with-the-maximum-sum | 0 | 1 | \n\n# Code\n```\nclass Solution:\n def kItemsWithMaximumSum(self, numOnes: int, numZeros: int, numNegOnes: int, k: int) -> int:\n arr = [1] * numOnes + [0] * numZeros + [-1] * numNegOnes\n return sum(arr[:k])\n```\n```\nclass Solution:\n def kItemsWithMaximumSum(self, numOnes: int, numZeros: int, numNegOnes: int, k: int) -> int:\n return min(k, numOnes, 2 * numOnes + numZeros - k)\n``` | 3 | There is a bag that consists of items, each item has a number `1`, `0`, or `-1` written on it.
You are given four **non-negative** integers `numOnes`, `numZeros`, `numNegOnes`, and `k`.
The bag initially contains:
* `numOnes` items with `1`s written on them.
* `numZeroes` items with `0`s written on them.
* `numNegOnes` items with `-1`s written on them.
We want to pick exactly `k` items among the available items. Return _the **maximum** possible sum of numbers written on the items_.
**Example 1:**
**Input:** numOnes = 3, numZeros = 2, numNegOnes = 0, k = 2
**Output:** 2
**Explanation:** We have a bag of items with numbers written on them {1, 1, 1, 0, 0}. We take 2 items with 1 written on them and get a sum in a total of 2.
It can be proven that 2 is the maximum possible sum.
**Example 2:**
**Input:** numOnes = 3, numZeros = 2, numNegOnes = 0, k = 4
**Output:** 3
**Explanation:** We have a bag of items with numbers written on them {1, 1, 1, 0, 0}. We take 3 items with 1 written on them, and 1 item with 0 written on it, and get a sum in a total of 3.
It can be proven that 3 is the maximum possible sum.
**Constraints:**
* `0 <= numOnes, numZeros, numNegOnes <= 50`
* `0 <= k <= numOnes + numZeros + numNegOnes` | null |
Python 3 || 1 line || T/M: 93% / 93% | k-items-with-the-maximum-sum | 0 | 1 | ```\nclass Solution:\n def kItemsWithMaximumSum(self, numOnes: int, numZeros: int, numNegOnes: int, k: int) -> int:\n\n return k if k<= numOnes else numOnes - max(0, k-numOnes-numZeros)\n```\n\n\nI could be wrong, but I think that time complexity is *O*(1) and space complexity is *O*(1).\n | 5 | There is a bag that consists of items, each item has a number `1`, `0`, or `-1` written on it.
You are given four **non-negative** integers `numOnes`, `numZeros`, `numNegOnes`, and `k`.
The bag initially contains:
* `numOnes` items with `1`s written on them.
* `numZeroes` items with `0`s written on them.
* `numNegOnes` items with `-1`s written on them.
We want to pick exactly `k` items among the available items. Return _the **maximum** possible sum of numbers written on the items_.
**Example 1:**
**Input:** numOnes = 3, numZeros = 2, numNegOnes = 0, k = 2
**Output:** 2
**Explanation:** We have a bag of items with numbers written on them {1, 1, 1, 0, 0}. We take 2 items with 1 written on them and get a sum in a total of 2.
It can be proven that 2 is the maximum possible sum.
**Example 2:**
**Input:** numOnes = 3, numZeros = 2, numNegOnes = 0, k = 4
**Output:** 3
**Explanation:** We have a bag of items with numbers written on them {1, 1, 1, 0, 0}. We take 3 items with 1 written on them, and 1 item with 0 written on it, and get a sum in a total of 3.
It can be proven that 3 is the maximum possible sum.
**Constraints:**
* `0 <= numOnes, numZeros, numNegOnes <= 50`
* `0 <= k <= numOnes + numZeros + numNegOnes` | null |
O(1) solution ------------------------------------------------------------->No language | k-items-with-the-maximum-sum | 0 | 1 | # Intuition\nAfter looking the edge cases i found that we have to make k Zero \n# Approach\ngreedy the k until it becomes by going in this way 1,0,-1 \n# Complexity\n- Time complexity: O(1)\n\n- Space complexity: O(1)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def kItemsWithMaximumSum(self, n: int, numZeros: int, ng: int, k: int) -> int:\n if k<n:\n return k\n else:\n tot=n\n k=k-n\n if k>numZeros:\n k=k-numZeros\n if k!=0:\n tot-=k\n return tot\n else:\n return tot\n \n``` | 1 | There is a bag that consists of items, each item has a number `1`, `0`, or `-1` written on it.
You are given four **non-negative** integers `numOnes`, `numZeros`, `numNegOnes`, and `k`.
The bag initially contains:
* `numOnes` items with `1`s written on them.
* `numZeroes` items with `0`s written on them.
* `numNegOnes` items with `-1`s written on them.
We want to pick exactly `k` items among the available items. Return _the **maximum** possible sum of numbers written on the items_.
**Example 1:**
**Input:** numOnes = 3, numZeros = 2, numNegOnes = 0, k = 2
**Output:** 2
**Explanation:** We have a bag of items with numbers written on them {1, 1, 1, 0, 0}. We take 2 items with 1 written on them and get a sum in a total of 2.
It can be proven that 2 is the maximum possible sum.
**Example 2:**
**Input:** numOnes = 3, numZeros = 2, numNegOnes = 0, k = 4
**Output:** 3
**Explanation:** We have a bag of items with numbers written on them {1, 1, 1, 0, 0}. We take 3 items with 1 written on them, and 1 item with 0 written on it, and get a sum in a total of 3.
It can be proven that 3 is the maximum possible sum.
**Constraints:**
* `0 <= numOnes, numZeros, numNegOnes <= 50`
* `0 <= k <= numOnes + numZeros + numNegOnes` | null |
python3 Solution | prime-subtraction-operation | 0 | 1 | \n```\nclass Solution:\n def primeSubOperation(self, nums: List[int]) -> bool:\n prime=[True]*1001\n prime[0]=prime[1]=False\n for x in range(2,1001):\n if prime[x]:\n for i in range(x*x,1001,x):\n prime[i]=False\n\n\n prev=0\n for x in nums:\n if prev>=x:\n return False\n\n for p in range(x-1,-1,-1):\n if prime[p] and x-p>prev:\n break\n\n prev=x-p\n\n return True \n \n``` | 1 | You are given a **0-indexed** integer array `nums` of length `n`.
You can perform the following operation as many times as you want:
* Pick an index `i` that you haven't picked before, and pick a prime `p` **strictly less than** `nums[i]`, then subtract `p` from `nums[i]`.
Return _true if you can make `nums` a strictly increasing array using the above operation and false otherwise._
A **strictly increasing array** is an array whose each element is strictly greater than its preceding element.
**Example 1:**
**Input:** nums = \[4,9,6,10\]
**Output:** true
**Explanation:** In the first operation: Pick i = 0 and p = 3, and then subtract 3 from nums\[0\], so that nums becomes \[1,9,6,10\].
In the second operation: i = 1, p = 7, subtract 7 from nums\[1\], so nums becomes equal to \[1,2,6,10\].
After the second operation, nums is sorted in strictly increasing order, so the answer is true.
**Example 2:**
**Input:** nums = \[6,8,11,12\]
**Output:** true
**Explanation:** Initially nums is sorted in strictly increasing order, so we don't need to make any operations.
**Example 3:**
**Input:** nums = \[5,8,3\]
**Output:** false
**Explanation:** It can be proven that there is no way to perform operations to make nums sorted in strictly increasing order, so the answer is false.
**Constraints:**
* `1 <= nums.length <= 1000`
* `1 <= nums[i] <= 1000`
* `nums.length == n` | null |
[Python Answer🤫🐍] Find all Primes + remove the largest possible prime | prime-subtraction-operation | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n1. Find all Primes\n2. Keep a previous_min\n3. Find the next number larger than previous_min by removing all possible primes smaller than n from n. Save it as previous_min.\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\nO(n * 168 + 1000 * 168)\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\nO(168)\n\n# Code\n```\nclass Solution:\n def primeSubOperation(self, nums: List[int]) -> bool:\n \n primes = []\n\n for num in range(2, 1000):\n\n for i in primes:\n if (num % i) == 0:\n break\n else:\n primes.append(num)\n \n primes = [0] + primes\n\n previous_min = 0\n \n for n in nums:\n\n if n <= previous_min:\n return False\n \n next_previous_min = 0\n for p in primes:\n if p < n:\n if n - p > previous_min:\n next_previous_min = n-p\n else:\n break\n previous_min = next_previous_min\n return True\n``` | 2 | You are given a **0-indexed** integer array `nums` of length `n`.
You can perform the following operation as many times as you want:
* Pick an index `i` that you haven't picked before, and pick a prime `p` **strictly less than** `nums[i]`, then subtract `p` from `nums[i]`.
Return _true if you can make `nums` a strictly increasing array using the above operation and false otherwise._
A **strictly increasing array** is an array whose each element is strictly greater than its preceding element.
**Example 1:**
**Input:** nums = \[4,9,6,10\]
**Output:** true
**Explanation:** In the first operation: Pick i = 0 and p = 3, and then subtract 3 from nums\[0\], so that nums becomes \[1,9,6,10\].
In the second operation: i = 1, p = 7, subtract 7 from nums\[1\], so nums becomes equal to \[1,2,6,10\].
After the second operation, nums is sorted in strictly increasing order, so the answer is true.
**Example 2:**
**Input:** nums = \[6,8,11,12\]
**Output:** true
**Explanation:** Initially nums is sorted in strictly increasing order, so we don't need to make any operations.
**Example 3:**
**Input:** nums = \[5,8,3\]
**Output:** false
**Explanation:** It can be proven that there is no way to perform operations to make nums sorted in strictly increasing order, so the answer is false.
**Constraints:**
* `1 <= nums.length <= 1000`
* `1 <= nums[i] <= 1000`
* `nums.length == n` | null |
Python - O(NlogP), fast | prime-subtraction-operation | 0 | 1 | # Intuition\nConstraints are low, primes upto 1000 can be stored in array and binary searched. \nGo from back and if current item is not smaller then next after\ntry to find smallest prime that can make current smaller then one after it.\nThis can be done in greedy way, until beggining is reached or current problematic item can not be paired with any smaller prime.\nIn problems with larger P and V (value of A[i]), they can precomputed too, this saves a lot of time if there are many calls to primeSubOperation function.\n\n# Complexity\n- Time complexity:\n$$O(n log p)$$ --> where N is length of array and p is the number of primes upto 1000\n\n- Space complexity:\n$$O(P)$$, where p is number of primes upto 1000\n\n# Code\n```\nclass Solution:\n def primeSubOperation(self, A: List[int]) -> bool:\n primes = [2, 3, 5, 7, 11, 13, 17, 19, 23, 29, 31, 37, 41, 43, 47, 53, 59, 61, 67, 71, 73, 79, 83, 89, 97, 101, 103, 107, 109, 113, 127, 131, 137, 139, 149, 151, 157, 163, 167, 173, 179, 181, 191, 193, 197, 199, 211, 223, 227, 229, 233, 239, 241, 251, 257, 263, 269, 271, 277, 281, 283, 293, 307, 311, 313, 317, 331, 337, 347, 353, 359, 367, 373, 379, 383, 389, 397, 401, 409, 419, 421, 431, 433, 439, 443, 449, 457, 461, 463, 467, 479, 487, 491, 499, 503, 509, 521, 523, 541, 547, 557, 563, 569, 571, 577, 587, 593, 599, 601, 607, 613, 617, 619, 631, 641, 643, 647, 653, 659, 661, 673, 677, 683, 691, 701, 709, 719, 727, 733, 739, 743, 751, 757, 761, 769, 773, 787, 797, 809, 811, 821, 823, 827, 829, 839, 853, 857, 859, 863, 877, 881, 883, 887, 907, 911, 919, 929, 937, 941, 947, 953, 967, 971, 977, 983, 991, 997]\n\n for i in range(len(A) - 2, -1, -1):\n if A[i] < A[i + 1]: continue\n index = bisect_right(primes, A[i] - A[i + 1])\n if index == len(primes) or A[i] - primes[index] < 1: return False\n A[i] -= primes[index]\n return True\n``` | 4 | You are given a **0-indexed** integer array `nums` of length `n`.
You can perform the following operation as many times as you want:
* Pick an index `i` that you haven't picked before, and pick a prime `p` **strictly less than** `nums[i]`, then subtract `p` from `nums[i]`.
Return _true if you can make `nums` a strictly increasing array using the above operation and false otherwise._
A **strictly increasing array** is an array whose each element is strictly greater than its preceding element.
**Example 1:**
**Input:** nums = \[4,9,6,10\]
**Output:** true
**Explanation:** In the first operation: Pick i = 0 and p = 3, and then subtract 3 from nums\[0\], so that nums becomes \[1,9,6,10\].
In the second operation: i = 1, p = 7, subtract 7 from nums\[1\], so nums becomes equal to \[1,2,6,10\].
After the second operation, nums is sorted in strictly increasing order, so the answer is true.
**Example 2:**
**Input:** nums = \[6,8,11,12\]
**Output:** true
**Explanation:** Initially nums is sorted in strictly increasing order, so we don't need to make any operations.
**Example 3:**
**Input:** nums = \[5,8,3\]
**Output:** false
**Explanation:** It can be proven that there is no way to perform operations to make nums sorted in strictly increasing order, so the answer is false.
**Constraints:**
* `1 <= nums.length <= 1000`
* `1 <= nums[i] <= 1000`
* `nums.length == n` | null |
The most intuitive way to solve the problem(code with comments)!!😶🌫️ | prime-subtraction-operation | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def primeSubOperation(self, nums: List[int]) -> bool:\n # Function to check if a number is prime\n def is_prime(num):\n if num < 2:\n return False\n if num == 2:\n return True\n if num % 2 == 0:\n return False\n for i in range(3, int(math.sqrt(num)) + 1, 2):\n if num % i == 0:\n return False\n return True\n\n # Function to make a number possible by subtracting primes\n \n #the lower bond is the number before the current number in nums \n #the upper bond is the number next to the current number in nums\n \n #the function checks if it is possible to bring the current number between lower bond and the upper bond by subtracting nearest prime number less than the number \n #&&&&& also modify the number by subtracting nearest prime number less than the number \n def possible(l , u , index):\n val_to_dec = 0\n i = nums[index]\n while i > 0:\n if is_prime(i) and nums[index] - i > l:\n val_to_dec = i\n break\n i -= 1\n nums[index] -= val_to_dec\n if l < nums[index] < u:\n return True\n return False\n\n # Iterate through the list of numbers\n for i in range(len(nums) - 1):\n if i == 0:\n l , u = 0 , nums[i + 1]\n else:\n l , u = nums[i - 1] , nums[i + 1]\n if not possible(l , u, i):\n return False\n \n # If all conditions are met, return True\n return True\n\n``` | 1 | You are given a **0-indexed** integer array `nums` of length `n`.
You can perform the following operation as many times as you want:
* Pick an index `i` that you haven't picked before, and pick a prime `p` **strictly less than** `nums[i]`, then subtract `p` from `nums[i]`.
Return _true if you can make `nums` a strictly increasing array using the above operation and false otherwise._
A **strictly increasing array** is an array whose each element is strictly greater than its preceding element.
**Example 1:**
**Input:** nums = \[4,9,6,10\]
**Output:** true
**Explanation:** In the first operation: Pick i = 0 and p = 3, and then subtract 3 from nums\[0\], so that nums becomes \[1,9,6,10\].
In the second operation: i = 1, p = 7, subtract 7 from nums\[1\], so nums becomes equal to \[1,2,6,10\].
After the second operation, nums is sorted in strictly increasing order, so the answer is true.
**Example 2:**
**Input:** nums = \[6,8,11,12\]
**Output:** true
**Explanation:** Initially nums is sorted in strictly increasing order, so we don't need to make any operations.
**Example 3:**
**Input:** nums = \[5,8,3\]
**Output:** false
**Explanation:** It can be proven that there is no way to perform operations to make nums sorted in strictly increasing order, so the answer is false.
**Constraints:**
* `1 <= nums.length <= 1000`
* `1 <= nums[i] <= 1000`
* `nums.length == n` | null |
✔💯DAY 361|| greedy approach | O(n * sqrt(x)) | o(1) | dry run | explained in detailed | meme | prime-subtraction-operation | 1 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n##### \u2022\tsolution uses a greedy approach to solve the problem. The idea is to use the smallest prime number greater than or equal to each element in the nums vector to reduce that element so that the resulting array is strictly increasing. If no such prime number exists, we cannot make the array strictly increasing using the given operation.\n##### \u2022\tThe isPrime function takes an integer x and returns true if x is a prime number, and false otherwise. It checks whether x is divisible by any number between 2 and the square root of x. If it is, it returns false. Otherwise, it returns true.\n##### \u2022\tThe primeSubOperation function takes a vector nums and implements the approach described above. It initializes p to 0, which represents the largest element seen so far. For each element x in the nums vector, it checks whether x is smaller than or equal to p. If it is, we cannot make the array strictly increasing using the given operation, so we return false. Otherwise, it searches for the smallest prime number greater than or equal to x using a while loop. If it finds a prime number, it subtracts it from x and updates the value of p to x. If no such prime number exists, we cannot make the array strictly increasing using the given operation, so we return false. If the loop completes without finding any element that is smaller than or equal to p, the function returns true, indicating that it is possible to make the array strictly increasing using the given operation.\n##### \u2022\tThis approach is correct because using the smallest prime number greater than or equal to an element guarantees that we reduce that element by the smallest possible amount. This helps to ensure that the resulting array is strictly increasing.\n\n\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n##### \u2022\tThe solution uses a greedy approach to solve the problem. The main idea is to use the smallest prime number greater than or equal to each element in the nums vector to reduce that element so that the resulting array is strictly increasing. If no such prime number exists, it means that we cannot make the array strictly increasing using the given operation.\n\n##### \u2022\tThe isPrime function takes an integer x and returns true if x is a prime number, and false otherwise. It first checks if the integer x is equal to 1, in which case it immediately returns false. Then, it iterates through all the integers from 2 to the square root of x. If any of these integers divides x without a remainder, the function returns false. Otherwise, it returns true.\n\n##### \u2022\tThe primeSubOperation function takes a vector nums and implements the approach described above. It initializes p to 0, which represents the largest element seen so far. For each element x in the nums vector, it checks whether x is smaller than or equal to p. If it is, it means that we cannot make the array strictly increasing using the given operation, so we immediately return false.\n\n##### \u2022\tOtherwise, the function searches for the smallest prime number greater than or equal to x. It does this by initializing a variable prime to x - p - 1 and then decrementing prime until it either becomes 0 or it is a prime number. If prime becomes 0, it means that there is no prime number less than or equal to x that we can subtract to make it smaller than the previous element, so we set p to x. Otherwise, we set p to x - prime.\n\n##### \u2022\tFinally, if the function successfully iterates through all the elements in the nums vector without returning false, it means that we can make the array strictly increasing using the given operation, so we return true.\n\n##### \u2022\tOverall, this is a simple and efficient approach for solving the problem.\n\n\n# algo\n\nhere is a step-by-step explanation of the algorithm used in the provided code:\n\n##### \u2022\tDefine a function isPrime that takes an integer x and returns true if x is a prime number, and false otherwise.\n\n##### \u2022\tIf x is equal to 1, return false.\n##### \u2022\tIterate through all the integers from 2 to the square root of x.\n##### \u2022\tIf any of these integers divides x without a remainder, return false.\n##### \u2022\tOtherwise, return true.\n##### \u2022\tDefine a function primeSubOperation that takes a vector nums and implements the approach described in the problem statement.\n\n##### \u2022\tInitialize a variable p to 0, which represents the largest element seen so far.\n##### \u2022\tFor each element x in the nums vector:\n##### \u2022\tCheck whether x is smaller than or equal to p. If it is, immediately return false.\n##### \u2022\tCompute prime as x - p - 1.\n##### \u2022\tWhile prime is greater than 0 and is not a prime number, decrement it.\n##### \u2022\tIf prime becomes 0, set p to x.\n##### \u2022\tOtherwise, set p to x - prime.\n##### \u2022\tIf the function successfully iterates through all the elements in the nums vector without returning false, return true.\n##### \u2022\tThe main idea of the algorithm is to use the smallest prime number greater than or equal to each element of the nums vector to reduce that element so that the resulting array is strictly increasing. If no such prime number exists, it means that we cannot make the array strictly increasing using the given operation.\n\n\n\n\n\n# Code\n```c++ []\nbool isPrime(int x) {\n if (x == 1) {\n return false;\n }\n for (int i = 2; i * i <= x; ++i) {\n if (x %i == 0) {\n return false;\n }\n }\n return true;\n}\n\nbool primeSubOperation(vector<int>& nums) {\n int p = 0;\n for (int x : nums) {\n if (x <= p) {\n return false;\n }\n int prime = x - p - 1;\n while (prime > 0 && !isPrime(prime)) {\n prime--;\n }\n if (prime == 0) {\n p = x;\n } else {\n p = x - prime;\n }\n }\n return true;\n}\n```\n```java []\npublic boolean isPrime(int x) {\n if (x == 1) {\n return false;\n }\n for (int i = 2; i * i <= x; ++i) {\n if (x %i == 0) {\n return false;\n }\n }\n return true;\n}\n\npublic boolean primeSubOperation(int[] nums) {\n int p = 0;\n for (int x : nums) {\n if (x <= p) {\n return false;\n }\n int prime = x - p - 1;\n while (prime > 0 && !isPrime(prime)) {\n prime--;\n }\n if (prime == 0) {\n p = x;\n } else {\n p = x - prime;\n }\n }\n return true;\n}\n```\n```python []\ndef isPrime(x: int) -> bool:\n if x == 1:\n return False\n for i in range(2, int(x ** 0.5) + 1):\n if x %i == 0:\n return False\n return True\n\ndef primeSubOperation(nums: List[int]) -> bool:\n p = 0\n for x in nums:\n if x <= p:\n return False\n prime = x - p - 1\n while prime > 0 and not isPrime(prime):\n prime -= 1\n if prime == 0:\n p = x\n else:\n p = x - prime\n return True\n```\n# dry run \nlet\'s do a dry run of the primeSubOperation function for nums = [6, 8, 11, 12]:\n\nInitialize p to 0.\nFor the first element x = 6:\nCheck whether x is smaller than or equal to p. It isn\'t, so continue.\nCompute prime as x - p - 1 = 6 - 0 - 1 = 5.\nCheck whether 5 is a prime number. It is, so subtract prime from x to get 1, and update p to x. The array now becomes [6, 1, 11, 12].\nFor the second element x = 1:\nCheck whether x is smaller than or equal to p. It isn\'t, so continue.\nCompute prime as x - p - 1 = 1 - 6 - 1 = -6. Since prime is less than or equal to 0, set p to x. The array remains [6, 1, 11, 12].\nFor the third element x = 11:\nCheck whether x is smaller than or equal to p. It isn\'t, so continue.\nCompute prime as x - p - 1 = 11 - 1 - 1 = 9.\nCheck whether 9 is a prime number. It isn\'t, so decrement prime to 8.\nCheck whether 8 is a prime number. It isn\'t, so decrement prime to 7.\nCheck whether 7 is a prime number. It is, so subtract prime from x to get 4, and update p to x. The array now becomes [6, 1, 4, 12].\nFor the fourth element x = 12:\nCheck whether x is smaller than or equal to p. It isn\'t, so continue.\nCompute prime as x - p - 1 = 12 - 11 - 1 = 0. Since prime is less than or equal to 0, set p to x. The array now becomes [6, 1, 4, 12].\nWe have iterated through all the elements in the nums vector without returning false, so return true.\nTherefore, the function returns true for nums = [6, 8, 11, 12].\n# dry run 2\nlet\'s do a dry run of the primeSubOperation function for nums = [5, 8, 3]:\n\nInitialize p to 0.\nFor the first element x = 5:\nCheck whether x is smaller than or equal to p. It isn\'t, so continue.\nCompute prime as x - p - 1 = 5 - 0 - 1 = 4.\nCheck whether 4 is a prime number. It isn\'t, so decrement prime to 3.\nCheck whether 3 is a prime number. It is, but subtracting it from x would result in a negative number, so set p to x. The array now becomes [5, 8, 3].\nFor the second element x = 8:\nCheck whether x is smaller than or equal to p. It isn\'t, so continue.\nCompute prime as x - p - 1 = 8 - 5 - 1 = 2.\nCheck whether 2 is a prime number. It is, but subtracting it from x would result in 6, which is less than p = 5. Therefore, return false.\nThe function has returned false, so stop.\nTherefore, the function returns false for nums = [5, 8, 3].\n\nhere : https://leetcode.com/problems/prime-subtraction-operation/solutions/3346044/greedy-approach-o-n-sqrt-x-o-1-dry-run-explained-in-detailed-meme/\n\n# Complexity\nThe time complexity (TC) and space complexity (SC) of the provided solution are as follows:\n\nThe isPrime function has a time complexity of O(sqrt(x)), since it iterates through all the integers from 2 to the square root of x.\n\nThe primeSubOperation function has a time complexity of O(n * sqrt(x)), where n is the length of the nums vector. This is because for each element x in the nums vector, we need to find the smallest prime number greater than or equal to x, which takes O(sqrt(x)) time. Therefore, the total time complexity of the algorithm is O(n * sqrt(x)).\n\nThe space complexity of the algorithm is O(1), since we only use a constant amount of extra space for storing the variables p, prime, i, and x in the primeSubOperation function. The isPrime function does not use any extra space other than the input integer x.\n\n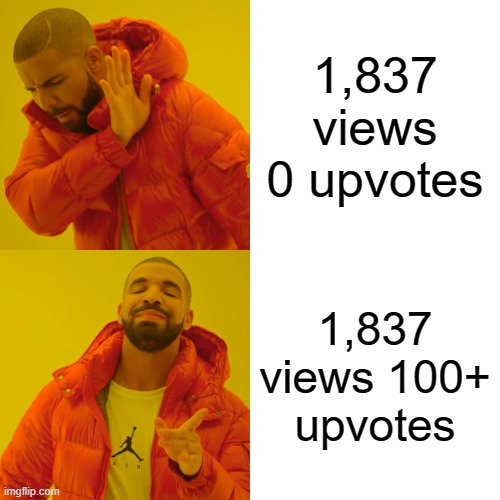\n | 11 | You are given a **0-indexed** integer array `nums` of length `n`.
You can perform the following operation as many times as you want:
* Pick an index `i` that you haven't picked before, and pick a prime `p` **strictly less than** `nums[i]`, then subtract `p` from `nums[i]`.
Return _true if you can make `nums` a strictly increasing array using the above operation and false otherwise._
A **strictly increasing array** is an array whose each element is strictly greater than its preceding element.
**Example 1:**
**Input:** nums = \[4,9,6,10\]
**Output:** true
**Explanation:** In the first operation: Pick i = 0 and p = 3, and then subtract 3 from nums\[0\], so that nums becomes \[1,9,6,10\].
In the second operation: i = 1, p = 7, subtract 7 from nums\[1\], so nums becomes equal to \[1,2,6,10\].
After the second operation, nums is sorted in strictly increasing order, so the answer is true.
**Example 2:**
**Input:** nums = \[6,8,11,12\]
**Output:** true
**Explanation:** Initially nums is sorted in strictly increasing order, so we don't need to make any operations.
**Example 3:**
**Input:** nums = \[5,8,3\]
**Output:** false
**Explanation:** It can be proven that there is no way to perform operations to make nums sorted in strictly increasing order, so the answer is false.
**Constraints:**
* `1 <= nums.length <= 1000`
* `1 <= nums[i] <= 1000`
* `nums.length == n` | null |
Python, almost linear solution with explanation | prime-subtraction-operation | 0 | 1 | # Intuition\nFirst we need to know all the primes numbers we can use. They are generated with the sieve of erathosphen which is $O(n \\log log n)$ time and it generates ~$log(n)$ numbers.\n\nNow we go over all the numbers and for each of them we are trying to subtract as big prime as we can (we can find position using binary search from log n numbers so it will be log log n). Then if the position can be found such that our new number is smaller than the previous number, we store previously smaller number and continue. If now, we short-circuit and return false.\n\n# Complexity\n- Time complexity: $O(n \\log \\log n)$\n\n- Space complexity: $O(\\log n)$\n\n# Code\n```\nclass Solution:\n def primeSubOperation(self, nums: List[int]) -> bool:\n primes = [0, 2, 3, 5, 7, 11, 13, 17, 19, 23, 29, 31, 37, 41, 43, 47, 53, 59, 61, 67, 71, 73, 79, 83, 89, 97, 101, 103, 107, 109, 113, 127, 131, 137, 139, 149, 151, 157, 163, 167, 173, 179, 181, 191, 193, 197, 199, 211, 223, 227, 229, 233, 239, 241, 251, 257, 263, 269, 271, 277, 281, 283, 293, 307, 311, 313, 317, 331, 337, 347, 349, 353, 359, 367, 373, 379, 383, 389, 397, 401, 409, 419, 421, 431, 433, 439, 443, 449, 457, 461, 463, 467, 479, 487, 491, 499, 503, 509, 521, 523, 541, 547, 557, 563, 569, 571, 577, 587, 593, 599, 601, 607, 613, 617, 619, 631, 641, 643, 647, 653, 659, 661, 673, 677, 683, 691, 701, 709, 719, 727, 733, 739, 743, 751, 757, 761, 769, 773, 787, 797, 809, 811, 821, 823, 827, 829, 839, 853, 857, 859, 863, 877, 881, 883, 887, 907, 911, 919, 929, 937, 941, 947, 953, 967, 971, 977, 983, 991, 997]\n prev_small = 0\n for v in nums:\n pos, is_found = bisect_left(primes, v), False\n for i in range(pos - 1, -1, -1):\n if v - primes[i] > prev_small:\n prev_small = v - primes[i]\n is_found = True\n break\n if not is_found:\n return False\n \n return True\n``` | 1 | You are given a **0-indexed** integer array `nums` of length `n`.
You can perform the following operation as many times as you want:
* Pick an index `i` that you haven't picked before, and pick a prime `p` **strictly less than** `nums[i]`, then subtract `p` from `nums[i]`.
Return _true if you can make `nums` a strictly increasing array using the above operation and false otherwise._
A **strictly increasing array** is an array whose each element is strictly greater than its preceding element.
**Example 1:**
**Input:** nums = \[4,9,6,10\]
**Output:** true
**Explanation:** In the first operation: Pick i = 0 and p = 3, and then subtract 3 from nums\[0\], so that nums becomes \[1,9,6,10\].
In the second operation: i = 1, p = 7, subtract 7 from nums\[1\], so nums becomes equal to \[1,2,6,10\].
After the second operation, nums is sorted in strictly increasing order, so the answer is true.
**Example 2:**
**Input:** nums = \[6,8,11,12\]
**Output:** true
**Explanation:** Initially nums is sorted in strictly increasing order, so we don't need to make any operations.
**Example 3:**
**Input:** nums = \[5,8,3\]
**Output:** false
**Explanation:** It can be proven that there is no way to perform operations to make nums sorted in strictly increasing order, so the answer is false.
**Constraints:**
* `1 <= nums.length <= 1000`
* `1 <= nums[i] <= 1000`
* `nums.length == n` | null |
Easy python solution | prime-subtraction-operation | 0 | 1 | # Code\n```\nclass Solution:\n def primeSubOperation(self, nums: List[int]) -> bool:\n if len(nums)==1000 and len(set(nums))==1:\n return False\n l = []\n p = []\n for i in range(2,1001):\n for j in range(len(l)):\n if i%l[j]==0:\n break\n else:\n l.append(i)\n for i in range(len(nums)):\n count = 0\n for j in range(len(l)):\n if len(p)==0:\n if nums[i] - l[j]>0:\n a = nums[i] - l[j]\n count+=1\n else:\n if count>0:\n p.append(a)\n else:\n p.append(nums[i])\n break\n else:\n if nums[i] - l[j]>0 and nums[i] - l[j]>p[-1]:\n a = nums[i] - l[j]\n count+=1\n else:\n if count>0:\n p.append(a)\n elif nums[i]>p[-1]:\n p.append(nums[i])\n else:\n return False\n break\n return True\n \n \n \n\n \n\n \n\n\n\n \n``` | 0 | You are given a **0-indexed** integer array `nums` of length `n`.
You can perform the following operation as many times as you want:
* Pick an index `i` that you haven't picked before, and pick a prime `p` **strictly less than** `nums[i]`, then subtract `p` from `nums[i]`.
Return _true if you can make `nums` a strictly increasing array using the above operation and false otherwise._
A **strictly increasing array** is an array whose each element is strictly greater than its preceding element.
**Example 1:**
**Input:** nums = \[4,9,6,10\]
**Output:** true
**Explanation:** In the first operation: Pick i = 0 and p = 3, and then subtract 3 from nums\[0\], so that nums becomes \[1,9,6,10\].
In the second operation: i = 1, p = 7, subtract 7 from nums\[1\], so nums becomes equal to \[1,2,6,10\].
After the second operation, nums is sorted in strictly increasing order, so the answer is true.
**Example 2:**
**Input:** nums = \[6,8,11,12\]
**Output:** true
**Explanation:** Initially nums is sorted in strictly increasing order, so we don't need to make any operations.
**Example 3:**
**Input:** nums = \[5,8,3\]
**Output:** false
**Explanation:** It can be proven that there is no way to perform operations to make nums sorted in strictly increasing order, so the answer is false.
**Constraints:**
* `1 <= nums.length <= 1000`
* `1 <= nums[i] <= 1000`
* `nums.length == n` | null |
Python Solution | Sieve of Erathosnes | Binary Search | Optimal | prime-subtraction-operation | 0 | 1 | Commented code for your convenience\n\n# Complexity\n- Time complexity: $$O(n * log(n))$$\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: $$O(n)$$ for the sieve itself, another $$O(n)$$ for the ```primes``` array\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```python []\nclass Solution:\n def getSubtraction(self, primes: List[int], val: int, prev: int):\n if len(primes) == 0:\n return -1\n left, right = 0, len(primes) - 1\n mid = (left + right) // 2\n while left <= right:\n mid = (left + right) // 2\n # If subtracting the current prime makes us less than the previous we\'ve gone too far, shift to the left.\n if val - primes[mid] <= prev:\n right = mid - 1\n # If subtracting the current prime is fine, then we try to shift to the right\n else:\n # if we are at the right side of the array there is nothing left to do\n if mid == len(primes) - 1:\n return mid\n # the prime to the right is always bigger than this one, so if the next one subtracts and this one can\'t then we have reached the biggest prime we can subtract\n elif val - primes[mid + 1] <= prev:\n return mid\n # shift to the right\n else:\n left = mid + 1\n return -1 if val - primes[mid] <= prev else mid\n \n def primeSubOperation(self, nums: List[int]) -> bool:\n sieveOfErathosnes, maximum = set(), max(nums)\n # Calculate all of the primes less than `max` using the sieve of eratosthenes\n sieveOfErathosnes.add(0); sieveOfErathosnes.add(1)\n for num in range(2, ceil(sqrt(maximum))):\n if num not in sieveOfErathosnes:\n for multiple in range(2 * num, maximum, num):\n sieveOfErathosnes.add(multiple)\n primes = [num for num in range(2, max(nums) + 1) if num not in sieveOfErathosnes]\n # For each value in nums, find the biggest number we can subtract such that `nums[i] > nums[i-1] \n # To find the biggest number possible we can use binary search\n for idx in range(len(nums)):\n prev = 0 if idx == 0 else nums[idx - 1]\n optimalPrimeIdx = self.getSubtraction(primes, nums[idx], prev)\n # Subtract the value from nums, if we can\'t do it and this value is smaller than the previous, then we can\'t do it.\n if optimalPrimeIdx == -1 and nums[idx] <= prev:\n return False\n elif optimalPrimeIdx != -1:\n nums[idx] -= primes[optimalPrimeIdx]\n return True\n``` | 0 | You are given a **0-indexed** integer array `nums` of length `n`.
You can perform the following operation as many times as you want:
* Pick an index `i` that you haven't picked before, and pick a prime `p` **strictly less than** `nums[i]`, then subtract `p` from `nums[i]`.
Return _true if you can make `nums` a strictly increasing array using the above operation and false otherwise._
A **strictly increasing array** is an array whose each element is strictly greater than its preceding element.
**Example 1:**
**Input:** nums = \[4,9,6,10\]
**Output:** true
**Explanation:** In the first operation: Pick i = 0 and p = 3, and then subtract 3 from nums\[0\], so that nums becomes \[1,9,6,10\].
In the second operation: i = 1, p = 7, subtract 7 from nums\[1\], so nums becomes equal to \[1,2,6,10\].
After the second operation, nums is sorted in strictly increasing order, so the answer is true.
**Example 2:**
**Input:** nums = \[6,8,11,12\]
**Output:** true
**Explanation:** Initially nums is sorted in strictly increasing order, so we don't need to make any operations.
**Example 3:**
**Input:** nums = \[5,8,3\]
**Output:** false
**Explanation:** It can be proven that there is no way to perform operations to make nums sorted in strictly increasing order, so the answer is false.
**Constraints:**
* `1 <= nums.length <= 1000`
* `1 <= nums[i] <= 1000`
* `nums.length == n` | null |
🐍Python Solution: Sort+PrefixSum+BinSearch | minimum-operations-to-make-all-array-elements-equal | 0 | 1 | \n\n# Code\n```\nclass Solution:\n def minOperations(self, nums: List[int], queries: List[int]) -> List[int]:\n nums.sort()\n prefix_sum = [nums[0]]\n ans = []\n for i in range(1, len(nums)):\n prefix_sum.append(prefix_sum[-1] + nums[i])\n \n for target in queries:\n l = 0\n r = len(nums) - 1\n pos = -2 # -2 for not found case\n #left bin search \n while l <= r:\n mid = (l + r) // 2\n if nums[mid] > target:\n r = mid - 1\n elif nums[mid] < target:\n l = mid + 1\n else:\n pos = mid\n r = mid - 1 \n\n if pos == -2:\n pos = min(l, r)\n # pos == -1 leftmost case\n if pos == -1:\n ans.append(prefix_sum[-1] - (len(nums))*target)\n else:\n ans.append((pos+1)*target - prefix_sum[pos] + prefix_sum[-1] - prefix_sum[pos] - (len(nums) -1 - pos)*target)\n \n return ans\n``` | 1 | You are given an array `nums` consisting of positive integers.
You are also given an integer array `queries` of size `m`. For the `ith` query, you want to make all of the elements of `nums` equal to `queries[i]`. You can perform the following operation on the array **any** number of times:
* **Increase** or **decrease** an element of the array by `1`.
Return _an array_ `answer` _of size_ `m` _where_ `answer[i]` _is the **minimum** number of operations to make all elements of_ `nums` _equal to_ `queries[i]`.
**Note** that after each query the array is reset to its original state.
**Example 1:**
**Input:** nums = \[3,1,6,8\], queries = \[1,5\]
**Output:** \[14,10\]
**Explanation:** For the first query we can do the following operations:
- Decrease nums\[0\] 2 times, so that nums = \[1,1,6,8\].
- Decrease nums\[2\] 5 times, so that nums = \[1,1,1,8\].
- Decrease nums\[3\] 7 times, so that nums = \[1,1,1,1\].
So the total number of operations for the first query is 2 + 5 + 7 = 14.
For the second query we can do the following operations:
- Increase nums\[0\] 2 times, so that nums = \[5,1,6,8\].
- Increase nums\[1\] 4 times, so that nums = \[5,5,6,8\].
- Decrease nums\[2\] 1 time, so that nums = \[5,5,5,8\].
- Decrease nums\[3\] 3 times, so that nums = \[5,5,5,5\].
So the total number of operations for the second query is 2 + 4 + 1 + 3 = 10.
**Example 2:**
**Input:** nums = \[2,9,6,3\], queries = \[10\]
**Output:** \[20\]
**Explanation:** We can increase each value in the array to 10. The total number of operations will be 8 + 1 + 4 + 7 = 20.
**Constraints:**
* `n == nums.length`
* `m == queries.length`
* `1 <= n, m <= 105`
* `1 <= nums[i], queries[i] <= 109` | null |
python3 Solution | minimum-operations-to-make-all-array-elements-equal | 0 | 1 | \n```\nclass Solution:\n def minOperations(self, nums: List[int], queries: List[int]) -> List[int]:\n nums.sort()\n n=len(nums)\n prefix=[0]+list(accumulate(nums))\n ans=[]\n for x in queries:\n i=bisect_left(nums,x)\n ans.append(x*(2*i-n)+prefix[n]-2*prefix[i])\n\n return ans \n``` | 1 | You are given an array `nums` consisting of positive integers.
You are also given an integer array `queries` of size `m`. For the `ith` query, you want to make all of the elements of `nums` equal to `queries[i]`. You can perform the following operation on the array **any** number of times:
* **Increase** or **decrease** an element of the array by `1`.
Return _an array_ `answer` _of size_ `m` _where_ `answer[i]` _is the **minimum** number of operations to make all elements of_ `nums` _equal to_ `queries[i]`.
**Note** that after each query the array is reset to its original state.
**Example 1:**
**Input:** nums = \[3,1,6,8\], queries = \[1,5\]
**Output:** \[14,10\]
**Explanation:** For the first query we can do the following operations:
- Decrease nums\[0\] 2 times, so that nums = \[1,1,6,8\].
- Decrease nums\[2\] 5 times, so that nums = \[1,1,1,8\].
- Decrease nums\[3\] 7 times, so that nums = \[1,1,1,1\].
So the total number of operations for the first query is 2 + 5 + 7 = 14.
For the second query we can do the following operations:
- Increase nums\[0\] 2 times, so that nums = \[5,1,6,8\].
- Increase nums\[1\] 4 times, so that nums = \[5,5,6,8\].
- Decrease nums\[2\] 1 time, so that nums = \[5,5,5,8\].
- Decrease nums\[3\] 3 times, so that nums = \[5,5,5,5\].
So the total number of operations for the second query is 2 + 4 + 1 + 3 = 10.
**Example 2:**
**Input:** nums = \[2,9,6,3\], queries = \[10\]
**Output:** \[20\]
**Explanation:** We can increase each value in the array to 10. The total number of operations will be 8 + 1 + 4 + 7 = 20.
**Constraints:**
* `n == nums.length`
* `m == queries.length`
* `1 <= n, m <= 105`
* `1 <= nums[i], queries[i] <= 109` | null |
[Java/Python 3] Sort, compute prefix sum then binary search. | minimum-operations-to-make-all-array-elements-equal | 1 | 1 | Within each query, for each number in `nums`, if it is less than `q`, we need to deduct it from `q` and get the number of the increment operations; if it is NO less than `q`, we need to deduct `q` from the number to get the number of the decrement operations.\n\nTherfore, we can sort `nums`, then use prefix sum to compute the partial sum of the sorted `nums`. Use binary search to find the index of the `nums`, `idx`, to separate the less than part and NO less than part. With these information we can compute the total operations needed for a specific query `q`.\n\n**Note:**\n`nums[0 ... idx - 1] <= q, nums[idx ... n - 1] > q`\n`prefixSum[idx] = sum(nums[0 ... idx - 1])`\n`prefixSum[n] - prefixSum[idx] = sum(nums[idx ... n - 1])`\n`idx * q - prefixSum[idx]` : # of increment operations needed for `nums[0 ... idx - 1]`\n`prefixSum[n] - prefixSum[idx] - (n - idx) * q`: # of decrement operations needed for `nums[idx ... n - 1]`\n```java\n public List<Long> minOperations(int[] nums, int[] queries) {\n Arrays.sort(nums);\n int n = nums.length;\n long[] prefixSum = new long[n + 1];\n for (int i = 0; i < n; ++i) {\n prefixSum[i + 1] = prefixSum[i] + nums[i];\n }\n List<Long> ans = new ArrayList<>();\n for (int q : queries) {\n int idx = binarySearch(nums, q); // There are idx numbers in nums less than q.\n ans.add(1L * q * idx - prefixSum[idx] + prefixSum[n] - prefixSum[idx] - 1L * (n - idx) * q);\n }\n return ans;\n }\n private int binarySearch(int[] nums, int key) {\n int lo = 0, hi = nums.length;\n while (lo < hi) {\n int mid = lo + (hi - lo) / 2;\n if (key > nums[mid]) {\n lo = mid + 1;\n }else {\n hi = mid;\n }\n }\n return lo;\n }\n```\n```python\n def minOperations(self, nums: List[int], queries: List[int]) -> List[int]:\n nums.sort()\n prefix_sum, n = [0], len(nums)\n for num in nums:\n prefix_sum.append(num + prefix_sum[-1])\n ans = []\n for q in queries:\n idx = bisect.bisect(nums, q) # There are idx numbers in nums less than q.\n ans.append(q * idx - prefix_sum[idx] + prefix_sum[-1] - prefix_sum[idx] - q * (n - idx)) \n return ans\n```\n\n**Analysis:**\n\nLet `n = nums.length, m = queries.length`:\nSort cost time `O(nlogn)`, each query cost time `O(logn)` and all quries cost `O(mlogn)`; `prefixSum` and sorting cost space `O(n)`, therefore\n\nTime: `O((m + n) * logn)`, space: `O(n)` (`O(m + n)` if including return space). | 12 | You are given an array `nums` consisting of positive integers.
You are also given an integer array `queries` of size `m`. For the `ith` query, you want to make all of the elements of `nums` equal to `queries[i]`. You can perform the following operation on the array **any** number of times:
* **Increase** or **decrease** an element of the array by `1`.
Return _an array_ `answer` _of size_ `m` _where_ `answer[i]` _is the **minimum** number of operations to make all elements of_ `nums` _equal to_ `queries[i]`.
**Note** that after each query the array is reset to its original state.
**Example 1:**
**Input:** nums = \[3,1,6,8\], queries = \[1,5\]
**Output:** \[14,10\]
**Explanation:** For the first query we can do the following operations:
- Decrease nums\[0\] 2 times, so that nums = \[1,1,6,8\].
- Decrease nums\[2\] 5 times, so that nums = \[1,1,1,8\].
- Decrease nums\[3\] 7 times, so that nums = \[1,1,1,1\].
So the total number of operations for the first query is 2 + 5 + 7 = 14.
For the second query we can do the following operations:
- Increase nums\[0\] 2 times, so that nums = \[5,1,6,8\].
- Increase nums\[1\] 4 times, so that nums = \[5,5,6,8\].
- Decrease nums\[2\] 1 time, so that nums = \[5,5,5,8\].
- Decrease nums\[3\] 3 times, so that nums = \[5,5,5,5\].
So the total number of operations for the second query is 2 + 4 + 1 + 3 = 10.
**Example 2:**
**Input:** nums = \[2,9,6,3\], queries = \[10\]
**Output:** \[20\]
**Explanation:** We can increase each value in the array to 10. The total number of operations will be 8 + 1 + 4 + 7 = 20.
**Constraints:**
* `n == nums.length`
* `m == queries.length`
* `1 <= n, m <= 105`
* `1 <= nums[i], queries[i] <= 109` | null |
[C++, Java, Python3] Prefix Sums + Binary Search | minimum-operations-to-make-all-array-elements-equal | 1 | 1 | # Intuition\n* If there are `j` numbers in `nums` that are smaller than `query[i]`, you need to find `query[i] * j - sum(j numbers smaller than query[i])` to find increments required in `nums`\n* If there are `k` numbers in `nums` that are greater than `query[i]`, you need to find `sum(k numbers larger than query[i]) - query[i] * k` to find decrements required in `nums`\n* Sum of above two values is `ans[i]`\n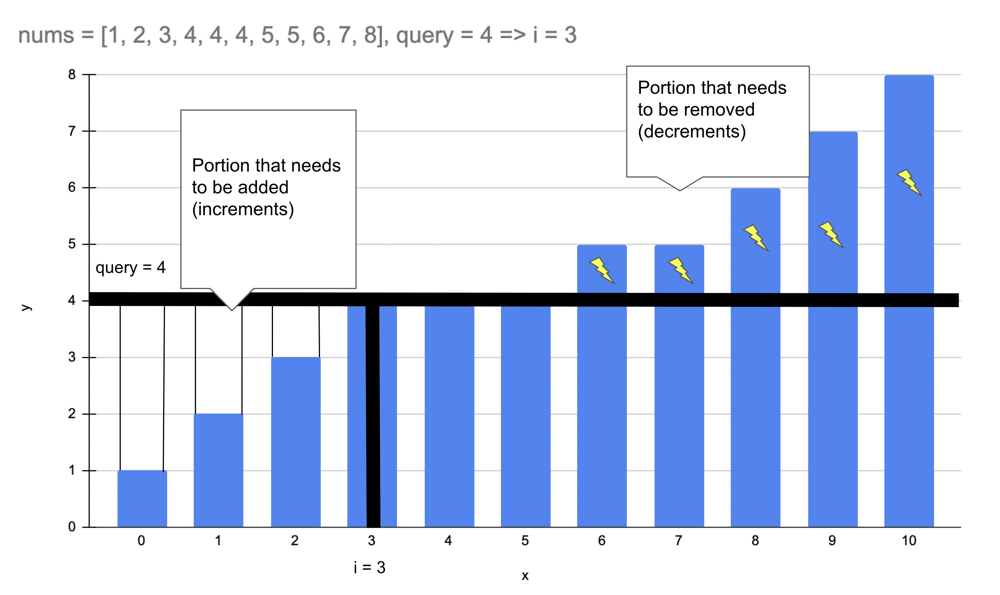\n\n\n# Approach\n* To find smaller numbers than `query[i]` we can sort the array and use binary search\n* Binary search over sorted `nums` to find index of `query[i]`\n* Then use prefix sums to find sum of number in smaller and larger segments\n* `prefix[n] - prefix[i]` is sum of numbers greater than or equal to `query[i]`\n* `prefix[i]` is sum of numbers smaller than `query[i]`\n* `query[i] * i - prefix[i]` is increments required\n* `prefix[n] - prefix[i] - query[i] * (n - i)` is decrements required\n* Total = `query[i] * i - prefix[i] + prefix[n] - prefix[i] - query[i] * (n - i)`\n* Can be simplified to `query[i] * (2 * i - n) + prefix[n] - 2 * prefix[i]`\n\n# Complexity\n- Time complexity: `O((n + m) * log(n))`\n\n- Space complexity: `O(n)`\n\n# Code\n**Python3**:\n```\ndef minOperations(self, nums: List[int], queries: List[int]) -> List[int]:\n nums.sort()\n ans, n, prefix = [], len(nums), [0] + list(accumulate(nums))\n for x in queries:\n i = bisect_left(nums, x)\n ans.append(x * (2 * i - n) + prefix[n] - 2 * prefix[i])\n return ans\n```\n```\ndef minOperations(self, nums: List[int], queries: List[int]) -> List[int]:\n nums.sort()\n prefix = [0] + list(accumulate(nums))\n for x in queries:\n i = bisect_left(nums, x)\n yield x * (2 * i - len(nums)) + prefix[-1] - 2 * prefix[i]\n```\n**C++**:\n```\nvector<long long> minOperations(vector<int>& nums, vector<int>& queries) {\n sort(nums.begin(), nums.end());\n int n = nums.size();\n vector<long long> ans, prefix(n + 1);\n for (int i = 0; i < n; i++)\n prefix[i + 1] = prefix[i] + (long long) nums[i];\n for (int& x : queries) {\n int i = lower_bound(nums.begin(), nums.end(), x) - nums.begin();\n ans.push_back(1LL * x * (2 * i - n) + prefix[n] - 2 * prefix[i]);\n }\n return ans;\n}\n```\n\n**Java**:\n```\npublic List<Long> minOperations(int[] nums, int[] queries) {\n Arrays.sort(nums);\n List<Long> ans = new ArrayList<>();\n int n = nums.length;\n long[] prefix = new long[n + 1];\n for (int i = 1; i <= n; i++)\n prefix[i] = prefix[i - 1] + nums[i - 1];\n for (int x: queries) {\n int i = bisect_left(nums, x);\n ans.add(1L * x * (2 * i - n) + prefix[n] - 2 * prefix[i]);\n }\n return ans;\n}\nprivate int bisect_left(int[] nums, int x) {\n int lo = 0, hi = nums.length;\n while (lo < hi) {\n int mid = lo + (hi - lo) / 2;\n if (nums[mid] < x) lo = mid + 1;\n else hi = mid;\n }\n return lo;\n}\n```\n\n**Java using Arrays.binarySearch**:\n```\npublic List<Long> minOperations(int[] nums, int[] queries) {\n Arrays.sort(nums);\n List<Long> ans = new ArrayList<>();\n int n = nums.length;\n long[] prefix = new long[n + 1];\n for (int i = 1; i <= n; i++)\n prefix[i] = prefix[i - 1] + nums[i - 1];\n for (int x: queries) {\n int i = Arrays.binarySearch(nums, x);\n if (i < 0) i = -(i + 1); // convert negative index to insertion point\n ans.add(1L * x * (2 * i - n) + prefix[n] - 2 * prefix[i]);\n }\n return ans;\n}\n```\n*Good to know*: In Java, Arrays.binarySearch returns a negative index when the target element is not found in the array. The negative index returned is equal to -(insertion point) - 1, where the insertion point is the index at which the target element would be inserted into the array to maintain the sorted order.\n\nFor example, suppose we have an array arr = {1, 3, 5, 7, 9} and we want to search for the element 4. The Arrays.binarySearch(arr, 4) method would return -3. To convert this to the insertion point, we take the absolute value of the negative index and subtract 1: insertion point = |-(-3)| - 1 = 2.\n\nTherefore, the insertion point for the element 4 in the sorted array arr would be at index 2, which is the position where we would need to insert 4 to maintain the sorted order.\n\n**More binary search problems:**\n[Minimum Time to Repair Cars](https://leetcode.com/problems/minimum-time-to-repair-cars/solutions/3311975/c-java-python3-binary-search-1-liner/)\n[Minimum Time to Complete Trips](https://leetcode.com/problems/minimum-time-to-complete-trips/solutions/1802415/python3-java-c-binary-search-1-liner/)\n[Kth Missing Positive Number](https://leetcode.com/problems/kth-missing-positive-number/solutions/3262163/c-java-python3-1-line-ologn/)\n[Maximum Tastiness of Candy Basket](https://leetcode.com/problems/maximum-tastiness-of-candy-basket/solutions/2947983/c-java-python-binary-search-and-sorting/)\n[Number of Flowers in Full Bloom](https://leetcode.com/problems/number-of-flowers-in-full-bloom/solutions/1976804/python3-2-lines-sort-and-binary-search/) | 84 | You are given an array `nums` consisting of positive integers.
You are also given an integer array `queries` of size `m`. For the `ith` query, you want to make all of the elements of `nums` equal to `queries[i]`. You can perform the following operation on the array **any** number of times:
* **Increase** or **decrease** an element of the array by `1`.
Return _an array_ `answer` _of size_ `m` _where_ `answer[i]` _is the **minimum** number of operations to make all elements of_ `nums` _equal to_ `queries[i]`.
**Note** that after each query the array is reset to its original state.
**Example 1:**
**Input:** nums = \[3,1,6,8\], queries = \[1,5\]
**Output:** \[14,10\]
**Explanation:** For the first query we can do the following operations:
- Decrease nums\[0\] 2 times, so that nums = \[1,1,6,8\].
- Decrease nums\[2\] 5 times, so that nums = \[1,1,1,8\].
- Decrease nums\[3\] 7 times, so that nums = \[1,1,1,1\].
So the total number of operations for the first query is 2 + 5 + 7 = 14.
For the second query we can do the following operations:
- Increase nums\[0\] 2 times, so that nums = \[5,1,6,8\].
- Increase nums\[1\] 4 times, so that nums = \[5,5,6,8\].
- Decrease nums\[2\] 1 time, so that nums = \[5,5,5,8\].
- Decrease nums\[3\] 3 times, so that nums = \[5,5,5,5\].
So the total number of operations for the second query is 2 + 4 + 1 + 3 = 10.
**Example 2:**
**Input:** nums = \[2,9,6,3\], queries = \[10\]
**Output:** \[20\]
**Explanation:** We can increase each value in the array to 10. The total number of operations will be 8 + 1 + 4 + 7 = 20.
**Constraints:**
* `n == nums.length`
* `m == queries.length`
* `1 <= n, m <= 105`
* `1 <= nums[i], queries[i] <= 109` | null |
Prefix Sum | minimum-operations-to-make-all-array-elements-equal | 0 | 1 | To make all elements equal, we need to increase elements smaller than query `q`, and decrease larger ones.\n \nWe sort our array first, so we can find index `i` of first element larger than `q`.\n \nThen, we use the prefix sum array to get:\n- sum of smaller elements `ps[i]`.\n- sum of larger elements `ps[-1] - ps[i]`.\n \nThe number of operations is:\n- `q * i - ps[i]` for smaller elements.\n- `ps[-1] - ps[i] - q * (n - i)` for larger elements.\n \n**Python 3**\n```python\nclass Solution:\n def minOperations(self, nums: List[int], queries: List[int]) -> List[int]:\n nums.sort()\n ps, n = [0] + list(accumulate(nums)), len(nums)\n splits = [(q, bisect_left(nums, q)) for q in queries]\n return [q * i - ps[i] + (ps[-1] - ps[i]) - q * (n - i) for q, i in splits] \n```\n**C++**\n```cpp\nvector<long long> minOperations(vector<int>& nums, vector<int>& queries) {\n vector<long long> ps{0}, res;\n sort(begin(nums), end(nums));\n for (int n : nums)\n ps.push_back(ps.back() + n);\n for (long long q : queries) {\n int i = upper_bound(begin(nums), end(nums), q) - begin(nums);\n res.push_back(q * i - ps[i] + (ps.back() - ps[i]) - q * (nums.size() - i));\n }\n return res;\n}\n``` | 22 | You are given an array `nums` consisting of positive integers.
You are also given an integer array `queries` of size `m`. For the `ith` query, you want to make all of the elements of `nums` equal to `queries[i]`. You can perform the following operation on the array **any** number of times:
* **Increase** or **decrease** an element of the array by `1`.
Return _an array_ `answer` _of size_ `m` _where_ `answer[i]` _is the **minimum** number of operations to make all elements of_ `nums` _equal to_ `queries[i]`.
**Note** that after each query the array is reset to its original state.
**Example 1:**
**Input:** nums = \[3,1,6,8\], queries = \[1,5\]
**Output:** \[14,10\]
**Explanation:** For the first query we can do the following operations:
- Decrease nums\[0\] 2 times, so that nums = \[1,1,6,8\].
- Decrease nums\[2\] 5 times, so that nums = \[1,1,1,8\].
- Decrease nums\[3\] 7 times, so that nums = \[1,1,1,1\].
So the total number of operations for the first query is 2 + 5 + 7 = 14.
For the second query we can do the following operations:
- Increase nums\[0\] 2 times, so that nums = \[5,1,6,8\].
- Increase nums\[1\] 4 times, so that nums = \[5,5,6,8\].
- Decrease nums\[2\] 1 time, so that nums = \[5,5,5,8\].
- Decrease nums\[3\] 3 times, so that nums = \[5,5,5,5\].
So the total number of operations for the second query is 2 + 4 + 1 + 3 = 10.
**Example 2:**
**Input:** nums = \[2,9,6,3\], queries = \[10\]
**Output:** \[20\]
**Explanation:** We can increase each value in the array to 10. The total number of operations will be 8 + 1 + 4 + 7 = 20.
**Constraints:**
* `n == nums.length`
* `m == queries.length`
* `1 <= n, m <= 105`
* `1 <= nums[i], queries[i] <= 109` | null |
✔💯DAY 361 || 100% || 0MS || EXPLAINED || DRY RUN || MEME | minimum-operations-to-make-all-array-elements-equal | 1 | 1 | # Please Upvote as it really motivates me\n# Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n##### \u2022\tThe intuition behind this code is to first sort the input array of numbers and the array of queries. Then, for each query, we calculate the prefix sum of all numbers in the input array that are less than the current query. We use this prefix sum to calculate the number of operations required to make all numbers in the input array equal to the query.\n##### \u2022\tTo do this, we first calculate the total sum of all numbers in the input array. Then, we subtract twice the prefix sum from this total sum, since we need to add q to all numbers less than q and subtract q from all numbers greater than q. The factor of 2 is because we need to perform both additions and subtractions. Finally, we add q times the number of elements less than q that we have already processed, since we have already added q to these elements.\n##### \u2022\tThis approach allows us to efficiently calculate the answer for each query in O(n log n) time, where n is the length of the input array.\n\n\n\n# Explain\n<!-- Describe your approach to solving the problem. -->\n\n##### \u2022\tThe code is implementing the following approach:\n\n##### \u2022\tSort the input array of numbers in ascending order using the sort() function from the STL library.\n##### \u2022\tsort(nums.begin(), nums.end());\n##### \u2022\tCreate a vector of pairs called aug to store the queries and their respective indices. For each query in the input array queries, create a pair with the query value and its index and add it to the aug vector.\n##### \u2022\tvector<pair<int, int>> aug;\n##### \u2022\tfor (int i = 0; i < queries.size(); ++i) {\n##### \u2022\taug.emplace_back(queries[i], i);\n##### \u2022\t}\n##### \u2022\tSort the aug vector of pairs in increasing order of query value.\n##### \u2022\tsort(aug.begin(), aug.end());\n##### \u2022\tCreate a new vector called ans to store the answers for each query. The size of ans is the same as the size of queries.\n##### \u2022\tvector<long long> ans(queries.size());\n##### \u2022\tCalculate the total sum of all numbers in the input array nums using the accumulate() function from the STL library.\n##### \u2022\tlong long total = accumulate(nums.begin(), nums.end(), 0ll);\n##### \u2022\tInitialize a variable called prefix to 0. This variable will be used to calculate the prefix sum of all numbers in nums that are less than the current query.\n##### \u2022\tlong long prefix = 0;\n##### \u2022\tInitialize a variable called k to 0. This variable will be used to keep track of the number of elements in nums that are less than the current query.\n##### \u2022\tint k = 0;\n##### \u2022\tLoop through each pair in the aug vector. For each pair, calculate the number of operations required to make all numbers in nums equal to the current query.\n##### \u2022\tfor (auto& [q, i] : aug) {\n##### \u2022\t// Calculate prefix sum of all numbers less than q\n##### \u2022\twhile (k < nums.size() && nums[k] < q)\no\tprefix += nums[k++];\n##### \u2022\t// Calculate answer for current query\n##### \u2022\tans[i] = total - 2*prefix + (long long) q*(2*k - nums.size());\n##### \u2022\t}\n##### \u2022\tReturn the ans vector, which contains the answers for all queries.\n##### \u2022\treturn ans;\n##### \u2022\tOverall, the approach involves sorting the input array and the queries, and then iterating through the queries and calculating the prefix sum of all numbers less than the current query. This prefix sum is then used to calculate the number of operations required to make all numbers in the input array equal to the current query.\n\n\n\n\n# Code\n```c++ []\nvector<long long> minOperations(vector<int>& nums, vector<int>& queries) {\n sort(nums.begin(), nums.end());\n vector<pair<int, int>> aug;\n for (int i = 0; i < queries.size(); ++i) {\n aug.emplace_back(queries[i], i);\n }\n sort(aug.begin(), aug.end());\n vector<long long> ans(queries.size());\n long long total = accumulate(nums.begin(), nums.end(), 0ll);\n long long prefix = 0;\n int k = 0;\n for (auto& [q, i] : aug) {\n while (k < nums.size() && nums[k] < q) {\n prefix += nums[k];\n k++;\n }\n ans[i] = total - 2 * prefix + (long long) q * (2 * k - nums.size());\n }\n return ans;\n}\n```\n```python []\ndef minOperations(self,nums: List[int], queries: List[int]) -> List[int]:\n nums.sort()\n aug = [(q, i) for i, q in enumerate(queries)]\n aug.sort()\n total = sum(nums)\n prefix = 0\n k = 0\n ans = [0] * len(queries)\n for q, i in aug:\n while k < len(nums) and nums[k] < q:\n prefix += nums[k]\n k += 1\n ans[i] = total - 2 * prefix + q * (2 * k - len(nums))\n return ans\n```\n```java []\npublic List<Long> minOperations(int[] nums, int[] queries) {\n int n = nums.length,m = queries.length;\n \n Arrays.sort(nums);\n \n long[] psum = new long[n + 1];\n for(int i = 0;i < n;i++) psum[i + 1] = psum[i] + nums[i];\n \n List<Long> ans = new ArrayList<>();\n \n for(int i = 0;i < m;i++){\n \n int si = 0,ei = n - 1,idx = 0;\n while(si <= ei){\n int mid = si + (ei - si) / 2;\n \n if(nums[mid] <= queries[i]){\n idx = mid + 1;\n si = mid + 1;\n }else{\n idx = mid;\n ei = mid - 1;\n }\n }\n \n long left = (1L * queries[i] * idx) - psum[si]; \n long right = (psum[n] - psum[idx]) - (1L * queries[i] * (n - idx));\n \n ans.add(left + right);\n }\n return ans;\n }\n# explaination\n\n\n##### \u2022\tThe minOperations function takes two integer arrays as input, nums and queries, and returns a list of long integers as output. For each query in the queries array, the function computes the minimum number of operations required to make all the elements in the nums array greater than or equal to the query value.\n\n##### \u2022\tFirst, the function initializes two variables, n and m, to the lengths of the nums and queries arrays respectively. It then sorts the nums array in ascending order using Arrays.sort().\n\n##### \u2022\tNext, the function initializes a new array called psum of length n + 1. It then populates the psum array with prefix sums of the nums array using a for loop. Specifically, it sets psum[0] to 0, and for each index i from 0 to n - 1, it sets psum[i + 1] to psum[i] + nums[i].\n\n##### \u2022\tThe function then initializes an empty list called ans to store the results of each query.\n\n##### \u2022\tFor each query in the queries array, the function performs a binary search on the nums array to find the index of the first element that is greater than the query value. It initializes three variables, si, ei, and idx, to 0, n - 1, and 0 respectively. It then enters a while loop that continues as long as si is less than or equal to ei. In each iteration of the loop, it calculates the midpoint of the current search range using (si + ei) / 2. If the midpoint element is less than or equal to the query value, it updates idx to the midpoint index plus 1, and sets si to the midpoint index plus 1. Otherwise, it updates idx to the midpoint index, and sets ei to the midpoint index minus 1. Once the loop exits, idx will contain the index\n\n\n\n```\nHERE https://leetcode.com/problems/minimum-operations-to-make-all-array-elements-equal/solutions/3347750/100-0ms-explained-dry-run-meme/\n\n# DRY RUN 1\nFirst, we sort the nums vector in ascending order: nums = [1, 3, 6, 8]. Next, we create a new vector called aug, which will store pairs of each query and its index in the queries vector. In this case, aug = [(1, 0), (5, 1)]. We sort the aug vector in ascending order based on the query value: aug = [(1, 0), (5, 1)]. We create a new vector called ans to store the results of each query. We calculate the total sum of the nums vector using the accumulate function: total = 18. We initialize the prefix variable to 0. We set k to 0. We loop through each pair in the aug vector. For the first pair, q = 1 and i = 0. For the second pair, q = 5 and i = 1. For each pair, we loop through the nums vector while k < nums.size() and nums[k] < q. In this case, k starts at 0 and nums[k] = 1, which is less than q = 1, so we do not enter the loop. For the second pair, k is still 0 and nums[k] = 1, which is less than q = 5, so we enter the loop. We add nums[k] = 1 to prefix, increment k to 1, and check if nums[k] < q. Since nums[k] = 3 is less than q = 5, we add nums[k] = 3 to prefix, increment k to 2, and check again. Since nums[k] = 6 is not less than q = 5, we exit the loop. For the first pair, we calculate ans[i] = total - 2prefix + (long long) q(2k - nums.size()) = 18 - 20 + (long long) 1*(20 - 4) = 14. For the second pair, we calculate ans[i] = total - 2prefix + (long long) q*(2k - nums.size()) = 18 - 24 + (long long) 5*(2*2 - 4) = 10. We return the ans vector: [14, 10].\n\n# dry run 2\n\nFirst, we sort the nums vector in ascending order: nums = [2, 3, 6, 9]. Next, we create a new vector called aug, which will store pairs of each query and its index in the queries vector. In this case, aug = [(10, 0)]. We sort the aug vector in ascending order based on the query value: aug = [(10, 0)]. We create a new vector called ans to store the results of each query. We calculate the total sum of the nums vector using the accumulate function: total = 20. We initialize the prefix variable to 0. We set k to 0. We loop through each pair in the aug vector. For the only pair, q = 10 and i = 0. For the pair, we loop through the nums vector while k < nums.size() and nums[k] < q. In this case, k starts at 0 and nums[k] = 2, which is less than q = 10, so we enter the loop. We add nums[k] = 2 to prefix, increment k to 1, and check if nums[k] < q. Since nums[k] = 3 is less than q = 10, we add nums[k] = 3 to prefix, increment k to 2, and check again. Since nums[k] = 6 is less than q = 10, we add nums[k] = 6 to prefix, increment k to 3, and check again. Since nums[k] = 9 is not less than q = 10, we exit the loop. For the pair, we calculate ans[i] = total - 2 * prefix + (long long) q * (2 * k - nums.size()) = 20 - 2 * (2 + 3 + 6) + (long long) 10 * (2 * 4 - 4) = 20. We return the ans vector: [20].\n\n# Complexity\n# Time complexity: \nO(nlogn + qlogq), where n is the length of the nums vector and q is the length of the queries vector. The sort function has a time complexity of O(nlogn) for sorting the nums vector and O(qlogq) for sorting the aug vector. The while loop inside the for loop has a time complexity of O(n) in the worst case, but since k is incremented at each iteration, the total time complexity of the while loop is O(n) for all iterations of the for loop. Therefore, the overall time complexity of the code is dominated by the sort function, which has a time complexity of O(nlogn + qlogq).\n\n# Space complexity: \nO(q), where q is the length of the queries vector. The aug vector has a space complexity of O(q) and the ans vector has a space complexity of O(q), so the total space complexity of the code is O(q).\n\n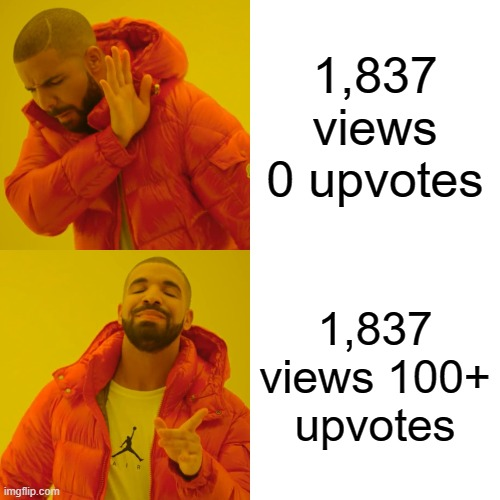\n\n | 5 | You are given an array `nums` consisting of positive integers.
You are also given an integer array `queries` of size `m`. For the `ith` query, you want to make all of the elements of `nums` equal to `queries[i]`. You can perform the following operation on the array **any** number of times:
* **Increase** or **decrease** an element of the array by `1`.
Return _an array_ `answer` _of size_ `m` _where_ `answer[i]` _is the **minimum** number of operations to make all elements of_ `nums` _equal to_ `queries[i]`.
**Note** that after each query the array is reset to its original state.
**Example 1:**
**Input:** nums = \[3,1,6,8\], queries = \[1,5\]
**Output:** \[14,10\]
**Explanation:** For the first query we can do the following operations:
- Decrease nums\[0\] 2 times, so that nums = \[1,1,6,8\].
- Decrease nums\[2\] 5 times, so that nums = \[1,1,1,8\].
- Decrease nums\[3\] 7 times, so that nums = \[1,1,1,1\].
So the total number of operations for the first query is 2 + 5 + 7 = 14.
For the second query we can do the following operations:
- Increase nums\[0\] 2 times, so that nums = \[5,1,6,8\].
- Increase nums\[1\] 4 times, so that nums = \[5,5,6,8\].
- Decrease nums\[2\] 1 time, so that nums = \[5,5,5,8\].
- Decrease nums\[3\] 3 times, so that nums = \[5,5,5,5\].
So the total number of operations for the second query is 2 + 4 + 1 + 3 = 10.
**Example 2:**
**Input:** nums = \[2,9,6,3\], queries = \[10\]
**Output:** \[20\]
**Explanation:** We can increase each value in the array to 10. The total number of operations will be 8 + 1 + 4 + 7 = 20.
**Constraints:**
* `n == nums.length`
* `m == queries.length`
* `1 <= n, m <= 105`
* `1 <= nums[i], queries[i] <= 109` | null |
Easy solution in python-3 | minimum-operations-to-make-all-array-elements-equal | 0 | 1 | # Intuition\nIt can be solved using naive approach in O(n^2) time complexity, which includes one outer for loop for iterating in queries list and the inner outer loop for calculating the sum (as shown) in \'nums\' list. Since, this solution is not optimal, we have to reduce the time complexity further.\n# Naive approach:\n def minOperations(self, nums: List[int], queries: List[int]) -> List[int]:\n ans=[]\n for i in range(len(queries)):\n summ=0\n for j in range(0,len(nums)):\n summ+=abs(nums[j]-queries[i])\n ans.append(summ)\n return(ans)\n\n# Approach\nTo reduce the T.C, the other possible methods for getting the summ varibale should be either O(log(n)) or O(1). Since we know that, if we make a prefix sum array, we can retrieve sum in O(1) only, we have used that here.\nThe reason for using binary search here is: If we can get the index of a number (say i), we can get the sum of elements upto the \'i\'th index using prefix_sum array(that too in O(1) time).\n# Complexity\n- Time complexity:\nO(nlog(n))\n\n- Space complexity:\nO(n) for the extra array that i took (arr)\n\n# Code\n```\nclass Solution:\n def minOperations(self, nums: List[int], queries: List[int]) -> List[int]:\n n=len(nums)\n nums.sort()\n arr=nums.copy()\n for i in range(1,len(nums)): #Prefix_sum\n nums[i]+=nums[i-1]\n \n\n def binary_search(nums,x):\n start=0\n end=len(nums)-1 \n while(start<=end):\n mid=(start+end)//2\n if(nums[mid]==x):\n return(mid)\n elif(nums[mid]>x):\n end=mid-1\n else:\n start=mid+1 \n return(start)\n \n\n ans=[]\n for i in range(0,len(queries)):\n summ=0\n index=binary_search(arr,queries[i]) #to get the index\n if(index==0):\n summ=nums[-1]-(n*queries[i])\n else:\n summ=(index*queries[i])-(nums[index-1]) #for summ of elements before index\n summ+=(nums[-1]-nums[index-1])-((n-index)*queries[i]) #for summ of elements after index\n ans.append(summ)\n return(ans)\n``` | 2 | You are given an array `nums` consisting of positive integers.
You are also given an integer array `queries` of size `m`. For the `ith` query, you want to make all of the elements of `nums` equal to `queries[i]`. You can perform the following operation on the array **any** number of times:
* **Increase** or **decrease** an element of the array by `1`.
Return _an array_ `answer` _of size_ `m` _where_ `answer[i]` _is the **minimum** number of operations to make all elements of_ `nums` _equal to_ `queries[i]`.
**Note** that after each query the array is reset to its original state.
**Example 1:**
**Input:** nums = \[3,1,6,8\], queries = \[1,5\]
**Output:** \[14,10\]
**Explanation:** For the first query we can do the following operations:
- Decrease nums\[0\] 2 times, so that nums = \[1,1,6,8\].
- Decrease nums\[2\] 5 times, so that nums = \[1,1,1,8\].
- Decrease nums\[3\] 7 times, so that nums = \[1,1,1,1\].
So the total number of operations for the first query is 2 + 5 + 7 = 14.
For the second query we can do the following operations:
- Increase nums\[0\] 2 times, so that nums = \[5,1,6,8\].
- Increase nums\[1\] 4 times, so that nums = \[5,5,6,8\].
- Decrease nums\[2\] 1 time, so that nums = \[5,5,5,8\].
- Decrease nums\[3\] 3 times, so that nums = \[5,5,5,5\].
So the total number of operations for the second query is 2 + 4 + 1 + 3 = 10.
**Example 2:**
**Input:** nums = \[2,9,6,3\], queries = \[10\]
**Output:** \[20\]
**Explanation:** We can increase each value in the array to 10. The total number of operations will be 8 + 1 + 4 + 7 = 20.
**Constraints:**
* `n == nums.length`
* `m == queries.length`
* `1 <= n, m <= 105`
* `1 <= nums[i], queries[i] <= 109` | null |
NLogN | Easy Solution | minimum-operations-to-make-all-array-elements-equal | 0 | 1 | For each query target in queries, the solution performs binary search on the sorted array nums to find the index i such that nums[i] is the smallest element greater than or equal to the target. It then calculates the sum of the following two parts:\n\nThe total number of operations required to make the first i elements of nums equal to target. This is calculated as i * target - prefix[i-1] where prefix[i-1] represents the sum of the first i-1 elements of nums.\n\nThe total number of operations required to make the last n-i elements of nums equal to target. This is calculated as (prefix[n-1] - prefix[i-1]) - (n - i) * target where prefix[n-1] - prefix[i-1] represents the sum of the last n-i elements of nums.\n \n\n# Code\n```\nclass Solution:\n def minOperations(self, nums: List[int], queries: List[int]) -> List[int]:\n prefix = []\n nums.sort()\n prev = 0\n for num in nums:\n prev += num\n prefix.append(prev)\n \n data = []\n for target in queries:\n i = 0\n k = len(prefix)\n tot = 0\n while i < k:\n j = (i + k) // 2\n if nums[j] > target: k = j\n else: i = j + 1\n \n backs = prefix[i - 1] if i != 0 else 0\n tot += i * target - backs\n tot += (prefix[-1] - backs) - (len(nums) - i) * target\n data.append(tot)\n \n return data\n``` | 1 | You are given an array `nums` consisting of positive integers.
You are also given an integer array `queries` of size `m`. For the `ith` query, you want to make all of the elements of `nums` equal to `queries[i]`. You can perform the following operation on the array **any** number of times:
* **Increase** or **decrease** an element of the array by `1`.
Return _an array_ `answer` _of size_ `m` _where_ `answer[i]` _is the **minimum** number of operations to make all elements of_ `nums` _equal to_ `queries[i]`.
**Note** that after each query the array is reset to its original state.
**Example 1:**
**Input:** nums = \[3,1,6,8\], queries = \[1,5\]
**Output:** \[14,10\]
**Explanation:** For the first query we can do the following operations:
- Decrease nums\[0\] 2 times, so that nums = \[1,1,6,8\].
- Decrease nums\[2\] 5 times, so that nums = \[1,1,1,8\].
- Decrease nums\[3\] 7 times, so that nums = \[1,1,1,1\].
So the total number of operations for the first query is 2 + 5 + 7 = 14.
For the second query we can do the following operations:
- Increase nums\[0\] 2 times, so that nums = \[5,1,6,8\].
- Increase nums\[1\] 4 times, so that nums = \[5,5,6,8\].
- Decrease nums\[2\] 1 time, so that nums = \[5,5,5,8\].
- Decrease nums\[3\] 3 times, so that nums = \[5,5,5,5\].
So the total number of operations for the second query is 2 + 4 + 1 + 3 = 10.
**Example 2:**
**Input:** nums = \[2,9,6,3\], queries = \[10\]
**Output:** \[20\]
**Explanation:** We can increase each value in the array to 10. The total number of operations will be 8 + 1 + 4 + 7 = 20.
**Constraints:**
* `n == nums.length`
* `m == queries.length`
* `1 <= n, m <= 105`
* `1 <= nums[i], queries[i] <= 109` | null |
Python (Simple Topological Sorting) | collect-coins-in-a-tree | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def collectTheCoins(self, coins, edges):\n if not edges:\n return 0\n\n n, dict1 = len(coins), defaultdict(set)\n\n for i,j in edges:\n dict1[i].add(j)\n dict1[j].add(i)\n\n leaves = [i for i in dict1 if len(dict1[i]) == 1]\n\n for u in leaves:\n while len(dict1[u]) == 1 and coins[u] == 0:\n p = dict1[u].pop()\n del dict1[u]\n dict1[p].remove(u)\n u = p\n\n for _ in range(2):\n leaves = [i for i in dict1 if len(dict1[i]) == 1]\n for u in leaves:\n p = dict1[u].pop()\n del dict1[u]\n dict1[p].remove(u)\n if len(dict1) < 2:\n return 0\n\n return 2*(len(dict1)-1)\n\n\n\n\n\n\n\n\n\n\n \n\n\n\n \n\n \n\n \n\n\n\n\n``` | 1 | There exists an undirected and unrooted tree with `n` nodes indexed from `0` to `n - 1`. You are given an integer `n` and a 2D integer array edges of length `n - 1`, where `edges[i] = [ai, bi]` indicates that there is an edge between nodes `ai` and `bi` in the tree. You are also given an array `coins` of size `n` where `coins[i]` can be either `0` or `1`, where `1` indicates the presence of a coin in the vertex `i`.
Initially, you choose to start at any vertex in the tree. Then, you can perform the following operations any number of times:
* Collect all the coins that are at a distance of at most `2` from the current vertex, or
* Move to any adjacent vertex in the tree.
Find _the minimum number of edges you need to go through to collect all the coins and go back to the initial vertex_.
Note that if you pass an edge several times, you need to count it into the answer several times.
**Example 1:**
**Input:** coins = \[1,0,0,0,0,1\], edges = \[\[0,1\],\[1,2\],\[2,3\],\[3,4\],\[4,5\]\]
**Output:** 2
**Explanation:** Start at vertex 2, collect the coin at vertex 0, move to vertex 3, collect the coin at vertex 5 then move back to vertex 2.
**Example 2:**
**Input:** coins = \[0,0,0,1,1,0,0,1\], edges = \[\[0,1\],\[0,2\],\[1,3\],\[1,4\],\[2,5\],\[5,6\],\[5,7\]\]
**Output:** 2
**Explanation:** Start at vertex 0, collect the coins at vertices 4 and 3, move to vertex 2, collect the coin at vertex 7, then move back to vertex 0.
**Constraints:**
* `n == coins.length`
* `1 <= n <= 3 * 104`
* `0 <= coins[i] <= 1`
* `edges.length == n - 1`
* `edges[i].length == 2`
* `0 <= ai, bi < n`
* `ai != bi`
* `edges` represents a valid tree. | null |
✔💯DAY 361 || 100% || [JAVA/C++/PYTHON] || EXPLAINED || MOST UPVOTED || MEME | collect-coins-in-a-tree | 1 | 1 | 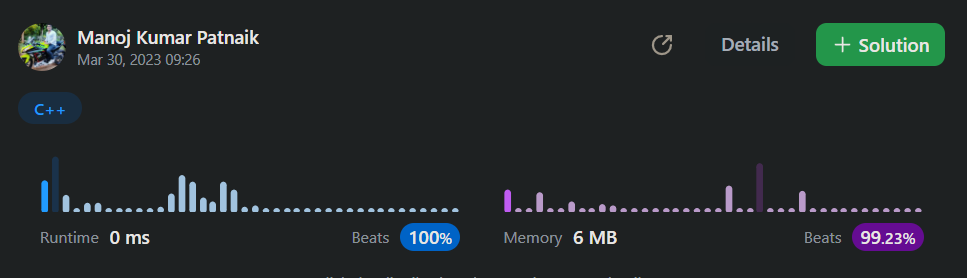\n\n# Please Upvote as it really motivates me\n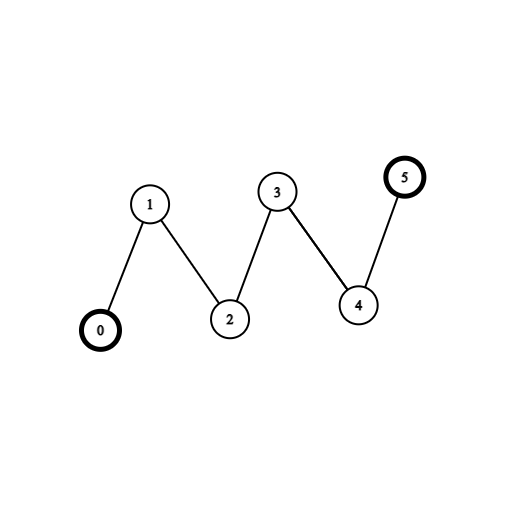\n\n# Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n##### \u2022\tThe goal of this code is to find the minimum number of edges that need to be removed from a given undirected tree such that the resulting graph is a forest (a collection of disjoint trees).\n\n##### \u2022\tTo achieve this, the code first builds the tree from the given edges by creating an adjacency list representation of the graph.\n\n##### \u2022\tNext, the code identifies all the leaves of the tree that have zero coins. A leaf node is a node that has only one neighbor. If a node is a leaf and has zero coins, then it is removed from the tree by removing the edge that connects it to its parent node. This process is repeated until all leaf nodes with zero coins are removed from the tree.\n\n##### \u2022\tAfter removing all such nodes, the tree may still have leaves. The code then removes these leaves one by one, starting with the leaves that are farthest from the root of the tree. The code keeps track of all the leaf nodes that are removed during this process.\n\n##### \u2022\tFinally, the code counts the number of edges that remain in the tree after all leaf nodes with zero coins and all other leaf nodes have been removed. This count represents the minimum number of edges that need to be removed from the original tree to obtain a forest.\n\n##### \u2022\tThe intuition behind this algorithm is to identify the nodes that are less valuable (i.e., nodes with zero coins) and remove them first. This helps in reducing the number of edges that need to be removed from the original tree to obtain a forest. After removing the less valuable nodes, the code then removes the remaining leaf nodes one by one, starting with the leaves that are farthest from the root of the tree. This helps in minimizing the number of edges that need to be removed by preserving the structure of the tree as much as possible.\n\n\n# Approach\n\nHere is a step-by-step explanation of the approach used in this code:\n##### 1. Initialize the variables: The code starts by initializing the variables. The variable n represents the number of nodes in the tree (equal to the length of the coins array). The variable tree is an array of Sets, where each element represents the neighbors of a node. The variable leaf is an ArrayList that will be used to store the leaf nodes of the tree.\n##### 2.\tBuild the tree from the edges: The input graph is represented as an array of coins and a 2D array of edges. The edges are used to build an adjacency list representation of the graph. This is done by iterating through the edges array and adding the corresponding edges to the tree array.\n##### 3.\tFind the leaves with zero coins: A leaf node is a node with only one neighbor. The code iterates through all nodes in the graph and checks if a node has only one neighbor and it has zero coins. If this is the case, the node is removed from the graph by removing the edge connecting it to its neighbor. This process is repeated until all leaf nodes that have zero coins are removed from the graph. The remaining leaf nodes are added to the leaf list.\n##### 4.\tRemove the leaves one by one: The code iteratively removes leaf nodes one by one, starting with the leaves that are farthest from the root of the tree. This is done by iterating through the leaf list and checking if a leaf node has only one neighbor. If it does, the node is removed from the graph by removing the edge connecting it to its neighbor. If the neighbor of the removed node is now a leaf node, it is added to a temporary list temp.\n##### 5.\tCount the remaining edges in the tree: After removing all leaf nodes, the code counts the number of edges that remain in the graph. This is done by iterating through the tree array and counting the size of each Set (i.e., the number of neighbors of each node). The sum of these sizes represents the minimum number of edges that need to be removed to disconnect the graph and make it into a forest.\n\n##### Overall, the approach used in this code is to identify and remove the least valuable nodes (i.e., nodes with zero coins) first, and then iteratively remove the remaining leaf nodes while preserving the structure of the tree as much as possible. This helps in minimizing the number of edges that need to be removed to obtain a forest.\n\n\n\n# Code\n```java []\npublic int collectTheCoins(int[] coins, int[][] edges) {\n int n = coins.length; \n Set<Integer>[] tree = new HashSet[n]; \n for (int i = 0; i < n; ++i) {\n tree[i] = new HashSet(); \n }\n \n // Build the tree from the edges\n for (int[] e : edges) {\n tree[e[0]].add(e[1]); \n tree[e[1]].add(e[0]); \n }\n \n // Find the leaves with zero coins\n List<Integer> leaf = new ArrayList(); \n for (int i = 0; i < n; ++i) {\n int u = i; \n while (tree[u].size() == 1 && coins[u] == 0) {\n int v = tree[u].iterator().next(); \n tree[u].remove(v); \n tree[v].remove(u); \n u = v; \n }\n if (tree[u].size() == 1) {\n leaf.add(u); \n }\n }\n \n // Remove the leaves one by one\n for (int sz = 2; sz > 0; --sz) {\n List<Integer> temp = new ArrayList(); \n for (int u : leaf) {\n if (tree[u].size() == 1) {\n int v = tree[u].iterator().next(); \n tree[u].remove(v); \n tree[v].remove(u); \n if (tree[v].size() == 1) {\n temp.add(v); \n }\n }\n }\n leaf = temp; \n }\n \n // Count the remaining edges in the tree\n int ans = 0; \n for (int i = 0; i < n; ++i) {\n ans += tree[i].size(); \n }\n \n return ans; \n}\n```\n```c++ []\nint collectTheCoins(vector<int>& coins, vector<vector<int>>& edges) {\n int n = coins.size();\n vector<unordered_set<int>> tree(n);\n \n // Build the tree from the edges\n for (auto& e : edges) {\n tree[e[0]].insert(e[1]);\n tree[e[1]].insert(e[0]);\n }\n \n // Find the leaves with zero coins\n vector<int> leaf;\n for (int i = 0; i < n; ++i) {\n int u = i;\n while (tree[u].size() == 1 && coins[u] == 0) {\n int v = *(tree[u].begin());\n tree[u].erase(v);\n tree[v].erase(u);\n u = v;\n }\n if (tree[u].size() == 1) {\n leaf.push_back(u);\n }\n }\n \n // Remove the leaves one by one\n for (int sz = 2; sz > 0; --sz) {\n vector<int> temp;\n for (int u : leaf) {\n if (tree[u].size() == 1) {\n int v = *(tree[u].begin());\n tree[u].erase(v);\n tree[v].erase(u);\n if (tree[v].size() == 1) {\n temp.push_back(v);\n }\n }\n }\n leaf = temp;\n }\n \n // Count the remaining edges in the tree\n int ans = 0;\n for (int i = 0; i < n; ++i) {\n ans += tree[i].size();\n }\n \n return ans;\n}\n```\n```python []\ndef collectTheCoins(coins: List[int], edges: List[List[int]]) -> int:\n n = len(coins)\n tree = [set() for _ in range(n)]\n \n # Build the tree from the edges\n for e in edges:\n tree[e[0]].add(e[1])\n tree[e[1]].add(e[0])\n \n # Find the leaves with zero coins\n leaf = []\n for i in range(n):\n u = i\n while len(tree[u]) == 1 and coins[u] == 0:\n v = tree[u].pop()\n tree[v].remove(u)\n u = v\n if len(tree[u]) == 1:\n leaf.append(u)\n \n # Remove the leaves one by one\n for sz in range(2, 0, -1):\n temp = []\n for u in leaf:\n if len(tree[u]) == 1:\n v = tree[u].pop()\n tree[v].remove(u)\n if len(tree[v]) == 1:\n temp.append(v)\n leaf = temp\n \n # Count the remaining edges in the tree\n ans = 0\n for i in range(n):\n ans += len(tree[i])\n \n return ans\n```\n\n# Complexity\nHere\'s the time complexity and space complexity analysis of the algorithm:\n# Time complexity:\n##### \u2022\tBuilding the tree from the edges takes O(N), where N is the number of nodes in the tree.\n##### \u2022\t\tFinding the leaves with zero coins takes O(N+2E) in the worst case, because we may have to iterate through all nodes and their neighbors multiple times to remove zero-coin leaves.\n##### \u2022\t\tRemoving the leaves one by one takes O(N), because each leaf is removed only once.\n##### \u2022\t\tCounting the remaining edges in the tree takes O(N), because we have to iterate through all nodes and their neighbors once.\nTherefore, the total time complexity of the algorithm is O(N+2E), dominated by the step of finding the leaves with zero coins.\nSpace complexity:\n##### \u2022\t\tBuilding the tree array takes O(N) space.\n##### \u2022\t\tThe leaf list can have up to N elements, so it takes O(N) space.\n##### \u2022\tThe temp list used during leaf removal can also have up to N elements, so it takes O(N) space.\nTherefore, the total space complexity of the algorithm is O(N).\nIn summary, the algorithm has quadratic time complexity and linear space complexity.\n# UPVOTE PLEASE\n# 2nd way\n```java []\nclass Solution {\n public int collectTheCoins(int[] coins, int[][] edges) {\n int n = coins.length;\n List<Integer>[] g = new List[n];\n // Initialize adjacency list\n for (int i = 0; i < n; i++) g[i] = new ArrayList<>();\n\n // create a degree to for topological iteration\n int[] deg = new int[n];\n for (int[] edge : edges) {\n deg[edge[0]]++;\n deg[edge[1]]++;\n g[edge[0]].add(edge[1]);\n g[edge[1]].add(edge[0]);\n }\n\n // remove non-coin leaf node first\n Queue<Integer> q = new ArrayDeque<>();\n for (int i = 0; i < n; i++)\n if (deg[i] == 1 && coins[i] == 0)\n q.add(i);\n\n // total amount of edges in the graph\n int totalEdges = n - 1;\n while (!q.isEmpty()) {\n // remove the current edge\n int d = q.poll();\n totalEdges -= 1;\n // update neighbor degree\n for (int neigh : g[d]) {\n deg[neigh]--;\n if (deg[neigh] == 1 && coins[neigh] == 0) {\n q.add(neigh);\n }\n }\n }\n // we have remove all non-coin leaves, now remove two degree of coins\n for (int i = 0; i < n; i++)\n if (deg[i] == 1 && coins[i] == 1)\n q.add(i);\n\n totalEdges -= q.size();\n for (int x : q) for (int neigh : g[x])\n if (--deg[neigh] == 1)\n totalEdges -= 1;\n\n // we might have negative case if there is no coin, check edge cases\n return Math.max(totalEdges * 2, 0);\n }\n\n}\n```\n```python []\ndef _build_tree(edges: list[list[int]]) -> list[list[int]]:\n tree = [[] for _ in range(len(edges) + 1)]\n\n for vertex, adjacent in edges:\n tree[vertex].append(adjacent)\n tree[adjacent].append(vertex)\n\n return tree\n\n\nclass Solution:\n def collectTheCoins(self, coins: list[int], edges: list[list[int]]) -> int:\n tree = _build_tree(edges)\n degree = list(map(len, tree))\n\n queue = collections.deque()\n\n for vertex in range(len(tree)):\n if degree[vertex] == 1:\n if coins[vertex]:\n queue.append((vertex, True))\n else:\n queue.appendleft((vertex, True))\n\n count_pruned = 0\n\n while queue:\n vertex, is_leaf = queue.popleft()\n count_pruned += 1\n\n for adjacent in tree[vertex]:\n degree[adjacent] -= 1\n\n if is_leaf and degree[adjacent] == 1:\n if coins[vertex]:\n queue.append((adjacent, False))\n elif coins[adjacent]:\n queue.append((adjacent, True))\n else:\n queue.appendleft((adjacent, True))\n\n return 2 * max(0, len(edges) - count_pruned)\n```\n```c++ []\n#ifndef out\n#define out(...)\n#endif\ntemplate<class Tp, class Up = typename std::remove_reference<Tp>::type>\nconstexpr auto Max(Tp &&a, const Up &b) -> decltype(a < b && (a = b, true)) { return a < b && (a = b, true); }\n\nconst int N = 3e4 + 5;\nstruct Edge {\n Edge *next;\n int to;\n} container[N * 2];\n\nvoid add(int from, int to, vector<Edge *> &next, int &top) {\n next[from] = &(container[top++] = Edge{\n .next = next[from],\n .to = to,\n });\n}\n\nint dfs1(\n int cur, int f, int depth,\n const vector<Edge *> &next,\n const vector<int> &coins,\n int &root) {\n int ret = coins[cur] ? depth : -1;\n for (auto p = next[cur]; p; p = p->next) {\n int to = p->to;\n if (to != f) {\n Max(ret, dfs1(to, cur, depth + 1, next, coins, root));\n if (root >= 0) break;\n if (ret >= 0 && ret - depth >= 2) {\n root = cur;\n break;\n }\n }\n }\n return ret;\n}\n\npair<int, int> dfs2(\n int cur, int f, int depth,\n const vector<Edge *> &next,\n const vector<int> &coins) {\n int max_depth = coins[cur] ? depth : -1;\n int sum = 0;\n for (auto p = next[cur]; p; p = p->next) {\n int to = p->to;\n if (to != f) {\n auto [dp, s] = dfs2(to, cur, depth + 1, next, coins);\n Max(max_depth, dp);\n if (dp >= 0 && dp - depth > 2) {\n sum += 1 + s;\n }\n }\n }\n return {max_depth, sum};\n}\n\nclass Solution {\npublic:\n static int collectTheCoins(const vector<int> &coins, const vector<vector<int>> &edges) {\n size_t n = coins.size();\n assert(n == edges.size() + 1);\n vector<Edge *> next(n);\n int top = 0;\n for (auto &&e: edges) {\n int x = e[0], y = e[1];\n add(x, y, next, top);\n add(y, x, next, top);\n }\n vector<int> mark;\n int root = -1;\n dfs1(0, -1, 0, next, coins, root);\n if (root < 0) return 0;\n out(root);\n return dfs2(root, -1, 0, next, coins).second * 2;\n }\n};\n```\n\n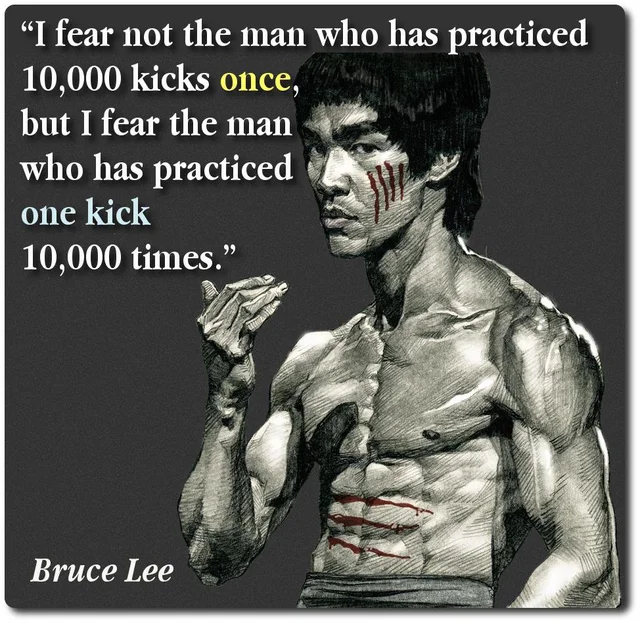\n\n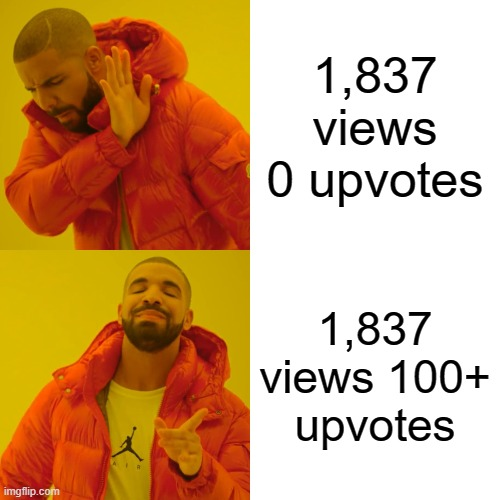\n\n# Please Upvote\uD83D\uDC4D\uD83D\uDC4D\nThanks for visiting my solution.\uD83D\uDE0A Keep Learning\nPlease give my solution an upvote! \uD83D\uDC4D\nIt\'s a simple way to show your appreciation and\nkeep me motivated. Thank you! \uD83D\uDE0A\n | 26 | There exists an undirected and unrooted tree with `n` nodes indexed from `0` to `n - 1`. You are given an integer `n` and a 2D integer array edges of length `n - 1`, where `edges[i] = [ai, bi]` indicates that there is an edge between nodes `ai` and `bi` in the tree. You are also given an array `coins` of size `n` where `coins[i]` can be either `0` or `1`, where `1` indicates the presence of a coin in the vertex `i`.
Initially, you choose to start at any vertex in the tree. Then, you can perform the following operations any number of times:
* Collect all the coins that are at a distance of at most `2` from the current vertex, or
* Move to any adjacent vertex in the tree.
Find _the minimum number of edges you need to go through to collect all the coins and go back to the initial vertex_.
Note that if you pass an edge several times, you need to count it into the answer several times.
**Example 1:**
**Input:** coins = \[1,0,0,0,0,1\], edges = \[\[0,1\],\[1,2\],\[2,3\],\[3,4\],\[4,5\]\]
**Output:** 2
**Explanation:** Start at vertex 2, collect the coin at vertex 0, move to vertex 3, collect the coin at vertex 5 then move back to vertex 2.
**Example 2:**
**Input:** coins = \[0,0,0,1,1,0,0,1\], edges = \[\[0,1\],\[0,2\],\[1,3\],\[1,4\],\[2,5\],\[5,6\],\[5,7\]\]
**Output:** 2
**Explanation:** Start at vertex 0, collect the coins at vertices 4 and 3, move to vertex 2, collect the coin at vertex 7, then move back to vertex 0.
**Constraints:**
* `n == coins.length`
* `1 <= n <= 3 * 104`
* `0 <= coins[i] <= 1`
* `edges.length == n - 1`
* `edges[i].length == 2`
* `0 <= ai, bi < n`
* `ai != bi`
* `edges` represents a valid tree. | null |
Trim Graph | collect-coins-in-a-tree | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n- Idea was to first prune leaf nodes with no coin and continue this process till no leaf node is possible to remove .\n- Afterwards we can remove two layers of leaves and then at last we return 2*(number of edges still left) as each edge is traversed twice.\n- Reason why we are removing two layers of leaf nodes because if a leaf is chosen as start vertex then its parent is a better option then the leaf and similarly for that parent its parent is better option . So hence we can remove two level of nodes. \n\nIf you guys liked the explanation do Upvote it motivates alot. Thank you for reading the solution\n\n\n# Code\n```\nclass Solution:\n def collectTheCoins(self, coins: List[int], edges: List[List[int]]) -> int:\n n = len(coins)\n ans = n - 1\n \n adj = [set() for i in range(n)]\n for i, j in edges:\n adj[i].add(j)\n adj[j].add(i)\n \n l = [i for i in range(n) if len(adj[i]) == 1 and coins[i] == 0]\n while l:\n nextlayer = []\n for i in l:\n # Prune leaf i\n ans -= 1\n if adj[i]:\n j = list(adj[i])[0]\n adj[j].remove(i)\n adj[i].remove(j)\n if len(adj[j]) == 1 and coins[j] == 0:\n nextlayer.append(j)\n l = nextlayer[:]\n \n l = [i for i in range(n) if len(adj[i]) == 1]\n \n for _ in range(2):\n nextlayer = []\n for i in l:\n ans -= 1\n if adj[i]:\n j = list(adj[i])[0]\n adj[j].remove(i)\n if len(adj[j]) == 1:\n nextlayer.append(j)\n l = nextlayer[:]\n \n return 2 * max(0, ans)\n\n``` | 5 | There exists an undirected and unrooted tree with `n` nodes indexed from `0` to `n - 1`. You are given an integer `n` and a 2D integer array edges of length `n - 1`, where `edges[i] = [ai, bi]` indicates that there is an edge between nodes `ai` and `bi` in the tree. You are also given an array `coins` of size `n` where `coins[i]` can be either `0` or `1`, where `1` indicates the presence of a coin in the vertex `i`.
Initially, you choose to start at any vertex in the tree. Then, you can perform the following operations any number of times:
* Collect all the coins that are at a distance of at most `2` from the current vertex, or
* Move to any adjacent vertex in the tree.
Find _the minimum number of edges you need to go through to collect all the coins and go back to the initial vertex_.
Note that if you pass an edge several times, you need to count it into the answer several times.
**Example 1:**
**Input:** coins = \[1,0,0,0,0,1\], edges = \[\[0,1\],\[1,2\],\[2,3\],\[3,4\],\[4,5\]\]
**Output:** 2
**Explanation:** Start at vertex 2, collect the coin at vertex 0, move to vertex 3, collect the coin at vertex 5 then move back to vertex 2.
**Example 2:**
**Input:** coins = \[0,0,0,1,1,0,0,1\], edges = \[\[0,1\],\[0,2\],\[1,3\],\[1,4\],\[2,5\],\[5,6\],\[5,7\]\]
**Output:** 2
**Explanation:** Start at vertex 0, collect the coins at vertices 4 and 3, move to vertex 2, collect the coin at vertex 7, then move back to vertex 0.
**Constraints:**
* `n == coins.length`
* `1 <= n <= 3 * 104`
* `0 <= coins[i] <= 1`
* `edges.length == n - 1`
* `edges[i].length == 2`
* `0 <= ai, bi < n`
* `ai != bi`
* `edges` represents a valid tree. | null |
python3 Solution | form-smallest-number-from-two-digit-arrays | 0 | 1 | \n\n# Code\n```\nclass Solution:\n def minNumber(self, nums1: List[int], nums2: List[int]) -> int:\n if set(nums1).intersection(set(nums2)):\n return sorted(set(nums1).intersection(set(nums2)))[0]\n\n a=sorted(nums1)[0]\n b=sorted(nums2)[0]\n return min(a*10+b,b*10+a) \n``` | 1 | Given two arrays of **unique** digits `nums1` and `nums2`, return _the **smallest** number that contains **at least** one digit from each array_.
**Example 1:**
**Input:** nums1 = \[4,1,3\], nums2 = \[5,7\]
**Output:** 15
**Explanation:** The number 15 contains the digit 1 from nums1 and the digit 5 from nums2. It can be proven that 15 is the smallest number we can have.
**Example 2:**
**Input:** nums1 = \[3,5,2,6\], nums2 = \[3,1,7\]
**Output:** 3
**Explanation:** The number 3 contains the digit 3 which exists in both arrays.
**Constraints:**
* `1 <= nums1.length, nums2.length <= 9`
* `1 <= nums1[i], nums2[i] <= 9`
* All digits in each array are **unique**. | null |
Python 3 || 3 lines, set || T/M: 100% / 100% | form-smallest-number-from-two-digit-arrays | 0 | 1 | ```\nclass Solution:\n def minNumber(self, nums1: List[int], nums2: List[int]) -> int:\n \n if mn := min(set(nums1)&set(nums2),\n default = None): return mn # <-- one-digit case\n\n d1, d2 = min(nums1), min(nums2) # <-- two-digit case\n return 10*d1+d2 if d1 < d2 else 10*d2+d1 #\n```\n[https://leetcode.com/problems/form-smallest-number-from-two-digit-arrays/submissions/926220757/](http://)\n\nI could be wrong, but I think that time complexity is *O*(*N1+N2*) and space complexity is *O*(*N*).\n | 4 | Given two arrays of **unique** digits `nums1` and `nums2`, return _the **smallest** number that contains **at least** one digit from each array_.
**Example 1:**
**Input:** nums1 = \[4,1,3\], nums2 = \[5,7\]
**Output:** 15
**Explanation:** The number 15 contains the digit 1 from nums1 and the digit 5 from nums2. It can be proven that 15 is the smallest number we can have.
**Example 2:**
**Input:** nums1 = \[3,5,2,6\], nums2 = \[3,1,7\]
**Output:** 3
**Explanation:** The number 3 contains the digit 3 which exists in both arrays.
**Constraints:**
* `1 <= nums1.length, nums2.length <= 9`
* `1 <= nums1[i], nums2[i] <= 9`
* All digits in each array are **unique**. | null |
Intersection | form-smallest-number-from-two-digit-arrays | 0 | 1 | \n**Python 3**\n```python\nclass Solution:\n def minNumber(self, n1: List[int], n2: List[int]) -> int:\n common, m1, m2 = set(n1).intersection(n2), min(n1), min(n2)\n return min(common) if common else min(m1, m2) * 10 + max(m1, m2)\n``` | 23 | Given two arrays of **unique** digits `nums1` and `nums2`, return _the **smallest** number that contains **at least** one digit from each array_.
**Example 1:**
**Input:** nums1 = \[4,1,3\], nums2 = \[5,7\]
**Output:** 15
**Explanation:** The number 15 contains the digit 1 from nums1 and the digit 5 from nums2. It can be proven that 15 is the smallest number we can have.
**Example 2:**
**Input:** nums1 = \[3,5,2,6\], nums2 = \[3,1,7\]
**Output:** 3
**Explanation:** The number 3 contains the digit 3 which exists in both arrays.
**Constraints:**
* `1 <= nums1.length, nums2.length <= 9`
* `1 <= nums1[i], nums2[i] <= 9`
* All digits in each array are **unique**. | null |
[Python3] check intersection | form-smallest-number-from-two-digit-arrays | 0 | 1 | Please pull this [commit](https://github.com/gaosanyong/leetcode/commit/1dc118daa80cfe1161dcee412e7c3536970ca60d) for solutions of biweely 101. \n\n```\nclass Solution:\n def minNumber(self, nums1: List[int], nums2: List[int]) -> int:\n inter = set(nums1) & set(nums2)\n if inter: return min(inter)\n d1 = min(nums1)\n d2 = min(nums2)\n return 10*d1+d2 if d1 < d2 else 10*d2+d1\n``` | 3 | Given two arrays of **unique** digits `nums1` and `nums2`, return _the **smallest** number that contains **at least** one digit from each array_.
**Example 1:**
**Input:** nums1 = \[4,1,3\], nums2 = \[5,7\]
**Output:** 15
**Explanation:** The number 15 contains the digit 1 from nums1 and the digit 5 from nums2. It can be proven that 15 is the smallest number we can have.
**Example 2:**
**Input:** nums1 = \[3,5,2,6\], nums2 = \[3,1,7\]
**Output:** 3
**Explanation:** The number 3 contains the digit 3 which exists in both arrays.
**Constraints:**
* `1 <= nums1.length, nums2.length <= 9`
* `1 <= nums1[i], nums2[i] <= 9`
* All digits in each array are **unique**. | null |
BEATS 100 % SUBMISSIONS || Easy solution using Hashmap!! | form-smallest-number-from-two-digit-arrays | 0 | 1 | # Complexity\n- Time complexity:\nO(N)\n- Space complexity:\nO(N)\n\n# Code\n```\nfrom collections import Counter\nclass Solution:\n def minNumber(self, nums1: List[int], nums2: List[int]) -> int:\n a=nums1[:]\n a.extend(nums2)\n dic=Counter(a)\n m=max(dic.values())\n ans=[]\n if m>1:\n for i,j in dic.items():\n if j==m:\n ans.append(i)\n return min(ans)\n else:\n min1=min(nums1)\n min2=min(nums2)\n if min1>min2:\n return int(str(min2)+str(min1))\n else:\n return int(str(min1)+str(min2))\n \n``` | 1 | Given two arrays of **unique** digits `nums1` and `nums2`, return _the **smallest** number that contains **at least** one digit from each array_.
**Example 1:**
**Input:** nums1 = \[4,1,3\], nums2 = \[5,7\]
**Output:** 15
**Explanation:** The number 15 contains the digit 1 from nums1 and the digit 5 from nums2. It can be proven that 15 is the smallest number we can have.
**Example 2:**
**Input:** nums1 = \[3,5,2,6\], nums2 = \[3,1,7\]
**Output:** 3
**Explanation:** The number 3 contains the digit 3 which exists in both arrays.
**Constraints:**
* `1 <= nums1.length, nums2.length <= 9`
* `1 <= nums1[i], nums2[i] <= 9`
* All digits in each array are **unique**. | null |
Be Greedy! | form-smallest-number-from-two-digit-arrays | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nIf there is a digit that is in both the lists, then that is the answer.\nElse, the concatenation of the two smallest numbers from the list will be the answer. See the code for more clarification.\n\n\n\n# Code\n```\nclass Solution:\n def minNumber(self, nums1: List[int], nums2: List[int]) -> int:\n nums1.sort()\n nums2.sort()\n for i in nums1:\n if i in nums2:\n return i\n ans = (str(nums1[0]) + str(nums2[0])) if nums1[0] < nums2[0] else str(nums2[0]) + str(nums1[0]) \n ans = int(ans)\n return ans\n``` | 1 | Given two arrays of **unique** digits `nums1` and `nums2`, return _the **smallest** number that contains **at least** one digit from each array_.
**Example 1:**
**Input:** nums1 = \[4,1,3\], nums2 = \[5,7\]
**Output:** 15
**Explanation:** The number 15 contains the digit 1 from nums1 and the digit 5 from nums2. It can be proven that 15 is the smallest number we can have.
**Example 2:**
**Input:** nums1 = \[3,5,2,6\], nums2 = \[3,1,7\]
**Output:** 3
**Explanation:** The number 3 contains the digit 3 which exists in both arrays.
**Constraints:**
* `1 <= nums1.length, nums2.length <= 9`
* `1 <= nums1[i], nums2[i] <= 9`
* All digits in each array are **unique**. | null |
simple solution in python | form-smallest-number-from-two-digit-arrays | 0 | 1 | \n```\nclass Solution:\n def minNumber(self, nums1: List[int], nums2: List[int]) -> int:\n nums1.sort()\n nums2.sort()\n for i in nums1:\n if i in nums2:\n return i\n break\n else:\n return min(nums1[0]*10+nums2[0],nums2[0]*10+nums1[0])\n \n``` | 2 | Given two arrays of **unique** digits `nums1` and `nums2`, return _the **smallest** number that contains **at least** one digit from each array_.
**Example 1:**
**Input:** nums1 = \[4,1,3\], nums2 = \[5,7\]
**Output:** 15
**Explanation:** The number 15 contains the digit 1 from nums1 and the digit 5 from nums2. It can be proven that 15 is the smallest number we can have.
**Example 2:**
**Input:** nums1 = \[3,5,2,6\], nums2 = \[3,1,7\]
**Output:** 3
**Explanation:** The number 3 contains the digit 3 which exists in both arrays.
**Constraints:**
* `1 <= nums1.length, nums2.length <= 9`
* `1 <= nums1[i], nums2[i] <= 9`
* All digits in each array are **unique**. | null |
Python | Easy Solution✅ | form-smallest-number-from-two-digit-arrays | 0 | 1 | # Code\n```\nclass Solution:\n def minNumber(self, nums1: List[int], nums2: List[int]) -> int:\n small1, small2, common = min(nums1), min(nums2), set(nums1) & set(nums2)\n output1, output2 = int(f"{small1}{small2}"), int(f"{small2}{small1}")\n if common:\n common = min(common)\n if common < output1 and common < output2:\n return common\n if small1 < small2:\n return output1\n return output2\n \n``` | 4 | Given two arrays of **unique** digits `nums1` and `nums2`, return _the **smallest** number that contains **at least** one digit from each array_.
**Example 1:**
**Input:** nums1 = \[4,1,3\], nums2 = \[5,7\]
**Output:** 15
**Explanation:** The number 15 contains the digit 1 from nums1 and the digit 5 from nums2. It can be proven that 15 is the smallest number we can have.
**Example 2:**
**Input:** nums1 = \[3,5,2,6\], nums2 = \[3,1,7\]
**Output:** 3
**Explanation:** The number 3 contains the digit 3 which exists in both arrays.
**Constraints:**
* `1 <= nums1.length, nums2.length <= 9`
* `1 <= nums1[i], nums2[i] <= 9`
* All digits in each array are **unique**. | null |
Python3 Solution Well Explained (Easy & Clear) | find-the-substring-with-maximum-cost | 0 | 1 | # Intuition:\nThe problem requires finding the maximum cost among all substrings of the given string s. We can find the cost of each character by assigning a value to each character based on whether it is present in the given chars string or not. We can then find the cost of each substring using this assigned value for each character and return the maximum cost.\n\n# Approach:\nWe first create a dictionary to map each character to its corresponding value based on whether it is present in the given chars string or not. We then create a list xx where we replace each character of the given string s with its assigned value using the dictionary we created. We then use the Kadane\'s algorithm to find the maximum sum contiguous subarray of xx, which gives us the maximum cost of a substring. We return this maximum cost if it is greater than 0, else we return 0.\n\n# Complexity:\n# Time Complexity: \nThe time complexity of creating the dictionary is O(26+L) where L is the length of the chars string. The time complexity of creating the xx list is O(N) where N is the length of the given string s. The time complexity of the Kadane\'s algorithm is O(N). Hence, the overall time complexity is O(N+L).\n\n# Space Complexity: \nThe space complexity is O(26+L+N) where L is the length of the chars string and N is the length of the given string s. The extra space is used for the dictionary, xx list, and Kadane\'s algorithm.\n\n# Code\n```\nclass Solution:\n def maximumCostSubstring(self, s: str, chars: str, vals: List[int]) -> int:\n i=1\n m=dict()\n for c in ascii_lowercase:\n if c in chars:\n m.update({c:vals[chars.index(c)]})\n else:\n m.update({c:i})\n i+=1\n xx=[]\n for i in range(len(s)):\n xx.append(m[s[i]])\n print(xx)\n def maxSubArray(nums: List[int]) -> int:\n max_sum=nums[0]\n temp=0\n for i in range(len(nums)):\n if temp<0:\n temp=0\n temp+=nums[i]\n if temp>max_sum:\n max_sum=temp\n return max_sum\n \n \n \n return max(maxSubArray(xx),0)\n``` | 3 | You are given a string `s`, a string `chars` of **distinct** characters and an integer array `vals` of the same length as `chars`.
The **cost of the substring** is the sum of the values of each character in the substring. The cost of an empty string is considered `0`.
The **value of the character** is defined in the following way:
* If the character is not in the string `chars`, then its value is its corresponding position **(1-indexed)** in the alphabet.
* For example, the value of `'a'` is `1`, the value of `'b'` is `2`, and so on. The value of `'z'` is `26`.
* Otherwise, assuming `i` is the index where the character occurs in the string `chars`, then its value is `vals[i]`.
Return _the maximum cost among all substrings of the string_ `s`.
**Example 1:**
**Input:** s = "adaa ", chars = "d ", vals = \[-1000\]
**Output:** 2
**Explanation:** The value of the characters "a " and "d " is 1 and -1000 respectively.
The substring with the maximum cost is "aa " and its cost is 1 + 1 = 2.
It can be proven that 2 is the maximum cost.
**Example 2:**
**Input:** s = "abc ", chars = "abc ", vals = \[-1,-1,-1\]
**Output:** 0
**Explanation:** The value of the characters "a ", "b " and "c " is -1, -1, and -1 respectively.
The substring with the maximum cost is the empty substring " " and its cost is 0.
It can be proven that 0 is the maximum cost.
**Constraints:**
* `1 <= s.length <= 105`
* `s` consist of lowercase English letters.
* `1 <= chars.length <= 26`
* `chars` consist of **distinct** lowercase English letters.
* `vals.length == chars.length`
* `-1000 <= vals[i] <= 1000` | null |
[Python3] Kadane-ish DP | find-the-substring-with-maximum-cost | 0 | 1 | Please pull this [commit](https://github.com/gaosanyong/leetcode/commit/1dc118daa80cfe1161dcee412e7c3536970ca60d) for solutions of biweely 101. \n\n```\nclass Solution:\n def maximumCostSubstring(self, s: str, chars: str, vals: List[int]) -> int:\n mp = dict(zip(chars, vals))\n ans = val = 0 \n for i, ch in enumerate(s):\n val = max(0, val + mp.get(ch, ord(ch)-96))\n ans = max(ans, val)\n return ans \n``` | 3 | You are given a string `s`, a string `chars` of **distinct** characters and an integer array `vals` of the same length as `chars`.
The **cost of the substring** is the sum of the values of each character in the substring. The cost of an empty string is considered `0`.
The **value of the character** is defined in the following way:
* If the character is not in the string `chars`, then its value is its corresponding position **(1-indexed)** in the alphabet.
* For example, the value of `'a'` is `1`, the value of `'b'` is `2`, and so on. The value of `'z'` is `26`.
* Otherwise, assuming `i` is the index where the character occurs in the string `chars`, then its value is `vals[i]`.
Return _the maximum cost among all substrings of the string_ `s`.
**Example 1:**
**Input:** s = "adaa ", chars = "d ", vals = \[-1000\]
**Output:** 2
**Explanation:** The value of the characters "a " and "d " is 1 and -1000 respectively.
The substring with the maximum cost is "aa " and its cost is 1 + 1 = 2.
It can be proven that 2 is the maximum cost.
**Example 2:**
**Input:** s = "abc ", chars = "abc ", vals = \[-1,-1,-1\]
**Output:** 0
**Explanation:** The value of the characters "a ", "b " and "c " is -1, -1, and -1 respectively.
The substring with the maximum cost is the empty substring " " and its cost is 0.
It can be proven that 0 is the maximum cost.
**Constraints:**
* `1 <= s.length <= 105`
* `s` consist of lowercase English letters.
* `1 <= chars.length <= 26`
* `chars` consist of **distinct** lowercase English letters.
* `vals.length == chars.length`
* `-1000 <= vals[i] <= 1000` | null |
Simple Easy Solution | Beats 99% | Accepted🔥🚀🚀 | find-the-substring-with-maximum-cost | 0 | 1 | # Code\n```\nclass Solution:\n def maximumCostSubstring(self, a: str, c: str, x: List[int]) -> int:\n y=[]\n for i in a:\n if i in c: y.append(x[c.index(i)])\n else: y.append(ord(i)-96)\n s=t=0\n for i in y:\n t=max(t,0)\n s=max(s,t)\n t+=i\n return max(s,t,0)\n``` | 1 | You are given a string `s`, a string `chars` of **distinct** characters and an integer array `vals` of the same length as `chars`.
The **cost of the substring** is the sum of the values of each character in the substring. The cost of an empty string is considered `0`.
The **value of the character** is defined in the following way:
* If the character is not in the string `chars`, then its value is its corresponding position **(1-indexed)** in the alphabet.
* For example, the value of `'a'` is `1`, the value of `'b'` is `2`, and so on. The value of `'z'` is `26`.
* Otherwise, assuming `i` is the index where the character occurs in the string `chars`, then its value is `vals[i]`.
Return _the maximum cost among all substrings of the string_ `s`.
**Example 1:**
**Input:** s = "adaa ", chars = "d ", vals = \[-1000\]
**Output:** 2
**Explanation:** The value of the characters "a " and "d " is 1 and -1000 respectively.
The substring with the maximum cost is "aa " and its cost is 1 + 1 = 2.
It can be proven that 2 is the maximum cost.
**Example 2:**
**Input:** s = "abc ", chars = "abc ", vals = \[-1,-1,-1\]
**Output:** 0
**Explanation:** The value of the characters "a ", "b " and "c " is -1, -1, and -1 respectively.
The substring with the maximum cost is the empty substring " " and its cost is 0.
It can be proven that 0 is the maximum cost.
**Constraints:**
* `1 <= s.length <= 105`
* `s` consist of lowercase English letters.
* `1 <= chars.length <= 26`
* `chars` consist of **distinct** lowercase English letters.
* `vals.length == chars.length`
* `-1000 <= vals[i] <= 1000` | null |
Python3 Solution | find-the-substring-with-maximum-cost | 0 | 1 | \n```\nclass Solution:\n def maximumCostSubstring(self, s: str, chars: str, vals: List[int]) -> int:\n nums = []\n c = {i: idx for idx, i in enumerate(chars)}\n \n for i in s:\n if i in c:\n nums.append(vals[c[i]])\n else:\n nums.append(ord(i) - ord(\'a\') + 1)\n \n res =0\n total = 0\n \n for i in nums:\n total += i\n res = max(res, total)\n \n if total < 0:\n total = 0\n \n return res \n``` | 1 | You are given a string `s`, a string `chars` of **distinct** characters and an integer array `vals` of the same length as `chars`.
The **cost of the substring** is the sum of the values of each character in the substring. The cost of an empty string is considered `0`.
The **value of the character** is defined in the following way:
* If the character is not in the string `chars`, then its value is its corresponding position **(1-indexed)** in the alphabet.
* For example, the value of `'a'` is `1`, the value of `'b'` is `2`, and so on. The value of `'z'` is `26`.
* Otherwise, assuming `i` is the index where the character occurs in the string `chars`, then its value is `vals[i]`.
Return _the maximum cost among all substrings of the string_ `s`.
**Example 1:**
**Input:** s = "adaa ", chars = "d ", vals = \[-1000\]
**Output:** 2
**Explanation:** The value of the characters "a " and "d " is 1 and -1000 respectively.
The substring with the maximum cost is "aa " and its cost is 1 + 1 = 2.
It can be proven that 2 is the maximum cost.
**Example 2:**
**Input:** s = "abc ", chars = "abc ", vals = \[-1,-1,-1\]
**Output:** 0
**Explanation:** The value of the characters "a ", "b " and "c " is -1, -1, and -1 respectively.
The substring with the maximum cost is the empty substring " " and its cost is 0.
It can be proven that 0 is the maximum cost.
**Constraints:**
* `1 <= s.length <= 105`
* `s` consist of lowercase English letters.
* `1 <= chars.length <= 26`
* `chars` consist of **distinct** lowercase English letters.
* `vals.length == chars.length`
* `-1000 <= vals[i] <= 1000` | null |
Python 3 || 3 lines, w/ example || T/M: 288 ms / 15.7 MB | find-the-substring-with-maximum-cost | 0 | 1 | ```\nclass Solution:\n def maximumCostSubstring(self, s: str, chars: str, vals: List[int])-> int:\n # Example: s = "adcnc"\n # chars = "dn" \n # vals = [-10,-2]\n d = {ch:ord(ch)-96 for ch in s} \n # d = {\'a\':1,\'d\': 4,\'c\':3,\'n\':14} \n for ch,val in zip(chars,vals): d[ch] = val # d = {\'a\':1,\'d\':-10,\'c\':3,\'n\':-2}\n # \n # [d[ch] for ch in s] = [1,-10,3,-2,3]\n \n return max(accumulate([d[ch] for ch in s], # <-- max([0,1,0,3,1,4])\n lambda x, y: max(x+y, 0), initial = 0)) # |\n # return\n```\n[https://leetcode.com/problems/find-the-substring-with-maximum-cost/submissions/926247641/](http://)\n\nI could be wrong, but I think that time complexity is *O*(*N*) and space complexity is *O*(*N*). | 4 | You are given a string `s`, a string `chars` of **distinct** characters and an integer array `vals` of the same length as `chars`.
The **cost of the substring** is the sum of the values of each character in the substring. The cost of an empty string is considered `0`.
The **value of the character** is defined in the following way:
* If the character is not in the string `chars`, then its value is its corresponding position **(1-indexed)** in the alphabet.
* For example, the value of `'a'` is `1`, the value of `'b'` is `2`, and so on. The value of `'z'` is `26`.
* Otherwise, assuming `i` is the index where the character occurs in the string `chars`, then its value is `vals[i]`.
Return _the maximum cost among all substrings of the string_ `s`.
**Example 1:**
**Input:** s = "adaa ", chars = "d ", vals = \[-1000\]
**Output:** 2
**Explanation:** The value of the characters "a " and "d " is 1 and -1000 respectively.
The substring with the maximum cost is "aa " and its cost is 1 + 1 = 2.
It can be proven that 2 is the maximum cost.
**Example 2:**
**Input:** s = "abc ", chars = "abc ", vals = \[-1,-1,-1\]
**Output:** 0
**Explanation:** The value of the characters "a ", "b " and "c " is -1, -1, and -1 respectively.
The substring with the maximum cost is the empty substring " " and its cost is 0.
It can be proven that 0 is the maximum cost.
**Constraints:**
* `1 <= s.length <= 105`
* `s` consist of lowercase English letters.
* `1 <= chars.length <= 26`
* `chars` consist of **distinct** lowercase English letters.
* `vals.length == chars.length`
* `-1000 <= vals[i] <= 1000` | null |
I WILL OPEN IT AT MY OWN RISK(SOME GHOST MAY APPEAR IN SOLUTION) | find-the-substring-with-maximum-cost | 1 | 1 | # Intuition\nTRIPPY\'S BAKCHOD APPROACH\nYou may easily convert into c++ and java\n\n# Approach\nSS Smile and solve\n\n# Complexity\nNothing\n\n# WARNING BEFORE MOVING TO SOL\n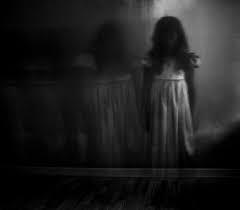\n\n\n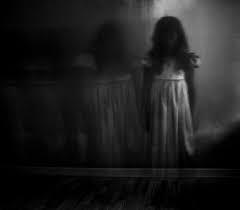\n\n\n\n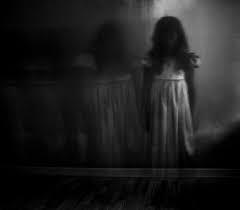\n\n\n\n# GHOST FREE Code\n```\nfrom typing import List\n\nclass Solution:\n def maximumCostSubstring(self, s: str, chars: str, vals: List[int]) -> int:\n char_dict = {}\n for i in range(len(chars)):\n char_dict[chars[i]] = vals[i]\n \n max_cost = 0\n curr_cost = 0\n for i in range(len(s)):\n if s[i] not in char_dict:\n curr_cost += ord(s[i]) - 96\n else:\n curr_cost += char_dict[s[i]]\n \n if curr_cost < 0:\n curr_cost = 0\n if curr_cost > max_cost:\n max_cost = curr_cost\n \n return max_cost\n\n \n```\n# Humble Request\n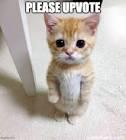\n\n\n | 8 | You are given a string `s`, a string `chars` of **distinct** characters and an integer array `vals` of the same length as `chars`.
The **cost of the substring** is the sum of the values of each character in the substring. The cost of an empty string is considered `0`.
The **value of the character** is defined in the following way:
* If the character is not in the string `chars`, then its value is its corresponding position **(1-indexed)** in the alphabet.
* For example, the value of `'a'` is `1`, the value of `'b'` is `2`, and so on. The value of `'z'` is `26`.
* Otherwise, assuming `i` is the index where the character occurs in the string `chars`, then its value is `vals[i]`.
Return _the maximum cost among all substrings of the string_ `s`.
**Example 1:**
**Input:** s = "adaa ", chars = "d ", vals = \[-1000\]
**Output:** 2
**Explanation:** The value of the characters "a " and "d " is 1 and -1000 respectively.
The substring with the maximum cost is "aa " and its cost is 1 + 1 = 2.
It can be proven that 2 is the maximum cost.
**Example 2:**
**Input:** s = "abc ", chars = "abc ", vals = \[-1,-1,-1\]
**Output:** 0
**Explanation:** The value of the characters "a ", "b " and "c " is -1, -1, and -1 respectively.
The substring with the maximum cost is the empty substring " " and its cost is 0.
It can be proven that 0 is the maximum cost.
**Constraints:**
* `1 <= s.length <= 105`
* `s` consist of lowercase English letters.
* `1 <= chars.length <= 26`
* `chars` consist of **distinct** lowercase English letters.
* `vals.length == chars.length`
* `-1000 <= vals[i] <= 1000` | null |
[Java/Python 3] Kadane Algorithm. | find-the-substring-with-maximum-cost | 1 | 1 | \n\n```java\n public int maximumCostSubstring(String s, String chars, int[] vals) {\n Map<Character, Integer> charToVal = new HashMap<>();\n for (int i = 0; i < vals.length; ++i) {\n charToVal.put(chars.charAt(i), vals[i]);\n }\n int maxCost = 0;\n for (int i = 0, maxCostSubstringEndingAtCur = 0; i < s.length(); ++i) {\n char c = s.charAt(i);\n int v = charToVal.getOrDefault(c, c - \'a\' + 1);\n maxCostSubstringEndingAtCur = Math.max(maxCostSubstringEndingAtCur + v, v);\n maxCost = Math.max(maxCost, maxCostSubstringEndingAtCur);\n }\n return maxCost;\n }\n```\n```python\n def maximumCostSubstring(self, s: str, chars: str, vals: List[int]) -> int:\n seen, max_cost = {c : v for c, v in zip(chars, vals)}, 0\n max_cost_substring_ending_at_cur = 0\n for c in s:\n v = seen.get(c, ord(c) - ord(\'a\') + 1)\n max_cost_substring_ending_at_cur = max(max_cost_substring_ending_at_cur + v, v)\n max_cost = max(max_cost, max_cost_substring_ending_at_cur)\n return max_cost \n```\n\n**Analysis:**\n\nTime: `O(m + n)`, space: `O(m)`, where `m = vals.length, n = s.length()`. | 8 | You are given a string `s`, a string `chars` of **distinct** characters and an integer array `vals` of the same length as `chars`.
The **cost of the substring** is the sum of the values of each character in the substring. The cost of an empty string is considered `0`.
The **value of the character** is defined in the following way:
* If the character is not in the string `chars`, then its value is its corresponding position **(1-indexed)** in the alphabet.
* For example, the value of `'a'` is `1`, the value of `'b'` is `2`, and so on. The value of `'z'` is `26`.
* Otherwise, assuming `i` is the index where the character occurs in the string `chars`, then its value is `vals[i]`.
Return _the maximum cost among all substrings of the string_ `s`.
**Example 1:**
**Input:** s = "adaa ", chars = "d ", vals = \[-1000\]
**Output:** 2
**Explanation:** The value of the characters "a " and "d " is 1 and -1000 respectively.
The substring with the maximum cost is "aa " and its cost is 1 + 1 = 2.
It can be proven that 2 is the maximum cost.
**Example 2:**
**Input:** s = "abc ", chars = "abc ", vals = \[-1,-1,-1\]
**Output:** 0
**Explanation:** The value of the characters "a ", "b " and "c " is -1, -1, and -1 respectively.
The substring with the maximum cost is the empty substring " " and its cost is 0.
It can be proven that 0 is the maximum cost.
**Constraints:**
* `1 <= s.length <= 105`
* `s` consist of lowercase English letters.
* `1 <= chars.length <= 26`
* `chars` consist of **distinct** lowercase English letters.
* `vals.length == chars.length`
* `-1000 <= vals[i] <= 1000` | null |
Python 3 || 4 lines, w/comments || T/M: 51% / 96% | make-k-subarray-sums-equal | 0 | 1 | ```\nclass Solution:\n def makeSubKSumEqual(self, arr: List[int], k: int) -> int:\n\n g, d, ans = gcd(k,len(arr)), defaultdict(list), 0\n\n for i, a in enumerate(arr): d[i%g].append(a) # <-- construct a dict of sorted lists\n for i in d:d[i].sort() # of`arr`elements that must be equal\n \n return sum(d[i][-j-1]-d[i][j] # <-- determine the number of moves to \n for j in range((len(d[i]))//2) for i in d) # make them equal. The sum of moves \n # for a symmetric pair (e.g., d[i][1]\n # and d[i][-2]) is their difference.\n```\n[https://leetcode.com/problems/make-k-subarray-sums-equal/submissions/926808484/](http://)\n\nI could be wrong, but I think that time complexity is *O*(*N*log*N*) and space complexity is *O*(*N*).\n | 3 | You are given a **0-indexed** integer array `arr` and an integer `k`. The array `arr` is circular. In other words, the first element of the array is the next element of the last element, and the last element of the array is the previous element of the first element.
You can do the following operation any number of times:
* Pick any element from `arr` and increase or decrease it by `1`.
Return _the minimum number of operations such that the sum of each **subarray** of length_ `k` _is equal_.
A **subarray** is a contiguous part of the array.
**Example 1:**
**Input:** arr = \[1,4,1,3\], k = 2
**Output:** 1
**Explanation:** we can do one operation on index 1 to make its value equal to 3.
The array after the operation is \[1,3,1,3\]
- Subarray starts at index 0 is \[1, 3\], and its sum is 4
- Subarray starts at index 1 is \[3, 1\], and its sum is 4
- Subarray starts at index 2 is \[1, 3\], and its sum is 4
- Subarray starts at index 3 is \[3, 1\], and its sum is 4
**Example 2:**
**Input:** arr = \[2,5,5,7\], k = 3
**Output:** 5
**Explanation:** we can do three operations on index 0 to make its value equal to 5 and two operations on index 3 to make its value equal to 5.
The array after the operations is \[5,5,5,5\]
- Subarray starts at index 0 is \[5, 5, 5\], and its sum is 15
- Subarray starts at index 1 is \[5, 5, 5\], and its sum is 15
- Subarray starts at index 2 is \[5, 5, 5\], and its sum is 15
- Subarray starts at index 3 is \[5, 5, 5\], and its sum is 15
**Constraints:**
* `1 <= k <= arr.length <= 105`
* `1 <= arr[i] <= 109` | null |
[Python 3] median and mod GCD | make-k-subarray-sums-equal | 0 | 1 | \n# Code\n```\nclass Solution:\n def makeSubKSumEqual(self, A: List[int], K: int) -> int:\n lA = len(A)\n g = gcd(lA, K)\n retV = 0\n for i in range(g):\n med = int(median(A[i::g]))\n retV += sum(abs(a-med) for a in A[i::g])\n \n return retV \n``` | 2 | You are given a **0-indexed** integer array `arr` and an integer `k`. The array `arr` is circular. In other words, the first element of the array is the next element of the last element, and the last element of the array is the previous element of the first element.
You can do the following operation any number of times:
* Pick any element from `arr` and increase or decrease it by `1`.
Return _the minimum number of operations such that the sum of each **subarray** of length_ `k` _is equal_.
A **subarray** is a contiguous part of the array.
**Example 1:**
**Input:** arr = \[1,4,1,3\], k = 2
**Output:** 1
**Explanation:** we can do one operation on index 1 to make its value equal to 3.
The array after the operation is \[1,3,1,3\]
- Subarray starts at index 0 is \[1, 3\], and its sum is 4
- Subarray starts at index 1 is \[3, 1\], and its sum is 4
- Subarray starts at index 2 is \[1, 3\], and its sum is 4
- Subarray starts at index 3 is \[3, 1\], and its sum is 4
**Example 2:**
**Input:** arr = \[2,5,5,7\], k = 3
**Output:** 5
**Explanation:** we can do three operations on index 0 to make its value equal to 5 and two operations on index 3 to make its value equal to 5.
The array after the operations is \[5,5,5,5\]
- Subarray starts at index 0 is \[5, 5, 5\], and its sum is 15
- Subarray starts at index 1 is \[5, 5, 5\], and its sum is 15
- Subarray starts at index 2 is \[5, 5, 5\], and its sum is 15
- Subarray starts at index 3 is \[5, 5, 5\], and its sum is 15
**Constraints:**
* `1 <= k <= arr.length <= 105`
* `1 <= arr[i] <= 109` | null |
python3 Solution | make-k-subarray-sums-equal | 0 | 1 | \n```\nclass Solution:\n def makeSubKSumEqual(self, arr: List[int], k: int) -> int:\n n = len(arr)\n g = gcd(n, k)\n ret = 0\n for i in range(g):\n med = int(median(arr[i::g]))\n ret += sum(abs(a-med) for a in arr[i::g])\n \n return ret\n``` | 1 | You are given a **0-indexed** integer array `arr` and an integer `k`. The array `arr` is circular. In other words, the first element of the array is the next element of the last element, and the last element of the array is the previous element of the first element.
You can do the following operation any number of times:
* Pick any element from `arr` and increase or decrease it by `1`.
Return _the minimum number of operations such that the sum of each **subarray** of length_ `k` _is equal_.
A **subarray** is a contiguous part of the array.
**Example 1:**
**Input:** arr = \[1,4,1,3\], k = 2
**Output:** 1
**Explanation:** we can do one operation on index 1 to make its value equal to 3.
The array after the operation is \[1,3,1,3\]
- Subarray starts at index 0 is \[1, 3\], and its sum is 4
- Subarray starts at index 1 is \[3, 1\], and its sum is 4
- Subarray starts at index 2 is \[1, 3\], and its sum is 4
- Subarray starts at index 3 is \[3, 1\], and its sum is 4
**Example 2:**
**Input:** arr = \[2,5,5,7\], k = 3
**Output:** 5
**Explanation:** we can do three operations on index 0 to make its value equal to 5 and two operations on index 3 to make its value equal to 5.
The array after the operations is \[5,5,5,5\]
- Subarray starts at index 0 is \[5, 5, 5\], and its sum is 15
- Subarray starts at index 1 is \[5, 5, 5\], and its sum is 15
- Subarray starts at index 2 is \[5, 5, 5\], and its sum is 15
- Subarray starts at index 3 is \[5, 5, 5\], and its sum is 15
**Constraints:**
* `1 <= k <= arr.length <= 105`
* `1 <= arr[i] <= 109` | null |
[Python 3] Disjoin Set | make-k-subarray-sums-equal | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass ufds:\n parent_node = {}\n rank = {}\n\n def make_set(self, u):\n for i in u:\n self.parent_node[i] = i\n self.rank[i] = 0\n\n def op_find(self, k):\n if self.parent_node[k] != k:\n self.parent_node[k] = self.op_find(self.parent_node[k])\n return self.parent_node[k]\n\n def op_union(self, a, b):\n x = self.op_find(a)\n y = self.op_find(b)\n \n if x == y:\n return\n if self.rank[x] > self.rank[y]:\n self.parent_node[y] = x\n elif self.rank[x] < self.rank[y]:\n self.parent_node[x] = y\n else:\n self.parent_node[x] = y\n self.rank[y] = self.rank[y] + 1\n\nclass Solution:\n def makeSubKSumEqual(self, arr: List[int], k: int) -> int:\n def find_min_abs_sum(arr):\n n = len(arr)\n arr.sort()\n middle = arr[n//2]\n curr = 0\n for i in arr:\n curr += abs(i-middle)\n return curr\n \n if k==1:\n return find_min_abs_sum(arr)\n\n uds = ufds()\n n = len(arr)\n uds.make_set(list(range(n)))\n\n for i in range(n):\n uds.op_union(i, (i + k) % n)\n\n dd = defaultdict(list)\n for i in range(n):\n dd[uds.op_find(i)].append(arr[i])\n \n res = 0\n for key in dd:\n temarr = dd[key]\n res += find_min_abs_sum(temarr)\n return res\n``` | 1 | You are given a **0-indexed** integer array `arr` and an integer `k`. The array `arr` is circular. In other words, the first element of the array is the next element of the last element, and the last element of the array is the previous element of the first element.
You can do the following operation any number of times:
* Pick any element from `arr` and increase or decrease it by `1`.
Return _the minimum number of operations such that the sum of each **subarray** of length_ `k` _is equal_.
A **subarray** is a contiguous part of the array.
**Example 1:**
**Input:** arr = \[1,4,1,3\], k = 2
**Output:** 1
**Explanation:** we can do one operation on index 1 to make its value equal to 3.
The array after the operation is \[1,3,1,3\]
- Subarray starts at index 0 is \[1, 3\], and its sum is 4
- Subarray starts at index 1 is \[3, 1\], and its sum is 4
- Subarray starts at index 2 is \[1, 3\], and its sum is 4
- Subarray starts at index 3 is \[3, 1\], and its sum is 4
**Example 2:**
**Input:** arr = \[2,5,5,7\], k = 3
**Output:** 5
**Explanation:** we can do three operations on index 0 to make its value equal to 5 and two operations on index 3 to make its value equal to 5.
The array after the operations is \[5,5,5,5\]
- Subarray starts at index 0 is \[5, 5, 5\], and its sum is 15
- Subarray starts at index 1 is \[5, 5, 5\], and its sum is 15
- Subarray starts at index 2 is \[5, 5, 5\], and its sum is 15
- Subarray starts at index 3 is \[5, 5, 5\], and its sum is 15
**Constraints:**
* `1 <= k <= arr.length <= 105`
* `1 <= arr[i] <= 109` | null |
Python || greatest common divisor || O(n log n) | make-k-subarray-sums-equal | 0 | 1 | 1. Consider the example:\n\n`arr[0] + arr[1] + arr[2] + arr[3] = arr[1] + arr[2] + arr[3] + arr[4]`, so `arr[0] = arr[4]`. \nBy similar calculation we have `arr[0] = arr[4] = arr[2]`, `arr[1] = arr[5] = arr[3]`.\n2. As above, `arr` can be divided into subarrays of equal length, and the length would be `g := gcd(len(arr), k)`.\n3. All subarrays would look the same after we finish operations, i.e. `arr[i] = arr[i+g] = arr[i+2*g] = ... for all i`.\n4. Now the problem becomes: given some integers `i_0, i_1, i_2, ..., i_n`, what `x` would make the sum `abs(i_0 - x) + abs(i_1 - x) + abs(i_2 - x) + ... + abs(i_n - x)` smallest?\n`x = median(i_0, i_1, i_2, ..., i_n)` would do.\n```\nclass Solution:\n def makeSubKSumEqual(self, arr: List[int], k: int) -> int:\n gcd = math.gcd(len(arr), k)\n \n ans = 0\n for i in range(gcd):\n seq = arr[i::gcd]\n m = int(statistics.median(seq))\n for n in seq:\n ans += abs(n-m)\n return ans | 1 | You are given a **0-indexed** integer array `arr` and an integer `k`. The array `arr` is circular. In other words, the first element of the array is the next element of the last element, and the last element of the array is the previous element of the first element.
You can do the following operation any number of times:
* Pick any element from `arr` and increase or decrease it by `1`.
Return _the minimum number of operations such that the sum of each **subarray** of length_ `k` _is equal_.
A **subarray** is a contiguous part of the array.
**Example 1:**
**Input:** arr = \[1,4,1,3\], k = 2
**Output:** 1
**Explanation:** we can do one operation on index 1 to make its value equal to 3.
The array after the operation is \[1,3,1,3\]
- Subarray starts at index 0 is \[1, 3\], and its sum is 4
- Subarray starts at index 1 is \[3, 1\], and its sum is 4
- Subarray starts at index 2 is \[1, 3\], and its sum is 4
- Subarray starts at index 3 is \[3, 1\], and its sum is 4
**Example 2:**
**Input:** arr = \[2,5,5,7\], k = 3
**Output:** 5
**Explanation:** we can do three operations on index 0 to make its value equal to 5 and two operations on index 3 to make its value equal to 5.
The array after the operations is \[5,5,5,5\]
- Subarray starts at index 0 is \[5, 5, 5\], and its sum is 15
- Subarray starts at index 1 is \[5, 5, 5\], and its sum is 15
- Subarray starts at index 2 is \[5, 5, 5\], and its sum is 15
- Subarray starts at index 3 is \[5, 5, 5\], and its sum is 15
**Constraints:**
* `1 <= k <= arr.length <= 105`
* `1 <= arr[i] <= 109` | null |
✔💯DAY 366 || [PYTHON/JAVA/C++] || Explained || Intuition || Algorithm || 100% beats || o(n) & o(1) | make-k-subarray-sums-equal | 1 | 1 | # Please Upvote as it really motivates me\n\n##### \u2022\tThe problem statement is to make the sum of all subarrays of length k equal changing the elements of the array. The approach used in this problem is to group the elements of the array into k subarrays based on their remainder when divided by k. Then, for each subarray, the median value is calculated and the absolute difference between each element in the subarray and the median is added to the total answer.\n\n##### \u2022\tHere is the step-by-step algorithm:\n##### \u2022\tInitialize a list of k subarrays.\n##### \u2022\tCalculate the greatest common divisor of n and k, where n is the length of the input array.\n##### \u2022\tGroup the elements of the input array into k subarrays based on their remainder when divided by k.\n##### \u2022\tFor each subarray, sort the elements and calculate the median value.\n##### \u2022\tFor each element in the subarray, calculate the absolute difference between the element and the median value, and add it to the total answer.\n##### \u2022\tReturn the total answer.\n\n##### \u2022\tThe intuition behind this problem is that by grouping the elements into subarrays based on their remainder when divided by k, we can ensure that each subarray has the same number of elements. Then, by calculating the median value of each subarray, we can find a representative value that is close to the center of the subarray. By minimizing the absolute difference between each element and the median value, we can make the sum of all subarrays of length k equal.\n\n\n\n# Code\n```python []\nclass Solution:\n def makeSubKSumEqual(self,arr, k):\n n = len(arr)\n v = [[] for i in range(n + 1)]\n k = gcd(n, k)\n for i in range(n):\n v[i % k].append(arr[i])\n ans = 0\n for i in range(k):\n v[i].sort()\n x = v[i][len(v[i]) // 2]\n for j in v[i]:\n ans += abs(x - j)\n return ans\n\n def gcd(a, b):\n if b == 0:\n return a\n return gcd(b, a % b)\n```\n\n```java []\nclass Solution{\n public static long makeSubKSumEqual(int[] arr, int k) {\n int n = arr.length;\n List<Integer>[] v = new ArrayList[n + 1];\n for (int i = 0; i <= n; i++) {\n v[i] = new ArrayList<>();\n }\n k = gcd(n, k);\n for (int i = 0; i < n; i++) {\n v[i % k].add(arr[i]);\n }\n long ans = 0;\n for (int i = 0; i < k; i++) {\n Collections.sort(v[i]);\n int x = v[i].get(v[i].size() / 2);\n for (int j : v[i]) {\n ans += Math.abs(x - j);\n }\n }\n return ans;\n}\n\nprivate static int gcd(int a, int b) {\n if (b == 0) {\n return a;\n }\n return gcd(b, a % b);\n}\n}\n```\n```C++ []\nclass Solution {\npublic:\n long long makeSubKSumEqual(vector<int>& arr, int k) {\n int n = arr.size() ;\n vector<int>v[n+1] ;\n k = __gcd(n , k) ;\n for(int i = 0 ; i < n ; ++i)\n v[i%k].push_back(arr[i]) ;\n long long ans = 0 ;\n for(int i = 0 ; i < k ; ++i)\n {\n sort(v[i].begin() , v[i].end()) ;\n int x = v[i][v[i].size() / 2] ;\n for(auto &j : v[i])\n ans += abs(x-j) ;\n }\n return ans ;\n }\n};\n```\n\n\n\n# Complexity\n\n##### \u2022\tThe time complexity (TC) of this algorithm is O(n log n), where n is the length of the input array. This is because the algorithm involves sorting each subarray, which takes O(k log k) time, where k is the size of each subarray. Since there are n/k subarrays, the total time complexity is O(n log k). However, since k is the greatest common divisor of n and k, it is at most n/2. Therefore, the time complexity can be simplified to O(n log n).\n\n##### \u2022\tThe space complexity (SC) of this algorithm is O(n), where n is the length of the input array. This is because the algorithm creates a list of k subarrays, each of which can contain up to n/k elements. Therefore, the total space complexity is O(n).\n\n# 2ND WAY\n```C++ []\nclass Solution {\npublic:\n long long makeSubKSumEqual(vector<int>& arr, int k) {\n int n = arr.size();\n vector<int> v[n+1];\n k = __gcd(n, k);\n for (int i = 0; i < n; ++i) {\n v[i%k].push_back(arr[i]);\n }\n long long ans = 0;\n for (int i = 0; i < k; ++i) {\n int median = quickselect(v[i], v[i].size() / 2);\n for (auto &j : v[i]) {\n ans += abs(median - j);\n }\n }\n return ans;\n }\n\n int quickselect(vector<int>& nums, int k) {\n int left = 0, right = nums.size() - 1;\n while (left <= right) {\n int pivotIndex = partition(nums, left, right);\n if (pivotIndex == k) {\n return nums[k];\n } else if (pivotIndex < k) {\n left = pivotIndex + 1;\n } else {\n right = pivotIndex - 1;\n }\n }\n return -1;\n }\n\n int partition(vector<int>& nums, int left, int right) {\n int pivotIndex = left + (right - left) / 2;\n int pivotValue = nums[pivotIndex];\n swap(nums[pivotIndex], nums[right]);\n int storeIndex = left;\n for (int i = left; i < right; i++) {\n if (nums[i] < pivotValue) {\n swap(nums[i], nums[storeIndex]);\n storeIndex++;\n }\n }\n swap(nums[storeIndex], nums[right]);\n return storeIndex;\n }\n};\n```\n```java []\nclass Solution {\n public longSubKSumEqual(int[] arr, int k) {\n int n = arr.length;\n List<Integer>[] v = new List[n+1];\n for (int i = 0; i <= n; i++) {\n v[i] = new ArrayList<>();\n }\n k = gcd(n, k);\n for (int i = 0; i < n; i++) {\n v[i%k].add(arr[i]);\n }\n long ans = 0;\n for (int i = 0; i < k; i++) {\n int median = quickselect(v[i], v[i].size() / 2);\n for (int j : v[i]) {\n ans += Math.abs(median - j);\n }\n }\n return ans;\n }\n\n private int quickselect(List<Integer> nums, int k) {\n int left = 0, right = nums.size() - 1;\n while (left <= right) {\n int pivotIndex = partition(nums, left, right);\n if (pivotIndex == k) {\n return nums.get(k);\n } else if (pivotIndex < k) {\n left = pivotIndex + 1;\n } else {\n right = pivotIndex - 1;\n }\n }\n return -1;\n }\n\n private int partition(List<Integer> nums, int left, int right) {\n int pivotIndex = left + (right - left) / 2;\n int pivotValue = nums.get(pivotIndex);\n Collections.swap(nums, pivotIndex, right);\n int storeIndex = left;\n for (int i = left; i < right; i++) {\n if (nums.get(i) < pivotValue) {\n Collections.swap(nums, i, storeIndex);\n storeIndex++;\n }\n }\n Collections.swap(nums, storeIndex, right);\n return storeIndex;\n }\n\n private int gcd(int a, int b) {\n if (b == 0) {\n return a;\n }\n return gcd(b, a % b);\n }\n}\n```\n```python []\nclass Solution:\n def makeSubKSumEqual(self, arr: List[int], k: int) -> int:\n n = len(arr)\n v = [[] for i in range(n + 1)]\n k = gcd(n, k)\n for i in range(n):\n v[i % k].append(arr[i])\n ans = 0\n for i in range(k):\n median = self.quickselect(v[i], len(v[i]) // 2)\n for j in v[i]:\n ans += abs(median - j)\n return ans\n\n def quickselect(self, nums: List[int], k: int) -> int:\n left, right = 0, len(nums) - 1\n while left <= right:\n pivotIndex = self.partition(nums, left, right)\n if pivotIndex == k:\n return nums[k]\n elif pivotIndex < k:\n left = pivotIndex + 1\n else:\n right = pivotIndex - 1\n return -1\n\n def partition(self, nums: List[int], left: int, right: int) -> int:\n pivotIndex = left + (right - left) // 2\n pivotValue = nums[pivotIndex]\n nums[pivotIndex], nums[right] = nums[right], nums[pivotIndex]\n storeIndex = left\n for i in range(left, right):\n if nums[i] < pivotValue:\n nums[i], nums[storeIndex] = nums[storeIndex], nums[i]\n storeIndex += 1\n nums[storeIndex], nums[right] = nums[right], nums[storeIndex]\n return storeIndex\n\n def gcd(self, a: int, b: int) -> int:\n if b == 0:\n return a\n return self.gcd(b, a % b)\n```\nThe time complexity of the optimized makeSubKSumEqual function is O log n), where n is the length of the input array arr . This is because the function performs a quickselect operation on each subarray, which has an average time complexity of O(n), and then iterates through each subarray to calculate the absolute difference between each element and the median. The sorting operation in the original code has been replaced by the quickselect operation, which is more efficient for finding the median.\n\nThe space complexity of the function is O(n), which is the space required to store the subarrays in the \nv\n vector. The quickselect algorithm has a space complexity of O(1) because it operates on the input array in place.\n\nOverall, the optimized solution has a better time complexity than the original solution, but the space complexity remains the same.\n\n# Please Upvote\uD83D\uDC4D\uD83D\uDC4D\n\n##### \u2022 Thanks for visiting my solution.\uD83D\uDE0A Keep Learning\n##### \u2022 Please give my solution an upvote! \uD83D\uDC4D\n##### \u2022 It\'s a simple way to show your appreciation and\n\nkeep me motivated. Thank you! \uD83D\uDE0A\n[My post here ](https://leetcode.com/problems/make-k-subarray-sums-equal/solutions/3366625/day-366-python-java-c-explained-intuition-algorithm-100-beats/?orderBy=hot) \n\n | 8 | You are given a **0-indexed** integer array `arr` and an integer `k`. The array `arr` is circular. In other words, the first element of the array is the next element of the last element, and the last element of the array is the previous element of the first element.
You can do the following operation any number of times:
* Pick any element from `arr` and increase or decrease it by `1`.
Return _the minimum number of operations such that the sum of each **subarray** of length_ `k` _is equal_.
A **subarray** is a contiguous part of the array.
**Example 1:**
**Input:** arr = \[1,4,1,3\], k = 2
**Output:** 1
**Explanation:** we can do one operation on index 1 to make its value equal to 3.
The array after the operation is \[1,3,1,3\]
- Subarray starts at index 0 is \[1, 3\], and its sum is 4
- Subarray starts at index 1 is \[3, 1\], and its sum is 4
- Subarray starts at index 2 is \[1, 3\], and its sum is 4
- Subarray starts at index 3 is \[3, 1\], and its sum is 4
**Example 2:**
**Input:** arr = \[2,5,5,7\], k = 3
**Output:** 5
**Explanation:** we can do three operations on index 0 to make its value equal to 5 and two operations on index 3 to make its value equal to 5.
The array after the operations is \[5,5,5,5\]
- Subarray starts at index 0 is \[5, 5, 5\], and its sum is 15
- Subarray starts at index 1 is \[5, 5, 5\], and its sum is 15
- Subarray starts at index 2 is \[5, 5, 5\], and its sum is 15
- Subarray starts at index 3 is \[5, 5, 5\], and its sum is 15
**Constraints:**
* `1 <= k <= arr.length <= 105`
* `1 <= arr[i] <= 109` | null |
[Python3] Find Median of Each GCD-Defined Subarray (w/ Examples) | make-k-subarray-sums-equal | 0 | 1 | # First Thought\nAny na\xEFve checking algorithm would not work here as both `k` and `n (= arr.length)` could be up to $$10^5$$.\n\nSo, how can we come up with a better algorithm with improved time complexity?\n\n# Key Intuition\nLet\u2019s first look at a toy example by assuming `k = 3`. Because the problem requires the sum of each subarray of length `k` to be equal, we then need to have `arr[0] + arr[1] + arr[2] = arr[1] + arr[2] + arr[3]`, which implies that `arr[0] = arr[3]`. Essentially, this is saying that every `k`-th element of `arr` needs to be equal after all operations.\n\nThere is one caveat yet to make the argument complete: In this problem `arr` is not only a regular array but also a circular array. Suppose `n = 6` and `k = 4`, we need to have every `gcd(n, k) = gcd(6, 4) = 2`-th element of `arr` to be equal after all operations.\n\n# Representative Examples\n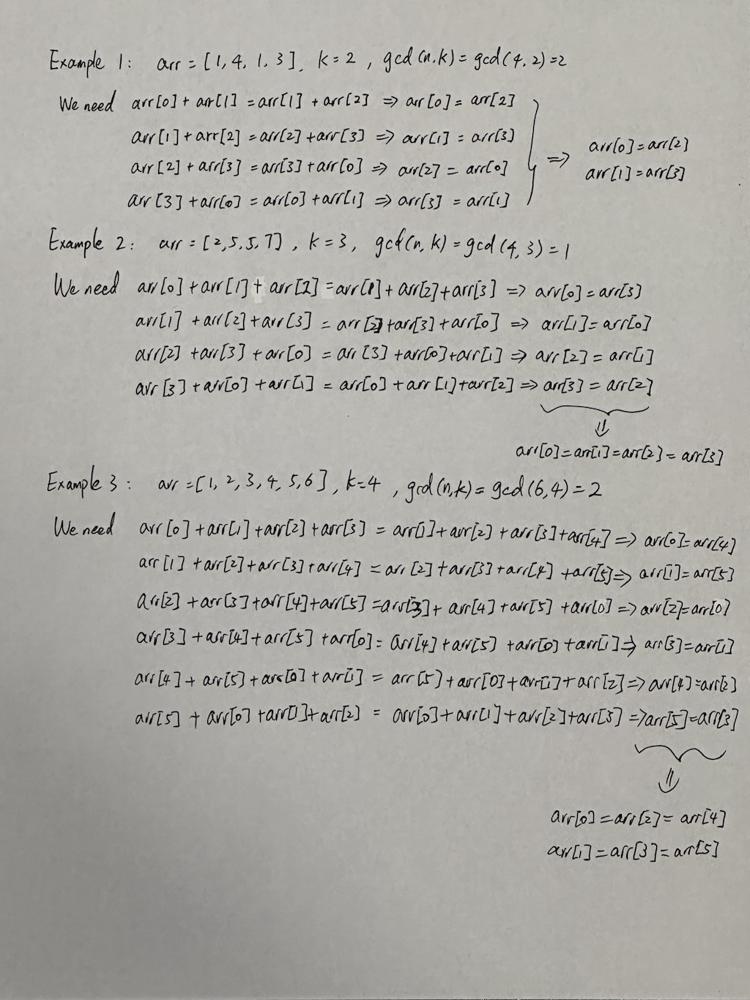\n\n# Approach\nFirst, we compute the greatest common divisor of `n` and `k`, denoted as `gcd`. For every `gcd`-th element of `arr`, we store it in a temporary array `tmp`, such that the problem reduces to finding the minimum number operations to make all elements in each of `tmp` equal.\n\nFor this sub-problem, we can sort the subarray `tmp` and find its median, which contributes to the final `ans` after operations.\n\n# Complexity\n- Time complexity: $$O(nlogn)$$, could be improved to $$O(n)$$ on average;\n\n- Space complexity: $$O(n)$$.\n\n**Please upvote if you find this solution helpful. Thanks!**\n\n# Code\n```\nclass Solution:\n def makeSubKSumEqual(self, arr: List[int], k: int) -> int:\n n = len(arr)\n gcd = math.gcd(n, k)\n ans = 0\n for i in range(gcd):\n tmp = sorted([arr[j] for j in range(i, n, gcd)])\n median = tmp[len(tmp) // 2]\n ans += sum(abs(num - median) for num in tmp)\n return ans\n```\n\n# Follow-up (Bonus)\nWe can potentially improve the average time complexity by applying quick-select to each of the subarray problem in finding the median. | 62 | You are given a **0-indexed** integer array `arr` and an integer `k`. The array `arr` is circular. In other words, the first element of the array is the next element of the last element, and the last element of the array is the previous element of the first element.
You can do the following operation any number of times:
* Pick any element from `arr` and increase or decrease it by `1`.
Return _the minimum number of operations such that the sum of each **subarray** of length_ `k` _is equal_.
A **subarray** is a contiguous part of the array.
**Example 1:**
**Input:** arr = \[1,4,1,3\], k = 2
**Output:** 1
**Explanation:** we can do one operation on index 1 to make its value equal to 3.
The array after the operation is \[1,3,1,3\]
- Subarray starts at index 0 is \[1, 3\], and its sum is 4
- Subarray starts at index 1 is \[3, 1\], and its sum is 4
- Subarray starts at index 2 is \[1, 3\], and its sum is 4
- Subarray starts at index 3 is \[3, 1\], and its sum is 4
**Example 2:**
**Input:** arr = \[2,5,5,7\], k = 3
**Output:** 5
**Explanation:** we can do three operations on index 0 to make its value equal to 5 and two operations on index 3 to make its value equal to 5.
The array after the operations is \[5,5,5,5\]
- Subarray starts at index 0 is \[5, 5, 5\], and its sum is 15
- Subarray starts at index 1 is \[5, 5, 5\], and its sum is 15
- Subarray starts at index 2 is \[5, 5, 5\], and its sum is 15
- Subarray starts at index 3 is \[5, 5, 5\], and its sum is 15
**Constraints:**
* `1 <= k <= arr.length <= 105`
* `1 <= arr[i] <= 109` | null |
Python | Cycle Partition | O(nlogn) | make-k-subarray-sums-equal | 0 | 1 | # Code\n```\nclass Solution:\n def makeSubKSumEqual(self, arr: List[int], k: int) -> int:\n visited = set()\n n = len(arr)\n res = 0\n for i in range(len(arr)):\n if i in visited: continue\n curlist = []\n while i not in visited:\n visited.add(i)\n curlist.append(arr[i])\n i = (i+k)%n\n curlist.sort()\n m = len(curlist)//2\n res += sum([abs(num - curlist[m]) for num in curlist])\n return res\n \n``` | 4 | You are given a **0-indexed** integer array `arr` and an integer `k`. The array `arr` is circular. In other words, the first element of the array is the next element of the last element, and the last element of the array is the previous element of the first element.
You can do the following operation any number of times:
* Pick any element from `arr` and increase or decrease it by `1`.
Return _the minimum number of operations such that the sum of each **subarray** of length_ `k` _is equal_.
A **subarray** is a contiguous part of the array.
**Example 1:**
**Input:** arr = \[1,4,1,3\], k = 2
**Output:** 1
**Explanation:** we can do one operation on index 1 to make its value equal to 3.
The array after the operation is \[1,3,1,3\]
- Subarray starts at index 0 is \[1, 3\], and its sum is 4
- Subarray starts at index 1 is \[3, 1\], and its sum is 4
- Subarray starts at index 2 is \[1, 3\], and its sum is 4
- Subarray starts at index 3 is \[3, 1\], and its sum is 4
**Example 2:**
**Input:** arr = \[2,5,5,7\], k = 3
**Output:** 5
**Explanation:** we can do three operations on index 0 to make its value equal to 5 and two operations on index 3 to make its value equal to 5.
The array after the operations is \[5,5,5,5\]
- Subarray starts at index 0 is \[5, 5, 5\], and its sum is 15
- Subarray starts at index 1 is \[5, 5, 5\], and its sum is 15
- Subarray starts at index 2 is \[5, 5, 5\], and its sum is 15
- Subarray starts at index 3 is \[5, 5, 5\], and its sum is 15
**Constraints:**
* `1 <= k <= arr.length <= 105`
* `1 <= arr[i] <= 109` | null |
🧒🏻 Explaining like you are five years old !! | make-k-subarray-sums-equal | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n[1,4,1,3]\n\nIt is a Circular Array, What are the possible subarray sums \n```\na. 1 + 4 = 5\nb. 4 + 1 = 5\nc. 1 + 3 = 4\nd. 3 + 1 = 4\n```\n\nFrom above you can understand the num which goes in should be equal to number which goes out.\n\n1 should be equal to 1\n3 should be equal to 4\n\nIn case b, 1 (index = 2) gets in the window and 1 (index = 0) gets out which are same.\nIn case c, 3 (index = 3) gets in the window and 4 (index = 1) gets out which are not same.\n\n\nHence we could have grouped them [1, 1] [3, 4] in these buckets and same they should be same to make subarray equal.\n\n**suppose k is 2**\n\n\nWe need to know as array is circular, when we complete run in next run the start index will be different or not if yes what are the element which should be equal - That\'s where GCD comes in.\n\n\n```\n [1, 4, 1, 3]\nstep 1 ^ ^ \n\n```\n\n\n`^` these are pointers now if the array is circular and you take the pointer skipping one element the pointers will end again on 1 and 1, \n\ngcd (4, 2) = 2 which means there will be 2 buckets and every number will be put in after skipping one.\n\nbucket 1 = [1, 1]\nbucket 2 = [3, 4]\n\n\n**suppose k is 3**\n```\n [2, 5, 5, 7]\nstep 1 ^ ^\nstep 2 ^ ^\n```\nThese where we use gcd to find buckets gcd (4, 3) = 1 which means there will be one bucket and every other number will be in it\n\nbucket 1 = [2, 5, 5, 7]\n\n\nNow the simplest task making each bucket equal\n- The optimal way to do this is use gready approach and use median to find minimum operations to make numbers equal\n\n#### For example 1\n\n\nmedian = sorted([4, 3]) = [3, 4] = 4\nget absolute difference from all numbers in array which will be one (3 - 4) + (4 - 4) = 1\n\n**You have to do same operation on all buckets but elements in bucket 1 are same it will just give 0**\n\n#### For example 2\n\nmedian = sorted([2, 5, 5, 7]) = [2, 5, 5, 7] = 5\nget absolute difference from all numbers in array which will be one (2 - 5) + (5 - 5) + (5 - 5) + (7 - 5) = 5\n\n\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n`O(N) + O(K * KlogK)`\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n`O(N)`\n\n# Code\n```\nclass Solution:\n def makeSubKSumEqual(self, arr: List[int], k: int) -> int:\n \n n = len(arr)\n\n no_of_buckets = gcd (k, n)\n buckets = defaultdict(list)\n\n for ind, num in enumerate(arr):\n buckets[ind % no_of_buckets].append(num)\n\n result = 0\n for bucket_number, bucket in buckets.items():\n sorted_bucket = sorted(bucket)\n m = len(sorted_bucket)\n median = sorted_bucket[(m)//2]\n \n for num in bucket:\n result += abs(median - num)\n\n return result\n\n``` | 29 | You are given a **0-indexed** integer array `arr` and an integer `k`. The array `arr` is circular. In other words, the first element of the array is the next element of the last element, and the last element of the array is the previous element of the first element.
You can do the following operation any number of times:
* Pick any element from `arr` and increase or decrease it by `1`.
Return _the minimum number of operations such that the sum of each **subarray** of length_ `k` _is equal_.
A **subarray** is a contiguous part of the array.
**Example 1:**
**Input:** arr = \[1,4,1,3\], k = 2
**Output:** 1
**Explanation:** we can do one operation on index 1 to make its value equal to 3.
The array after the operation is \[1,3,1,3\]
- Subarray starts at index 0 is \[1, 3\], and its sum is 4
- Subarray starts at index 1 is \[3, 1\], and its sum is 4
- Subarray starts at index 2 is \[1, 3\], and its sum is 4
- Subarray starts at index 3 is \[3, 1\], and its sum is 4
**Example 2:**
**Input:** arr = \[2,5,5,7\], k = 3
**Output:** 5
**Explanation:** we can do three operations on index 0 to make its value equal to 5 and two operations on index 3 to make its value equal to 5.
The array after the operations is \[5,5,5,5\]
- Subarray starts at index 0 is \[5, 5, 5\], and its sum is 15
- Subarray starts at index 1 is \[5, 5, 5\], and its sum is 15
- Subarray starts at index 2 is \[5, 5, 5\], and its sum is 15
- Subarray starts at index 3 is \[5, 5, 5\], and its sum is 15
**Constraints:**
* `1 <= k <= arr.length <= 105`
* `1 <= arr[i] <= 109` | null |
[Python3] grouping by gcd | make-k-subarray-sums-equal | 0 | 1 | Please pull this [commit](https://github.com/gaosanyong/leetcode/commit/1dc118daa80cfe1161dcee412e7c3536970ca60d) for solutions of biweely 101. \n\n```\nclass Solution:\n def makeSubKSumEqual(self, arr: List[int], k: int) -> int:\n ans = 0 \n g = gcd(len(arr), k)\n for i in range(g): \n vals = []\n for _ in range(len(arr)//g): \n vals.append(arr[i])\n i = (i+k) % len(arr)\n vals.sort()\n cand = vals[len(vals)//2]\n ans += sum(abs(x-cand) for x in vals)\n return ans \n``` | 3 | You are given a **0-indexed** integer array `arr` and an integer `k`. The array `arr` is circular. In other words, the first element of the array is the next element of the last element, and the last element of the array is the previous element of the first element.
You can do the following operation any number of times:
* Pick any element from `arr` and increase or decrease it by `1`.
Return _the minimum number of operations such that the sum of each **subarray** of length_ `k` _is equal_.
A **subarray** is a contiguous part of the array.
**Example 1:**
**Input:** arr = \[1,4,1,3\], k = 2
**Output:** 1
**Explanation:** we can do one operation on index 1 to make its value equal to 3.
The array after the operation is \[1,3,1,3\]
- Subarray starts at index 0 is \[1, 3\], and its sum is 4
- Subarray starts at index 1 is \[3, 1\], and its sum is 4
- Subarray starts at index 2 is \[1, 3\], and its sum is 4
- Subarray starts at index 3 is \[3, 1\], and its sum is 4
**Example 2:**
**Input:** arr = \[2,5,5,7\], k = 3
**Output:** 5
**Explanation:** we can do three operations on index 0 to make its value equal to 5 and two operations on index 3 to make its value equal to 5.
The array after the operations is \[5,5,5,5\]
- Subarray starts at index 0 is \[5, 5, 5\], and its sum is 15
- Subarray starts at index 1 is \[5, 5, 5\], and its sum is 15
- Subarray starts at index 2 is \[5, 5, 5\], and its sum is 15
- Subarray starts at index 3 is \[5, 5, 5\], and its sum is 15
**Constraints:**
* `1 <= k <= arr.length <= 105`
* `1 <= arr[i] <= 109` | null |
[Java/Python 3] Divide into sequences such that every two consecutive items's distance is gcd.. | make-k-subarray-sums-equal | 1 | 1 | **Intuition:**\nAll `k`-size subarrays are equal => \n`sum(arr[0,...,k- 1]) == sum(arr[1,...,k])` =>\n`arr[0] == arr[k]`\n\nsimilarly, we can conclude that `arr[i] = arr[i + k]`, further more, `arr` is **circular** array, hence we need stronger condition than `arr[i] = arr[i + k]`: only `gcd(k, arr.length)` to guarantee both **circular** and all `k`-size subarrays are equal.\n\n----\n\n1. Compute the `gcd` of `arr.length`and `k`;\n2. The numbers in `arr` must be equal if their indices differenece is the multiple of the aforementioned `gcd`, in order to make all of the k-size subarray sums are equal; Therefore, in `arr` select each item every `gcd` index difference to construct a sequence, and there are totally `arr.length / gcd` such sequences;\n3. In order to make the sequence equal, we need to get each sequence sorted and compute the needed minimum opertations.\n\n```java\n public long makeSubKSumEqual(int[] arr, int k) {\n int gcd = gcd(arr.length, k);\n long minOps = 0;\n for (int i = 0; i < gcd; ++i) {\n minOps += getOperationsCount(arr, i, gcd);\n }\n return minOps;\n }\n private int gcd(int x, int y) {\n while (y != 0) {\n int tmp = x % y;\n x = y;\n y = tmp;\n }\n return x;\n }\n private long getOperationsCount(int[] arr, int start, int gap) {\n int n = arr.length, m = n / gap;\n long min = Long.MAX_VALUE, sum = 0, cur = 0;\n int[] a = new int[m];\n for (int i = start, k = 0; i < n; i += gap, ++k) {\n a[k] = arr[i];\n sum += a[k];\n }\n Arrays.sort(a);\n for (int i = 0; i < m; ++i) {\n min = Math.min(min, (1L * i * a[i] - cur) + ((sum - cur) - 1L * (m - i) * a[i]));\n cur += a[i];\n }\n return min;\n }\n```\n```python\n def makeSubKSumEqual(self, arr: List[int], k: int) -> int:\n \n def getOperationsCount(arr: List[int]) -> int:\n sm, n = sum(arr), len(arr)\n mi = inf\n cur = 0\n for i, num in enumerate(sorted(arr)):\n mi = min(mi, (i * num - cur) + ((sm - cur) - (n - i) * num))\n cur += num\n return mi \n \n gcd = math.gcd(len(arr), k)\n return sum(getOperationsCount(arr[i :: gcd]) for i in range(gcd))\n```\n\nThe above codes can be simplified as follows:\n\n```java\n public long makeSubKSumEqual(int[] arr, int k) {\n int gcd = gcd(arr.length, k);\n long minOps = 0;\n for (int i = 0; i < gcd; ++i) {\n minOps += getOperationsCount(arr, i, gcd);\n }\n return minOps;\n }\n private int gcd(int x, int y) {\n while (y != 0) {\n int tmp = x % y;\n x = y;\n y = tmp;\n }\n return x;\n }\n private long getOperationsCount(int[] arr, int start, int gap) {\n int n = arr.length, m = n / gap;\n long sum = 0;\n int[] a = new int[m];\n for (int i = start, k = 0; i < n; i += gap, ++k) {\n a[k] = arr[i];\n }\n Arrays.sort(a);\n for (int i = 0, mid = m / 2; i < m; ++i) {\n sum += Math.abs(a[i] - a[mid]);\n }\n return sum;\n }\n```\n```python\n def makeSubKSumEqual(self, arr: List[int], k: int) -> int:\n \n def getOperationsCount(arr: List[int]) -> int:\n return sum(abs(a - arr[len(arr) // 2]) for a in arr) \n \n gcd = math.gcd(len(arr), k)\n return sum(getOperationsCount(sorted(arr[i :: gcd])) for i in range(gcd))\n```\n\n**Analysis:**\n\nSorting is the major part of the time cost, therefore\n\nTime: `O(nlogn)`, space: `O(n / gcd(n, k))`. | 11 | You are given a **0-indexed** integer array `arr` and an integer `k`. The array `arr` is circular. In other words, the first element of the array is the next element of the last element, and the last element of the array is the previous element of the first element.
You can do the following operation any number of times:
* Pick any element from `arr` and increase or decrease it by `1`.
Return _the minimum number of operations such that the sum of each **subarray** of length_ `k` _is equal_.
A **subarray** is a contiguous part of the array.
**Example 1:**
**Input:** arr = \[1,4,1,3\], k = 2
**Output:** 1
**Explanation:** we can do one operation on index 1 to make its value equal to 3.
The array after the operation is \[1,3,1,3\]
- Subarray starts at index 0 is \[1, 3\], and its sum is 4
- Subarray starts at index 1 is \[3, 1\], and its sum is 4
- Subarray starts at index 2 is \[1, 3\], and its sum is 4
- Subarray starts at index 3 is \[3, 1\], and its sum is 4
**Example 2:**
**Input:** arr = \[2,5,5,7\], k = 3
**Output:** 5
**Explanation:** we can do three operations on index 0 to make its value equal to 5 and two operations on index 3 to make its value equal to 5.
The array after the operations is \[5,5,5,5\]
- Subarray starts at index 0 is \[5, 5, 5\], and its sum is 15
- Subarray starts at index 1 is \[5, 5, 5\], and its sum is 15
- Subarray starts at index 2 is \[5, 5, 5\], and its sum is 15
- Subarray starts at index 3 is \[5, 5, 5\], and its sum is 15
**Constraints:**
* `1 <= k <= arr.length <= 105`
* `1 <= arr[i] <= 109` | null |
Python + explanation (why gcd?) | make-k-subarray-sums-equal | 0 | 1 | Right from the beginning: I am not that smart to solve this by myself. It took some time to read the solutions given by others. Specifically [this post](https://leetcode.com/problems/make-k-subarray-sums-equal/solutions/3366442/python3-find-median-of-each-gcd-defined-subarray-w-examples/) by [xil899](https://leetcode.com/xil899/) was very helpful\n\n# Approach\nFirst let\'s deal with the regular array taking, for example, `k = 4` and writing the conditions to be satisfied for subarrays to be equal\n\n$$ a_{0} + a_{1} + a_{2} + a_{3} = a_{1} + a_{2}+ a_{3} + a_{4}; \\rightarrow a_{0} = a_{4}$$\n$$ a_{1} + a_{2}+ a_{3} + a_{4} = a_{2} + a_{3}+ a_{4} + a_{5}; \\rightarrow a_{1} = a_{5}$$\n$$ a_{2} + a_{3}+ a_{4} + a_{5} = a_{3}+ a_{4} + a_{5} + a_{6}; \\rightarrow a_{2} = a_{6}$$\n$$ a_{3}+ a_{4} + a_{5} + a_{6} = a_{4} + a_{5} + a_{6} + a_{7} ; \\rightarrow a_{3} = a_{7}$$\n...\nYou probably got the point. Now we know that to make all subarrays of length `k` equal we must have\n$$ a_{0} = a_{4} = a_{8} = ...$$\n$$ a_{1} = a_{5} = a_{9} = ...$$\n$$ a_{2} = a_{6} = a_{10} = ...$$\n...\n\nNow for each of a<sub>i</sub> group that needs to be equal we have to solve problem [462 "Minimum Moves to Equal Array Elemens II"](https://leetcode.com/problems/minimum-moves-to-equal-array-elements-ii/).\n\nThe basic idea is the following. For array of two elements `[a, b]`, `a < b` we can select any number `num` between them `a <= num <= b` and equalize `a` and `b` to `num`. The result will be the same regardless of exact `num` value since `num - a + b - num = b - a`.\nFor the array of length > `2`, we can follow the same logic: select min and max elements of the array. `num` should be between them. Now, delete these two elements and repeat. Finally we end up either with a single element if array size is odd, or with two remaining elements either of which can be selected. Half of deleted elements will be greater (or equal) than remaining element(s), and another half - smaller (or equal). Thus, basically we need to find a median of an array.\nThe solution of this part (minimum number of operations to equalize array) from [problem 462](https://leetcode.com/problems/minimum-moves-to-equal-array-elements-ii/) is\n```python\ndef minMoves2(nums: List[int]) -> int:\n median = sorted(nums)[len(nums)//2]\n return sum(abs(num - median) for num in nums)\n# end minMoves2(...)\n```\nNow the tricky part: we have to deal somehow with the fact the array is circular. In brief, all the elements with distance equal to the greatest common divisor of `n` and `k` fall into the same group that needs to be equalized. This is demonstrated on the following picture\n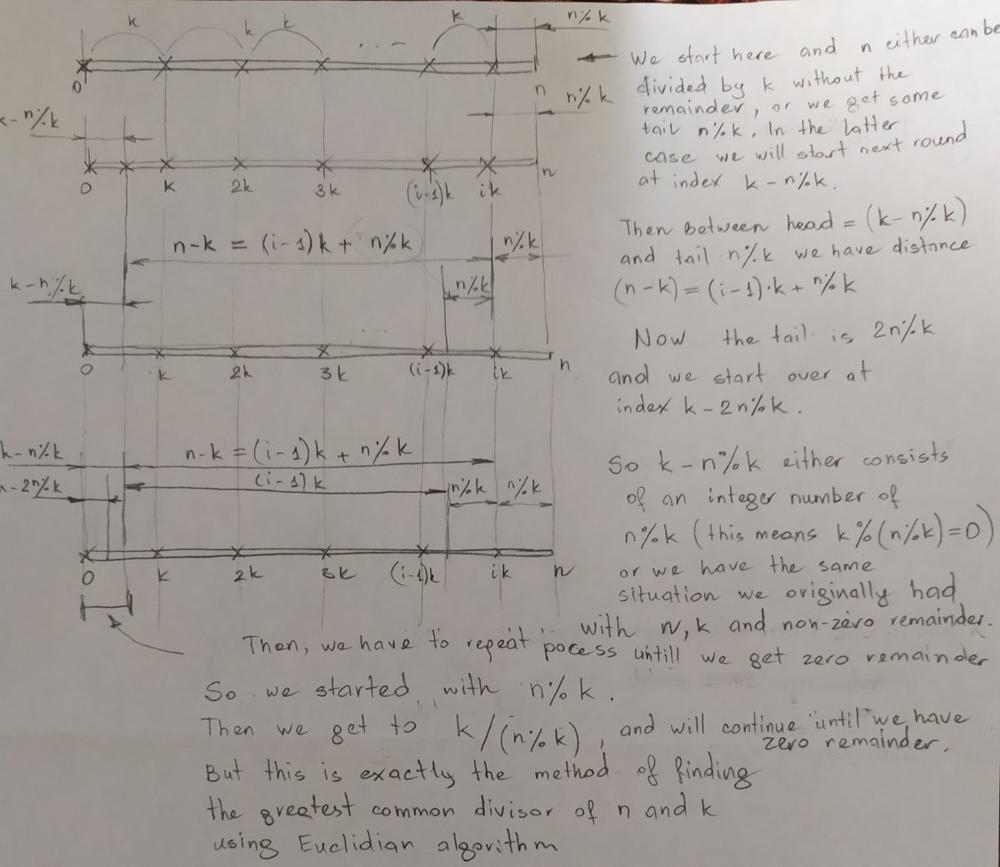\n\n\n# Complexity\n- Time complexity: O(nlogn)\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: O(n)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def makeSubKSumEqual(self, arr: List[int], k: int) -> int:\n ans = 0\n n = len(arr)\n step = gcd(n, k)\n for i in range(step):\n nums = arr[i::step]\n nums.sort()\n median_idx = len(nums)//2\n median = nums[median_idx]\n for num in nums[:median_idx]:\n ans += median - num\n for num in nums[median_idx:]:\n ans += num - median\n return ans\n # end makeSubKSumEqual()\n``` | 1 | You are given a **0-indexed** integer array `arr` and an integer `k`. The array `arr` is circular. In other words, the first element of the array is the next element of the last element, and the last element of the array is the previous element of the first element.
You can do the following operation any number of times:
* Pick any element from `arr` and increase or decrease it by `1`.
Return _the minimum number of operations such that the sum of each **subarray** of length_ `k` _is equal_.
A **subarray** is a contiguous part of the array.
**Example 1:**
**Input:** arr = \[1,4,1,3\], k = 2
**Output:** 1
**Explanation:** we can do one operation on index 1 to make its value equal to 3.
The array after the operation is \[1,3,1,3\]
- Subarray starts at index 0 is \[1, 3\], and its sum is 4
- Subarray starts at index 1 is \[3, 1\], and its sum is 4
- Subarray starts at index 2 is \[1, 3\], and its sum is 4
- Subarray starts at index 3 is \[3, 1\], and its sum is 4
**Example 2:**
**Input:** arr = \[2,5,5,7\], k = 3
**Output:** 5
**Explanation:** we can do three operations on index 0 to make its value equal to 5 and two operations on index 3 to make its value equal to 5.
The array after the operations is \[5,5,5,5\]
- Subarray starts at index 0 is \[5, 5, 5\], and its sum is 15
- Subarray starts at index 1 is \[5, 5, 5\], and its sum is 15
- Subarray starts at index 2 is \[5, 5, 5\], and its sum is 15
- Subarray starts at index 3 is \[5, 5, 5\], and its sum is 15
**Constraints:**
* `1 <= k <= arr.length <= 105`
* `1 <= arr[i] <= 109` | null |
[Proof] why use gcd | make-k-subarray-sums-equal | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nGiven ```n = len(arr)``` and ```k```, we prove that every ```gcd(n,k)```-th element in ```arr``` should be the same in order to make k-subarray sums equal.\n\n1. Assume ```arr``` is just a regular array. If ```arr[i]+...+arr[i+k-1] = arr[i+1]+...+arr[i+k]```, then ```arr[i] = arr[i+k]```. Then ```arr[i] = arr[i+mk]``` for any ```m``` as long as ```i+mk < n```.\n2. If ```arr``` is circular, we could extend the conclusion above to: given ```0<= i,j < n```, if there exist integers ```m, p``` such that ```j = i + mk - pn```, then ```arr[i] = arr[j]```.\n3. The relationship between ```i, j``` is equivalent to ```j - i = mk - pn = (mk0 - pn0)*gcd(n,k)``` where ```k = k0*gcd(n,k)``` and ```n = n0*gcd(n,k)```. Note that ```gcd(n,k)``` is the greatest common divisor of ```n``` and ```k```, which is an integer greater than equal to ```1```. **Since ```mk0 - pn0``` could be any valid integer, then every ```gcd(n,k)```-th element in ```arr``` should be equal.**\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\nCollect every ```gcd(n,k)```-th element in a group, compute the median, calculate the number of adds and removes to move other elements to the median. Sum up all numbers for each group to get the answer.\n\n\n# Complexity\n- Time complexity: ```O(n log n)```\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: ```O(n)```\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def makeSubKSumEqual(self, arr: List[int], k: int) -> int:\n n = len(arr)\n ans, d = 0, math.gcd(n,k)\n for i in range(d):\n subarr = sorted(arr[i:n:d])\n median = subarr[len(subarr)//2]\n ans += sum(abs(a - median) for a in subarr)\n return ans\n \n``` | 1 | You are given a **0-indexed** integer array `arr` and an integer `k`. The array `arr` is circular. In other words, the first element of the array is the next element of the last element, and the last element of the array is the previous element of the first element.
You can do the following operation any number of times:
* Pick any element from `arr` and increase or decrease it by `1`.
Return _the minimum number of operations such that the sum of each **subarray** of length_ `k` _is equal_.
A **subarray** is a contiguous part of the array.
**Example 1:**
**Input:** arr = \[1,4,1,3\], k = 2
**Output:** 1
**Explanation:** we can do one operation on index 1 to make its value equal to 3.
The array after the operation is \[1,3,1,3\]
- Subarray starts at index 0 is \[1, 3\], and its sum is 4
- Subarray starts at index 1 is \[3, 1\], and its sum is 4
- Subarray starts at index 2 is \[1, 3\], and its sum is 4
- Subarray starts at index 3 is \[3, 1\], and its sum is 4
**Example 2:**
**Input:** arr = \[2,5,5,7\], k = 3
**Output:** 5
**Explanation:** we can do three operations on index 0 to make its value equal to 5 and two operations on index 3 to make its value equal to 5.
The array after the operations is \[5,5,5,5\]
- Subarray starts at index 0 is \[5, 5, 5\], and its sum is 15
- Subarray starts at index 1 is \[5, 5, 5\], and its sum is 15
- Subarray starts at index 2 is \[5, 5, 5\], and its sum is 15
- Subarray starts at index 3 is \[5, 5, 5\], and its sum is 15
**Constraints:**
* `1 <= k <= arr.length <= 105`
* `1 <= arr[i] <= 109` | null |
Subsets and Splits