title
stringlengths 1
100
| titleSlug
stringlengths 3
77
| Java
int64 0
1
| Python3
int64 1
1
| content
stringlengths 28
44.4k
| voteCount
int64 0
3.67k
| question_content
stringlengths 65
5k
| question_hints
stringclasses 970
values |
---|---|---|---|---|---|---|---|
[Python] Binary search, 2-liner, 1-liner | minimum-cost-to-make-array-equal | 0 | 1 | # Intuition\nIf we try to calculate the cost of any value from min(nums) to max(nums), we see that the cost starts high, goes down to a minimum, and then goes up again. For example, for nums = `[1,3,5,2]` and cost = `[2,3,1,14]`, the cost for any value from 1 (min of nums) to 5 (max of nums) is `[24,8,20,38,56]`.\n\nTo find the minimum, simply binary search for the first number whose next number is larger than itself.\n\n# Full\n```\nclass Solution:\n def minCost(self, nums: List[int], cost: List[int]) -> int:\n def total(target):\n return sum(abs(target - n) * c for n, c in zip(nums, cost))\n \n lo, hi = min(nums), max(nums)\n\n while lo < hi:\n mid = (lo + hi) // 2\n if total(mid) < total(mid + 1):\n hi = mid\n else:\n lo = mid + 1\n \n return total(lo)\n\n```\n\n# 2-liner\n```\nclass Solution:\n def minCost(self, nums: List[int], cost: List[int]) -> int:\n total = lambda target: sum(abs(target - n) * c for n, c in zip(nums, cost))\n return total(min(nums) + bisect_left(range(min(nums), max(nums)), True, key=lambda n: total(n) < total(n + 1)))\n\n```\n\n# Dumb long 1-liner\n```\nclass Solution:\n def minCost(self, nums: List[int], cost: List[int]) -> int:\n return (f := lambda target: sum(abs(target - n) * c for n, c in zip(nums, cost)))(min(nums) + bisect_left(range(min(nums), max(nums)), True, key=lambda n: f(n) < f(n + 1)))\n\n```\n | 2 | You are given two **0-indexed** arrays `nums` and `cost` consisting each of `n` **positive** integers.
You can do the following operation **any** number of times:
* Increase or decrease **any** element of the array `nums` by `1`.
The cost of doing one operation on the `ith` element is `cost[i]`.
Return _the **minimum** total cost such that all the elements of the array_ `nums` _become **equal**_.
**Example 1:**
**Input:** nums = \[1,3,5,2\], cost = \[2,3,1,14\]
**Output:** 8
**Explanation:** We can make all the elements equal to 2 in the following way:
- Increase the 0th element one time. The cost is 2.
- Decrease the 1st element one time. The cost is 3.
- Decrease the 2nd element three times. The cost is 1 + 1 + 1 = 3.
The total cost is 2 + 3 + 3 = 8.
It can be shown that we cannot make the array equal with a smaller cost.
**Example 2:**
**Input:** nums = \[2,2,2,2,2\], cost = \[4,2,8,1,3\]
**Output:** 0
**Explanation:** All the elements are already equal, so no operations are needed.
**Constraints:**
* `n == nums.length == cost.length`
* `1 <= n <= 105`
* `1 <= nums[i], cost[i] <= 106` | null |
Sort odd and even fully explained Python. | minimum-number-of-operations-to-make-arrays-similar | 0 | 1 | 1. Seperate tha given nums and target arrays into two i.e., nums1 and target1 for even and nums2 and target2 for odd. Because, by adding multiples of 2 to an odd number, we can only can an odd number and same goes with even one.\n\n2. Now Sort them, because then only we will find the nearest number which can be acheived by either increasing or decreasing the number in nums.\n\n3. Now we can check for either the increasing case or the decreasing case of numbers to acheive the targets and store it in a variable.Because when an increase operation is performed automatically decrease should also be performed and both will be counted as a single operation. \n\n\n```\n def makeSimilar(self, nums: List[int], target: List[int]) -> int:\n nums1 = []\n target1 = []\n nums2 = []\n target2 = []\n nums.sort()\n target.sort()\n for i in range(len(nums)):\n if nums[i]%2==0:\n nums1.append(nums[i])\n else:\n nums2.append(nums[i])\n if target[i]%2==0:\n target1.append(target[i])\n else:\n target2.append(target[i])\n inc = 0\n dec = 0\n for i in range(len(nums1)):\n if nums1[i]<target1[i]:\n inc+=((target1[i]-nums1[i])//2) #dividing by 2 because to increase it by 2 you need 1 operation.\n \n for i in range(len(nums2)):\n if nums2[i]<target2[i]:\n inc+=((target2[i]-nums2[i])//2)\n return inc\n``` | 2 | You are given two positive integer arrays `nums` and `target`, of the same length.
In one operation, you can choose any two **distinct** indices `i` and `j` where `0 <= i, j < nums.length` and:
* set `nums[i] = nums[i] + 2` and
* set `nums[j] = nums[j] - 2`.
Two arrays are considered to be **similar** if the frequency of each element is the same.
Return _the minimum number of operations required to make_ `nums` _similar to_ `target`. The test cases are generated such that `nums` can always be similar to `target`.
**Example 1:**
**Input:** nums = \[8,12,6\], target = \[2,14,10\]
**Output:** 2
**Explanation:** It is possible to make nums similar to target in two operations:
- Choose i = 0 and j = 2, nums = \[10,12,4\].
- Choose i = 1 and j = 2, nums = \[10,14,2\].
It can be shown that 2 is the minimum number of operations needed.
**Example 2:**
**Input:** nums = \[1,2,5\], target = \[4,1,3\]
**Output:** 1
**Explanation:** We can make nums similar to target in one operation:
- Choose i = 1 and j = 2, nums = \[1,4,3\].
**Example 3:**
**Input:** nums = \[1,1,1,1,1\], target = \[1,1,1,1,1\]
**Output:** 0
**Explanation:** The array nums is already similiar to target.
**Constraints:**
* `n == nums.length == target.length`
* `1 <= n <= 105`
* `1 <= nums[i], target[i] <= 106`
* It is possible to make `nums` similar to `target`. | null |
[Python3] SortedList + Greedy O(NlogN) | minimum-number-of-operations-to-make-arrays-similar | 0 | 1 | ```\nfrom sortedcontainers import SortedList\nclass Solution:\n def makeSimilar(self, nums: List[int], target: List[int]) -> int:\n odd_nums = SortedList([num for num in nums if num % 2 == 1])\n even_nums = SortedList([num for num in nums if num % 2 == 0])\n odd_target = SortedList([num for num in target if num % 2 == 1])\n even_target = SortedList([num for num in target if num % 2 == 0])\n ans = 0\n while odd_nums:\n ans += abs(odd_nums[0] - odd_target[0]) // 2\n odd_nums.pop(0)\n odd_target.remove(odd_target[0])\n while even_nums:\n ans += abs(even_nums[0] - even_target[0]) // 2\n even_nums.pop(0)\n even_target.remove(even_target[0])\n return ans // 2\n``` | 2 | You are given two positive integer arrays `nums` and `target`, of the same length.
In one operation, you can choose any two **distinct** indices `i` and `j` where `0 <= i, j < nums.length` and:
* set `nums[i] = nums[i] + 2` and
* set `nums[j] = nums[j] - 2`.
Two arrays are considered to be **similar** if the frequency of each element is the same.
Return _the minimum number of operations required to make_ `nums` _similar to_ `target`. The test cases are generated such that `nums` can always be similar to `target`.
**Example 1:**
**Input:** nums = \[8,12,6\], target = \[2,14,10\]
**Output:** 2
**Explanation:** It is possible to make nums similar to target in two operations:
- Choose i = 0 and j = 2, nums = \[10,12,4\].
- Choose i = 1 and j = 2, nums = \[10,14,2\].
It can be shown that 2 is the minimum number of operations needed.
**Example 2:**
**Input:** nums = \[1,2,5\], target = \[4,1,3\]
**Output:** 1
**Explanation:** We can make nums similar to target in one operation:
- Choose i = 1 and j = 2, nums = \[1,4,3\].
**Example 3:**
**Input:** nums = \[1,1,1,1,1\], target = \[1,1,1,1,1\]
**Output:** 0
**Explanation:** The array nums is already similiar to target.
**Constraints:**
* `n == nums.length == target.length`
* `1 <= n <= 105`
* `1 <= nums[i], target[i] <= 106`
* It is possible to make `nums` similar to `target`. | null |
Python | Simple Odd/Even Sorting O(nlogn) | Walmart OA India | Simple Explanation | minimum-number-of-operations-to-make-arrays-similar | 0 | 1 | ```\nclass Solution:\n def makeSimilar(self, A: List[int], B: List[int]) -> int:\n if sum(A)!=sum(B): return 0\n # The first intuition is that only odd numbers can be chaged to odd numbers and even to even hence separate them\n # Now minimum steps to making the target to highest number in B is by converting max of A to max of B similarily\n # every number in A can be paired with a number in B by index hence sorting\n # now we need only the number of positives or number of negatives.\n oddA,evenA=[i for i in A if i%2],[i for i in A if i%2==0]\n oddB,evenB=[i for i in B if i%2],[i for i in B if i%2==0] \n oddA.sort(),evenA.sort()\n oddB.sort(),evenB.sort()\n res=0\n for i,j in zip(oddA,oddB):\n if i>=j: res+=i-j\n \n for i,j in zip(evenA,evenB):\n if i>=j: res+=i-j\n \n return res//2\n``` | 4 | You are given two positive integer arrays `nums` and `target`, of the same length.
In one operation, you can choose any two **distinct** indices `i` and `j` where `0 <= i, j < nums.length` and:
* set `nums[i] = nums[i] + 2` and
* set `nums[j] = nums[j] - 2`.
Two arrays are considered to be **similar** if the frequency of each element is the same.
Return _the minimum number of operations required to make_ `nums` _similar to_ `target`. The test cases are generated such that `nums` can always be similar to `target`.
**Example 1:**
**Input:** nums = \[8,12,6\], target = \[2,14,10\]
**Output:** 2
**Explanation:** It is possible to make nums similar to target in two operations:
- Choose i = 0 and j = 2, nums = \[10,12,4\].
- Choose i = 1 and j = 2, nums = \[10,14,2\].
It can be shown that 2 is the minimum number of operations needed.
**Example 2:**
**Input:** nums = \[1,2,5\], target = \[4,1,3\]
**Output:** 1
**Explanation:** We can make nums similar to target in one operation:
- Choose i = 1 and j = 2, nums = \[1,4,3\].
**Example 3:**
**Input:** nums = \[1,1,1,1,1\], target = \[1,1,1,1,1\]
**Output:** 0
**Explanation:** The array nums is already similiar to target.
**Constraints:**
* `n == nums.length == target.length`
* `1 <= n <= 105`
* `1 <= nums[i], target[i] <= 106`
* It is possible to make `nums` similar to `target`. | null |
Python Hard | minimum-number-of-operations-to-make-arrays-similar | 0 | 1 | ```\nclass Solution:\n def makeSimilar(self, nums: List[int], target: List[int]) -> int:\n \'\'\'\n keep track of even and odd numbers in target\n \n \'\'\'\n ans = 0\n nums.sort()\n target.sort()\n\n hOdd = []\n hEven = []\n hTargetOdd = []\n hTargetEven = []\n\n for n in nums:\n if n % 2 == 0:\n heapq.heappush(hEven, n)\n\n else:\n heapq.heappush(hOdd, n)\n\n for n in target:\n if n % 2 == 0:\n heapq.heappush(hTargetEven, n)\n\n else:\n heapq.heappush(hTargetOdd, n)\n\n totalEven = 0\n totalOdd = 0\n while hOdd:\n a, b = heapq.heappop(hOdd), heapq.heappop(hTargetOdd)\n if a - b > 0:\n totalOdd += a - b\n\n while hEven:\n a, b = heapq.heappop(hEven), heapq.heappop(hTargetEven)\n if a - b > 0:\n totalEven += a - b\n\n\n\n return totalEven // 2 + totalOdd // 2\n\n\n\n``` | 0 | You are given two positive integer arrays `nums` and `target`, of the same length.
In one operation, you can choose any two **distinct** indices `i` and `j` where `0 <= i, j < nums.length` and:
* set `nums[i] = nums[i] + 2` and
* set `nums[j] = nums[j] - 2`.
Two arrays are considered to be **similar** if the frequency of each element is the same.
Return _the minimum number of operations required to make_ `nums` _similar to_ `target`. The test cases are generated such that `nums` can always be similar to `target`.
**Example 1:**
**Input:** nums = \[8,12,6\], target = \[2,14,10\]
**Output:** 2
**Explanation:** It is possible to make nums similar to target in two operations:
- Choose i = 0 and j = 2, nums = \[10,12,4\].
- Choose i = 1 and j = 2, nums = \[10,14,2\].
It can be shown that 2 is the minimum number of operations needed.
**Example 2:**
**Input:** nums = \[1,2,5\], target = \[4,1,3\]
**Output:** 1
**Explanation:** We can make nums similar to target in one operation:
- Choose i = 1 and j = 2, nums = \[1,4,3\].
**Example 3:**
**Input:** nums = \[1,1,1,1,1\], target = \[1,1,1,1,1\]
**Output:** 0
**Explanation:** The array nums is already similiar to target.
**Constraints:**
* `n == nums.length == target.length`
* `1 <= n <= 105`
* `1 <= nums[i], target[i] <= 106`
* It is possible to make `nums` similar to `target`. | null |
Python Solve | minimum-number-of-operations-to-make-arrays-similar | 0 | 1 | \n\n# Code\n```\nclass Solution:\n def makeSimilar(self, nums: List[int], target: List[int]) -> int:\n n=len(nums)\n inc=0\n dec=0\n arr=[]\n nums.sort()\n target.sort()\n i=0\n j=0\n\n while i<n and j<n:\n if nums[i]%2==0 and target[j]%2==0:\n arr.append((nums[i], target[j]))\n i+=1\n j+=1\n else:\n if nums[i]%2!=0:\n i+=1\n if target[j]%2!=0:\n j+=1\n\n i=0\n j=0\n while i<n and j<n:\n if nums[i]%2!=0 and target[j]%2!=0:\n arr.append((nums[i], target[j]))\n i+=1\n j+=1\n \n else:\n if nums[i]%2==0:\n i+=1\n if target[j]%2==0:\n j+=1\n \n for i in range(n):\n if arr[i][0]==arr[i][1]:\n continue\n\n diff=abs(arr[i][0]-arr[i][1])\n if diff%2==0:\n if arr[i][0]<arr[i][1]:\n inc+=diff//2\n else:\n dec+=diff//2\n\n return -1 if inc!=dec else inc\n \n\n``` | 0 | You are given two positive integer arrays `nums` and `target`, of the same length.
In one operation, you can choose any two **distinct** indices `i` and `j` where `0 <= i, j < nums.length` and:
* set `nums[i] = nums[i] + 2` and
* set `nums[j] = nums[j] - 2`.
Two arrays are considered to be **similar** if the frequency of each element is the same.
Return _the minimum number of operations required to make_ `nums` _similar to_ `target`. The test cases are generated such that `nums` can always be similar to `target`.
**Example 1:**
**Input:** nums = \[8,12,6\], target = \[2,14,10\]
**Output:** 2
**Explanation:** It is possible to make nums similar to target in two operations:
- Choose i = 0 and j = 2, nums = \[10,12,4\].
- Choose i = 1 and j = 2, nums = \[10,14,2\].
It can be shown that 2 is the minimum number of operations needed.
**Example 2:**
**Input:** nums = \[1,2,5\], target = \[4,1,3\]
**Output:** 1
**Explanation:** We can make nums similar to target in one operation:
- Choose i = 1 and j = 2, nums = \[1,4,3\].
**Example 3:**
**Input:** nums = \[1,1,1,1,1\], target = \[1,1,1,1,1\]
**Output:** 0
**Explanation:** The array nums is already similiar to target.
**Constraints:**
* `n == nums.length == target.length`
* `1 <= n <= 105`
* `1 <= nums[i], target[i] <= 106`
* It is possible to make `nums` similar to `target`. | null |
Easy to understand python solution | odd-string-difference | 0 | 1 | \n\n# Code\n```\nclass Solution:\n def oddString(self, words: List[str]) -> str:\n k=len(words[0])\n arr=[]\n for i in words:\n l=[]\n for j in range(1,k):\n diff=ord(i[j])-ord(i[j-1])\n l.append(diff)\n arr.append(l)\n for i in range(len(arr)):\n if arr.count(arr[i])==1:\n return words[i]\n``` | 4 | You are given an array of equal-length strings `words`. Assume that the length of each string is `n`.
Each string `words[i]` can be converted into a **difference integer array** `difference[i]` of length `n - 1` where `difference[i][j] = words[i][j+1] - words[i][j]` where `0 <= j <= n - 2`. Note that the difference between two letters is the difference between their **positions** in the alphabet i.e. the position of `'a'` is `0`, `'b'` is `1`, and `'z'` is `25`.
* For example, for the string `"acb "`, the difference integer array is `[2 - 0, 1 - 2] = [2, -1]`.
All the strings in words have the same difference integer array, **except one**. You should find that string.
Return _the string in_ `words` _that has different **difference integer array**._
**Example 1:**
**Input:** words = \[ "adc ", "wzy ", "abc "\]
**Output:** "abc "
**Explanation:**
- The difference integer array of "adc " is \[3 - 0, 2 - 3\] = \[3, -1\].
- The difference integer array of "wzy " is \[25 - 22, 24 - 25\]= \[3, -1\].
- The difference integer array of "abc " is \[1 - 0, 2 - 1\] = \[1, 1\].
The odd array out is \[1, 1\], so we return the corresponding string, "abc ".
**Example 2:**
**Input:** words = \[ "aaa ", "bob ", "ccc ", "ddd "\]
**Output:** "bob "
**Explanation:** All the integer arrays are \[0, 0\] except for "bob ", which corresponds to \[13, -13\].
**Constraints:**
* `3 <= words.length <= 100`
* `n == words[i].length`
* `2 <= n <= 20`
* `words[i]` consists of lowercase English letters. | null |
2 liner in python for fun | odd-string-difference | 0 | 1 | \n\n# Code\n```\nclass Solution:\n def oddString(self, words: List[str]) -> str:\n a = [[ord(s[i])-ord(s[i-1]) for i in range(1,len(s))] for s in words]\n return words[a.index(*[arr for arr in a if a.count(arr) == 1])]\n``` | 2 | You are given an array of equal-length strings `words`. Assume that the length of each string is `n`.
Each string `words[i]` can be converted into a **difference integer array** `difference[i]` of length `n - 1` where `difference[i][j] = words[i][j+1] - words[i][j]` where `0 <= j <= n - 2`. Note that the difference between two letters is the difference between their **positions** in the alphabet i.e. the position of `'a'` is `0`, `'b'` is `1`, and `'z'` is `25`.
* For example, for the string `"acb "`, the difference integer array is `[2 - 0, 1 - 2] = [2, -1]`.
All the strings in words have the same difference integer array, **except one**. You should find that string.
Return _the string in_ `words` _that has different **difference integer array**._
**Example 1:**
**Input:** words = \[ "adc ", "wzy ", "abc "\]
**Output:** "abc "
**Explanation:**
- The difference integer array of "adc " is \[3 - 0, 2 - 3\] = \[3, -1\].
- The difference integer array of "wzy " is \[25 - 22, 24 - 25\]= \[3, -1\].
- The difference integer array of "abc " is \[1 - 0, 2 - 1\] = \[1, 1\].
The odd array out is \[1, 1\], so we return the corresponding string, "abc ".
**Example 2:**
**Input:** words = \[ "aaa ", "bob ", "ccc ", "ddd "\]
**Output:** "bob "
**Explanation:** All the integer arrays are \[0, 0\] except for "bob ", which corresponds to \[13, -13\].
**Constraints:**
* `3 <= words.length <= 100`
* `n == words[i].length`
* `2 <= n <= 20`
* `words[i]` consists of lowercase English letters. | null |
Odd String Difference with step by step explanation | odd-string-difference | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n1. First, we initialize n with the length of the words array and m with the length of each word in the words array.\n\n2. Next, we initialize an empty list diff to store the difference arrays of all the words.\n\n3. Then, we loop through all the words in the words array and calculate the difference array of each word. The difference array is calculated by subtracting the ASCII value of the current character from the ASCII value of the next character in each word.\n\n4. After that, we use the collections.Counter function to count the frequency of each difference array in the diff list.\n\n5. Finally, we loop through the diff list and return the first word whose difference array has a frequency of 1 in the diff list. This word is the word with a different difference array.\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def oddString(self, words: List[str]) -> str:\n n = len(words)\n m = len(words[0])\n diff = []\n for i in range(n):\n cur = []\n for j in range(m-1):\n cur.append(ord(words[i][j+1]) - ord(words[i][j]))\n diff.append(tuple(cur))\n \n cnt = collections.Counter(diff)\n for i in range(n):\n if cnt[diff[i]] == 1:\n return words[i]\n\n``` | 1 | You are given an array of equal-length strings `words`. Assume that the length of each string is `n`.
Each string `words[i]` can be converted into a **difference integer array** `difference[i]` of length `n - 1` where `difference[i][j] = words[i][j+1] - words[i][j]` where `0 <= j <= n - 2`. Note that the difference between two letters is the difference between their **positions** in the alphabet i.e. the position of `'a'` is `0`, `'b'` is `1`, and `'z'` is `25`.
* For example, for the string `"acb "`, the difference integer array is `[2 - 0, 1 - 2] = [2, -1]`.
All the strings in words have the same difference integer array, **except one**. You should find that string.
Return _the string in_ `words` _that has different **difference integer array**._
**Example 1:**
**Input:** words = \[ "adc ", "wzy ", "abc "\]
**Output:** "abc "
**Explanation:**
- The difference integer array of "adc " is \[3 - 0, 2 - 3\] = \[3, -1\].
- The difference integer array of "wzy " is \[25 - 22, 24 - 25\]= \[3, -1\].
- The difference integer array of "abc " is \[1 - 0, 2 - 1\] = \[1, 1\].
The odd array out is \[1, 1\], so we return the corresponding string, "abc ".
**Example 2:**
**Input:** words = \[ "aaa ", "bob ", "ccc ", "ddd "\]
**Output:** "bob "
**Explanation:** All the integer arrays are \[0, 0\] except for "bob ", which corresponds to \[13, -13\].
**Constraints:**
* `3 <= words.length <= 100`
* `n == words[i].length`
* `2 <= n <= 20`
* `words[i]` consists of lowercase English letters. | null |
Python ✅✅✅ || Faster than 90.54% || Memory Beats 78.47% | odd-string-difference | 0 | 1 | ### ***Note***: List Comprehension is used in my code!\n\n# Code\n```\nclass Solution:\n def oddString(self, words):\n difference = []\n alphabet = "abcdefghijklmnopqrstuvwxyz"\n for word in words:\n diff = []\n for j in range(len(word) - 1):\n diff.append(alphabet.index(word[j+1]) - alphabet.index(word[j]))\n difference.append(diff)\n return [ words[d] for d in range(len(difference)) if difference.count(difference[d]) == 1 ][0]\n```\n\n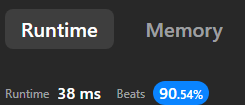\n\n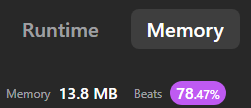\n\n | 1 | You are given an array of equal-length strings `words`. Assume that the length of each string is `n`.
Each string `words[i]` can be converted into a **difference integer array** `difference[i]` of length `n - 1` where `difference[i][j] = words[i][j+1] - words[i][j]` where `0 <= j <= n - 2`. Note that the difference between two letters is the difference between their **positions** in the alphabet i.e. the position of `'a'` is `0`, `'b'` is `1`, and `'z'` is `25`.
* For example, for the string `"acb "`, the difference integer array is `[2 - 0, 1 - 2] = [2, -1]`.
All the strings in words have the same difference integer array, **except one**. You should find that string.
Return _the string in_ `words` _that has different **difference integer array**._
**Example 1:**
**Input:** words = \[ "adc ", "wzy ", "abc "\]
**Output:** "abc "
**Explanation:**
- The difference integer array of "adc " is \[3 - 0, 2 - 3\] = \[3, -1\].
- The difference integer array of "wzy " is \[25 - 22, 24 - 25\]= \[3, -1\].
- The difference integer array of "abc " is \[1 - 0, 2 - 1\] = \[1, 1\].
The odd array out is \[1, 1\], so we return the corresponding string, "abc ".
**Example 2:**
**Input:** words = \[ "aaa ", "bob ", "ccc ", "ddd "\]
**Output:** "bob "
**Explanation:** All the integer arrays are \[0, 0\] except for "bob ", which corresponds to \[13, -13\].
**Constraints:**
* `3 <= words.length <= 100`
* `n == words[i].length`
* `2 <= n <= 20`
* `words[i]` consists of lowercase English letters. | null |
[Python] Simple HashSet solution O((N+M) * L^3) | words-within-two-edits-of-dictionary | 0 | 1 | With the help of a generator we can produce all the possible modifications:\n* 1 character is different (e.g. wood --> `[\'*ood\', \'w*od\', \'wo*d\', \'woo*\']`)\n* 2 characters are different (e.g. wood --> `[\'**od\', \'*o*d\', \'*oo*\', \'w**d\', \'w*o*\', \'wo**\']`)\n\nWe traverse the dictionary array and store each modification in a HashSet.\nLastly, we traverse the queries array and we to try find any of the possible modifications in the HashSet. If we\'re able to do so, then we include that word in our answer, as it can match a word from dictionary after at most two edits.\n\n```\nclass Solution:\n def twoEditWords(self, queries: List[str], dictionary: List[str]) -> List[str]:\n # T: ((N + M) * L^3), S: O(M * L^3)\n N, M, L = len(queries), len(dictionary), len(queries[0])\n validWords = set()\n \n for word in dictionary:\n for w in self.wordModifications(word):\n validWords.add(w)\n \n ans = []\n for word in queries:\n for w in self.wordModifications(word):\n if w in validWords:\n ans.append(word)\n break\n\n return ans\n \n def wordModifications(self, word):\n # T: O(L^3)\n L = len(word)\n for i in range(L):\n yield word[:i] + "*" + word[i+1:]\n\n for i, j in itertools.combinations(range(L), 2):\n yield word[:i] + "*" + word[i+1:j] + "*" + word[j+1:]\n``` | 2 | You are given two string arrays, `queries` and `dictionary`. All words in each array comprise of lowercase English letters and have the same length.
In one **edit** you can take a word from `queries`, and change any letter in it to any other letter. Find all words from `queries` that, after a **maximum** of two edits, equal some word from `dictionary`.
Return _a list of all words from_ `queries`_,_ _that match with some word from_ `dictionary` _after a maximum of **two edits**_. Return the words in the **same order** they appear in `queries`.
**Example 1:**
**Input:** queries = \[ "word ", "note ", "ants ", "wood "\], dictionary = \[ "wood ", "joke ", "moat "\]
**Output:** \[ "word ", "note ", "wood "\]
**Explanation:**
- Changing the 'r' in "word " to 'o' allows it to equal the dictionary word "wood ".
- Changing the 'n' to 'j' and the 't' to 'k' in "note " changes it to "joke ".
- It would take more than 2 edits for "ants " to equal a dictionary word.
- "wood " can remain unchanged (0 edits) and match the corresponding dictionary word.
Thus, we return \[ "word ", "note ", "wood "\].
**Example 2:**
**Input:** queries = \[ "yes "\], dictionary = \[ "not "\]
**Output:** \[\]
**Explanation:**
Applying any two edits to "yes " cannot make it equal to "not ". Thus, we return an empty array.
**Constraints:**
* `1 <= queries.length, dictionary.length <= 100`
* `n == queries[i].length == dictionary[j].length`
* `1 <= n <= 100`
* All `queries[i]` and `dictionary[j]` are composed of lowercase English letters. | null |
Words Within Two Edits of Dictionary with step by step explanation | words-within-two-edits-of-dictionary | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n- The function is_valid takes two words as input, query and word, and returns True if the number of different characters between the two words is less than or equal to 2.\n- The main function wordWithinTwoEdits loops through each query in queries and then loops through each word in dictionary. If is_valid returns True for the current query and word, the query is added to the result list res and the inner loop breaks.\n- Finally, the function returns the res list.\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nfrom typing import List\n\nclass Solution:\n def twoEditWords(self, queries: List[str], dictionary: List[str]) -> List[str]:\n def is_valid(query, word):\n count = 0\n for i in range(len(word)):\n if word[i] != query[i]:\n count += 1\n if count > 2:\n return False\n return count <= 2\n\n res = []\n for query in queries:\n for word in dictionary:\n if is_valid(query, word):\n res.append(query)\n break\n return res\n``` | 1 | You are given two string arrays, `queries` and `dictionary`. All words in each array comprise of lowercase English letters and have the same length.
In one **edit** you can take a word from `queries`, and change any letter in it to any other letter. Find all words from `queries` that, after a **maximum** of two edits, equal some word from `dictionary`.
Return _a list of all words from_ `queries`_,_ _that match with some word from_ `dictionary` _after a maximum of **two edits**_. Return the words in the **same order** they appear in `queries`.
**Example 1:**
**Input:** queries = \[ "word ", "note ", "ants ", "wood "\], dictionary = \[ "wood ", "joke ", "moat "\]
**Output:** \[ "word ", "note ", "wood "\]
**Explanation:**
- Changing the 'r' in "word " to 'o' allows it to equal the dictionary word "wood ".
- Changing the 'n' to 'j' and the 't' to 'k' in "note " changes it to "joke ".
- It would take more than 2 edits for "ants " to equal a dictionary word.
- "wood " can remain unchanged (0 edits) and match the corresponding dictionary word.
Thus, we return \[ "word ", "note ", "wood "\].
**Example 2:**
**Input:** queries = \[ "yes "\], dictionary = \[ "not "\]
**Output:** \[\]
**Explanation:**
Applying any two edits to "yes " cannot make it equal to "not ". Thus, we return an empty array.
**Constraints:**
* `1 <= queries.length, dictionary.length <= 100`
* `n == queries[i].length == dictionary[j].length`
* `1 <= n <= 100`
* All `queries[i]` and `dictionary[j]` are composed of lowercase English letters. | null |
[Java/Python 3] Bruteforce code. | words-within-two-edits-of-dictionary | 1 | 1 | **Q & A**\n*Q1*: What is the `outer` in java code?\n*A1*: It is a java statement label. Please refer to [w3resource](https://www.w3resource.com/java-tutorial/java-branching-statements.php#:~:text=Labeled%20Statements.%20Although%20many%20statements%20in%20a%20Java,valid%20identifier%20that%20ends%20with%20a%20colon%20%28%3A%29.) and [Oracle The Java\u2122 Tutorials](https://docs.oracle.com/javase/tutorial/java/nutsandbolts/branch.html) for more details.\n\n**End of Q & A**\n\n----\n\nTraverse the `queries`, for each query, `q`, traverse the `dictionary` to look for if any word has no more than 2 different characters from `q`; If yes, store it into the solution `ans`, and continue to the next query;\n\n```java\n public List<String> twoEditWords(String[] queries, String[] dictionary) {\n List<String> ans = new ArrayList<>();\n outer:\n for (String q : queries) {\n for (String w : dictionary) {\n int cnt = 0;\n for (int i = 0; cnt < 3 && i < q.length(); ++i) {\n if (q.charAt(i) != w.charAt(i)) {\n ++cnt;\n }\n }\n if (cnt < 3) {\n ans.add(q);\n continue outer;\n }\n }\n }\n return ans;\n }\n```\nor simplified as:\n```java\n public List<String> twoEditWords(String[] queries, String[] dictionary) {\n List<String> ans = new ArrayList<>();\n for (String q : queries) {\n if (Arrays.stream(dictionary).anyMatch(w -> IntStream.range(0, w.length()).filter(i -> w.charAt(i) != q.charAt(i)).count() <= 2)) {\n ans.add(q);\n }\n }\n return ans;\n }\n```\n```python\n def twoEditWords(self, queries: List[str], dictionary: List[str]) -> List[str]:\n ans = []\n for q in queries:\n for w in dictionary:\n cnt = 0\n for c, d in zip(w, q):\n if c != d:\n cnt += 1\n if cnt > 2:\n break\n if cnt <= 2:\n ans.append(q)\n break\n return ans\n```\nor simplified as:\n```python\n def twoEditWords(self, queries: List[str], dictionary: List[str]) -> List[str]:\n ans = []\n for q in queries:\n if any(sum(c != d for c, d in zip(w, q)) <= 2 for w in dictionary):\n ans.append(q)\n return ans\n```\n\n**Analysis:**\n\nTime: `O(Q * D * W)`, space: `O(Q * W)`, where `Q = queries.length, D = dictionary.length, W = word size`. | 9 | You are given two string arrays, `queries` and `dictionary`. All words in each array comprise of lowercase English letters and have the same length.
In one **edit** you can take a word from `queries`, and change any letter in it to any other letter. Find all words from `queries` that, after a **maximum** of two edits, equal some word from `dictionary`.
Return _a list of all words from_ `queries`_,_ _that match with some word from_ `dictionary` _after a maximum of **two edits**_. Return the words in the **same order** they appear in `queries`.
**Example 1:**
**Input:** queries = \[ "word ", "note ", "ants ", "wood "\], dictionary = \[ "wood ", "joke ", "moat "\]
**Output:** \[ "word ", "note ", "wood "\]
**Explanation:**
- Changing the 'r' in "word " to 'o' allows it to equal the dictionary word "wood ".
- Changing the 'n' to 'j' and the 't' to 'k' in "note " changes it to "joke ".
- It would take more than 2 edits for "ants " to equal a dictionary word.
- "wood " can remain unchanged (0 edits) and match the corresponding dictionary word.
Thus, we return \[ "word ", "note ", "wood "\].
**Example 2:**
**Input:** queries = \[ "yes "\], dictionary = \[ "not "\]
**Output:** \[\]
**Explanation:**
Applying any two edits to "yes " cannot make it equal to "not ". Thus, we return an empty array.
**Constraints:**
* `1 <= queries.length, dictionary.length <= 100`
* `n == queries[i].length == dictionary[j].length`
* `1 <= n <= 100`
* All `queries[i]` and `dictionary[j]` are composed of lowercase English letters. | null |
python3 Solution O(1) and O(Q*D*L) | words-within-two-edits-of-dictionary | 0 | 1 | ```\n\nclass Solution:\n def twoEditWords(self, queries: List[str], dictionary: List[str]) -> List[str]:\n res=[]\n N=len(queries[0])\n for query in queries:\n for word in dictionary:\n delta=0\n \n for i in range(N):\n if query[i]!=word[i]:\n delta+=1\n \n if delta<=2:\n res.append(query)\n break\n \n return res | 2 | You are given two string arrays, `queries` and `dictionary`. All words in each array comprise of lowercase English letters and have the same length.
In one **edit** you can take a word from `queries`, and change any letter in it to any other letter. Find all words from `queries` that, after a **maximum** of two edits, equal some word from `dictionary`.
Return _a list of all words from_ `queries`_,_ _that match with some word from_ `dictionary` _after a maximum of **two edits**_. Return the words in the **same order** they appear in `queries`.
**Example 1:**
**Input:** queries = \[ "word ", "note ", "ants ", "wood "\], dictionary = \[ "wood ", "joke ", "moat "\]
**Output:** \[ "word ", "note ", "wood "\]
**Explanation:**
- Changing the 'r' in "word " to 'o' allows it to equal the dictionary word "wood ".
- Changing the 'n' to 'j' and the 't' to 'k' in "note " changes it to "joke ".
- It would take more than 2 edits for "ants " to equal a dictionary word.
- "wood " can remain unchanged (0 edits) and match the corresponding dictionary word.
Thus, we return \[ "word ", "note ", "wood "\].
**Example 2:**
**Input:** queries = \[ "yes "\], dictionary = \[ "not "\]
**Output:** \[\]
**Explanation:**
Applying any two edits to "yes " cannot make it equal to "not ". Thus, we return an empty array.
**Constraints:**
* `1 <= queries.length, dictionary.length <= 100`
* `n == queries[i].length == dictionary[j].length`
* `1 <= n <= 100`
* All `queries[i]` and `dictionary[j]` are composed of lowercase English letters. | null |
[Java/Python 3] Count the frequency of the remainder of modulo. | destroy-sequential-targets | 1 | 1 | **Q & A**\n\nQ1: What does `freqs.merge(n, 1, Integer::sum)` mean in Java code?\nA1: `freqs.merge(n, 1, Integer::sum)` is simiar to `freqs.put(n, freqs.getOrDefault(n, 0) + 1)`, which increase the frequency (occurrence) of `n` by `1`; The difference between them is that `merge` return the increased frequency but `put` returns old value: the frequency before being increased (or `null`).\n\n**End of Q & A**\n\n----\n\n1. Count the frequencies of `remainder = nums[i] % space`;\n2. Traverse the input array `nums` again and locate the minimum.\n\n```java\n public int destroyTargets(int[] nums, int space) {\n Map<Integer, Integer> freqs = new HashMap<>();\n int maxFreq = 0;\n for (int n : nums) {\n n %= space;\n maxFreq = Math.max(maxFreq, freqs.merge(n, 1, Integer::sum));\n }\n int min = Integer.MAX_VALUE;\n for (int n : nums) {\n if (freqs.get(n % space) == maxFreq && min > n) {\n min = n;\n }\n }\n return min;\n }\n```\n```python\n def destroyTargets(self, nums: List[int], space: int) -> int:\n freqs, mi = Counter(), inf\n for num in nums:\n freqs[num % space] += 1\n max_freq = max(freqs.values())\n for num in nums:\n if freqs[num % space] == max_freq and num < mi:\n mi = num\n return mi\n```\n\n**Analysis:**\n\nTime & space: `O(n)`, where `n = nums.length`.\n\n----\n\nPlease let me know if you have any questions, and **upvote** if the post is helpful. | 18 | You are given a **0-indexed** array `nums` consisting of positive integers, representing targets on a number line. You are also given an integer `space`.
You have a machine which can destroy targets. **Seeding** the machine with some `nums[i]` allows it to destroy all targets with values that can be represented as `nums[i] + c * space`, where `c` is any non-negative integer. You want to destroy the **maximum** number of targets in `nums`.
Return _the **minimum value** of_ `nums[i]` _you can seed the machine with to destroy the maximum number of targets._
**Example 1:**
**Input:** nums = \[3,7,8,1,1,5\], space = 2
**Output:** 1
**Explanation:** If we seed the machine with nums\[3\], then we destroy all targets equal to 1,3,5,7,9,...
In this case, we would destroy 5 total targets (all except for nums\[2\]).
It is impossible to destroy more than 5 targets, so we return nums\[3\].
**Example 2:**
**Input:** nums = \[1,3,5,2,4,6\], space = 2
**Output:** 1
**Explanation:** Seeding the machine with nums\[0\], or nums\[3\] destroys 3 targets.
It is not possible to destroy more than 3 targets.
Since nums\[0\] is the minimal integer that can destroy 3 targets, we return 1.
**Example 3:**
**Input:** nums = \[6,2,5\], space = 100
**Output:** 2
**Explanation:** Whatever initial seed we select, we can only destroy 1 target. The minimal seed is nums\[1\].
**Constraints:**
* `1 <= nums.length <= 105`
* `1 <= nums[i] <= 109`
* `1 <= space <= 109` | null |
[Python 3] Hash Table + Counting + Greedy - Easy to Understand | destroy-sequential-targets | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity: $$O(n)$$\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: $$O(n)$$\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def destroyTargets(self, nums: List[int], space: int) -> int:\n c = collections.Counter()\n max_target = 0\n for num in nums:\n val = num % space\n c[val] += 1\n max_target = max(max_target, c[val])\n \n res = max(nums)\n for num in nums:\n val = num % space\n if c[val] == max_target:\n res = min(res, num)\n return res\n``` | 3 | You are given a **0-indexed** array `nums` consisting of positive integers, representing targets on a number line. You are also given an integer `space`.
You have a machine which can destroy targets. **Seeding** the machine with some `nums[i]` allows it to destroy all targets with values that can be represented as `nums[i] + c * space`, where `c` is any non-negative integer. You want to destroy the **maximum** number of targets in `nums`.
Return _the **minimum value** of_ `nums[i]` _you can seed the machine with to destroy the maximum number of targets._
**Example 1:**
**Input:** nums = \[3,7,8,1,1,5\], space = 2
**Output:** 1
**Explanation:** If we seed the machine with nums\[3\], then we destroy all targets equal to 1,3,5,7,9,...
In this case, we would destroy 5 total targets (all except for nums\[2\]).
It is impossible to destroy more than 5 targets, so we return nums\[3\].
**Example 2:**
**Input:** nums = \[1,3,5,2,4,6\], space = 2
**Output:** 1
**Explanation:** Seeding the machine with nums\[0\], or nums\[3\] destroys 3 targets.
It is not possible to destroy more than 3 targets.
Since nums\[0\] is the minimal integer that can destroy 3 targets, we return 1.
**Example 3:**
**Input:** nums = \[6,2,5\], space = 100
**Output:** 2
**Explanation:** Whatever initial seed we select, we can only destroy 1 target. The minimal seed is nums\[1\].
**Constraints:**
* `1 <= nums.length <= 105`
* `1 <= nums[i] <= 109`
* `1 <= space <= 109` | null |
Python (Simple Maths) | destroy-sequential-targets | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def destroyTargets(self, nums, space):\n dict1 = defaultdict(int)\n\n for i in nums:\n dict1[i%space] += 1\n\n max_val = max(dict1.values())\n\n return min([i for i in nums if dict1[i%space] == max_val])\n\n\n``` | 1 | You are given a **0-indexed** array `nums` consisting of positive integers, representing targets on a number line. You are also given an integer `space`.
You have a machine which can destroy targets. **Seeding** the machine with some `nums[i]` allows it to destroy all targets with values that can be represented as `nums[i] + c * space`, where `c` is any non-negative integer. You want to destroy the **maximum** number of targets in `nums`.
Return _the **minimum value** of_ `nums[i]` _you can seed the machine with to destroy the maximum number of targets._
**Example 1:**
**Input:** nums = \[3,7,8,1,1,5\], space = 2
**Output:** 1
**Explanation:** If we seed the machine with nums\[3\], then we destroy all targets equal to 1,3,5,7,9,...
In this case, we would destroy 5 total targets (all except for nums\[2\]).
It is impossible to destroy more than 5 targets, so we return nums\[3\].
**Example 2:**
**Input:** nums = \[1,3,5,2,4,6\], space = 2
**Output:** 1
**Explanation:** Seeding the machine with nums\[0\], or nums\[3\] destroys 3 targets.
It is not possible to destroy more than 3 targets.
Since nums\[0\] is the minimal integer that can destroy 3 targets, we return 1.
**Example 3:**
**Input:** nums = \[6,2,5\], space = 100
**Output:** 2
**Explanation:** Whatever initial seed we select, we can only destroy 1 target. The minimal seed is nums\[1\].
**Constraints:**
* `1 <= nums.length <= 105`
* `1 <= nums[i] <= 109`
* `1 <= space <= 109` | null |
Python | Greedy | Group | Example | destroy-sequential-targets | 0 | 1 | \n```\nclass Solution:\n def destroyTargets(self, nums: List[int], space: int) -> int:\n\t\t# example: nums = [3,7,8,1,1,5], space = 2\n groups = defaultdict(list)\n for num in nums:\n groups[num % space].append(num)\n \n # print(groups) # defaultdict(<class \'list\'>, {1: [3, 7, 1, 1, 5], 0: [8]}) groups is [3, 7, 1, 1, 5] and [8] \n """ min of [3, 7, 1, 1, 5] can destroy all others (greedy approach) => 1 can destory 1,3,5,7 ... """\n performance = defaultdict(list)\n for group in groups.values():\n performance[len(group)].append(min(group))\n \n # print(performance) # defaultdict(<class \'list\'>, {5: [1], 1: [8]})\n\t\t# nums that can destory 5 targets are [1], nums that can destory 1 target are [8] \n return min(performance[max(performance)])\n``` | 3 | You are given a **0-indexed** array `nums` consisting of positive integers, representing targets on a number line. You are also given an integer `space`.
You have a machine which can destroy targets. **Seeding** the machine with some `nums[i]` allows it to destroy all targets with values that can be represented as `nums[i] + c * space`, where `c` is any non-negative integer. You want to destroy the **maximum** number of targets in `nums`.
Return _the **minimum value** of_ `nums[i]` _you can seed the machine with to destroy the maximum number of targets._
**Example 1:**
**Input:** nums = \[3,7,8,1,1,5\], space = 2
**Output:** 1
**Explanation:** If we seed the machine with nums\[3\], then we destroy all targets equal to 1,3,5,7,9,...
In this case, we would destroy 5 total targets (all except for nums\[2\]).
It is impossible to destroy more than 5 targets, so we return nums\[3\].
**Example 2:**
**Input:** nums = \[1,3,5,2,4,6\], space = 2
**Output:** 1
**Explanation:** Seeding the machine with nums\[0\], or nums\[3\] destroys 3 targets.
It is not possible to destroy more than 3 targets.
Since nums\[0\] is the minimal integer that can destroy 3 targets, we return 1.
**Example 3:**
**Input:** nums = \[6,2,5\], space = 100
**Output:** 2
**Explanation:** Whatever initial seed we select, we can only destroy 1 target. The minimal seed is nums\[1\].
**Constraints:**
* `1 <= nums.length <= 105`
* `1 <= nums[i] <= 109`
* `1 <= space <= 109` | null |
Python, use a Counter (almost always) | destroy-sequential-targets | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nAs the hint suggests, the first step is to calculate for all ```nums[i]``` the value ```nums[i] % space```; let\'s refer to this as ```mod_num[i]```. It turns out that ```nums``` that go into the same "kill group" (the subset of ```nums``` that could be killed by the same "seed") have the same ```mod_num[i]```.\n\nWe want the *maximum* kill group. So, for each possible value of ```mod_num[i]```, we want to count how many ```nums``` have that value, and select the ```mod_num[i]``` that appears most frequently. But, we want the *minimal* seed, the smallest value in ```nums``` that maps into the most frequently. To get this, we have to recover all the ```nums``` that map into the maximal ```mod_num``` and then take the minimum one.\n\nOne further elaboration: In some cases, there will be *multiple* maximum kill groups. In that case, we calculate the minimal seed for each of the kill groups and take the minimum of *those*.\n# Approach\n<!-- Describe your approach to solving the problem. -->\nSo, how to implement all the stuff described above? For me, the answer was easy: Use Python\'s nifty ```Counter``` class, in conjunction with the ```Counter``` method ```most_common```. The statement\n``` \nmod_nums_counts = Counter( mod_nums ).most_common\n```\ntakes the ```mod_nums``` we\'ve calculated and determines how many of each value in ```mod_nums``` there are. Specifically, it produces a structure like:\n```\n( ( mod_num_A, count(mod_num_A) ), ( mod_num_B, count( mod_num_B ), ... ) )\n```\nThis provides us with most of the required information (which ```mod_num```(s) is/are most frequent). Then it\'s a matter of Pythonery to loop over the structure above, and for each, using a *list comprehension* to map back to the original ```nums```. \n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\nThe first statement in the program loops over all elements in ```nums``` and thus is $$O(n)$$ where n is the number of ```nums```.\nThe ```for``` loop executes once per kill group. As we can see in the third Example, it\'s possible that all the kill groups are singletons, in which case *that* loop is $$O(n)$$. One additional factor: lurking in the ```for``` loop, there is a loop comprehension, and the effect is that the overall time complexity is $$O(n*k)$$ where $$n$$ is the number of ```nums``` and $$k$$ is the number of kill groups, so worst case, $$O(n^2)$$. In practice, for the test cases, this worst case didn\'t appear much, if at all.\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\nThere are three non-scalar variables (not counting input): ```mod_nums```, which is $$O(n)$$ in storage, ```mod_nums_counts``` , which is $$O(k)$$ and ```nums_with_mod_count```, which is also $$O(n)$$ for an overall space complexity of $$O(n)$$.\n# Code\n```\nclass Solution:\n def destroyTargets(self, nums: List[int], space: int) -> int:\n mod_nums = [ num % space for num in nums ]\n mod_nums_counts = Counter( mod_nums ).most_common()\n max_count = mod_nums_counts[ 0 ][ 1 ]\n min_num = math.inf\n for mod_num, count in mod_nums_counts:\n if count < max_count:\n break\n # OK, so "mod_num" is one of the most frequent (maximum number of\n # targets destroyed). If there is more than one such "mod_num",\n # select the one whose "count bucket" contains the smallest value:\n nums_with_mod_count = [ num for num in nums if num % space == mod_num ]\n this_min_num = min( nums_with_mod_count )\n min_num = min( min_num, this_min_num )\n return min_num\n``` | 0 | You are given a **0-indexed** array `nums` consisting of positive integers, representing targets on a number line. You are also given an integer `space`.
You have a machine which can destroy targets. **Seeding** the machine with some `nums[i]` allows it to destroy all targets with values that can be represented as `nums[i] + c * space`, where `c` is any non-negative integer. You want to destroy the **maximum** number of targets in `nums`.
Return _the **minimum value** of_ `nums[i]` _you can seed the machine with to destroy the maximum number of targets._
**Example 1:**
**Input:** nums = \[3,7,8,1,1,5\], space = 2
**Output:** 1
**Explanation:** If we seed the machine with nums\[3\], then we destroy all targets equal to 1,3,5,7,9,...
In this case, we would destroy 5 total targets (all except for nums\[2\]).
It is impossible to destroy more than 5 targets, so we return nums\[3\].
**Example 2:**
**Input:** nums = \[1,3,5,2,4,6\], space = 2
**Output:** 1
**Explanation:** Seeding the machine with nums\[0\], or nums\[3\] destroys 3 targets.
It is not possible to destroy more than 3 targets.
Since nums\[0\] is the minimal integer that can destroy 3 targets, we return 1.
**Example 3:**
**Input:** nums = \[6,2,5\], space = 100
**Output:** 2
**Explanation:** Whatever initial seed we select, we can only destroy 1 target. The minimal seed is nums\[1\].
**Constraints:**
* `1 <= nums.length <= 105`
* `1 <= nums[i] <= 109`
* `1 <= space <= 109` | null |
Python | HashMap Solution| O(n) | destroy-sequential-targets | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nKeeping track of remainders is the key to this problem.\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\nProblem can be divided into following cases:\n1. len(nums) == 1: only 1 value then return it as seed value\n2. find max(nums), if space >= max(nums) then num in nums cannot be divided by `space`, hence in this case simply return number which occurs the most in the array as seed value.\n3. Here space < max(nums): \n 1. keep track of remainders count as well as min_seed corresponding to that remainder in a dict(hash map)\n 2. get min_seed with max remainder freq.\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\nO(n)\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\nO(n)\n\n# Code\n```\nclass Solution:\n \'\'\'\n [3,5,8,9,7,1,3] : \n What is the maximum length of array? : 1 to 10^5\n What is the range of numbers?: 1 to 10^9\n Are the numbers unique? or duplicates are allowed? : non-unique/ follow-up: does it matter?\n\n space : integer : range? : 1 to 10^9\n\n problem: find max number of numbers in nums array that can be destroyed?\n destroy func: if seed + c*space == num : destroy it\n Find seed value: it should be one of num in nums (minimum)\n\n Cases: \n 1: L = 1: -> seed = nums[0]\n 2: space >= max(nums) : seed = num with max freq in nums\n 3: space < max(nums): \n [1,3,5,2,4,6] --> [1,1,1,0,0,0]\n {1:(3, 1), 0:(3,2)} -> get min of maxcount items\n max_count = 0\n min_seed = inf\n for key, val in store.items():\n count, seed = val\n if count > max_count:\n max_count = count\n min_seed = seed\n elif count == max_count:\n min_seed = min(min_seed, seed)\n 4: space < min(nums):\n [3,5,4,6] space = 2\n [1,1,0,0]\n\n \'\'\'\n def destroyTargets(self, nums: List[int], space: int) -> int:\n n = len(nums)\n if n == 1: return nums[0]\n max_ = max(nums)\n\n if space >= max_:\n freq = defaultdict(int)\n for num in nums:\n freq[num] += 1\n max_freq = 0\n target = -1\n for key, val in freq.items():\n if val > max_freq:\n max_freq = val\n target = key\n elif val == max_freq:\n if key < target:\n target = key\n return target\n \n store = {}\n\n for num in nums:\n r = num%space\n if r not in store:\n store[r] = (1, num)\n else:\n count, min_seed = store[r]\n store[r] = (count+1, min(min_seed, num))\n \n max_count = 0\n min_seed = inf\n for key, val in store.items():\n count, seed = val\n if count > max_count:\n max_count = count\n min_seed = seed\n elif count == max_count:\n min_seed = min(min_seed, seed)\n \n return min_seed\n \n \n``` | 0 | You are given a **0-indexed** array `nums` consisting of positive integers, representing targets on a number line. You are also given an integer `space`.
You have a machine which can destroy targets. **Seeding** the machine with some `nums[i]` allows it to destroy all targets with values that can be represented as `nums[i] + c * space`, where `c` is any non-negative integer. You want to destroy the **maximum** number of targets in `nums`.
Return _the **minimum value** of_ `nums[i]` _you can seed the machine with to destroy the maximum number of targets._
**Example 1:**
**Input:** nums = \[3,7,8,1,1,5\], space = 2
**Output:** 1
**Explanation:** If we seed the machine with nums\[3\], then we destroy all targets equal to 1,3,5,7,9,...
In this case, we would destroy 5 total targets (all except for nums\[2\]).
It is impossible to destroy more than 5 targets, so we return nums\[3\].
**Example 2:**
**Input:** nums = \[1,3,5,2,4,6\], space = 2
**Output:** 1
**Explanation:** Seeding the machine with nums\[0\], or nums\[3\] destroys 3 targets.
It is not possible to destroy more than 3 targets.
Since nums\[0\] is the minimal integer that can destroy 3 targets, we return 1.
**Example 3:**
**Input:** nums = \[6,2,5\], space = 100
**Output:** 2
**Explanation:** Whatever initial seed we select, we can only destroy 1 target. The minimal seed is nums\[1\].
**Constraints:**
* `1 <= nums.length <= 105`
* `1 <= nums[i] <= 109`
* `1 <= space <= 109` | null |
[Python3] intermediate stack | next-greater-element-iv | 0 | 1 | Please pull this [commit](https://github.com/gaosanyong/leetcode/commit/a90ca3f9de0f99297262514c111f27700c24c04a) for solutions of biweekly 90. \n\n```\nclass Solution:\n def secondGreaterElement(self, nums: List[int]) -> List[int]:\n ans = [-1] * len(nums)\n s, ss = [], []\n for i, x in enumerate(nums): \n while ss and nums[ss[-1]] < x: ans[ss.pop()] = x\n buff = []\n while s and nums[s[-1]] < x: buff.append(s.pop())\n while buff: ss.append(buff.pop())\n s.append(i)\n return ans \n``` | 1 | You are given a **0-indexed** array of non-negative integers `nums`. For each integer in `nums`, you must find its respective **second greater** integer.
The **second greater** integer of `nums[i]` is `nums[j]` such that:
* `j > i`
* `nums[j] > nums[i]`
* There exists **exactly one** index `k` such that `nums[k] > nums[i]` and `i < k < j`.
If there is no such `nums[j]`, the second greater integer is considered to be `-1`.
* For example, in the array `[1, 2, 4, 3]`, the second greater integer of `1` is `4`, `2` is `3`, and that of `3` and `4` is `-1`.
Return _an integer array_ `answer`_, where_ `answer[i]` _is the second greater integer of_ `nums[i]`_._
**Example 1:**
**Input:** nums = \[2,4,0,9,6\]
**Output:** \[9,6,6,-1,-1\]
**Explanation:**
0th index: 4 is the first integer greater than 2, and 9 is the second integer greater than 2, to the right of 2.
1st index: 9 is the first, and 6 is the second integer greater than 4, to the right of 4.
2nd index: 9 is the first, and 6 is the second integer greater than 0, to the right of 0.
3rd index: There is no integer greater than 9 to its right, so the second greater integer is considered to be -1.
4th index: There is no integer greater than 6 to its right, so the second greater integer is considered to be -1.
Thus, we return \[9,6,6,-1,-1\].
**Example 2:**
**Input:** nums = \[3,3\]
**Output:** \[-1,-1\]
**Explanation:**
We return \[-1,-1\] since neither integer has any integer greater than it.
**Constraints:**
* `1 <= nums.length <= 105`
* `0 <= nums[i] <= 109` | null |
Python3 (First ,Helper and Second stacks) || O(n) || 100% Faster | next-greater-element-iv | 0 | 1 | \n```\nclass Solution:\n def secondGreaterElement(self, nums: List[int]) -> List[int]:\n st1 = [] # monotonic decreasing first stack.\n st2 = [] # Monotonic decreasing second stack.(having only that elements which have first greater)\n helper = [] # monotonic increasing helper stack(help in moving elements from st1 to st2 with maintaining monotonicity of st2) \n n = len(nums)\n ans =[-1]*n\n for i in range(n):\n while st2 and nums[i]>nums[st2[-1]]: # if element can be second largest for st2 elements\n ans[st2.pop()] = nums[i]\n while st1 and nums[i]>nums[st1[-1]]: # if element can be second largest for st2 elements\n helper.append(st1.pop())\n while helper:\n st2.append(helper.pop()) # for maintain monotonicity of st2\n st1.append(i)\n return ans \n```\n**If like , Upvote :)**\n\n\n | 2 | You are given a **0-indexed** array of non-negative integers `nums`. For each integer in `nums`, you must find its respective **second greater** integer.
The **second greater** integer of `nums[i]` is `nums[j]` such that:
* `j > i`
* `nums[j] > nums[i]`
* There exists **exactly one** index `k` such that `nums[k] > nums[i]` and `i < k < j`.
If there is no such `nums[j]`, the second greater integer is considered to be `-1`.
* For example, in the array `[1, 2, 4, 3]`, the second greater integer of `1` is `4`, `2` is `3`, and that of `3` and `4` is `-1`.
Return _an integer array_ `answer`_, where_ `answer[i]` _is the second greater integer of_ `nums[i]`_._
**Example 1:**
**Input:** nums = \[2,4,0,9,6\]
**Output:** \[9,6,6,-1,-1\]
**Explanation:**
0th index: 4 is the first integer greater than 2, and 9 is the second integer greater than 2, to the right of 2.
1st index: 9 is the first, and 6 is the second integer greater than 4, to the right of 4.
2nd index: 9 is the first, and 6 is the second integer greater than 0, to the right of 0.
3rd index: There is no integer greater than 9 to its right, so the second greater integer is considered to be -1.
4th index: There is no integer greater than 6 to its right, so the second greater integer is considered to be -1.
Thus, we return \[9,6,6,-1,-1\].
**Example 2:**
**Input:** nums = \[3,3\]
**Output:** \[-1,-1\]
**Explanation:**
We return \[-1,-1\] since neither integer has any integer greater than it.
**Constraints:**
* `1 <= nums.length <= 105`
* `0 <= nums[i] <= 109` | null |
Python beats 100% | next-greater-element-iv | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nJust hold another stack with those already having 1 successor.\nRememeber to reverse the order\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def secondGreaterElement(self, nums: List[int]) -> List[int]:\n n = len(nums)\n res = [-1]*(n)\n # t = [-1]*n\n l = nums+nums\n st = []\n st2 = []\n for i in range(0, n):\n # print(st, st2)\n while len(st2)>0 and l[st2[-1]]<l[i]:\n res[st2.pop()]=l[i]\n temp=[]\n while len(st)>0 and l[st[-1]]<l[i]:\n # res[st.pop()]=l[i]\n # r = \n temp.append(st.pop())\n # st2.append(st.pop())\n # t[r]=l[i]\n # for i in reversed(temp):\n # st2.append(i)\n st2.extend(temp[::-1])\n st.append(i)\n # print(t)\n # print(res)\n return res\n``` | 0 | You are given a **0-indexed** array of non-negative integers `nums`. For each integer in `nums`, you must find its respective **second greater** integer.
The **second greater** integer of `nums[i]` is `nums[j]` such that:
* `j > i`
* `nums[j] > nums[i]`
* There exists **exactly one** index `k` such that `nums[k] > nums[i]` and `i < k < j`.
If there is no such `nums[j]`, the second greater integer is considered to be `-1`.
* For example, in the array `[1, 2, 4, 3]`, the second greater integer of `1` is `4`, `2` is `3`, and that of `3` and `4` is `-1`.
Return _an integer array_ `answer`_, where_ `answer[i]` _is the second greater integer of_ `nums[i]`_._
**Example 1:**
**Input:** nums = \[2,4,0,9,6\]
**Output:** \[9,6,6,-1,-1\]
**Explanation:**
0th index: 4 is the first integer greater than 2, and 9 is the second integer greater than 2, to the right of 2.
1st index: 9 is the first, and 6 is the second integer greater than 4, to the right of 4.
2nd index: 9 is the first, and 6 is the second integer greater than 0, to the right of 0.
3rd index: There is no integer greater than 9 to its right, so the second greater integer is considered to be -1.
4th index: There is no integer greater than 6 to its right, so the second greater integer is considered to be -1.
Thus, we return \[9,6,6,-1,-1\].
**Example 2:**
**Input:** nums = \[3,3\]
**Output:** \[-1,-1\]
**Explanation:**
We return \[-1,-1\] since neither integer has any integer greater than it.
**Constraints:**
* `1 <= nums.length <= 105`
* `0 <= nums[i] <= 109` | null |
mono stack + minheap python3 concise solution | next-greater-element-iv | 0 | 1 | # Intuition\nmono stack to find the first larger\nminheap to find the second larger\n\n# Approach\nBuild a mono stack to find the \'first larger\' of each num, while poping smaller nums off the stack, push them onto a minheap. Then before pushing any number onto the stack, try to compare it with the numbers in the heap, if the current number is greater than the numbers in heap, this number must be the second larger because a). we\'ve found the first large if a number is in the heap; b). we are processing the numbers one by one from left to right, so the number we found after the first larger must be the second larger\n\n# Complexity\n- Time complexity:\n$$O(n)$$\n\n- Space complexity:\n$$O(n)$$\n\n# Code\n```\nfrom heapq import heappush, heappop\n\nclass Solution:\n def secondGreaterElement(self, nums: List[int]) -> List[int]:\n stack = []\n minheap = []\n answers = [-1] * len(nums)\n\n for index, num in enumerate(nums):\n while minheap and minheap[0][0] < num:\n _value, heaptopi = heappop(minheap)\n answers[heaptopi] = num\n\n while stack and num > nums[stack[-1]]:\n stacktopi = stack.pop()\n heappush(minheap, (nums[stacktopi], stacktopi))\n \n stack.append(index)\n\n return answers\n\n \n\n \n\n\n\n\n\n\n\n\n\n\n\n\n\n\n\n\n\n\n\n\n\n``` | 0 | You are given a **0-indexed** array of non-negative integers `nums`. For each integer in `nums`, you must find its respective **second greater** integer.
The **second greater** integer of `nums[i]` is `nums[j]` such that:
* `j > i`
* `nums[j] > nums[i]`
* There exists **exactly one** index `k` such that `nums[k] > nums[i]` and `i < k < j`.
If there is no such `nums[j]`, the second greater integer is considered to be `-1`.
* For example, in the array `[1, 2, 4, 3]`, the second greater integer of `1` is `4`, `2` is `3`, and that of `3` and `4` is `-1`.
Return _an integer array_ `answer`_, where_ `answer[i]` _is the second greater integer of_ `nums[i]`_._
**Example 1:**
**Input:** nums = \[2,4,0,9,6\]
**Output:** \[9,6,6,-1,-1\]
**Explanation:**
0th index: 4 is the first integer greater than 2, and 9 is the second integer greater than 2, to the right of 2.
1st index: 9 is the first, and 6 is the second integer greater than 4, to the right of 4.
2nd index: 9 is the first, and 6 is the second integer greater than 0, to the right of 0.
3rd index: There is no integer greater than 9 to its right, so the second greater integer is considered to be -1.
4th index: There is no integer greater than 6 to its right, so the second greater integer is considered to be -1.
Thus, we return \[9,6,6,-1,-1\].
**Example 2:**
**Input:** nums = \[3,3\]
**Output:** \[-1,-1\]
**Explanation:**
We return \[-1,-1\] since neither integer has any integer greater than it.
**Constraints:**
* `1 <= nums.length <= 105`
* `0 <= nums[i] <= 109` | null |
Easy Python Solution | average-value-of-even-numbers-that-are-divisible-by-three | 0 | 1 | # Code\n```\nclass Solution:\n def averageValue(self, nums: List[int]) -> int:\n l=[]\n for i in nums:\n if i%6==0:\n l.append(i)\n return sum(l)//len(l) if len(l)>0 else 0\n``` | 2 | Given an integer array `nums` of **positive** integers, return _the average value of all even integers that are divisible by_ `3`_._
Note that the **average** of `n` elements is the **sum** of the `n` elements divided by `n` and **rounded down** to the nearest integer.
**Example 1:**
**Input:** nums = \[1,3,6,10,12,15\]
**Output:** 9
**Explanation:** 6 and 12 are even numbers that are divisible by 3. (6 + 12) / 2 = 9.
**Example 2:**
**Input:** nums = \[1,2,4,7,10\]
**Output:** 0
**Explanation:** There is no single number that satisfies the requirement, so return 0.
**Constraints:**
* `1 <= nums.length <= 1000`
* `1 <= nums[i] <= 1000` | null |
Python Super Simple 2-Lines Pythonic Solution | average-value-of-even-numbers-that-are-divisible-by-three | 0 | 1 | # Approach\nFirst we filter all the even numbers that are divisible by three.\nThen we calculate their average.\n\n# Code\n```\nclass Solution:\n def averageValue(self, nums: List[int]) -> int:\n res = [a for a in nums if ((a % 2 == 0) and (a % 3 == 0))]\n return 0 if len(res) == 0 else sum(res) // len(res)\n``` | 1 | Given an integer array `nums` of **positive** integers, return _the average value of all even integers that are divisible by_ `3`_._
Note that the **average** of `n` elements is the **sum** of the `n` elements divided by `n` and **rounded down** to the nearest integer.
**Example 1:**
**Input:** nums = \[1,3,6,10,12,15\]
**Output:** 9
**Explanation:** 6 and 12 are even numbers that are divisible by 3. (6 + 12) / 2 = 9.
**Example 2:**
**Input:** nums = \[1,2,4,7,10\]
**Output:** 0
**Explanation:** There is no single number that satisfies the requirement, so return 0.
**Constraints:**
* `1 <= nums.length <= 1000`
* `1 <= nums[i] <= 1000` | null |
Easiest Python Solution || With Explanation ✔ | average-value-of-even-numbers-that-are-divisible-by-three | 0 | 1 | Here is my solution in Python:-\n```\nclass Solution:\n def averageValue(self, nums: List[int]) -> int:\n ans=0 # ans will store the sum of elements which are even and divisible by 3; \n cnt=0 # cnt will store the number of elements which are even and divisible by 3; \n\t\t\t\t\t\t\t\t\t\t \n for ele in nums:\n\t\t# Elements which are divisible by 3 and are even simply means **It must be divisible by 6** So we are checking that in the loop\n\t\t# we are adding it to ans if it is divisible by 6 and increase cnt by 1;\n if (ele%6==0):\n ans+=ele;\n cnt+=1;\n if (cnt==0):\n return 0; # if no element is found return 0;\n return (floor(ans/cnt)); # else return the floor value ofaverage that is sum of elements divided by no. of elements\n\n``` | 1 | Given an integer array `nums` of **positive** integers, return _the average value of all even integers that are divisible by_ `3`_._
Note that the **average** of `n` elements is the **sum** of the `n` elements divided by `n` and **rounded down** to the nearest integer.
**Example 1:**
**Input:** nums = \[1,3,6,10,12,15\]
**Output:** 9
**Explanation:** 6 and 12 are even numbers that are divisible by 3. (6 + 12) / 2 = 9.
**Example 2:**
**Input:** nums = \[1,2,4,7,10\]
**Output:** 0
**Explanation:** There is no single number that satisfies the requirement, so return 0.
**Constraints:**
* `1 <= nums.length <= 1000`
* `1 <= nums[i] <= 1000` | null |
Python one pass O(n) | average-value-of-even-numbers-that-are-divisible-by-three | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nFrom the question, we know we need to check which numbers are both even and divisible by three.\n# Approach\n<!-- Describe your approach to solving the problem. -->\nLoop through nums, check what fits reqs, add it to array. If the array has anything in it, divide the sum by the length, else just return 0.\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\nO(n)\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\nI think it\'s also O(n)? I\'d really appreciate it if someone could clarify.\n# Code\n```\nclass Solution:\n def averageValue(self, nums: List[int]) -> int:\n _ans =[]\n for i in nums:\n if (i%2==0) and (i%3==0):\n _ans.append(i)\n return sum(_ans)//len(_ans) if len(_ans) > 0 else 0\n\n``` | 1 | Given an integer array `nums` of **positive** integers, return _the average value of all even integers that are divisible by_ `3`_._
Note that the **average** of `n` elements is the **sum** of the `n` elements divided by `n` and **rounded down** to the nearest integer.
**Example 1:**
**Input:** nums = \[1,3,6,10,12,15\]
**Output:** 9
**Explanation:** 6 and 12 are even numbers that are divisible by 3. (6 + 12) / 2 = 9.
**Example 2:**
**Input:** nums = \[1,2,4,7,10\]
**Output:** 0
**Explanation:** There is no single number that satisfies the requirement, so return 0.
**Constraints:**
* `1 <= nums.length <= 1000`
* `1 <= nums[i] <= 1000` | null |
Python | Easy Solution✅ | average-value-of-even-numbers-that-are-divisible-by-three | 0 | 1 | # Code\u2705\n```\nclass Solution:\n def averageValue(self, nums: List[int]) -> int:\n nums = list(filter(lambda x: x % 2 == 0 and x % 3 == 0, nums)) # list(filter(lambda x: x % 6 == 0, nums)) will also works\n return 0 if not len(nums) else sum(nums)//len(nums)\n``` | 4 | Given an integer array `nums` of **positive** integers, return _the average value of all even integers that are divisible by_ `3`_._
Note that the **average** of `n` elements is the **sum** of the `n` elements divided by `n` and **rounded down** to the nearest integer.
**Example 1:**
**Input:** nums = \[1,3,6,10,12,15\]
**Output:** 9
**Explanation:** 6 and 12 are even numbers that are divisible by 3. (6 + 12) / 2 = 9.
**Example 2:**
**Input:** nums = \[1,2,4,7,10\]
**Output:** 0
**Explanation:** There is no single number that satisfies the requirement, so return 0.
**Constraints:**
* `1 <= nums.length <= 1000`
* `1 <= nums[i] <= 1000` | null |
Python simple two lines solution | average-value-of-even-numbers-that-are-divisible-by-three | 0 | 1 | # Approach\nLeave in the array only the even elements that divided to 3.\nCalaulate the average of the array.\n\n\n# Code\n```\nclass Solution:\n def averageValue(self, nums: List[int]) -> int:\n nums = [i for i in nums if not i % 3 and not i % 2]\n return 0 if not len(nums) else (sum(nums) // len(nums))\n```\n\nLike it ? Please upvote! | 3 | Given an integer array `nums` of **positive** integers, return _the average value of all even integers that are divisible by_ `3`_._
Note that the **average** of `n` elements is the **sum** of the `n` elements divided by `n` and **rounded down** to the nearest integer.
**Example 1:**
**Input:** nums = \[1,3,6,10,12,15\]
**Output:** 9
**Explanation:** 6 and 12 are even numbers that are divisible by 3. (6 + 12) / 2 = 9.
**Example 2:**
**Input:** nums = \[1,2,4,7,10\]
**Output:** 0
**Explanation:** There is no single number that satisfies the requirement, so return 0.
**Constraints:**
* `1 <= nums.length <= 1000`
* `1 <= nums[i] <= 1000` | null |
Average Value of Even Numbers Divisible by Three | average-value-of-even-numbers-that-are-divisible-by-three | 0 | 1 | \n# Intuition\nThe task is to find the average value of even numbers in a list that are divisible by three. The solution iterates through each element in the input list, identifies even numbers divisible by three, and calculates their average. The check for an empty list is included to handle the case where no qualifying numbers are found. This straightforward approach ensures that the result is rounded down to the nearest integer, as specified in the problem.\n\n\n# Approach\n1. Initialize variables `even_num` and `cnt` to 0. These will be used to accumulate the sum and count of qualifying even numbers, respectively.\n2. Iterate through each element `i` in the input list `nums`.\n - Check if `i` is both even and divisible by three (`i % 3 == 0` and `i % 2 == 0`).\n - If true, add `i` to the `even_num` sum and increment the count `cnt`.\n3. Check if the count `cnt` is not zero to avoid division by zero.\n - If not zero, calculate the average by dividing the sum `even_num` by the count `cnt`.\n - Return the rounded-down integer value of the average.\n4. If the count `cnt` is zero, return 0, indicating that there are no qualifying numbers in the list.\n\n\n# Complexity\n-Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n- The code iterates through each element in the input list nums exactly once.\n- Inside the loop, constant-time operations are performed (conditional checks and additions).\n- Therefore, the time complexity is O(n), where n is the length of the input list.\n\n-Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n- The space complexity is O(1) because the space used by the algorithm remains constant regardless of the input size.\n- The algorithm uses a fixed amount of additional space to store even_num and cnt.\n\n# Code\n```\nclass Solution:\n def averageValue(self, nums: List[int]) -> int:\n even_num =0\n cnt=0\n for i in nums:\n if i % 3 == 0 and i % 2 == 0:\n even_num +=i\n cnt+=1\n\n # Check if even_num is empty to avoid division by zero\n if even_num:\n return int(even_num / cnt)\n else:\n return 0\n``` | 0 | Given an integer array `nums` of **positive** integers, return _the average value of all even integers that are divisible by_ `3`_._
Note that the **average** of `n` elements is the **sum** of the `n` elements divided by `n` and **rounded down** to the nearest integer.
**Example 1:**
**Input:** nums = \[1,3,6,10,12,15\]
**Output:** 9
**Explanation:** 6 and 12 are even numbers that are divisible by 3. (6 + 12) / 2 = 9.
**Example 2:**
**Input:** nums = \[1,2,4,7,10\]
**Output:** 0
**Explanation:** There is no single number that satisfies the requirement, so return 0.
**Constraints:**
* `1 <= nums.length <= 1000`
* `1 <= nums[i] <= 1000` | null |
[ Python ]🐍🐍Simple Python Solution ✅✅ | average-value-of-even-numbers-that-are-divisible-by-three | 0 | 1 | ```\nclass Solution:\n def averageValue(self, nums: List[int]) -> int:\n s=0\n k=0\n for i in nums:\n if i%6==0:\n k+=1\n s+=i\n \n if k==0:\n return 0\n else:\n return(s//k)\n``` | 2 | Given an integer array `nums` of **positive** integers, return _the average value of all even integers that are divisible by_ `3`_._
Note that the **average** of `n` elements is the **sum** of the `n` elements divided by `n` and **rounded down** to the nearest integer.
**Example 1:**
**Input:** nums = \[1,3,6,10,12,15\]
**Output:** 9
**Explanation:** 6 and 12 are even numbers that are divisible by 3. (6 + 12) / 2 = 9.
**Example 2:**
**Input:** nums = \[1,2,4,7,10\]
**Output:** 0
**Explanation:** There is no single number that satisfies the requirement, so return 0.
**Constraints:**
* `1 <= nums.length <= 1000`
* `1 <= nums[i] <= 1000` | null |
pandas | most-popular-video-creator | 0 | 1 | Doesn\'t actually work since leetcode doesn\'t support pandas\n\n\n# Approach\nUse pandas\n\n# Code\n```\nimport pandas as pd \nimport numpy as np \nclass Solution:\n def mostPopularCreator(self, creators: List[str], ids: List[str], views: List[int]) -> List[List[str]]:\n df = pd.DataFrame({"creators": creators, "ids": ids, "views": views})\n view_df = df.groupby(by="creators").sum()["views"]\n max_count = view_df.max()\n popular_creators = set(view_df[view_df == max_count].index)\n \n view_gb = df.groupby(by="creators").max()["views"]\n df["max_view"] = [view_gb[name] for name in df["creators"]]\n df["most_popular"] = df["creators"].apply(lambda x: x in popular_creators)\n \n answer_df = df[(df["max_view"] == df["views"]) & df["most_popular"]]\n answer_gb = answer_df.groupby("creators").min()["ids"]\n return list(zip(answer_gb.index, answer_gb))\n \n \n \n \n``` | 1 | You are given two string arrays `creators` and `ids`, and an integer array `views`, all of length `n`. The `ith` video on a platform was created by `creator[i]`, has an id of `ids[i]`, and has `views[i]` views.
The **popularity** of a creator is the **sum** of the number of views on **all** of the creator's videos. Find the creator with the **highest** popularity and the id of their **most** viewed video.
* If multiple creators have the highest popularity, find all of them.
* If multiple videos have the highest view count for a creator, find the lexicographically **smallest** id.
Return _a 2D array of strings_ `answer` _where_ `answer[i] = [creatori, idi]` _means that_ `creatori` _has the **highest** popularity and_ `idi` _is the id of their most popular video._ The answer can be returned in any order.
**Example 1:**
**Input:** creators = \[ "alice ", "bob ", "alice ", "chris "\], ids = \[ "one ", "two ", "three ", "four "\], views = \[5,10,5,4\]
**Output:** \[\[ "alice ", "one "\],\[ "bob ", "two "\]\]
**Explanation:**
The popularity of alice is 5 + 5 = 10.
The popularity of bob is 10.
The popularity of chris is 4.
alice and bob are the most popular creators.
For bob, the video with the highest view count is "two ".
For alice, the videos with the highest view count are "one " and "three ". Since "one " is lexicographically smaller than "three ", it is included in the answer.
**Example 2:**
**Input:** creators = \[ "alice ", "alice ", "alice "\], ids = \[ "a ", "b ", "c "\], views = \[1,2,2\]
**Output:** \[\[ "alice ", "b "\]\]
**Explanation:**
The videos with id "b " and "c " have the highest view count.
Since "b " is lexicographically smaller than "c ", it is included in the answer.
**Constraints:**
* `n == creators.length == ids.length == views.length`
* `1 <= n <= 105`
* `1 <= creators[i].length, ids[i].length <= 5`
* `creators[i]` and `ids[i]` consist only of lowercase English letters.
* `0 <= views[i] <= 105` | null |
Python Hashmap solution | No Sorting | most-popular-video-creator | 0 | 1 | Approach: \nI have declared a hashmap to collect the following values,\n1. total number current views for the creator\n2. store the lexicographic id of the creator\'s popular video\n3. current popular view of the creator\n\nSo my final hashmap for the given basic test case looks like {\'alice\': [10, \'one\', 5], \'bob\': [10, \'two\', 10], \'chris\': [4, \'four\', 4]}\nmemo["alice"][0] = total number of views for alice\nmemo["alice"][1] = min lexicographic id for alice\'s popular video\nmemo["alice"][2] = max popular video views for alice\n\nAlongside with these, I have also initiated overall_max_popular_video_count to track the max_total_number_of_views for a creator\nOnce we collect all the above mentioned values,\nI compare each and every creator\'s total number of views with the current_popular_video_count and make my result array.\nThanks for reading!\n\n```\nclass Solution:\n def mostPopularCreator(self, creators: List[str], ids: List[str], views: List[int]) -> List[List[str]]:\n memo = {}\n\t\t#tracking the max popular video count\n overall_max_popular_video_count = -1\n #looping over the creators\n for i in range(len(creators)):\n if creators[i] in memo:\n #Step 1: update number of views for the creator\n memo[creators[i]][0] += views[i]\n #Step 2: update current_popular_video_view and id_of_most_popular_video_so_far\n if memo[creators[i]][2] < views[i]:\n memo[creators[i]][1] = ids[i]\n memo[creators[i]][2] = views[i]\n #Step 2a: finding the lexicographically smallest id as we hit the current_popularity_video_view again!\n elif memo[creators[i]][2] == views[i]:\n memo[creators[i]][1] = min(memo[creators[i]][1],ids[i])\n else:\n\t\t\t#adding new entry to our memo\n\t\t\t#new entry is of the format memo[creator[i]] = [total number current views for the creator, store the lexicographic id of the popular video, current popular view of the creator]\n memo[creators[i]] = [views[i],ids[i],views[i]]\n\t\t\t#track the max popular video count\n overall_max_popular_video_count = max(memo[creators[i]][0],overall_max_popular_video_count)\n \n result = []\n for i in memo:\n if memo[i][0] == overall_max_popular_video_count:\n result.append([i,memo[i][1]])\n return result\n``` | 6 | You are given two string arrays `creators` and `ids`, and an integer array `views`, all of length `n`. The `ith` video on a platform was created by `creator[i]`, has an id of `ids[i]`, and has `views[i]` views.
The **popularity** of a creator is the **sum** of the number of views on **all** of the creator's videos. Find the creator with the **highest** popularity and the id of their **most** viewed video.
* If multiple creators have the highest popularity, find all of them.
* If multiple videos have the highest view count for a creator, find the lexicographically **smallest** id.
Return _a 2D array of strings_ `answer` _where_ `answer[i] = [creatori, idi]` _means that_ `creatori` _has the **highest** popularity and_ `idi` _is the id of their most popular video._ The answer can be returned in any order.
**Example 1:**
**Input:** creators = \[ "alice ", "bob ", "alice ", "chris "\], ids = \[ "one ", "two ", "three ", "four "\], views = \[5,10,5,4\]
**Output:** \[\[ "alice ", "one "\],\[ "bob ", "two "\]\]
**Explanation:**
The popularity of alice is 5 + 5 = 10.
The popularity of bob is 10.
The popularity of chris is 4.
alice and bob are the most popular creators.
For bob, the video with the highest view count is "two ".
For alice, the videos with the highest view count are "one " and "three ". Since "one " is lexicographically smaller than "three ", it is included in the answer.
**Example 2:**
**Input:** creators = \[ "alice ", "alice ", "alice "\], ids = \[ "a ", "b ", "c "\], views = \[1,2,2\]
**Output:** \[\[ "alice ", "b "\]\]
**Explanation:**
The videos with id "b " and "c " have the highest view count.
Since "b " is lexicographically smaller than "c ", it is included in the answer.
**Constraints:**
* `n == creators.length == ids.length == views.length`
* `1 <= n <= 105`
* `1 <= creators[i].length, ids[i].length <= 5`
* `creators[i]` and `ids[i]` consist only of lowercase English letters.
* `0 <= views[i] <= 105` | null |
Easy Solution using Hash Table !! | most-popular-video-creator | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\nO(N)\n\n- Space complexity:\nO(N)\n\n# Code\n```\nclass Solution:\n def mostPopularCreator(self, creators: List[str], ids: List[str], views: List[int]) -> List[List[str]]:\n res={}\n total = -1\n for i in range(len(creators)):\n if creators[i] in res:\n res[creators[i]][0] += views[i]\n if res[creators[i]][2] < views[i]:\n res[creators[i]][1] = ids[i]\n res[creators[i]][2] = views[i]\n elif res[creators[i]][2] == views[i]:\n res[creators[i]][1] = min(res[creators[i]][1],ids[i])\n else:\n res[creators[i]] = [views[i],ids[i],views[i]]\n total = max(res[creators[i]][0],total)\n \n result = []\n for i in res:\n if res[i][0] == total:\n result.append([i,res[i][1]])\n return result\n``` | 1 | You are given two string arrays `creators` and `ids`, and an integer array `views`, all of length `n`. The `ith` video on a platform was created by `creator[i]`, has an id of `ids[i]`, and has `views[i]` views.
The **popularity** of a creator is the **sum** of the number of views on **all** of the creator's videos. Find the creator with the **highest** popularity and the id of their **most** viewed video.
* If multiple creators have the highest popularity, find all of them.
* If multiple videos have the highest view count for a creator, find the lexicographically **smallest** id.
Return _a 2D array of strings_ `answer` _where_ `answer[i] = [creatori, idi]` _means that_ `creatori` _has the **highest** popularity and_ `idi` _is the id of their most popular video._ The answer can be returned in any order.
**Example 1:**
**Input:** creators = \[ "alice ", "bob ", "alice ", "chris "\], ids = \[ "one ", "two ", "three ", "four "\], views = \[5,10,5,4\]
**Output:** \[\[ "alice ", "one "\],\[ "bob ", "two "\]\]
**Explanation:**
The popularity of alice is 5 + 5 = 10.
The popularity of bob is 10.
The popularity of chris is 4.
alice and bob are the most popular creators.
For bob, the video with the highest view count is "two ".
For alice, the videos with the highest view count are "one " and "three ". Since "one " is lexicographically smaller than "three ", it is included in the answer.
**Example 2:**
**Input:** creators = \[ "alice ", "alice ", "alice "\], ids = \[ "a ", "b ", "c "\], views = \[1,2,2\]
**Output:** \[\[ "alice ", "b "\]\]
**Explanation:**
The videos with id "b " and "c " have the highest view count.
Since "b " is lexicographically smaller than "c ", it is included in the answer.
**Constraints:**
* `n == creators.length == ids.length == views.length`
* `1 <= n <= 105`
* `1 <= creators[i].length, ids[i].length <= 5`
* `creators[i]` and `ids[i]` consist only of lowercase English letters.
* `0 <= views[i] <= 105` | null |
Python | With Example | Greedy | minimum-addition-to-make-integer-beautiful | 0 | 1 | ```\n\nclass Solution:\n def makeIntegerBeautiful(self, n: int, target: int) -> int:\n total = added = 0\n mask = 10\n while sum([int(c) for c in str(n)]) > target:\n added = mask - n % mask\n if added != mask:\n n += added\n total += added\n # print(\'n\',n,\'added\',added,\'mask\',mask)\n mask *= 10\n return total\n```\n\nExample:\n```\nn: 94598\ntarget: 6\n\nPrinted:\nn 94600 added 2 mask 10\nn 95000 added 400 mask 1000\nn 100000 added 5000 mask 10000\n\ntotal is 2 + 400 + 5000\n``` | 1 | You are given two positive integers `n` and `target`.
An integer is considered **beautiful** if the sum of its digits is less than or equal to `target`.
Return the _minimum **non-negative** integer_ `x` _such that_ `n + x` _is beautiful_. The input will be generated such that it is always possible to make `n` beautiful.
**Example 1:**
**Input:** n = 16, target = 6
**Output:** 4
**Explanation:** Initially n is 16 and its digit sum is 1 + 6 = 7. After adding 4, n becomes 20 and digit sum becomes 2 + 0 = 2. It can be shown that we can not make n beautiful with adding non-negative integer less than 4.
**Example 2:**
**Input:** n = 467, target = 6
**Output:** 33
**Explanation:** Initially n is 467 and its digit sum is 4 + 6 + 7 = 17. After adding 33, n becomes 500 and digit sum becomes 5 + 0 + 0 = 5. It can be shown that we can not make n beautiful with adding non-negative integer less than 33.
**Example 3:**
**Input:** n = 1, target = 1
**Output:** 0
**Explanation:** Initially n is 1 and its digit sum is 1, which is already smaller than or equal to target.
**Constraints:**
* `1 <= n <= 1012`
* `1 <= target <= 150`
* The input will be generated such that it is always possible to make `n` beautiful. | null |
Python 3 || 6 lines, w/example || T/M: 99% / 71% | minimum-addition-to-make-integer-beautiful | 0 | 1 | ```\nclass Solution:\n def makeIntegerBeautiful(self, n: int, target: int) -> int:\n\n sm = lambda n: sum(map(int,list(str(n))))\n\n zeros, diff = 10, 0 # Ex: n = 5617 ; target = 7\n\n while sm(n + diff) > target: # n zeros diff n+diff sm(n+diff)\n # ----- \u2013\u2013\u2013\u2013\u2013 \u2013\u2013\u2013\u2013\u2013 \u2013\u2013\u2013\u2013\u2013\u2013 \u2013\u2013\u2013\u2013\u2013\u2013\u2013\u2013\u2013 \n diff = zeros - n%zeros # 5617 10 3 5620 13 \n # 5617 100 83 5700 12\n zeros*= 10 # 5617 1000 383 6000 6 <-- less than target\n # |\n return diff # answer\n```\n[https://leetcode.com/submissions/detail/848785961/](http://)\n\n | 6 | You are given two positive integers `n` and `target`.
An integer is considered **beautiful** if the sum of its digits is less than or equal to `target`.
Return the _minimum **non-negative** integer_ `x` _such that_ `n + x` _is beautiful_. The input will be generated such that it is always possible to make `n` beautiful.
**Example 1:**
**Input:** n = 16, target = 6
**Output:** 4
**Explanation:** Initially n is 16 and its digit sum is 1 + 6 = 7. After adding 4, n becomes 20 and digit sum becomes 2 + 0 = 2. It can be shown that we can not make n beautiful with adding non-negative integer less than 4.
**Example 2:**
**Input:** n = 467, target = 6
**Output:** 33
**Explanation:** Initially n is 467 and its digit sum is 4 + 6 + 7 = 17. After adding 33, n becomes 500 and digit sum becomes 5 + 0 + 0 = 5. It can be shown that we can not make n beautiful with adding non-negative integer less than 33.
**Example 3:**
**Input:** n = 1, target = 1
**Output:** 0
**Explanation:** Initially n is 1 and its digit sum is 1, which is already smaller than or equal to target.
**Constraints:**
* `1 <= n <= 1012`
* `1 <= target <= 150`
* The input will be generated such that it is always possible to make `n` beautiful. | null |
Greedy Algorithm - O(log n) - Comprehensive Explanation | minimum-addition-to-make-integer-beautiful | 0 | 1 | # Intuition\nTo find the minimum number to add to an integer n such that the sum of its digits is no more than a target value, we can use a greedy approach. We will try to minimize the added value while ensuring that the sum of the digits does not exceed the target.\n\n# Approach\nThe approach involves iterating through the digits of the number from right to left (least significant to most significant digit). We will add the smallest value possible to the current digit, so the sum of the digits remains below the target. This process will continue until the sum of the digits reaches the target or we have processed all digits.\n\n# Algorithm\n1. Define a generator function digits that yields the digits of a given number n.\n2. Initialize variables multiplier and min_add to 1 and 0, respectively.\n3. While the sum of the digits of n + min_add is greater than target, perform the following:\n a. Multiply multiplier by 10.\n b. Update min_add to multiplier - n % multiplier.\n4. Return the value of min_add.\n\n# Proof\nThe greedy algorithm works because, at each step, we add the smallest possible value to n to get closer to the target sum of digits without exceeding it. By multiplying multiplier by 10 and updating min_add accordingly, we ensure that we\'re always considering the smallest possible value to add to n that would change the current digit. This approach guarantees that the added value is minimized.\n\n# Complexity\n - Time complexity: $$O(\\log{n})$$\nThe time complexity is determined by the number of digits in n. In each iteration of the while loop, we process one digit of n. Since there are $$O(\\log{n})$$ digits in n, the time complexity is $$O(\\log{n})$$.\n\n - Space complexity: $$O(1)$$\nThe space complexity is constant because we only use a few variables that do not depend on the input size.\n\n# Code\n```\nclass Solution:\n def makeIntegerBeautiful(self, n: int, target: int) -> int:\n def digits(n: int):\n while n > 0:\n yield n % 10\n n //= 10\n\n multiplier = 1\n min_add = 0\n while sum(digits(n + min_add)) > target:\n multiplier *= 10\n min_add = multiplier - n % multiplier\n \n return min_add\n``` | 1 | You are given two positive integers `n` and `target`.
An integer is considered **beautiful** if the sum of its digits is less than or equal to `target`.
Return the _minimum **non-negative** integer_ `x` _such that_ `n + x` _is beautiful_. The input will be generated such that it is always possible to make `n` beautiful.
**Example 1:**
**Input:** n = 16, target = 6
**Output:** 4
**Explanation:** Initially n is 16 and its digit sum is 1 + 6 = 7. After adding 4, n becomes 20 and digit sum becomes 2 + 0 = 2. It can be shown that we can not make n beautiful with adding non-negative integer less than 4.
**Example 2:**
**Input:** n = 467, target = 6
**Output:** 33
**Explanation:** Initially n is 467 and its digit sum is 4 + 6 + 7 = 17. After adding 33, n becomes 500 and digit sum becomes 5 + 0 + 0 = 5. It can be shown that we can not make n beautiful with adding non-negative integer less than 33.
**Example 3:**
**Input:** n = 1, target = 1
**Output:** 0
**Explanation:** Initially n is 1 and its digit sum is 1, which is already smaller than or equal to target.
**Constraints:**
* `1 <= n <= 1012`
* `1 <= target <= 150`
* The input will be generated such that it is always possible to make `n` beautiful. | null |
Check next 10, next 100, next 1000 and so on... | minimum-addition-to-make-integer-beautiful | 0 | 1 | e.g 467\nif 467 is not sufficient, we have to directly check 470 because till 470 sum will increase only.\nafter 470,check 500 beacuse till 500 sum will increase only\nthus we have to check next 10th base every time\n\n```\nclass Solution:\n def makeIntegerBeautiful(self, n: int, target: int) -> int:\n i=n\n l=1\n while i<=10**12:\n s=0\n for j in str(i):\n s+=int(j)\n if s<=target:\n return i-n\n i//=10**l\n i+=1\n i*=10**l\n l+=1\n``` | 3 | You are given two positive integers `n` and `target`.
An integer is considered **beautiful** if the sum of its digits is less than or equal to `target`.
Return the _minimum **non-negative** integer_ `x` _such that_ `n + x` _is beautiful_. The input will be generated such that it is always possible to make `n` beautiful.
**Example 1:**
**Input:** n = 16, target = 6
**Output:** 4
**Explanation:** Initially n is 16 and its digit sum is 1 + 6 = 7. After adding 4, n becomes 20 and digit sum becomes 2 + 0 = 2. It can be shown that we can not make n beautiful with adding non-negative integer less than 4.
**Example 2:**
**Input:** n = 467, target = 6
**Output:** 33
**Explanation:** Initially n is 467 and its digit sum is 4 + 6 + 7 = 17. After adding 33, n becomes 500 and digit sum becomes 5 + 0 + 0 = 5. It can be shown that we can not make n beautiful with adding non-negative integer less than 33.
**Example 3:**
**Input:** n = 1, target = 1
**Output:** 0
**Explanation:** Initially n is 1 and its digit sum is 1, which is already smaller than or equal to target.
**Constraints:**
* `1 <= n <= 1012`
* `1 <= target <= 150`
* The input will be generated such that it is always possible to make `n` beautiful. | null |
Simple math | minimum-addition-to-make-integer-beautiful | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution: \n def makeIntegerBeautiful(self, n: int, target: int) -> int:\n def sumofc(num):\n s = 0\n while num != 0:\n s += num % 10\n num = int(num/10)\n return s\n ans = 0\n tmp = n\n mult = 1\n\t\t#keep making last digit zero till sum of digits is less than target\n while sumofc(tmp) > target:\n lastn = tmp % 10\n tmp = int(tmp/10) + 1\n#making first digit of our answer\n ans = ans + mult * (10 - lastn)\n multp = mult * 10\n return ans \n``` | 1 | You are given two positive integers `n` and `target`.
An integer is considered **beautiful** if the sum of its digits is less than or equal to `target`.
Return the _minimum **non-negative** integer_ `x` _such that_ `n + x` _is beautiful_. The input will be generated such that it is always possible to make `n` beautiful.
**Example 1:**
**Input:** n = 16, target = 6
**Output:** 4
**Explanation:** Initially n is 16 and its digit sum is 1 + 6 = 7. After adding 4, n becomes 20 and digit sum becomes 2 + 0 = 2. It can be shown that we can not make n beautiful with adding non-negative integer less than 4.
**Example 2:**
**Input:** n = 467, target = 6
**Output:** 33
**Explanation:** Initially n is 467 and its digit sum is 4 + 6 + 7 = 17. After adding 33, n becomes 500 and digit sum becomes 5 + 0 + 0 = 5. It can be shown that we can not make n beautiful with adding non-negative integer less than 33.
**Example 3:**
**Input:** n = 1, target = 1
**Output:** 0
**Explanation:** Initially n is 1 and its digit sum is 1, which is already smaller than or equal to target.
**Constraints:**
* `1 <= n <= 1012`
* `1 <= target <= 150`
* The input will be generated such that it is always possible to make `n` beautiful. | null |
Segment Tree | O(nlogn) | height-of-binary-tree-after-subtree-removal-queries | 0 | 1 | # Approach\nDo a dfs traversal to append each depth (from root to leaf) to compute a list `depth`. \n\n`pre` and `post` determine the range of element in `depth` list so that all values inside this range are from path rooted at this node. \n\nFor each queries, we want to find out the max depth without choosing element from this range, so we use segment tree to query max depth before pre[node] and max depth after pre node, as well as the depth of the parent of this node.\n\n# Complexity\n- Time complexity: O(nlogn)\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: O(n)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\n# Definition for a binary tree node.\n# class TreeNode:\n# def __init__(self, val=0, left=None, right=None):\n# self.val = val\n# self.left = left\n# self.right = right\n\nclass SegmentTree:\n def __init__(self, n, fn): # fn is transformation function\n self.n = n\n self.fn = fn\n self.tree = [0] * (2 * self.n)\n \n def update(self, idx, val):\n idx += self.n\n \n self.tree[idx] = val\n while idx > 1:\n idx >>= 1\n self.tree[idx] = self.fn(self.tree[2 * idx], self.tree[2 * idx + 1])\n \n def query(self, lo, hi):\n lo += self.n\n hi += self.n + 1\n res = 0\n \n while lo < hi:\n if lo & 1 == 1:\n res = self.fn(res, self.tree[lo])\n lo += 1\n if hi & 1 == 1:\n hi -= 1\n res = self.fn(res, self.tree[hi])\n lo >>= 1\n hi >>= 1\n \n return res \n \nclass Solution:\n def treeQueries(self, root: Optional[TreeNode], queries: List[int]) -> List[int]:\n pre = defaultdict(int)\n post = defaultdict(int)\n height = defaultdict(int)\n depth = []\n \n def dfs(node, curr):\n if not node: return\n \n height[node.val] = curr\n pre[node.val] = len(depth)\n dfs(node.left, curr + 1)\n dfs(node.right, curr + 1)\n \n if len(depth) == pre[node.val]: # node is leaf\n post[node.val] = pre[node.val]\n depth.append(curr)\n else:\n post[node.val] = len(depth) - 1\n \n dfs(root, 0)\n seg = SegmentTree(len(depth), max)\n for i in range(len(depth)):\n seg.update(i, depth[i])\n \n res = []\n for q in queries:\n lo, hi = pre[q], post[q]\n \n d = 0\n if lo == 0 and hi == len(depth) - 1:\n res.append(height[q] - 1)\n elif lo == 0:\n res.append(max(seg.query(hi + 1, len(depth) - 1), height[q] - 1))\n elif hi == len(depth) - 1:\n res.append(max(seg.query(0, lo - 1), height[q] - 1))\n else:\n res.append(max(seg.query(0, lo - 1), seg.query(hi + 1, len(depth) - 1), height[q] - 1))\n \n return res \n \n \n \n \n \n \n \n \n \n\n``` | 5 | You are given the `root` of a **binary tree** with `n` nodes. Each node is assigned a unique value from `1` to `n`. You are also given an array `queries` of size `m`.
You have to perform `m` **independent** queries on the tree where in the `ith` query you do the following:
* **Remove** the subtree rooted at the node with the value `queries[i]` from the tree. It is **guaranteed** that `queries[i]` will **not** be equal to the value of the root.
Return _an array_ `answer` _of size_ `m` _where_ `answer[i]` _is the height of the tree after performing the_ `ith` _query_.
**Note**:
* The queries are independent, so the tree returns to its **initial** state after each query.
* The height of a tree is the **number of edges in the longest simple path** from the root to some node in the tree.
**Example 1:**
**Input:** root = \[1,3,4,2,null,6,5,null,null,null,null,null,7\], queries = \[4\]
**Output:** \[2\]
**Explanation:** The diagram above shows the tree after removing the subtree rooted at node with value 4.
The height of the tree is 2 (The path 1 -> 3 -> 2).
**Example 2:**
**Input:** root = \[5,8,9,2,1,3,7,4,6\], queries = \[3,2,4,8\]
**Output:** \[3,2,3,2\]
**Explanation:** We have the following queries:
- Removing the subtree rooted at node with value 3. The height of the tree becomes 3 (The path 5 -> 8 -> 2 -> 4).
- Removing the subtree rooted at node with value 2. The height of the tree becomes 2 (The path 5 -> 8 -> 1).
- Removing the subtree rooted at node with value 4. The height of the tree becomes 3 (The path 5 -> 8 -> 2 -> 6).
- Removing the subtree rooted at node with value 8. The height of the tree becomes 2 (The path 5 -> 9 -> 3).
**Constraints:**
* The number of nodes in the tree is `n`.
* `2 <= n <= 105`
* `1 <= Node.val <= n`
* All the values in the tree are **unique**.
* `m == queries.length`
* `1 <= m <= min(n, 104)`
* `1 <= queries[i] <= n`
* `queries[i] != root.val` | null |
simple and fast - DFS + heap | height-of-binary-tree-after-subtree-removal-queries | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nEach node has a height and a depth associated with it. Height is the number of levels above the node and depth is the number of levels below the node. So the nodes potential contribution to the maximum height of the binary tree is its height + depth (+/- 1 depending on how you track these variables). We will have a max heap for each level of the tree. We will calculate the potential contribution of each nodes height+depth to the max height of the tree and put this value into its levels heap. We put this into the max_heap (depth + height, height, node.val). Finally we have three situations to consider:\n\n1, q is the only node on this level. We return the HEIGHT of any of the nodes one level above our level.\n\n2, we have more than one node in our lvl, but q is on the top of the heap. We simply lookup the next biggest HEIGHT + DEPTH value in the heap.\n\n3, we have more than one node in our lvl and q is not on the top of the heap. We simply add the HEIGHT + DEPTH value from the top of the heap.\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\nO(N) + q * O(logN)\nO(N) - to precompute the answers\nO(logN) - per query to look up the answer\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\nO(N) - the combined heaps hold N nodes\n\n\n# Code\n```\n# Definition for a binary tree node.\n# class TreeNode:\n# def __init__(self, val=0, left=None, right=None):\n# self.val = val\n# self.left = left\n# self.right = right\nclass Solution:\n def treeQueries(self, root: Optional[TreeNode], queries: List[int]) -> List[int]:\n node_to_lvl = {}\n lvls = defaultdict(list)\n\n def dfs(node, height):\n if not node:\n return 0\n height += 1\n left = dfs(node.left, height) + 1\n right = dfs(node.right, height) + 1\n node_to_lvl[node.val] = height\n heapq.heappush(lvls[height], (-max(left, right) - height + 1, height, node.val))\n return max(left, right)\n \n dfs(root, -1)\n res = []\n\n for q in queries:\n lvl = node_to_lvl[q]\n if q == root.val:\n res.append(0)\n elif len(lvls[lvl]) == 1:\n res.append(lvls[lvl - 1][0][1])\n elif lvls[lvl][0][2] == q:\n temp = heapq.heappop(lvls[lvl])\n res.append(-lvls[lvl][0][0])\n heapq.heappush(lvls[lvl], temp)\n elif lvls[lvl][0][2] != q:\n res.append(-lvls[lvl][0][0])\n \n return res\n \n\n``` | 3 | You are given the `root` of a **binary tree** with `n` nodes. Each node is assigned a unique value from `1` to `n`. You are also given an array `queries` of size `m`.
You have to perform `m` **independent** queries on the tree where in the `ith` query you do the following:
* **Remove** the subtree rooted at the node with the value `queries[i]` from the tree. It is **guaranteed** that `queries[i]` will **not** be equal to the value of the root.
Return _an array_ `answer` _of size_ `m` _where_ `answer[i]` _is the height of the tree after performing the_ `ith` _query_.
**Note**:
* The queries are independent, so the tree returns to its **initial** state after each query.
* The height of a tree is the **number of edges in the longest simple path** from the root to some node in the tree.
**Example 1:**
**Input:** root = \[1,3,4,2,null,6,5,null,null,null,null,null,7\], queries = \[4\]
**Output:** \[2\]
**Explanation:** The diagram above shows the tree after removing the subtree rooted at node with value 4.
The height of the tree is 2 (The path 1 -> 3 -> 2).
**Example 2:**
**Input:** root = \[5,8,9,2,1,3,7,4,6\], queries = \[3,2,4,8\]
**Output:** \[3,2,3,2\]
**Explanation:** We have the following queries:
- Removing the subtree rooted at node with value 3. The height of the tree becomes 3 (The path 5 -> 8 -> 2 -> 4).
- Removing the subtree rooted at node with value 2. The height of the tree becomes 2 (The path 5 -> 8 -> 1).
- Removing the subtree rooted at node with value 4. The height of the tree becomes 3 (The path 5 -> 8 -> 2 -> 6).
- Removing the subtree rooted at node with value 8. The height of the tree becomes 2 (The path 5 -> 9 -> 3).
**Constraints:**
* The number of nodes in the tree is `n`.
* `2 <= n <= 105`
* `1 <= Node.val <= n`
* All the values in the tree are **unique**.
* `m == queries.length`
* `1 <= m <= min(n, 104)`
* `1 <= queries[i] <= n`
* `queries[i] != root.val` | null |
Python3 | 100% T | 85% S | O(N) Time and Space | Explained for Beginner Friendly | height-of-binary-tree-after-subtree-removal-queries | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nWe can think of solving offline query. \nNot all nodes will affect the height of tree, only those node who are in path of maximum height will affect the height of tree\n# Approach\n<!-- Describe your approach to solving the problem. -->\nLet\'s say leaf node <code> X</code> is giving the longest height. Now let\'s say path from root to <code> X</code> is like n1 -> n2 -> n3 -> n4 -> <code> X</code>.\nSo removing only these node will alter your height. \nSo we start from root, check the level of left and right, and whichever is greater, removing that will affect the answer. Whichever is lower, removing that won\'t change our height. \nWe do recursively to all nodes. \n<code> curmx </code> is the current maximum height if we remove the curent node.\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\nO(N)\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\nO(N)\n# Code\n```\n# Definition for a binary tree node.\n# class TreeNode:\n# def __init__(self, val=0, left=None, right=None):\n# self.val = val\n# self.left = left\n# self.right = right\nclass Solution:\n def treeQueries(self, root: Optional[TreeNode], q : List[int]) -> List[int]:\n lvl = defaultdict(int)\n mx = 0\n def level(node):\n nonlocal mx\n if not node:\n return 0\n l = level(node.left)\n r = level(node.right)\n lvl[node.val] = max(l,r) +1\n mx = max(mx, lvl[node.val])\n return lvl[node.val]\n level(root)\n n = len(lvl) + 3\n ans = [mx-1 for i in range(n+1)]\n curmx = 0\n def helper(node, cur):\n if not node:\n return \n nonlocal curmx\n # print(node.val)\n l,r = 0,0\n if node.left:\n l = lvl[node.left.val] \n if node.right:\n r = lvl[node.right.val] \n if r > l:\n right = node.right.val\n curmx = max(curmx, cur + l)\n ans[right] = curmx\n helper(node.right, cur + 1)\n elif l > r:\n left = node.left.val\n curmx = max(curmx, cur + r)\n ans[left] = curmx\n helper(node.left, cur + 1)\n else:\n return\n helper(root, 0)\n return [ans[i] for i in q]\n\n \n``` | 1 | You are given the `root` of a **binary tree** with `n` nodes. Each node is assigned a unique value from `1` to `n`. You are also given an array `queries` of size `m`.
You have to perform `m` **independent** queries on the tree where in the `ith` query you do the following:
* **Remove** the subtree rooted at the node with the value `queries[i]` from the tree. It is **guaranteed** that `queries[i]` will **not** be equal to the value of the root.
Return _an array_ `answer` _of size_ `m` _where_ `answer[i]` _is the height of the tree after performing the_ `ith` _query_.
**Note**:
* The queries are independent, so the tree returns to its **initial** state after each query.
* The height of a tree is the **number of edges in the longest simple path** from the root to some node in the tree.
**Example 1:**
**Input:** root = \[1,3,4,2,null,6,5,null,null,null,null,null,7\], queries = \[4\]
**Output:** \[2\]
**Explanation:** The diagram above shows the tree after removing the subtree rooted at node with value 4.
The height of the tree is 2 (The path 1 -> 3 -> 2).
**Example 2:**
**Input:** root = \[5,8,9,2,1,3,7,4,6\], queries = \[3,2,4,8\]
**Output:** \[3,2,3,2\]
**Explanation:** We have the following queries:
- Removing the subtree rooted at node with value 3. The height of the tree becomes 3 (The path 5 -> 8 -> 2 -> 4).
- Removing the subtree rooted at node with value 2. The height of the tree becomes 2 (The path 5 -> 8 -> 1).
- Removing the subtree rooted at node with value 4. The height of the tree becomes 3 (The path 5 -> 8 -> 2 -> 6).
- Removing the subtree rooted at node with value 8. The height of the tree becomes 2 (The path 5 -> 9 -> 3).
**Constraints:**
* The number of nodes in the tree is `n`.
* `2 <= n <= 105`
* `1 <= Node.val <= n`
* All the values in the tree are **unique**.
* `m == queries.length`
* `1 <= m <= min(n, 104)`
* `1 <= queries[i] <= n`
* `queries[i] != root.val` | null |
Python (Simple DFS) | height-of-binary-tree-after-subtree-removal-queries | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def treeQueries(self, root, queries):\n @lru_cache(None)\n def height(node):\n if not node:\n return -1\n\n return 1 + max(height(node.left),height(node.right))\n\n dict1 = collections.defaultdict(int)\n\n def dfs(node,depth,max_val):\n if not node: return \n\n dict1[node.val] = max_val\n\n dfs(node.left,depth+1,max(max_val,depth+1+height(node.right)))\n dfs(node.right,depth+1,max(max_val,depth+1+height(node.left)))\n\n dfs(root,0,0)\n\n return [dict1[i] for i in queries]\n\n\n\n \n\n\n \n\n \n\n\n``` | 6 | You are given the `root` of a **binary tree** with `n` nodes. Each node is assigned a unique value from `1` to `n`. You are also given an array `queries` of size `m`.
You have to perform `m` **independent** queries on the tree where in the `ith` query you do the following:
* **Remove** the subtree rooted at the node with the value `queries[i]` from the tree. It is **guaranteed** that `queries[i]` will **not** be equal to the value of the root.
Return _an array_ `answer` _of size_ `m` _where_ `answer[i]` _is the height of the tree after performing the_ `ith` _query_.
**Note**:
* The queries are independent, so the tree returns to its **initial** state after each query.
* The height of a tree is the **number of edges in the longest simple path** from the root to some node in the tree.
**Example 1:**
**Input:** root = \[1,3,4,2,null,6,5,null,null,null,null,null,7\], queries = \[4\]
**Output:** \[2\]
**Explanation:** The diagram above shows the tree after removing the subtree rooted at node with value 4.
The height of the tree is 2 (The path 1 -> 3 -> 2).
**Example 2:**
**Input:** root = \[5,8,9,2,1,3,7,4,6\], queries = \[3,2,4,8\]
**Output:** \[3,2,3,2\]
**Explanation:** We have the following queries:
- Removing the subtree rooted at node with value 3. The height of the tree becomes 3 (The path 5 -> 8 -> 2 -> 4).
- Removing the subtree rooted at node with value 2. The height of the tree becomes 2 (The path 5 -> 8 -> 1).
- Removing the subtree rooted at node with value 4. The height of the tree becomes 3 (The path 5 -> 8 -> 2 -> 6).
- Removing the subtree rooted at node with value 8. The height of the tree becomes 2 (The path 5 -> 9 -> 3).
**Constraints:**
* The number of nodes in the tree is `n`.
* `2 <= n <= 105`
* `1 <= Node.val <= n`
* All the values in the tree are **unique**.
* `m == queries.length`
* `1 <= m <= min(n, 104)`
* `1 <= queries[i] <= n`
* `queries[i] != root.val` | null |
[Python 3]Topological sort + Hashmap | height-of-binary-tree-after-subtree-removal-queries | 0 | 1 | ```\n# Definition for a binary tree node.\n# class TreeNode:\n# def __init__(self, val=0, left=None, right=None):\n# self.val = val\n# self.left = left\n# self.right = right\nclass Solution:\n def treeQueries(self, root: Optional[TreeNode], queries: List[int]) -> List[int]:\n par = {} # keep track of parent nodes\n height = {} # height for each node\n \n def dfs(node, level):\n if not node:\n return\n height[node.val] = level\n if node.left:\n par[node.left.val] = node.val\n dfs(node.left, level+1)\n if node.right:\n par[node.right.val] = node.val\n dfs(node.right, level+1)\n \n dfs(root, 0)\n \n # Topological sort\n deg = defaultdict(int)\n for k in par:\n deg[par[k]] += 1\n # starts from leaf nodes \n q = [k for k in height if not deg[k]]\n h = height.copy() # propagate depth to parent nodes\n \n while q:\n cur = q.pop()\n if cur not in par: continue\n p = par[cur]\n deg[p] -= 1\n h[p] = max(h[p], h[cur])\n if not deg[p]:\n q.append(p)\n \n # for each level, only store top 2 nodes with deepest path\n h_node = defaultdict(list)\n for k in height:\n heappush(h_node[height[k]], (h[k], k))\n if len(h_node[height[k]]) > 2:\n heappop(h_node[height[k]])\n \n \n ans = []\n for q in queries:\n # if current level only one node then whole level gone, the height will minus 1\n if len(h_node[height[q]]) == 1:\n ans.append(height[q] - 1)\n # if deleted node equals the node with deepest path at current level, then chose the second deepest\n elif q == h_node[height[q]][1][1]:\n ans.append(h_node[height[q]][0][0])\n # otherwise select the top one\n else:\n ans.append(h_node[height[q]][1][0])\n\n \n return ans | 1 | You are given the `root` of a **binary tree** with `n` nodes. Each node is assigned a unique value from `1` to `n`. You are also given an array `queries` of size `m`.
You have to perform `m` **independent** queries on the tree where in the `ith` query you do the following:
* **Remove** the subtree rooted at the node with the value `queries[i]` from the tree. It is **guaranteed** that `queries[i]` will **not** be equal to the value of the root.
Return _an array_ `answer` _of size_ `m` _where_ `answer[i]` _is the height of the tree after performing the_ `ith` _query_.
**Note**:
* The queries are independent, so the tree returns to its **initial** state after each query.
* The height of a tree is the **number of edges in the longest simple path** from the root to some node in the tree.
**Example 1:**
**Input:** root = \[1,3,4,2,null,6,5,null,null,null,null,null,7\], queries = \[4\]
**Output:** \[2\]
**Explanation:** The diagram above shows the tree after removing the subtree rooted at node with value 4.
The height of the tree is 2 (The path 1 -> 3 -> 2).
**Example 2:**
**Input:** root = \[5,8,9,2,1,3,7,4,6\], queries = \[3,2,4,8\]
**Output:** \[3,2,3,2\]
**Explanation:** We have the following queries:
- Removing the subtree rooted at node with value 3. The height of the tree becomes 3 (The path 5 -> 8 -> 2 -> 4).
- Removing the subtree rooted at node with value 2. The height of the tree becomes 2 (The path 5 -> 8 -> 1).
- Removing the subtree rooted at node with value 4. The height of the tree becomes 3 (The path 5 -> 8 -> 2 -> 6).
- Removing the subtree rooted at node with value 8. The height of the tree becomes 2 (The path 5 -> 9 -> 3).
**Constraints:**
* The number of nodes in the tree is `n`.
* `2 <= n <= 105`
* `1 <= Node.val <= n`
* All the values in the tree are **unique**.
* `m == queries.length`
* `1 <= m <= min(n, 104)`
* `1 <= queries[i] <= n`
* `queries[i] != root.val` | null |
Python Solution (Beats 99.50%) || 41ms|| O(N) || Easy Solution | apply-operations-to-an-array | 0 | 1 | # Complexity\n- Time complexity:\n O(N)\n# Code\n```\nclass Solution:\n def applyOperations(self, nums: List[int]) -> List[int]:\n zeros=0\n i=0\n while(i<(len(nums)-1)):\n if(nums[i]==nums[i+1]):\n nums[i]*=2\n nums[i+1]=0\n i+=1\n i+=1\n # print(nums)\n zeros=nums.count(0)\n nums = [i for i in nums if i != 0]\n return nums+([0]*zeros)\n``` | 3 | You are given a **0-indexed** array `nums` of size `n` consisting of **non-negative** integers.
You need to apply `n - 1` operations to this array where, in the `ith` operation (**0-indexed**), you will apply the following on the `ith` element of `nums`:
* If `nums[i] == nums[i + 1]`, then multiply `nums[i]` by `2` and set `nums[i + 1]` to `0`. Otherwise, you skip this operation.
After performing **all** the operations, **shift** all the `0`'s to the **end** of the array.
* For example, the array `[1,0,2,0,0,1]` after shifting all its `0`'s to the end, is `[1,2,1,0,0,0]`.
Return _the resulting array_.
**Note** that the operations are applied **sequentially**, not all at once.
**Example 1:**
**Input:** nums = \[1,2,2,1,1,0\]
**Output:** \[1,4,2,0,0,0\]
**Explanation:** We do the following operations:
- i = 0: nums\[0\] and nums\[1\] are not equal, so we skip this operation.
- i = 1: nums\[1\] and nums\[2\] are equal, we multiply nums\[1\] by 2 and change nums\[2\] to 0. The array becomes \[1,**4**,**0**,1,1,0\].
- i = 2: nums\[2\] and nums\[3\] are not equal, so we skip this operation.
- i = 3: nums\[3\] and nums\[4\] are equal, we multiply nums\[3\] by 2 and change nums\[4\] to 0. The array becomes \[1,4,0,**2**,**0**,0\].
- i = 4: nums\[4\] and nums\[5\] are equal, we multiply nums\[4\] by 2 and change nums\[5\] to 0. The array becomes \[1,4,0,2,**0**,**0**\].
After that, we shift the 0's to the end, which gives the array \[1,4,2,0,0,0\].
**Example 2:**
**Input:** nums = \[0,1\]
**Output:** \[1,0\]
**Explanation:** No operation can be applied, we just shift the 0 to the end.
**Constraints:**
* `2 <= nums.length <= 2000`
* `0 <= nums[i] <= 1000` | null |
Sliding window with hashmap to count distinct | maximum-sum-of-distinct-subarrays-with-length-k | 0 | 1 | ```\nfrom collections import Counter\n\nclass Solution:\n def maximumSubarraySum(self, nums: List[int], k: int) -> int:\n n = len(nums)\n s = 0\n res = 0\n ctr = Counter()\n l = 0\n for i in range(n):\n s += nums[i]\n if ctr[nums[i]] == 0:\n l += 1\n ctr[nums[i]] += 1\n if i >= k:\n s -= nums[i - k]\n ctr[nums[i - k]] -= 1\n if ctr[nums[i - k]] == 0:\n l -= 1\n if i >= k - 1:\n if l == k:\n res = max(res, s)\n return res\n``` | 3 | You are given an integer array `nums` and an integer `k`. Find the maximum subarray sum of all the subarrays of `nums` that meet the following conditions:
* The length of the subarray is `k`, and
* All the elements of the subarray are **distinct**.
Return _the maximum subarray sum of all the subarrays that meet the conditions__._ If no subarray meets the conditions, return `0`.
_A **subarray** is a contiguous non-empty sequence of elements within an array._
**Example 1:**
**Input:** nums = \[1,5,4,2,9,9,9\], k = 3
**Output:** 15
**Explanation:** The subarrays of nums with length 3 are:
- \[1,5,4\] which meets the requirements and has a sum of 10.
- \[5,4,2\] which meets the requirements and has a sum of 11.
- \[4,2,9\] which meets the requirements and has a sum of 15.
- \[2,9,9\] which does not meet the requirements because the element 9 is repeated.
- \[9,9,9\] which does not meet the requirements because the element 9 is repeated.
We return 15 because it is the maximum subarray sum of all the subarrays that meet the conditions
**Example 2:**
**Input:** nums = \[4,4,4\], k = 3
**Output:** 0
**Explanation:** The subarrays of nums with length 3 are:
- \[4,4,4\] which does not meet the requirements because the element 4 is repeated.
We return 0 because no subarrays meet the conditions.
**Constraints:**
* `1 <= k <= nums.length <= 105`
* `1 <= nums[i] <= 105` | null |
Python O(n) by prefix sum + sliding window [w/ Hint] | maximum-sum-of-distinct-subarrays-with-length-k | 0 | 1 | **Hint**\n\nThis is a combination of [**Leetcode #303 Range Sum Query**](https://leetcode.com/problems/range-sum-query-immutable/) and [**Leetcode #3 Longest substring without repetition**](https://leetcode.com/problems/longest-substring-without-repeating-characters/) in numerical version.\n\nWhen it comes to **range sum operation in array**, think of **prefix sum**.\n\nWhen it comes to **specific goal function optimization of substring / subarray**, think of **sliding window**.\n\n---\n\n**Python**:\n\n```\nclass Solution:\n def maximumSubarraySum(self, nums: List[int], k: int) -> int:\n \n # Build prefix sum table to help us calculate range sum in O(1)\n prefixS = [ nums[0] ]\n for i in range( 1, len(nums) ):\n prefixS.append( prefixS[-1] + nums[i] )\n \n \n \n best = 0 # maximum subarray sum\n start = 0 # left index of sliding window\n summation = 0 # current subarray sum\n \n \n ## dictionary\n # key: number\n # value: latest index of number on the left hand side\n lastIndexOf = defaultdict( lambda : -1)\n \n \n # Sliding windows bounded in [start, end] inclusively\n for end, number in enumerate(nums):\n \n if lastIndexOf[number] >= start:\n # current number has shown up before\n summation = prefixS[end] - prefixS[ lastIndexOf[number] ]\n start = lastIndexOf[number] + 1\n \n elif start + k == end:\n # current number is unique, but we are forced to discard the leftmost element to keep window size k\n summation = prefixS[end] - prefixS[start]\n start += 1\n \n else:\n # current number is unique, just adding it to summation\n summation += nums[end]\n \n \n if (end - start + 1) == k:\n # Update subarray sum only when window size is k\n best = max(best, summation)\n \n # update lasest index of number\n lastIndexOf[ number ] = end\n \n return best\n \n``` | 1 | You are given an integer array `nums` and an integer `k`. Find the maximum subarray sum of all the subarrays of `nums` that meet the following conditions:
* The length of the subarray is `k`, and
* All the elements of the subarray are **distinct**.
Return _the maximum subarray sum of all the subarrays that meet the conditions__._ If no subarray meets the conditions, return `0`.
_A **subarray** is a contiguous non-empty sequence of elements within an array._
**Example 1:**
**Input:** nums = \[1,5,4,2,9,9,9\], k = 3
**Output:** 15
**Explanation:** The subarrays of nums with length 3 are:
- \[1,5,4\] which meets the requirements and has a sum of 10.
- \[5,4,2\] which meets the requirements and has a sum of 11.
- \[4,2,9\] which meets the requirements and has a sum of 15.
- \[2,9,9\] which does not meet the requirements because the element 9 is repeated.
- \[9,9,9\] which does not meet the requirements because the element 9 is repeated.
We return 15 because it is the maximum subarray sum of all the subarrays that meet the conditions
**Example 2:**
**Input:** nums = \[4,4,4\], k = 3
**Output:** 0
**Explanation:** The subarrays of nums with length 3 are:
- \[4,4,4\] which does not meet the requirements because the element 4 is repeated.
We return 0 because no subarrays meet the conditions.
**Constraints:**
* `1 <= k <= nums.length <= 105`
* `1 <= nums[i] <= 105` | null |
python/java solution | maximum-sum-of-distinct-subarrays-with-length-k | 1 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def maximumSubarraySum(self, nums: List[int], k: int) -> int:\n seen = collections.defaultdict(int)\n s = 0\n res = 0\n for i in range(k):\n s += nums[i]\n seen[nums[i]] += 1\n if len(seen) == k:\n res = s\n for i in range(k, len(nums)):\n s -= nums[i - k]\n s += nums[i]\n seen[nums[i - k]] -= 1\n if seen[nums[i - k]] == 0:\n del seen[nums[i - k]]\n seen[nums[i]] += 1\n if len(seen) == k:\n res = max(res, s)\n return res\n\nclass Solution {\n public long maximumSubarraySum(int[] nums, int k) {\n Map<Integer, Integer> seen = new HashMap<>();\n long s = 0;\n long res = 0;\n for (int i = 0; i < k; i ++) {\n s += (long)nums[i];\n seen.put(nums[i], seen.getOrDefault(nums[i], 0) + 1);\n }\n if (seen.size() == k) {\n res = s;\n }\n for (int i = k; i < nums.length; i ++) {\n s -= nums[i - k];\n s += nums[i];\n seen.put(nums[i - k], seen.get(nums[i - k]) - 1);\n if (seen.get(nums[i - k]) == 0) {\n seen.remove(nums[i - k]);\n }\n seen.put(nums[i], seen.getOrDefault(nums[i], 0) + 1);\n if (seen.size() == k) {\n res = Math.max(res, s);\n }\n }\n return res;\n }\n}\n``` | 1 | You are given an integer array `nums` and an integer `k`. Find the maximum subarray sum of all the subarrays of `nums` that meet the following conditions:
* The length of the subarray is `k`, and
* All the elements of the subarray are **distinct**.
Return _the maximum subarray sum of all the subarrays that meet the conditions__._ If no subarray meets the conditions, return `0`.
_A **subarray** is a contiguous non-empty sequence of elements within an array._
**Example 1:**
**Input:** nums = \[1,5,4,2,9,9,9\], k = 3
**Output:** 15
**Explanation:** The subarrays of nums with length 3 are:
- \[1,5,4\] which meets the requirements and has a sum of 10.
- \[5,4,2\] which meets the requirements and has a sum of 11.
- \[4,2,9\] which meets the requirements and has a sum of 15.
- \[2,9,9\] which does not meet the requirements because the element 9 is repeated.
- \[9,9,9\] which does not meet the requirements because the element 9 is repeated.
We return 15 because it is the maximum subarray sum of all the subarrays that meet the conditions
**Example 2:**
**Input:** nums = \[4,4,4\], k = 3
**Output:** 0
**Explanation:** The subarrays of nums with length 3 are:
- \[4,4,4\] which does not meet the requirements because the element 4 is repeated.
We return 0 because no subarrays meet the conditions.
**Constraints:**
* `1 <= k <= nums.length <= 105`
* `1 <= nums[i] <= 105` | null |
Clear Explanation | 1 Queue and 2 Priority-Queues | total-cost-to-hire-k-workers | 0 | 1 | # Intuition\nThe conditions are clearly stated.\nImplement the conditions stated.\n\n\n# Approach\n- Use 2 priority-queues to store and obtain the smallest costs for the first and last candidates respectively.\n\n- Store the middle elements(elements that are not currently in any of the priority-queues) in a Queue.\n- Compare the smallest values of the two priorty queues.\n- Pop the smallest and add the cost to the total cost to hire workers.\n- If there is any element in the middle Queue add the first or the last element(first element if the smallest was in the first priority queue) to the priority-queue that contained the smallest cost.\n- Repeat till k workers are selected.\n\n# Complexity\n- Time complexity:\nO(NLog(N))\n\n- Space complexity:\nO(N)\n\n# Code\n```\nfrom collections import deque\nfrom heapq import *\nclass Solution:\n def totalCost(self, costs: List[int], k: int, candidates: int) -> int:\n\n middle = deque()\n left = [float("inf")]\n right = [float("inf")]\n \n for i, cost in enumerate(costs):\n if i < candidates: \n heappush(left, cost)\n elif i >= len(costs) - candidates:\n heappush(right, cost)\n else:\n middle.append(cost)\n\n\n total_cost = 0\n for _ in range(k):\n if left[0] <= right[0]:\n total_cost += heappop(left)\n middle and heappush(left, middle.popleft())\n else:\n total_cost += heappop(right)\n middle and heappush(right, middle.pop())\n\n return total_cost\n\n \n\n \n\n\n\n``` | 1 | You are given a **0-indexed** integer array `costs` where `costs[i]` is the cost of hiring the `ith` worker.
You are also given two integers `k` and `candidates`. We want to hire exactly `k` workers according to the following rules:
* You will run `k` sessions and hire exactly one worker in each session.
* In each hiring session, choose the worker with the lowest cost from either the first `candidates` workers or the last `candidates` workers. Break the tie by the smallest index.
* For example, if `costs = [3,2,7,7,1,2]` and `candidates = 2`, then in the first hiring session, we will choose the `4th` worker because they have the lowest cost `[3,2,7,7,**1**,2]`.
* In the second hiring session, we will choose `1st` worker because they have the same lowest cost as `4th` worker but they have the smallest index `[3,**2**,7,7,2]`. Please note that the indexing may be changed in the process.
* If there are fewer than candidates workers remaining, choose the worker with the lowest cost among them. Break the tie by the smallest index.
* A worker can only be chosen once.
Return _the total cost to hire exactly_ `k` _workers._
**Example 1:**
**Input:** costs = \[17,12,10,2,7,2,11,20,8\], k = 3, candidates = 4
**Output:** 11
**Explanation:** We hire 3 workers in total. The total cost is initially 0.
- In the first hiring round we choose the worker from \[17,12,10,2,7,2,11,20,8\]. The lowest cost is 2, and we break the tie by the smallest index, which is 3. The total cost = 0 + 2 = 2.
- In the second hiring round we choose the worker from \[17,12,10,7,2,11,20,8\]. The lowest cost is 2 (index 4). The total cost = 2 + 2 = 4.
- In the third hiring round we choose the worker from \[17,12,10,7,11,20,8\]. The lowest cost is 7 (index 3). The total cost = 4 + 7 = 11. Notice that the worker with index 3 was common in the first and last four workers.
The total hiring cost is 11.
**Example 2:**
**Input:** costs = \[1,2,4,1\], k = 3, candidates = 3
**Output:** 4
**Explanation:** We hire 3 workers in total. The total cost is initially 0.
- In the first hiring round we choose the worker from \[1,2,4,1\]. The lowest cost is 1, and we break the tie by the smallest index, which is 0. The total cost = 0 + 1 = 1. Notice that workers with index 1 and 2 are common in the first and last 3 workers.
- In the second hiring round we choose the worker from \[2,4,1\]. The lowest cost is 1 (index 2). The total cost = 1 + 1 = 2.
- In the third hiring round there are less than three candidates. We choose the worker from the remaining workers \[2,4\]. The lowest cost is 2 (index 0). The total cost = 2 + 2 = 4.
The total hiring cost is 4.
**Constraints:**
* `1 <= costs.length <= 105`
* `1 <= costs[i] <= 105`
* `1 <= k, candidates <= costs.length` | null |
EASY PYTHON SOLUTION || HEAPSORT | total-cost-to-hire-k-workers | 0 | 1 | \n# Code\n```\nimport heapq\nclass Solution:\n def totalCost(self, costs: List[int], k: int, candidates: int) -> int:\n n=len(costs)\n if 2*candidates>=n:\n costs.sort()\n return sum(costs[:k])\n df=n-(2*candidates)\n df2=df\n sm=0\n s1=costs[:candidates]\n s2=costs[n-candidates:]\n heapq.heapify(s1)\n heapq.heapify(s2)\n i=candidates\n j=n-candidates-1\n # print(costs[i],costs[j])\n ct=k\n while df>0 and k>0:\n x=heapq.heappop(s1)\n y=heapq.heappop(s2)\n if x<=y:\n sm+=x\n heapq.heappush(s1,costs[i])\n heapq.heappush(s2,y)\n i+=1\n else:\n sm+=y\n heapq.heappush(s1,x)\n heapq.heappush(s2,costs[j])\n j-=1\n df-=1\n k-=1\n lst1=heapq.nsmallest(min(k,len(s1)),s1)\n lst2=heapq.nsmallest(min(k,len(s2)),s2)\n lst=[]\n lst+=lst1[:]\n lst+=lst2[:]\n lst.sort()\n return sm+sum(lst[:k])\n\n \n``` | 1 | You are given a **0-indexed** integer array `costs` where `costs[i]` is the cost of hiring the `ith` worker.
You are also given two integers `k` and `candidates`. We want to hire exactly `k` workers according to the following rules:
* You will run `k` sessions and hire exactly one worker in each session.
* In each hiring session, choose the worker with the lowest cost from either the first `candidates` workers or the last `candidates` workers. Break the tie by the smallest index.
* For example, if `costs = [3,2,7,7,1,2]` and `candidates = 2`, then in the first hiring session, we will choose the `4th` worker because they have the lowest cost `[3,2,7,7,**1**,2]`.
* In the second hiring session, we will choose `1st` worker because they have the same lowest cost as `4th` worker but they have the smallest index `[3,**2**,7,7,2]`. Please note that the indexing may be changed in the process.
* If there are fewer than candidates workers remaining, choose the worker with the lowest cost among them. Break the tie by the smallest index.
* A worker can only be chosen once.
Return _the total cost to hire exactly_ `k` _workers._
**Example 1:**
**Input:** costs = \[17,12,10,2,7,2,11,20,8\], k = 3, candidates = 4
**Output:** 11
**Explanation:** We hire 3 workers in total. The total cost is initially 0.
- In the first hiring round we choose the worker from \[17,12,10,2,7,2,11,20,8\]. The lowest cost is 2, and we break the tie by the smallest index, which is 3. The total cost = 0 + 2 = 2.
- In the second hiring round we choose the worker from \[17,12,10,7,2,11,20,8\]. The lowest cost is 2 (index 4). The total cost = 2 + 2 = 4.
- In the third hiring round we choose the worker from \[17,12,10,7,11,20,8\]. The lowest cost is 7 (index 3). The total cost = 4 + 7 = 11. Notice that the worker with index 3 was common in the first and last four workers.
The total hiring cost is 11.
**Example 2:**
**Input:** costs = \[1,2,4,1\], k = 3, candidates = 3
**Output:** 4
**Explanation:** We hire 3 workers in total. The total cost is initially 0.
- In the first hiring round we choose the worker from \[1,2,4,1\]. The lowest cost is 1, and we break the tie by the smallest index, which is 0. The total cost = 0 + 1 = 1. Notice that workers with index 1 and 2 are common in the first and last 3 workers.
- In the second hiring round we choose the worker from \[2,4,1\]. The lowest cost is 1 (index 2). The total cost = 1 + 1 = 2.
- In the third hiring round there are less than three candidates. We choose the worker from the remaining workers \[2,4\]. The lowest cost is 2 (index 0). The total cost = 2 + 2 = 4.
The total hiring cost is 4.
**Constraints:**
* `1 <= costs.length <= 105`
* `1 <= costs[i] <= 105`
* `1 <= k, candidates <= costs.length` | null |
O(n^3) solution, sort + shift the minimum subarray of assignments | minimum-total-distance-traveled | 0 | 1 | # Intuition\nAs you shift the factory assignment of any given robot from left to right, the distance traveled first decreases to a minimum (or starts from the minimum), and then starts to increase again. As we go through the robots\' initial positions one by one from left the right, the closest factory either stays the same or shifts to one of the factories to the right. So, it never makes sense for the robots to cross path. The only complication is that factories may run out of capacity: In that case, a subarray of the sorted `robot` have to shift assignments at the same time.\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\nExpand the full list of capacity of sorted `factory` as `cap` list. Initially assign the sorted `robot` to the leftmost subarray. Consider the robots in the reverse order. Shift the assignments of the smallest subarray starting from the `i`th robot of the sorted `robot` from left to right until the distance travled starts to increase.\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\nO(n^3), `n = max(len(robot), len(factory))`\n\n- Space complexity:\nO(n^2) due to the `cap` list\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def minimumTotalDistance(self, robot: List[int], factory: List[List[int]]) -> int: \n robot.sort()\n factory.sort()\n cap = []\n for x, limit in factory:\n cap.extend([x] * limit)\n m = len(robot)\n n = len(cap)\n indices = list(range(m))\n ans = sum(abs(x - y) for x, y in zip(robot, cap))\n \n def increment(i):\n diff = 0\n while i < m:\n if indices[i] + 1 < n:\n diff -= abs(robot[i] - cap[indices[i]])\n diff += abs(robot[i] - cap[indices[i] + 1])\n else:\n return math.inf, i + 1\n if i + 1 < m and indices[i] + 1 == indices[i + 1]:\n i += 1\n else:\n return diff, i + 1\n for i in reversed(range(m)):\n while True:\n diff, j = increment(i)\n if diff <= 0:\n ans += diff\n for x in range(i, j):\n indices[x] += 1\n else:\n break\n return ans\n``` | 1 | There are some robots and factories on the X-axis. You are given an integer array `robot` where `robot[i]` is the position of the `ith` robot. You are also given a 2D integer array `factory` where `factory[j] = [positionj, limitj]` indicates that `positionj` is the position of the `jth` factory and that the `jth` factory can repair at most `limitj` robots.
The positions of each robot are **unique**. The positions of each factory are also **unique**. Note that a robot can be **in the same position** as a factory initially.
All the robots are initially broken; they keep moving in one direction. The direction could be the negative or the positive direction of the X-axis. When a robot reaches a factory that did not reach its limit, the factory repairs the robot, and it stops moving.
**At any moment**, you can set the initial direction of moving for **some** robot. Your target is to minimize the total distance traveled by all the robots.
Return _the minimum total distance traveled by all the robots_. The test cases are generated such that all the robots can be repaired.
**Note that**
* All robots move at the same speed.
* If two robots move in the same direction, they will never collide.
* If two robots move in opposite directions and they meet at some point, they do not collide. They cross each other.
* If a robot passes by a factory that reached its limits, it crosses it as if it does not exist.
* If the robot moved from a position `x` to a position `y`, the distance it moved is `|y - x|`.
**Example 1:**
**Input:** robot = \[0,4,6\], factory = \[\[2,2\],\[6,2\]\]
**Output:** 4
**Explanation:** As shown in the figure:
- The first robot at position 0 moves in the positive direction. It will be repaired at the first factory.
- The second robot at position 4 moves in the negative direction. It will be repaired at the first factory.
- The third robot at position 6 will be repaired at the second factory. It does not need to move.
The limit of the first factory is 2, and it fixed 2 robots.
The limit of the second factory is 2, and it fixed 1 robot.
The total distance is |2 - 0| + |2 - 4| + |6 - 6| = 4. It can be shown that we cannot achieve a better total distance than 4.
**Example 2:**
**Input:** robot = \[1,-1\], factory = \[\[-2,1\],\[2,1\]\]
**Output:** 2
**Explanation:** As shown in the figure:
- The first robot at position 1 moves in the positive direction. It will be repaired at the second factory.
- The second robot at position -1 moves in the negative direction. It will be repaired at the first factory.
The limit of the first factory is 1, and it fixed 1 robot.
The limit of the second factory is 1, and it fixed 1 robot.
The total distance is |2 - 1| + |(-2) - (-1)| = 2. It can be shown that we cannot achieve a better total distance than 2.
**Constraints:**
* `1 <= robot.length, factory.length <= 100`
* `factory[j].length == 2`
* `-109 <= robot[i], positionj <= 109`
* `0 <= limitj <= robot.length`
* The input will be generated such that it is always possible to repair every robot. | null |
[Python3] O(MN) DP | minimum-total-distance-traveled | 0 | 1 | Please pull this [commit](https://github.com/gaosanyong/leetcode/commit/6a94b729b8594be1319f14da1cf3c97ddb12a427) for solutions of weekly 318.\n\nThis is an overkill for this problem as cubic solutions can pass the OJ as well. But a quadratic solution is possible. \n\n**Intuition**\nHere, I define `dp[i][j]` as the minimum moves to fix `robot[i:]` with `factory[j:]`. Clearly, `dp[0][0]` is the desired answer. The recurrence relation is \n\n```\ndp[i][j] = min(dp[i][j+1], \n\t\t\t |robot[i] - pos| + dp[i+1][j+1], \n\t\t\t |robot[i] - pos| + |robot[i+1] - pos| + dp[i+2][j+1], \n\t\t\t ...\n\t\t\t |robot[i] - pos| + ... + |robot[i+limit-1] - pos| + dp[i+limit][j+1])\n```\nThis can be rewritten as \n```\ndp[i][j] = |robot[i] - pos| + ... + |robot[i+limit-1] - pos| + min(dp[i][j+1] - |robot[i] - pos| - ... - |robot[i+limit-1][j+1]|, \n\t\t dp[i+1][j+1] - |robot[i+1] - pos| - ... - |robot[i+limit-1][j+1]|, \n\t\t\t\t\t\t\t\t\t\t\t\t\t\t\t\t ... \n\t\t\t\t\t\t\t\t\t\t\t\t\t\t\t\t dp[i+limit][j+1]) \n```\nWhat\'s nice about this form is that I can break `dp[i][j]` into two parts \n* prefix sum of absolute differences \n* minimum of `dp[*][j+1]` - prefix sum. \n\nNotice that even though the prefix sum in the 2nd part differ for `dp[*][j+1]` it happens to be the prefix sum when `dp[*][j]` is being computed. To elaborate, when I compute `dp[i][j]` the prefix sum is `|robot[i] - pos| + ... + |robot[i+limit-1] - pos|` and this is the term trailing `dp[i][j+1]` in the min calculation. Similarly, when computing `dp[i+1][j]` the prefix sum is `|robot[i+1] - pos| + ... + |robot[i+limit-1] - pos|` which happens to be the term trailing `dp[i+1][j+1]` in the min calculation. \n\nWith this breakdown, I can throw `dp[*][j+1] - prefix` into a mono-deque to compute the minimum in `O(1)` time, which is essentially a running minimum with fixed window. \n\nSo the overall time complexity of this implementation is `O(MN)`. The space complexity is also `O(MN)`. \n\n```\nclass Solution:\n def minimumTotalDistance(self, robot: List[int], factory: List[List[int]]) -> int:\n robot.sort()\n factory.sort()\n m, n = len(robot), len(factory)\n dp = [[0]*(n+1) for _ in range(m+1)] \n for i in range(m): dp[i][-1] = inf \n for j in range(n-1, -1, -1): \n prefix = 0 \n qq = deque([(m, 0)])\n for i in range(m-1, -1, -1): \n prefix += abs(robot[i] - factory[j][0])\n if qq[0][0] > i+factory[j][1]: qq.popleft()\n while qq and qq[-1][1] >= dp[i][j+1] - prefix: qq.pop()\n qq.append((i, dp[i][j+1] - prefix))\n dp[i][j] = qq[0][1] + prefix\n return dp[0][0]\n``` | 16 | There are some robots and factories on the X-axis. You are given an integer array `robot` where `robot[i]` is the position of the `ith` robot. You are also given a 2D integer array `factory` where `factory[j] = [positionj, limitj]` indicates that `positionj` is the position of the `jth` factory and that the `jth` factory can repair at most `limitj` robots.
The positions of each robot are **unique**. The positions of each factory are also **unique**. Note that a robot can be **in the same position** as a factory initially.
All the robots are initially broken; they keep moving in one direction. The direction could be the negative or the positive direction of the X-axis. When a robot reaches a factory that did not reach its limit, the factory repairs the robot, and it stops moving.
**At any moment**, you can set the initial direction of moving for **some** robot. Your target is to minimize the total distance traveled by all the robots.
Return _the minimum total distance traveled by all the robots_. The test cases are generated such that all the robots can be repaired.
**Note that**
* All robots move at the same speed.
* If two robots move in the same direction, they will never collide.
* If two robots move in opposite directions and they meet at some point, they do not collide. They cross each other.
* If a robot passes by a factory that reached its limits, it crosses it as if it does not exist.
* If the robot moved from a position `x` to a position `y`, the distance it moved is `|y - x|`.
**Example 1:**
**Input:** robot = \[0,4,6\], factory = \[\[2,2\],\[6,2\]\]
**Output:** 4
**Explanation:** As shown in the figure:
- The first robot at position 0 moves in the positive direction. It will be repaired at the first factory.
- The second robot at position 4 moves in the negative direction. It will be repaired at the first factory.
- The third robot at position 6 will be repaired at the second factory. It does not need to move.
The limit of the first factory is 2, and it fixed 2 robots.
The limit of the second factory is 2, and it fixed 1 robot.
The total distance is |2 - 0| + |2 - 4| + |6 - 6| = 4. It can be shown that we cannot achieve a better total distance than 4.
**Example 2:**
**Input:** robot = \[1,-1\], factory = \[\[-2,1\],\[2,1\]\]
**Output:** 2
**Explanation:** As shown in the figure:
- The first robot at position 1 moves in the positive direction. It will be repaired at the second factory.
- The second robot at position -1 moves in the negative direction. It will be repaired at the first factory.
The limit of the first factory is 1, and it fixed 1 robot.
The limit of the second factory is 1, and it fixed 1 robot.
The total distance is |2 - 1| + |(-2) - (-1)| = 2. It can be shown that we cannot achieve a better total distance than 2.
**Constraints:**
* `1 <= robot.length, factory.length <= 100`
* `factory[j].length == 2`
* `-109 <= robot[i], positionj <= 109`
* `0 <= limitj <= robot.length`
* The input will be generated such that it is always possible to repair every robot. | null |
[Python] assignment problem with scipy | minimum-total-distance-traveled | 0 | 1 | classic assignment problem, scipy implements linear_sum_assignment with [Jonker-Volgenant/Hungarian algorithm](https://docs.scipy.org/doc/scipy/reference/generated/scipy.optimize.linear_sum_assignment.html). Time complexity is o(n^3). \n\nAssignment solution treats robots and factories as bipatite graph, it didn\'t utilize the fact that all robots and factories share the same number axis, which was why DP solution with sorting achieves quadratic complexity. \n\n\n```\nfrom scipy import optimize\nimport numpy\n\nclass Solution:\n def minimumTotalDistance(self, rs: List[int], fs: List[List[int]]) -> int:\n costs = []\n for i, k in fs:\n c = [abs(j-i) for j in rs]\n for _ in range(k):\n costs.append(c)\n costs = numpy.array(costs)\n return costs[optimize.linear_sum_assignment(costs)].sum() \n \n``` | 10 | There are some robots and factories on the X-axis. You are given an integer array `robot` where `robot[i]` is the position of the `ith` robot. You are also given a 2D integer array `factory` where `factory[j] = [positionj, limitj]` indicates that `positionj` is the position of the `jth` factory and that the `jth` factory can repair at most `limitj` robots.
The positions of each robot are **unique**. The positions of each factory are also **unique**. Note that a robot can be **in the same position** as a factory initially.
All the robots are initially broken; they keep moving in one direction. The direction could be the negative or the positive direction of the X-axis. When a robot reaches a factory that did not reach its limit, the factory repairs the robot, and it stops moving.
**At any moment**, you can set the initial direction of moving for **some** robot. Your target is to minimize the total distance traveled by all the robots.
Return _the minimum total distance traveled by all the robots_. The test cases are generated such that all the robots can be repaired.
**Note that**
* All robots move at the same speed.
* If two robots move in the same direction, they will never collide.
* If two robots move in opposite directions and they meet at some point, they do not collide. They cross each other.
* If a robot passes by a factory that reached its limits, it crosses it as if it does not exist.
* If the robot moved from a position `x` to a position `y`, the distance it moved is `|y - x|`.
**Example 1:**
**Input:** robot = \[0,4,6\], factory = \[\[2,2\],\[6,2\]\]
**Output:** 4
**Explanation:** As shown in the figure:
- The first robot at position 0 moves in the positive direction. It will be repaired at the first factory.
- The second robot at position 4 moves in the negative direction. It will be repaired at the first factory.
- The third robot at position 6 will be repaired at the second factory. It does not need to move.
The limit of the first factory is 2, and it fixed 2 robots.
The limit of the second factory is 2, and it fixed 1 robot.
The total distance is |2 - 0| + |2 - 4| + |6 - 6| = 4. It can be shown that we cannot achieve a better total distance than 4.
**Example 2:**
**Input:** robot = \[1,-1\], factory = \[\[-2,1\],\[2,1\]\]
**Output:** 2
**Explanation:** As shown in the figure:
- The first robot at position 1 moves in the positive direction. It will be repaired at the second factory.
- The second robot at position -1 moves in the negative direction. It will be repaired at the first factory.
The limit of the first factory is 1, and it fixed 1 robot.
The limit of the second factory is 1, and it fixed 1 robot.
The total distance is |2 - 1| + |(-2) - (-1)| = 2. It can be shown that we cannot achieve a better total distance than 2.
**Constraints:**
* `1 <= robot.length, factory.length <= 100`
* `factory[j].length == 2`
* `-109 <= robot[i], positionj <= 109`
* `0 <= limitj <= robot.length`
* The input will be generated such that it is always possible to repair every robot. | null |
Python || Easy || Sorting || O(nlogn) Solution | number-of-distinct-averages | 0 | 1 | ```\nclass Solution:\n def distinctAverages(self, nums: List[int]) -> int:\n n=len(nums)\n i=0\n j=n-1\n s=set()\n nums.sort()\n while i<=j:\n s.add((nums[i]+nums[j])/2)\n i+=1\n j-=1\n return len(s)\n```\n\n**Upvote if you like the solution or ask if there is any query** | 1 | You are given a **0-indexed** integer array `nums` of **even** length.
As long as `nums` is **not** empty, you must repetitively:
* Find the minimum number in `nums` and remove it.
* Find the maximum number in `nums` and remove it.
* Calculate the average of the two removed numbers.
The **average** of two numbers `a` and `b` is `(a + b) / 2`.
* For example, the average of `2` and `3` is `(2 + 3) / 2 = 2.5`.
Return _the number of **distinct** averages calculated using the above process_.
**Note** that when there is a tie for a minimum or maximum number, any can be removed.
**Example 1:**
**Input:** nums = \[4,1,4,0,3,5\]
**Output:** 2
**Explanation:**
1. Remove 0 and 5, and the average is (0 + 5) / 2 = 2.5. Now, nums = \[4,1,4,3\].
2. Remove 1 and 4. The average is (1 + 4) / 2 = 2.5, and nums = \[4,3\].
3. Remove 3 and 4, and the average is (3 + 4) / 2 = 3.5.
Since there are 2 distinct numbers among 2.5, 2.5, and 3.5, we return 2.
**Example 2:**
**Input:** nums = \[1,100\]
**Output:** 1
**Explanation:**
There is only one average to be calculated after removing 1 and 100, so we return 1.
**Constraints:**
* `2 <= nums.length <= 100`
* `nums.length` is even.
* `0 <= nums[i] <= 100` | null |
✅✅[ Python ] 🐍🐍 Simple Python Solution ✅✅ | number-of-distinct-averages | 0 | 1 | ```\nclass Solution:\n def distinctAverages(self, nums: List[int]) -> int:\n a=[]\n for i in range(len(nums)//2):\n a.append((max(nums)+min(nums))/2)\n nums.remove(max(nums))\n nums.remove(min(nums))\n b=set(a)\n print(a)\n print(b)\n return len(b)\n``` | 1 | You are given a **0-indexed** integer array `nums` of **even** length.
As long as `nums` is **not** empty, you must repetitively:
* Find the minimum number in `nums` and remove it.
* Find the maximum number in `nums` and remove it.
* Calculate the average of the two removed numbers.
The **average** of two numbers `a` and `b` is `(a + b) / 2`.
* For example, the average of `2` and `3` is `(2 + 3) / 2 = 2.5`.
Return _the number of **distinct** averages calculated using the above process_.
**Note** that when there is a tie for a minimum or maximum number, any can be removed.
**Example 1:**
**Input:** nums = \[4,1,4,0,3,5\]
**Output:** 2
**Explanation:**
1. Remove 0 and 5, and the average is (0 + 5) / 2 = 2.5. Now, nums = \[4,1,4,3\].
2. Remove 1 and 4. The average is (1 + 4) / 2 = 2.5, and nums = \[4,3\].
3. Remove 3 and 4, and the average is (3 + 4) / 2 = 3.5.
Since there are 2 distinct numbers among 2.5, 2.5, and 3.5, we return 2.
**Example 2:**
**Input:** nums = \[1,100\]
**Output:** 1
**Explanation:**
There is only one average to be calculated after removing 1 and 100, so we return 1.
**Constraints:**
* `2 <= nums.length <= 100`
* `nums.length` is even.
* `0 <= nums[i] <= 100` | null |
[Python Answer🤫🐍🐍🐍] Heap and Set Solution | number-of-distinct-averages | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\nUse a heap to remember the highest and lowest values\nUse a set to remember the averages\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\nO(nlogn)\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\nO(n)\n\n# Code\n```\nclass Solution:\n def distinctAverages(self, nums: List[int]) -> int:\n \n h1 = nums.copy()\n \n h2 = [-i for i in nums.copy()]\n \n ans = set()\n \n heapify(h1)\n heapify(h2)\n \n while h1 and h2:\n \n n1 = heappop(h1)\n n2= - heappop(h2)\n\n ans.add(mean([n2,n1]))\n\n return len(ans)\n``` | 1 | You are given a **0-indexed** integer array `nums` of **even** length.
As long as `nums` is **not** empty, you must repetitively:
* Find the minimum number in `nums` and remove it.
* Find the maximum number in `nums` and remove it.
* Calculate the average of the two removed numbers.
The **average** of two numbers `a` and `b` is `(a + b) / 2`.
* For example, the average of `2` and `3` is `(2 + 3) / 2 = 2.5`.
Return _the number of **distinct** averages calculated using the above process_.
**Note** that when there is a tie for a minimum or maximum number, any can be removed.
**Example 1:**
**Input:** nums = \[4,1,4,0,3,5\]
**Output:** 2
**Explanation:**
1. Remove 0 and 5, and the average is (0 + 5) / 2 = 2.5. Now, nums = \[4,1,4,3\].
2. Remove 1 and 4. The average is (1 + 4) / 2 = 2.5, and nums = \[4,3\].
3. Remove 3 and 4, and the average is (3 + 4) / 2 = 3.5.
Since there are 2 distinct numbers among 2.5, 2.5, and 3.5, we return 2.
**Example 2:**
**Input:** nums = \[1,100\]
**Output:** 1
**Explanation:**
There is only one average to be calculated after removing 1 and 100, so we return 1.
**Constraints:**
* `2 <= nums.length <= 100`
* `nums.length` is even.
* `0 <= nums[i] <= 100` | null |
✅✅easy python solution|| easy to understand | number-of-distinct-averages | 0 | 1 | ```\nclass Solution:\n def distinctAverages(self, nums: List[int]) -> int:\n lis = []\n \n while nums!=[]:\n if (min(nums)+max(nums))/2 not in lis:\n lis.append((min(nums)+max(nums))/2)\n nums.remove(max(nums))\n nums.remove(min(nums))\n return len(lis)\n \n \n``` | 1 | You are given a **0-indexed** integer array `nums` of **even** length.
As long as `nums` is **not** empty, you must repetitively:
* Find the minimum number in `nums` and remove it.
* Find the maximum number in `nums` and remove it.
* Calculate the average of the two removed numbers.
The **average** of two numbers `a` and `b` is `(a + b) / 2`.
* For example, the average of `2` and `3` is `(2 + 3) / 2 = 2.5`.
Return _the number of **distinct** averages calculated using the above process_.
**Note** that when there is a tie for a minimum or maximum number, any can be removed.
**Example 1:**
**Input:** nums = \[4,1,4,0,3,5\]
**Output:** 2
**Explanation:**
1. Remove 0 and 5, and the average is (0 + 5) / 2 = 2.5. Now, nums = \[4,1,4,3\].
2. Remove 1 and 4. The average is (1 + 4) / 2 = 2.5, and nums = \[4,3\].
3. Remove 3 and 4, and the average is (3 + 4) / 2 = 3.5.
Since there are 2 distinct numbers among 2.5, 2.5, and 3.5, we return 2.
**Example 2:**
**Input:** nums = \[1,100\]
**Output:** 1
**Explanation:**
There is only one average to be calculated after removing 1 and 100, so we return 1.
**Constraints:**
* `2 <= nums.length <= 100`
* `nums.length` is even.
* `0 <= nums[i] <= 100` | null |
[Python3] set | number-of-distinct-averages | 0 | 1 | Please pull this [commit](https://github.com/gaosanyong/leetcode/commit/e8b87d04cc192c5227286692921910fe93fee05d) for solutions of biweekly 91. \n\n```\nclass Solution:\n def distinctAverages(self, nums: List[int]) -> int:\n nums.sort()\n seen = set()\n for i in range(len(nums)//2): \n seen.add((nums[i] + nums[~i])/2)\n return len(seen)\n``` | 1 | You are given a **0-indexed** integer array `nums` of **even** length.
As long as `nums` is **not** empty, you must repetitively:
* Find the minimum number in `nums` and remove it.
* Find the maximum number in `nums` and remove it.
* Calculate the average of the two removed numbers.
The **average** of two numbers `a` and `b` is `(a + b) / 2`.
* For example, the average of `2` and `3` is `(2 + 3) / 2 = 2.5`.
Return _the number of **distinct** averages calculated using the above process_.
**Note** that when there is a tie for a minimum or maximum number, any can be removed.
**Example 1:**
**Input:** nums = \[4,1,4,0,3,5\]
**Output:** 2
**Explanation:**
1. Remove 0 and 5, and the average is (0 + 5) / 2 = 2.5. Now, nums = \[4,1,4,3\].
2. Remove 1 and 4. The average is (1 + 4) / 2 = 2.5, and nums = \[4,3\].
3. Remove 3 and 4, and the average is (3 + 4) / 2 = 3.5.
Since there are 2 distinct numbers among 2.5, 2.5, and 3.5, we return 2.
**Example 2:**
**Input:** nums = \[1,100\]
**Output:** 1
**Explanation:**
There is only one average to be calculated after removing 1 and 100, so we return 1.
**Constraints:**
* `2 <= nums.length <= 100`
* `nums.length` is even.
* `0 <= nums[i] <= 100` | null |
Easy Python Solution || using two pointers 99.80% runtime | number-of-distinct-averages | 0 | 1 | 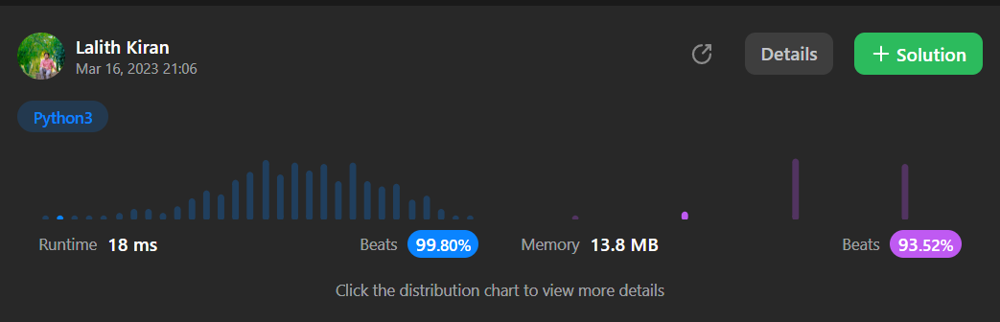\n\n# Code\n```\nclass Solution:\n def distinctAverages(self, nums: List[int]) -> int:\n nums.sort()\n i=0\n j=len(nums)-1\n l=[]\n while i<j:\n l.append((nums[i]+nums[j])/2)\n i+=1\n j-=1\n return len(set(l))\n``` | 2 | You are given a **0-indexed** integer array `nums` of **even** length.
As long as `nums` is **not** empty, you must repetitively:
* Find the minimum number in `nums` and remove it.
* Find the maximum number in `nums` and remove it.
* Calculate the average of the two removed numbers.
The **average** of two numbers `a` and `b` is `(a + b) / 2`.
* For example, the average of `2` and `3` is `(2 + 3) / 2 = 2.5`.
Return _the number of **distinct** averages calculated using the above process_.
**Note** that when there is a tie for a minimum or maximum number, any can be removed.
**Example 1:**
**Input:** nums = \[4,1,4,0,3,5\]
**Output:** 2
**Explanation:**
1. Remove 0 and 5, and the average is (0 + 5) / 2 = 2.5. Now, nums = \[4,1,4,3\].
2. Remove 1 and 4. The average is (1 + 4) / 2 = 2.5, and nums = \[4,3\].
3. Remove 3 and 4, and the average is (3 + 4) / 2 = 3.5.
Since there are 2 distinct numbers among 2.5, 2.5, and 3.5, we return 2.
**Example 2:**
**Input:** nums = \[1,100\]
**Output:** 1
**Explanation:**
There is only one average to be calculated after removing 1 and 100, so we return 1.
**Constraints:**
* `2 <= nums.length <= 100`
* `nums.length` is even.
* `0 <= nums[i] <= 100` | null |
Easy Python Solution | number-of-distinct-averages | 0 | 1 | # Code\n```\nclass Solution:\n def distinctAverages(self, nums: List[int]) -> int:\n av=[]\n nums.sort()\n while nums:\n av.append((nums[-1]+nums[0])/2)\n nums.pop(-1)\n nums.pop(0)\n return len(set(av))\n``` | 3 | You are given a **0-indexed** integer array `nums` of **even** length.
As long as `nums` is **not** empty, you must repetitively:
* Find the minimum number in `nums` and remove it.
* Find the maximum number in `nums` and remove it.
* Calculate the average of the two removed numbers.
The **average** of two numbers `a` and `b` is `(a + b) / 2`.
* For example, the average of `2` and `3` is `(2 + 3) / 2 = 2.5`.
Return _the number of **distinct** averages calculated using the above process_.
**Note** that when there is a tie for a minimum or maximum number, any can be removed.
**Example 1:**
**Input:** nums = \[4,1,4,0,3,5\]
**Output:** 2
**Explanation:**
1. Remove 0 and 5, and the average is (0 + 5) / 2 = 2.5. Now, nums = \[4,1,4,3\].
2. Remove 1 and 4. The average is (1 + 4) / 2 = 2.5, and nums = \[4,3\].
3. Remove 3 and 4, and the average is (3 + 4) / 2 = 3.5.
Since there are 2 distinct numbers among 2.5, 2.5, and 3.5, we return 2.
**Example 2:**
**Input:** nums = \[1,100\]
**Output:** 1
**Explanation:**
There is only one average to be calculated after removing 1 and 100, so we return 1.
**Constraints:**
* `2 <= nums.length <= 100`
* `nums.length` is even.
* `0 <= nums[i] <= 100` | null |
[Java/Python 3] Put into HashSet Int instead of double/float. | number-of-distinct-averages | 1 | 1 | Essentially a two pointers algorithm is impelemented.\n```java\n public int distinctAverages(int[] nums) {\n Set<Integer> seen = new HashSet<>();\n Arrays.sort(nums);\n for (int i = 0, n = nums.length; i < n / 2; ++i) {\n seen.add(nums[i] + nums[n - 1 - i]);\n }\n return seen.size();\n }\n```\n\nNote: when `i` iterates from `0` to `n - 1`, `~i` iterates for `-1` to `-n` at the same time.\n\n\n```python\n def distinctAverages(self, nums: List[int]) -> int:\n a, n = sorted(nums), len(nums) // 2\n return len({a[i] + a[~i] for i in range(n)})\n```\n\nUse Deque.\n```java\n public int distinctAverages(int[] nums) {\n Set<Integer> seen = new HashSet<>();\n Arrays.sort(nums);\n Deque<Integer> dq = new ArrayDeque<>();\n for (int n : nums) {\n dq.offer(n);\n }\n while (!dq.isEmpty()) {\n seen.add(dq.poll() + dq.pollLast());\n }\n return seen.size();\n }\n```\n\n\n**Analysis:**\n\nSorting is the major part of the time cost.\n\nTime: `O(nlogn)`, space: `O(n)`, where `n = nums.length`. | 18 | You are given a **0-indexed** integer array `nums` of **even** length.
As long as `nums` is **not** empty, you must repetitively:
* Find the minimum number in `nums` and remove it.
* Find the maximum number in `nums` and remove it.
* Calculate the average of the two removed numbers.
The **average** of two numbers `a` and `b` is `(a + b) / 2`.
* For example, the average of `2` and `3` is `(2 + 3) / 2 = 2.5`.
Return _the number of **distinct** averages calculated using the above process_.
**Note** that when there is a tie for a minimum or maximum number, any can be removed.
**Example 1:**
**Input:** nums = \[4,1,4,0,3,5\]
**Output:** 2
**Explanation:**
1. Remove 0 and 5, and the average is (0 + 5) / 2 = 2.5. Now, nums = \[4,1,4,3\].
2. Remove 1 and 4. The average is (1 + 4) / 2 = 2.5, and nums = \[4,3\].
3. Remove 3 and 4, and the average is (3 + 4) / 2 = 3.5.
Since there are 2 distinct numbers among 2.5, 2.5, and 3.5, we return 2.
**Example 2:**
**Input:** nums = \[1,100\]
**Output:** 1
**Explanation:**
There is only one average to be calculated after removing 1 and 100, so we return 1.
**Constraints:**
* `2 <= nums.length <= 100`
* `nums.length` is even.
* `0 <= nums[i] <= 100` | null |
Python | Easy Solution✅ | number-of-distinct-averages | 0 | 1 | # Code\n```\nclass Solution:\n def distinctAverages(self, nums: List[int]) -> int:\n distinct = set()\n while len(nums) != 0:\n nums = sorted(nums)\n avg = (nums.pop(0) + nums.pop(len(nums)-1))/2\n distinct.add(avg)\n return len(distinct)\n``` | 3 | You are given a **0-indexed** integer array `nums` of **even** length.
As long as `nums` is **not** empty, you must repetitively:
* Find the minimum number in `nums` and remove it.
* Find the maximum number in `nums` and remove it.
* Calculate the average of the two removed numbers.
The **average** of two numbers `a` and `b` is `(a + b) / 2`.
* For example, the average of `2` and `3` is `(2 + 3) / 2 = 2.5`.
Return _the number of **distinct** averages calculated using the above process_.
**Note** that when there is a tie for a minimum or maximum number, any can be removed.
**Example 1:**
**Input:** nums = \[4,1,4,0,3,5\]
**Output:** 2
**Explanation:**
1. Remove 0 and 5, and the average is (0 + 5) / 2 = 2.5. Now, nums = \[4,1,4,3\].
2. Remove 1 and 4. The average is (1 + 4) / 2 = 2.5, and nums = \[4,3\].
3. Remove 3 and 4, and the average is (3 + 4) / 2 = 3.5.
Since there are 2 distinct numbers among 2.5, 2.5, and 3.5, we return 2.
**Example 2:**
**Input:** nums = \[1,100\]
**Output:** 1
**Explanation:**
There is only one average to be calculated after removing 1 and 100, so we return 1.
**Constraints:**
* `2 <= nums.length <= 100`
* `nums.length` is even.
* `0 <= nums[i] <= 100` | null |
Python shortest 1-liner. Recursive DP. Functional programming. | count-ways-to-build-good-strings | 0 | 1 | # Approach\n1. Say there is a function `f`, which returns the number of good strings possible with lengths between `low (l)` and `high (h)`, given that the string is already of length `n`.\n i.e `def f(n: int) -> int: ...`\n\n2. In ideal case, recursively try both options of appending `zero` number of `0`s or `one` number of `1`s to the string, and `add` the results.\n\n\n3. There can only be 3 possiblities, so function `f` would look:\n Note: Add `@cache` to memoize the results.\n ```python\n @cache\n def f(n: int) -> int:\n if n > h: return 0\n if n < l: return f(n + zero) + f(n + one)\n if l <= n <= h: return 1 + f(n + zero) + f(n + one)\n ```\n\n4. These 3 possibilities can be combined into one statement as:\n ```python\n @cache\n def f(n: int) -> int:\n return n <= h and (n >= l) + f(n + zero) + f(n + one)\n ```\n\n5. To make it fun, the entire 3 line can be reduced to a 1-liner as shown in the final solution below.\n\n# Complexity\n- Time complexity: $$O(h)$$\n\n- Space complexity: $$O(h)$$\n\nwhere, `h = high`.\n\n# Code\n```python\nclass Solution:\n def countGoodStrings(self, l: int, h: int, zero: int, one: int) -> int:\n return (f := cache(lambda n: n <= h and ((n >= l) + f(n + zero) + f(n + one)) % 1_000_000_007))(0)\n\n\n``` | 2 | Given the integers `zero`, `one`, `low`, and `high`, we can construct a string by starting with an empty string, and then at each step perform either of the following:
* Append the character `'0'` `zero` times.
* Append the character `'1'` `one` times.
This can be performed any number of times.
A **good** string is a string constructed by the above process having a **length** between `low` and `high` (**inclusive**).
Return _the number of **different** good strings that can be constructed satisfying these properties._ Since the answer can be large, return it **modulo** `109 + 7`.
**Example 1:**
**Input:** low = 3, high = 3, zero = 1, one = 1
**Output:** 8
**Explanation:**
One possible valid good string is "011 ".
It can be constructed as follows: " " -> "0 " -> "01 " -> "011 ".
All binary strings from "000 " to "111 " are good strings in this example.
**Example 2:**
**Input:** low = 2, high = 3, zero = 1, one = 2
**Output:** 5
**Explanation:** The good strings are "00 ", "11 ", "000 ", "110 ", and "011 ".
**Constraints:**
* `1 <= low <= high <= 105`
* `1 <= zero, one <= low` | null |
Python Elegant & Short | Top-Down DP | Memoization | count-ways-to-build-good-strings | 0 | 1 | # Complexity\n\n- Time complexity: $$O(n)$$\n- Space complexity: $$O(n)$$\n\n# Code\n```\nclass Solution:\n MOD = 1_000_000_007\n\n def countGoodStrings(self, low: int, high: int, zero: int, one: int) -> int:\n @cache\n def dp(length: int) -> int:\n if length > high:\n return 0\n return (\n int(low <= length) +\n dp(length + zero) +\n dp(length + one)\n ) % self.MOD\n\n return dp(0)\n```\n | 2 | Given the integers `zero`, `one`, `low`, and `high`, we can construct a string by starting with an empty string, and then at each step perform either of the following:
* Append the character `'0'` `zero` times.
* Append the character `'1'` `one` times.
This can be performed any number of times.
A **good** string is a string constructed by the above process having a **length** between `low` and `high` (**inclusive**).
Return _the number of **different** good strings that can be constructed satisfying these properties._ Since the answer can be large, return it **modulo** `109 + 7`.
**Example 1:**
**Input:** low = 3, high = 3, zero = 1, one = 1
**Output:** 8
**Explanation:**
One possible valid good string is "011 ".
It can be constructed as follows: " " -> "0 " -> "01 " -> "011 ".
All binary strings from "000 " to "111 " are good strings in this example.
**Example 2:**
**Input:** low = 2, high = 3, zero = 1, one = 2
**Output:** 5
**Explanation:** The good strings are "00 ", "11 ", "000 ", "110 ", and "011 ".
**Constraints:**
* `1 <= low <= high <= 105`
* `1 <= zero, one <= low` | null |
🚀 Beats 100% | Java, C++, Python3 | Forward-style DP iterative solution | count-ways-to-build-good-strings | 1 | 1 | > \u26A0\uFE0F **Disclaimer**: The original solution was crafted in Java. Thus, when we mention "Beats 100%" it applies specifically to Java submissions. The performance may vary for other languages.\n\n**Dont forget to upvote if you like the content below. \uD83D\uDE43**\n\n\n[TOC]\n\n# Problem\nThe problem involves creating "good strings" with a length within the range `[low, high]`. A "good string" is defined as a string that does not have more than `zero` consecutive \'0\'s and `one` consecutive \'1\'s.\n\n---\n\n# Normal solution (Beats 100% time)\n\n## Approach\nThe approach is based on Dynamic Programming (DP). \n\nFirst, a check is made to see if `zero > one`. If this is the case, we call the same function but swap `zero` and `one`. This is due to the fact that the type of characters (0 or 1) is interchangeable, and we want to ensure `zero <= one` for the following steps.\n\nA DP array `dp` of size `high + 1` is then initialized, where `dp[i]` means how many "good strings" of length `i` we can build, with `dp[0] = 1`, as there is one way to build a string of length zero: an empty string.\n\nWe then iterate over `i` from 1 to `high`:\n\n- If `i` is greater than or equal to `one`, we set `dp[i]` to `dp[i - zero] + dp[i - one]`. This update signifies the ways of building strings of length `i` by appending a \'0\' to strings of `i-zero` lengths and \'1\' to strings of `i-one` lengths, while adhering to the maximum consecutive counts.\n\n- If `i` is less than `one` but greater than or equal to `zero`, we set `dp[i]` to `dp[i - zero]`. *This little optimisation was made because after some time `i` will always be greater than `zero` and execution will not ever fall into this branch again.*\n\n- If `i` is greater than or equal to `low`, we update our answer `ans` by adding `dp[i]`. Strings of length `i` contribute to our final count of good strings.\n\nFinally, we return `ans` modulo `MOD`, as the problem asks for the answer modulo `1e9 + 7`.\n\n\n**Note:** according to modular arithmetic, `(a + b) % c = (a % c + b % c) % c`. This property allows us to take the modulus at each step rather than at the end, preventing potential overflow if `a + b` exceeds the limit of the data type.\n\n\n## Complexity\n- Time complexity: $$O(high)$$ due to the single pass through the `dp` array.\n- Space complexity: $$O(high)$$ as we use a `dp` array of size `high + 1`.\n\n## Code\n```java []\nclass Solution {\n private static final int MOD = (int) 1e9 + 7;\n\n public int countGoodStrings(int low, int high, int zero, int one) {\n if (zero > one) {\n return countGoodStrings(low, high, one, zero);\n }\n int[] dp = new int[high + 1];\n dp[0] = 1;\n int ans = 0;\n for (int i = 1; i <= high; i++) {\n if (i >= one) {\n dp[i] = (dp[i - zero] + dp[i - one]) % MOD;\n } else if (i >= zero) {\n dp[i] = dp[i - zero] % MOD;\n }\n if (i >= low) {\n ans = (ans + dp[i]) % MOD;\n }\n }\n return ans;\n }\n}\n```\n```cpp []\nclass Solution {\npublic:\n static const int MOD = 1e9 + 7;\n\n int countGoodStrings(int low, int high, int zero, int one) {\n if (zero > one) {\n return countGoodStrings(low, high, one, zero);\n }\n vector<int> dp(high + 1, 0);\n dp[0] = 1;\n int ans = 0;\n for (int i = 1; i <= high; i++) {\n if (i >= one) {\n dp[i] = (dp[i - zero] + dp[i - one]) % MOD;\n } else if (i >= zero) {\n dp[i] = dp[i - zero] % MOD;\n }\n if (i >= low) {\n ans = (ans + dp[i]) % MOD;\n }\n }\n return ans;\n }\n};\n```\n``` python3 []\nclass Solution:\n MOD = 10**9 + 7\n\n def countGoodStrings(self, low: int, high: int, zero: int, one: int) -> int:\n if zero > one:\n zero, one = one, zero\n dp = [0] * (high + 1)\n dp[0] = 1\n ans = 0\n for i in range(1, high + 1):\n if i >= one:\n dp[i] = (dp[i - zero] + dp[i - one]) % self.MOD\n elif i >= zero:\n dp[i] = dp[i - zero] % self.MOD\n if i >= low:\n ans = (ans + dp[i]) % self.MOD\n return ans\n\n```\n---\n# Optimised solution (Beats 100% time and memory)\n\n## Approach\nThe approach is based on optimization through the GCD of `zero` and `one` and reducing space for `dp`.\n\nThe function `countGoodStrings` first calculates the GCD of `zero` and `one` using Stein\'s algorithm. It then calls a helper function `count` with `low`, `high`, `zero`, and `one` divided by the GCD. This scales down the problem space, effectively reducing the time and space complexities.\n\nThe helper function `count` follows the same logic as the non-optimized solution, but with `zero` and `one` divided by the GCD, and the `dp` array size is now `one+1` (also divided by the GCD). In addition, the `dp` array is now indexed using the modulus operator to save space.\n\n*Instead of keeping track of all `i` from 1 to `high`, this solution only maintains a window of the last `one + 1` results (`dp[i % n]`). This is because for any `i` larger than `one`, the result only depends on the `i - one` and `i - zero` results. This technique significantly reduces the space requirement.*\n\n## Complexity\nLet\'s denote `GCD` as `g`. \n\n- Time complexity: $$O(\\log(\\min(zero, one))) + O(\\frac{high}{g})$$ due to Stein\'s algorithm and the DP loop.\n- Space complexity: $$O(\\frac{one}{g})$$ as we use a `dp` array of size `one/g + 1`.\n\n## Code\n``` java\nclass Solution {\n private static final int MOD = (int) 1e9 + 7;\n\n public int countGoodStrings(int low, int high, int zero, int one) {\n int gcd = gcdBySteinsAlgorithm(zero, one);\n return count((low + gcd - 1) / gcd, high / gcd, zero / gcd, one / gcd);\n }\n\n private static int count(int low, int high, int zero, int one) {\n if (zero > one) {\n return count(low, high, one, zero);\n }\n int n = one + 1;\n int[] dp = new int[one + 1];\n dp[0] = 1;\n int ans = 0;\n for (int i = 1; i <= high; i++) {\n if (i >= one) {\n dp[i % n] = (dp[(i - zero) % n] + dp[(i - one) % n]) % MOD;\n } else if (i >= zero) {\n dp[i % n] = (dp[(i - zero) % n]) % MOD;\n }\n if (i >= low) {\n ans = (ans + dp[i % n]) % MOD;\n }\n }\n return ans;\n }\n\n private static int gcdBySteinsAlgorithm(int n1, int n2) {\n if (n1 == 0) {\n return n2;\n }\n if (n2 == 0) {\n return n1;\n }\n\n int n;\n for (n = 0; ((n1 | n2) & 1) == 0; n++) {\n n1 >>= 1;\n n2 >>= 1;\n }\n\n while ((n1 & 1) == 0) {\n n1 >>= 1;\n }\n\n do {\n while ((n2 & 1) == 0) {\n n2 >>= 1;\n }\n if (n1 > n2) {\n int temp = n1;\n n1 = n2;\n n2 = temp;\n }\n n2 = (n2 - n1);\n } while (n2 != 0);\n\n return n1 << n;\n }\n}\n```\n\n | 1 | Given the integers `zero`, `one`, `low`, and `high`, we can construct a string by starting with an empty string, and then at each step perform either of the following:
* Append the character `'0'` `zero` times.
* Append the character `'1'` `one` times.
This can be performed any number of times.
A **good** string is a string constructed by the above process having a **length** between `low` and `high` (**inclusive**).
Return _the number of **different** good strings that can be constructed satisfying these properties._ Since the answer can be large, return it **modulo** `109 + 7`.
**Example 1:**
**Input:** low = 3, high = 3, zero = 1, one = 1
**Output:** 8
**Explanation:**
One possible valid good string is "011 ".
It can be constructed as follows: " " -> "0 " -> "01 " -> "011 ".
All binary strings from "000 " to "111 " are good strings in this example.
**Example 2:**
**Input:** low = 2, high = 3, zero = 1, one = 2
**Output:** 5
**Explanation:** The good strings are "00 ", "11 ", "000 ", "110 ", and "011 ".
**Constraints:**
* `1 <= low <= high <= 105`
* `1 <= zero, one <= low` | null |
Python3 dp solution (beats 100%) | count-ways-to-build-good-strings | 0 | 1 | # Code\n```\nclass Solution:\n def countGoodStrings(self, low: int, high: int, zero: int, one: int) -> int:\n strCount = 0\n\n dp = [0]*(high+1)\n dp[0] = 1\n\n greater = max(zero,one)\n lesser = min(zero,one)\n\n for i in range(lesser,greater):\n dp[i] += dp[i-lesser]%(10**9 + 7)\n \n for i in range(greater,high+1):\n dp[i] += (dp[i-zero]+dp[i-one])%(10**9 + 7)\n \n return sum(dp[low:high+1])%(10**9 + 7)\n\n``` | 1 | Given the integers `zero`, `one`, `low`, and `high`, we can construct a string by starting with an empty string, and then at each step perform either of the following:
* Append the character `'0'` `zero` times.
* Append the character `'1'` `one` times.
This can be performed any number of times.
A **good** string is a string constructed by the above process having a **length** between `low` and `high` (**inclusive**).
Return _the number of **different** good strings that can be constructed satisfying these properties._ Since the answer can be large, return it **modulo** `109 + 7`.
**Example 1:**
**Input:** low = 3, high = 3, zero = 1, one = 1
**Output:** 8
**Explanation:**
One possible valid good string is "011 ".
It can be constructed as follows: " " -> "0 " -> "01 " -> "011 ".
All binary strings from "000 " to "111 " are good strings in this example.
**Example 2:**
**Input:** low = 2, high = 3, zero = 1, one = 2
**Output:** 5
**Explanation:** The good strings are "00 ", "11 ", "000 ", "110 ", and "011 ".
**Constraints:**
* `1 <= low <= high <= 105`
* `1 <= zero, one <= low` | null |
Dynamic Programming based Python solution | count-ways-to-build-good-strings | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def countGoodStrings(self, low: int, high: int, zero: int, one: int) -> int:\n MAX = 1000000007\n table = [0]*(high+1)\n table[zero] += 1\n table[one] += 1\n start = min(zero, one)\n\n for i in range(start, high+1):\n # Fill in table[i]\n if i-zero >= 0:\n table[i] += table[i-zero] % MAX\n if i-one >= 0:\n table[i] += table[i-one] % MAX\n \n total_count = 0\n for i in range(low, high+1):\n total_count += table[i] % MAX\n return total_count % MAX\n``` | 1 | Given the integers `zero`, `one`, `low`, and `high`, we can construct a string by starting with an empty string, and then at each step perform either of the following:
* Append the character `'0'` `zero` times.
* Append the character `'1'` `one` times.
This can be performed any number of times.
A **good** string is a string constructed by the above process having a **length** between `low` and `high` (**inclusive**).
Return _the number of **different** good strings that can be constructed satisfying these properties._ Since the answer can be large, return it **modulo** `109 + 7`.
**Example 1:**
**Input:** low = 3, high = 3, zero = 1, one = 1
**Output:** 8
**Explanation:**
One possible valid good string is "011 ".
It can be constructed as follows: " " -> "0 " -> "01 " -> "011 ".
All binary strings from "000 " to "111 " are good strings in this example.
**Example 2:**
**Input:** low = 2, high = 3, zero = 1, one = 2
**Output:** 5
**Explanation:** The good strings are "00 ", "11 ", "000 ", "110 ", and "011 ".
**Constraints:**
* `1 <= low <= high <= 105`
* `1 <= zero, one <= low` | null |
Easy solution | count-ways-to-build-good-strings | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nComputing the number of strings is similar to computing Fibonacci numbers.\nThe number of strings of length n is equal to the number of strings of length n-zero plus the number of strings of length n-one, depending on what sequence they end in.\n\nTo start off the sequence, we assume that the number of strings of length 0 is 1, meaning we start from the empty string. Obviously, the number of strings with negative length is 0.\n\n# Complexity\n- Time complexity: $O(high)$.\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: $O(high)$.\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def countGoodStrings(self, low: int, high: int, zero: int, one: int) -> int: \n p=10**9+7 \n table=[1]*(high+1)\n for i in range(1, high+1):\n table[i]=((table[i-zero] if i>=zero else 0)+(table[i-one] if i>=one else 0))%p\n return sum(table[i] for i in range(low, high+1))%p\n``` | 1 | Given the integers `zero`, `one`, `low`, and `high`, we can construct a string by starting with an empty string, and then at each step perform either of the following:
* Append the character `'0'` `zero` times.
* Append the character `'1'` `one` times.
This can be performed any number of times.
A **good** string is a string constructed by the above process having a **length** between `low` and `high` (**inclusive**).
Return _the number of **different** good strings that can be constructed satisfying these properties._ Since the answer can be large, return it **modulo** `109 + 7`.
**Example 1:**
**Input:** low = 3, high = 3, zero = 1, one = 1
**Output:** 8
**Explanation:**
One possible valid good string is "011 ".
It can be constructed as follows: " " -> "0 " -> "01 " -> "011 ".
All binary strings from "000 " to "111 " are good strings in this example.
**Example 2:**
**Input:** low = 2, high = 3, zero = 1, one = 2
**Output:** 5
**Explanation:** The good strings are "00 ", "11 ", "000 ", "110 ", and "011 ".
**Constraints:**
* `1 <= low <= high <= 105`
* `1 <= zero, one <= low` | null |
Python3 Solution | count-ways-to-build-good-strings | 0 | 1 | \n```\nclass Solution:\n def countGoodStrings(self, low: int, high: int, zero: int, one: int) -> int:\n mod=10**9+7\n dp=[1]\n total=0\n\n for i in range(1,high+1):\n dp.append(0)\n\n if i-zero>=0:\n dp[i]+=dp[i-zero]\n\n if i-one>=0:\n dp[i]+=dp[i-one]\n\n dp[i]%=mod\n\n if low<=i<=high:\n total+=dp[i]\n total%=mod\n\n return total%mod \n``` | 1 | Given the integers `zero`, `one`, `low`, and `high`, we can construct a string by starting with an empty string, and then at each step perform either of the following:
* Append the character `'0'` `zero` times.
* Append the character `'1'` `one` times.
This can be performed any number of times.
A **good** string is a string constructed by the above process having a **length** between `low` and `high` (**inclusive**).
Return _the number of **different** good strings that can be constructed satisfying these properties._ Since the answer can be large, return it **modulo** `109 + 7`.
**Example 1:**
**Input:** low = 3, high = 3, zero = 1, one = 1
**Output:** 8
**Explanation:**
One possible valid good string is "011 ".
It can be constructed as follows: " " -> "0 " -> "01 " -> "011 ".
All binary strings from "000 " to "111 " are good strings in this example.
**Example 2:**
**Input:** low = 2, high = 3, zero = 1, one = 2
**Output:** 5
**Explanation:** The good strings are "00 ", "11 ", "000 ", "110 ", and "011 ".
**Constraints:**
* `1 <= low <= high <= 105`
* `1 <= zero, one <= low` | null |
Python 3, DFS Backtracking with Explanation | most-profitable-path-in-a-tree | 0 | 1 | Since the undirected tree has $$n$$ nodes and $$n-1$$ edges, there is only one path from bob to root $$0$$. The path from bob to root 0 as well as the time Bob reaches each node on the path is used to compute the reward (price) Alice gets at each node. \n\nWe will first use a DFS backtracking to determine Bob\'s path. The second backtracking is then implemented to compute the incomes Alice has when she travels to each leaf node.\n\nInline explanation is provided in the code. The code looks long but the two backtracking functions are quite similar, so the code is actually easy to understand.\n\nTime complexity: $$O(n)$$.\n\n# Python\n```\nclass Solution:\n def mostProfitablePath(self, edges: List[List[int]], bob: int, amount: List[int]) -> int:\n n = len(amount) # number of nodes\n graph = [set() for _ in range(n)] # adjacency list presentation of the tree\n for i,j in edges:\n graph[i].add(j)\n graph[j].add(i)\n bobpath = dict() # use hashmap to record nodes on the path from bob to root 0 \n # as well as time: node = key, time = value\n self.stop = False # True when Bob reaches root 0, this helps stop the DFS\n visited = [False]*n # True if node is visited, initialized as False for all nodes\n def backtrackbob(node,time): # the first backtracking, with time at each node\n bobpath[node] = time # add node:time to the hashmap\n visited[node] = True # mark node as visited\n if node==0: # root 0 is reached\n self.stop = True # this helps stop the DFS\n return None\n count = 0 # this helps determine if a node is leaf node\n for nei in graph[node]: \n if not visited[nei]:\n count += 1\n break\n if count==0: # node is leaf node if all neighbors are already visited\n del bobpath[node] # delete leaf node from hashmap before retreating from leaf node\n return None\n for nei in graph[node]: # explore unvisited neighbors of node\n if self.stop: return None # if root 0 is already reached, stop the DFS\n if not visited[nei]:\n backtrackbob(nei,time+1)\n if not self.stop: # if root 0 is reached, keep node in the hashmap when backtracking\n del bobpath[node] # otherwise, delete node before retreating\n return None\n\n backtrackbob(bob,0) # execute the first backtracking, time at bob is initialized = 0\n\n self.ans = float(-inf) # answer of the problem\n self.income = 0 # income of Alice when travelling to leaf nodes, initialized = 0\n visited = [False]*n # True if node is visited, initialized as False for all nodes\n def backtrackalice(node,time): # second backtracking, with time at each node\n visited[node] = True\n if node in bobpath: # if the node Alice visits is on Bob\'s path, there are 3 cases\n if time == bobpath[node]: # Alice and Bob reach the node at the same time\n reward = amount[node]//2\n elif time<bobpath[node]: # Alice reachs node before Bob\n reward = amount[node]\n else: # Alice reachs node after Bob\n reward = 0\n else: # if the node Alice visits is not on Bob\'s path\n reward = amount[node]\n self.income += reward # add the reward (price) at this node to the income\n count = 0 # this helps determine if a node is leaf node\n for nei in graph[node]:\n if not visited[nei]:\n count += 1\n break\n if count==0: # node is leaf node if all neighbors are already visited\n self.ans = max(self.ans,self.income) # update the answer\n self.income -= reward # remove the reward (price) at leaf node before backtracking\n return None\n for nei in graph[node]: # explore unvisited neighbors of node\n if not visited[nei]:\n backtrackalice(nei,time+1)\n self.income -= reward # remove the reward (price) at this node before retreating\n return None\n\n backtrackalice(0,0) # execute the second backtracking, time at root 0 is initialized = 0\n\n return self.ans\n``` | 1 | There is an undirected tree with `n` nodes labeled from `0` to `n - 1`, rooted at node `0`. You are given a 2D integer array `edges` of length `n - 1` where `edges[i] = [ai, bi]` indicates that there is an edge between nodes `ai` and `bi` in the tree.
At every node `i`, there is a gate. You are also given an array of even integers `amount`, where `amount[i]` represents:
* the price needed to open the gate at node `i`, if `amount[i]` is negative, or,
* the cash reward obtained on opening the gate at node `i`, otherwise.
The game goes on as follows:
* Initially, Alice is at node `0` and Bob is at node `bob`.
* At every second, Alice and Bob **each** move to an adjacent node. Alice moves towards some **leaf node**, while Bob moves towards node `0`.
* For **every** node along their path, Alice and Bob either spend money to open the gate at that node, or accept the reward. Note that:
* If the gate is **already open**, no price will be required, nor will there be any cash reward.
* If Alice and Bob reach the node **simultaneously**, they share the price/reward for opening the gate there. In other words, if the price to open the gate is `c`, then both Alice and Bob pay `c / 2` each. Similarly, if the reward at the gate is `c`, both of them receive `c / 2` each.
* If Alice reaches a leaf node, she stops moving. Similarly, if Bob reaches node `0`, he stops moving. Note that these events are **independent** of each other.
Return _the **maximum** net income Alice can have if she travels towards the optimal leaf node._
**Example 1:**
**Input:** edges = \[\[0,1\],\[1,2\],\[1,3\],\[3,4\]\], bob = 3, amount = \[-2,4,2,-4,6\]
**Output:** 6
**Explanation:**
The above diagram represents the given tree. The game goes as follows:
- Alice is initially on node 0, Bob on node 3. They open the gates of their respective nodes.
Alice's net income is now -2.
- Both Alice and Bob move to node 1.
Since they reach here simultaneously, they open the gate together and share the reward.
Alice's net income becomes -2 + (4 / 2) = 0.
- Alice moves on to node 3. Since Bob already opened its gate, Alice's income remains unchanged.
Bob moves on to node 0, and stops moving.
- Alice moves on to node 4 and opens the gate there. Her net income becomes 0 + 6 = 6.
Now, neither Alice nor Bob can make any further moves, and the game ends.
It is not possible for Alice to get a higher net income.
**Example 2:**
**Input:** edges = \[\[0,1\]\], bob = 1, amount = \[-7280,2350\]
**Output:** -7280
**Explanation:**
Alice follows the path 0->1 whereas Bob follows the path 1->0.
Thus, Alice opens the gate at node 0 only. Hence, her net income is -7280.
**Constraints:**
* `2 <= n <= 105`
* `edges.length == n - 1`
* `edges[i].length == 2`
* `0 <= ai, bi < n`
* `ai != bi`
* `edges` represents a valid tree.
* `1 <= bob < n`
* `amount.length == n`
* `amount[i]` is an **even** integer in the range `[-104, 104]`. | null |
Double DFS | most-profitable-path-in-a-tree | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\nBasically we don\'t care about the cost of Bob\'s path as long as he finds the root. since its a tree there should only be one way of getting to the root. do DFS on Bob and record the timestamps of the the node visits on the successful DFS run.\n\nDo DFS for alice and cross reference node visits with Bob\'s visits. add alice\'s income based on the given formula and cross referencing Bob\'s visits\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nfrom collections import defaultdict, deque\n\nclass Solution:\n def mostProfitablePath(self, edges: List[List[int]], bob: int, amount: List[int]) -> int:\n self.graph, self.leaves = self.buildGraph(edges)\n self.cost = amount\n self.bobTime = {}\n self.maxAmount = -1000000\n self.moveBob(bob, set([bob]), 0)\n self.moveAlice(0, 0, set([0]), 0)\n return int(self.maxAmount)\n\n def moveAlice(self, curNode, curAmount, seen, time):\n curAmount = self.getAmountFromNode(curNode, curAmount, time)\n # my proxy for a leaf node\n if curNode in self.leaves:\n self.maxAmount = max(self.maxAmount, curAmount)\n return\n for node in self.graph[curNode]:\n if node not in seen:\n seen.add(node)\n self.moveAlice(node, curAmount, seen, time + 1)\n # q = deque([(curNode, 0, 0)])\n # while q:\n # node, income, time = q.popleft()\n # income = self.getAmountFromNode(node, income, time)\n # if node in self.leaves:\n # self.maxAmount = max(self.maxAmount, income)\n # continue\n # for neighbor in self.graph[node]:\n # if neighbor not in seen:\n # seen.add(neighbor)\n # q.append((neighbor, income, time + 1))\n\n def getAmountFromNode(self, curNode, curAmount, time):\n if curNode in self.bobTime and self.bobTime[curNode] < time:\n pass\n elif curNode in self.bobTime and self.bobTime[curNode] == time:\n curAmount += self.cost[curNode] / 2\n elif curNode not in self.bobTime or self.bobTime[curNode] > time:\n curAmount += self.cost[curNode]\n return curAmount\n\n def buildGraph(self, edges: List[List[int]]):\n graph = defaultdict(list)\n leaves = set()\n for n1, n2 in edges:\n graph[n1].append(n2)\n graph[n2].append(n1)\n for node in graph:\n if len(graph[node]) == 1 and node != 0:\n leaves.add(node)\n return graph, leaves\n\n def moveBob(self, curNode, seen, time):\n if curNode == 0:\n return True\n # annotate all paths to the root for Bob because we don\'t know which is best for alice\n self.bobTime[curNode] = time\n for node in self.graph[curNode]:\n if node not in seen:\n seen.add(node)\n found = self.moveBob(node, seen, time + 1)\n if found:\n return found\n self.bobTime.pop(curNode, None)\n return False\n\n``` | 0 | There is an undirected tree with `n` nodes labeled from `0` to `n - 1`, rooted at node `0`. You are given a 2D integer array `edges` of length `n - 1` where `edges[i] = [ai, bi]` indicates that there is an edge between nodes `ai` and `bi` in the tree.
At every node `i`, there is a gate. You are also given an array of even integers `amount`, where `amount[i]` represents:
* the price needed to open the gate at node `i`, if `amount[i]` is negative, or,
* the cash reward obtained on opening the gate at node `i`, otherwise.
The game goes on as follows:
* Initially, Alice is at node `0` and Bob is at node `bob`.
* At every second, Alice and Bob **each** move to an adjacent node. Alice moves towards some **leaf node**, while Bob moves towards node `0`.
* For **every** node along their path, Alice and Bob either spend money to open the gate at that node, or accept the reward. Note that:
* If the gate is **already open**, no price will be required, nor will there be any cash reward.
* If Alice and Bob reach the node **simultaneously**, they share the price/reward for opening the gate there. In other words, if the price to open the gate is `c`, then both Alice and Bob pay `c / 2` each. Similarly, if the reward at the gate is `c`, both of them receive `c / 2` each.
* If Alice reaches a leaf node, she stops moving. Similarly, if Bob reaches node `0`, he stops moving. Note that these events are **independent** of each other.
Return _the **maximum** net income Alice can have if she travels towards the optimal leaf node._
**Example 1:**
**Input:** edges = \[\[0,1\],\[1,2\],\[1,3\],\[3,4\]\], bob = 3, amount = \[-2,4,2,-4,6\]
**Output:** 6
**Explanation:**
The above diagram represents the given tree. The game goes as follows:
- Alice is initially on node 0, Bob on node 3. They open the gates of their respective nodes.
Alice's net income is now -2.
- Both Alice and Bob move to node 1.
Since they reach here simultaneously, they open the gate together and share the reward.
Alice's net income becomes -2 + (4 / 2) = 0.
- Alice moves on to node 3. Since Bob already opened its gate, Alice's income remains unchanged.
Bob moves on to node 0, and stops moving.
- Alice moves on to node 4 and opens the gate there. Her net income becomes 0 + 6 = 6.
Now, neither Alice nor Bob can make any further moves, and the game ends.
It is not possible for Alice to get a higher net income.
**Example 2:**
**Input:** edges = \[\[0,1\]\], bob = 1, amount = \[-7280,2350\]
**Output:** -7280
**Explanation:**
Alice follows the path 0->1 whereas Bob follows the path 1->0.
Thus, Alice opens the gate at node 0 only. Hence, her net income is -7280.
**Constraints:**
* `2 <= n <= 105`
* `edges.length == n - 1`
* `edges[i].length == 2`
* `0 <= ai, bi < n`
* `ai != bi`
* `edges` represents a valid tree.
* `1 <= bob < n`
* `amount.length == n`
* `amount[i]` is an **even** integer in the range `[-104, 104]`. | null |
[Python3] Intuitive two DFS | most-profitable-path-in-a-tree | 0 | 1 | # Approach\n<!-- Describe your approach to solving the problem. -->\nRecord Bob\'s path and his time in reaching each node. Then compare with Alice\'s path, if there is interaction, split the rewards/costs. Also, if Alice reached this node earlier than Bob, then Alice can take the whole rewards/costs.\n\n\n# Complexity\n- Time complexity: O(2N) => O(N)\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: O(N)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```python3\nclass Solution:\n def mostProfitablePath(self, edges: List[List[int]], bob: int, amount: List[int]) -> int:\n graph = defaultdict(list)\n for edge in edges:\n graph[edge[0]].append(edge[1])\n graph[edge[1]].append(edge[0])\n\n bob_path = {}\n def get_bob_path(bob, steps, visited):\n nonlocal bob_path\n if bob in visited:\n return\n if bob == 0:\n if not bob_path:\n bob_path = steps.copy()\n else:\n bob_path = min(bob_path, steps, key = len)\n return\n visited.add(bob)\n for neighbor in graph[bob]:\n if neighbor not in visited:\n steps[neighbor] = steps[bob] + 1\n get_bob_path(neighbor, steps, visited)\n steps.pop(neighbor)\n\n get_bob_path(bob, {bob:0}, set())\n bob_path.pop(0) # we don\'t need to care about bob reached 0\n\n\n result = float("-inf")\n def get_alice_income(steps, visited, curr_income):\n nonlocal result\n index, step = steps\n if index in bob_path: # if alice and bob has interaction\n if step == bob_path[index]: # if they reached the same node at the same time\n curr_income += amount[index] // 2\n elif step < bob_path[index]: # if alice reached this node earlier than bob\n curr_income += amount[index]\n else: # no interaction\n curr_income += amount[index]\n if index != 0 and len(graph[index]) == 1: # leaf node, stop\n result = max(result, curr_income)\n return\n\n visited.add(index)\n for neighbor in graph[index]:\n if neighbor not in visited:\n get_alice_income((neighbor, step + 1), visited, curr_income)\n\n get_alice_income((0, 0), set(), 0)\n return result\n\n``` | 0 | There is an undirected tree with `n` nodes labeled from `0` to `n - 1`, rooted at node `0`. You are given a 2D integer array `edges` of length `n - 1` where `edges[i] = [ai, bi]` indicates that there is an edge between nodes `ai` and `bi` in the tree.
At every node `i`, there is a gate. You are also given an array of even integers `amount`, where `amount[i]` represents:
* the price needed to open the gate at node `i`, if `amount[i]` is negative, or,
* the cash reward obtained on opening the gate at node `i`, otherwise.
The game goes on as follows:
* Initially, Alice is at node `0` and Bob is at node `bob`.
* At every second, Alice and Bob **each** move to an adjacent node. Alice moves towards some **leaf node**, while Bob moves towards node `0`.
* For **every** node along their path, Alice and Bob either spend money to open the gate at that node, or accept the reward. Note that:
* If the gate is **already open**, no price will be required, nor will there be any cash reward.
* If Alice and Bob reach the node **simultaneously**, they share the price/reward for opening the gate there. In other words, if the price to open the gate is `c`, then both Alice and Bob pay `c / 2` each. Similarly, if the reward at the gate is `c`, both of them receive `c / 2` each.
* If Alice reaches a leaf node, she stops moving. Similarly, if Bob reaches node `0`, he stops moving. Note that these events are **independent** of each other.
Return _the **maximum** net income Alice can have if she travels towards the optimal leaf node._
**Example 1:**
**Input:** edges = \[\[0,1\],\[1,2\],\[1,3\],\[3,4\]\], bob = 3, amount = \[-2,4,2,-4,6\]
**Output:** 6
**Explanation:**
The above diagram represents the given tree. The game goes as follows:
- Alice is initially on node 0, Bob on node 3. They open the gates of their respective nodes.
Alice's net income is now -2.
- Both Alice and Bob move to node 1.
Since they reach here simultaneously, they open the gate together and share the reward.
Alice's net income becomes -2 + (4 / 2) = 0.
- Alice moves on to node 3. Since Bob already opened its gate, Alice's income remains unchanged.
Bob moves on to node 0, and stops moving.
- Alice moves on to node 4 and opens the gate there. Her net income becomes 0 + 6 = 6.
Now, neither Alice nor Bob can make any further moves, and the game ends.
It is not possible for Alice to get a higher net income.
**Example 2:**
**Input:** edges = \[\[0,1\]\], bob = 1, amount = \[-7280,2350\]
**Output:** -7280
**Explanation:**
Alice follows the path 0->1 whereas Bob follows the path 1->0.
Thus, Alice opens the gate at node 0 only. Hence, her net income is -7280.
**Constraints:**
* `2 <= n <= 105`
* `edges.length == n - 1`
* `edges[i].length == 2`
* `0 <= ai, bi < n`
* `ai != bi`
* `edges` represents a valid tree.
* `1 <= bob < n`
* `amount.length == n`
* `amount[i]` is an **even** integer in the range `[-104, 104]`. | null |
[Python] Simple dumb O(N) solution, no binary search needed | split-message-based-on-limit | 0 | 1 | # Intuition\nThe idea is to figure out how many characters the parts count take up. Once we know that, generating all the parts is trivial.\n\n# Approach\nSimply check if 9, 99, 999, or 9999 parts are enough to split the whole message, since 9, 99, 999, or 9999 are the maximum possible parts count for 1, 2, 3, or 4 characters.\n\nLet\'s say a message splits into 14 parts. For that string, the `splitable` function below will return `True` for 99, but `False` for 9 -> we need to reserve 2 characters for the parts count.\n\n# Complexity\n- Time complexity: O(n)\n- Space complexity: O(n)\n\n# Code\n```\nclass Solution:\n def splitMessage(self, message: str, limit: int) -> List[str]:\n def splitable_within(parts_limit):\n # check the message length achievable with <parts_limit> parts\n length = sum(limit - len(str(i)) - len(str(parts_limit)) - 3 for i in range(1, parts_limit + 1))\n return length >= len(message)\n \n parts_limit = 9\n if not splitable_within(parts_limit):\n parts_limit = 99\n if not splitable_within(parts_limit):\n parts_limit = 999\n if not splitable_within(parts_limit):\n parts_limit = 9999\n if not splitable_within(parts_limit):\n return []\n \n # generate the actual message parts\n parts = []\n m_index = 0 # message index\n for part_index in range(1, parts_limit + 1):\n if m_index >= len(message): break\n length = limit - len(str(part_index)) - len(str(parts_limit)) - 3\n parts.append(message[m_index:m_index + length])\n m_index += length\n \n return [f\'{part}<{i + 1}/{len(parts)}>\' for i, part in enumerate(parts)]\n\n``` | 3 | You are given a string, `message`, and a positive integer, `limit`.
You must **split** `message` into one or more **parts** based on `limit`. Each resulting part should have the suffix `" "`, where `"b "` is to be **replaced** with the total number of parts and `"a "` is to be **replaced** with the index of the part, starting from `1` and going up to `b`. Additionally, the length of each resulting part (including its suffix) should be **equal** to `limit`, except for the last part whose length can be **at most** `limit`.
The resulting parts should be formed such that when their suffixes are removed and they are all concatenated **in order**, they should be equal to `message`. Also, the result should contain as few parts as possible.
Return _the parts_ `message` _would be split into as an array of strings_. If it is impossible to split `message` as required, return _an empty array_.
**Example 1:**
**Input:** message = "this is really a very awesome message ", limit = 9
**Output:** \[ "thi<1/14> ", "s i<2/14> ", "s r<3/14> ", "eal<4/14> ", "ly <5/14> ", "a v<6/14> ", "ery<7/14> ", " aw<8/14> ", "eso<9/14> ", "me<10/14> ", " m<11/14> ", "es<12/14> ", "sa<13/14> ", "ge<14/14> "\]
**Explanation:**
The first 9 parts take 3 characters each from the beginning of message.
The next 5 parts take 2 characters each to finish splitting message.
In this example, each part, including the last, has length 9.
It can be shown it is not possible to split message into less than 14 parts.
**Example 2:**
**Input:** message = "short message ", limit = 15
**Output:** \[ "short mess<1/2> ", "age<2/2> "\]
**Explanation:**
Under the given constraints, the string can be split into two parts:
- The first part comprises of the first 10 characters, and has a length 15.
- The next part comprises of the last 3 characters, and has a length 8.
**Constraints:**
* `1 <= message.length <= 104`
* `message` consists only of lowercase English letters and `' '`.
* `1 <= limit <= 104` | null |
Total parts number calculation | split-message-based-on-limit | 0 | 1 | # Intuition\nThe tricky part of this task is that the size of payload is changing even in already processed parts of the message. Because of this we need some structure, that will maintain the size of message\'s part. Also it\'s not a good idea to use some kind of "greedy" approach because we would have to rearrange payload size in every produced message if the number of parts acquires another digit, which could be plenty of work and waste of memory (strings in python are immutable).\n\n# Approach\nThe root of sorting out this problem is to find the number of parts. The number of parts depends on the size of limit and the number of digits in itself. So, every time we add a decimal place to number of parts, we have to reduce the number of processed characters by number of produced parts (every part\'s payload is reduced by one character). We will do this until we consume all the characters from message. \nAfter succesful calculation of the number of parts, producing the list of parts is trivial.\n\n# Complexity\n- Time complexity:\n$$O(n)$$\n\n- Space complexity:\n$$O(n)$$\n\n# Code\n```\nfrom itertools import count, cycle, accumulate, takewhile\nfrom operator import mul, gt\nfrom functools import partial\n\n\ndef calculate_chars_in_power(limit: int, parts_in_power: int, suffix_length: int, remainder: int) -> tuple[int, int]:\n payload_length = limit - suffix_length\n whole_parts = remainder // payload_length\n if remainded_chars := remainder % payload_length:\n whole_parts += 1\n\n if parts_in_power > whole_parts:\n return whole_parts, remainder\n else:\n return parts_in_power, min(remainder, parts_in_power * payload_length)\n\n\ndef calculate_number_of_parts(total_length: int, limit: int) -> int:\n parts_number = 0\n parts_in_power_iter = accumulate(cycle([10, ]), mul, initial=9)\n\n states = zip(parts_in_power_iter, takewhile(partial(gt, limit), count(5, step=2)))\n chars_in_power_with_limit = partial(calculate_chars_in_power, limit)\n\n for parts_in_power, suffix_length in states:\n parts_ready, chars_ready = chars_in_power_with_limit(parts_in_power, suffix_length, total_length)\n total_length -= chars_ready\n parts_number += parts_ready\n\n if total_length == 0:\n return parts_number\n else:\n total_length += parts_number\n\n else:\n if total_length != 0:\n return 0\n \n return parts_number\n\n\ndef split(limit: int, message: str) -> list[str]:\n chars_processed = 0\n splitted = []\n number_of_parts = calculate_number_of_parts(len(message), limit)\n for part_number in range(1, number_of_parts + 1):\n mark = f\'<{part_number}/{number_of_parts}>\'\n payload = message[chars_processed:chars_processed + limit - len(mark)]\n chars_processed += len(payload)\n splitted.append(f\'{payload}{mark}\')\n\n return splitted\n\n\nclass Solution:\n def splitMessage(self, message: str, limit: int) -> List[str]:\n return split(limit, message) \n``` | 0 | You are given a string, `message`, and a positive integer, `limit`.
You must **split** `message` into one or more **parts** based on `limit`. Each resulting part should have the suffix `" "`, where `"b "` is to be **replaced** with the total number of parts and `"a "` is to be **replaced** with the index of the part, starting from `1` and going up to `b`. Additionally, the length of each resulting part (including its suffix) should be **equal** to `limit`, except for the last part whose length can be **at most** `limit`.
The resulting parts should be formed such that when their suffixes are removed and they are all concatenated **in order**, they should be equal to `message`. Also, the result should contain as few parts as possible.
Return _the parts_ `message` _would be split into as an array of strings_. If it is impossible to split `message` as required, return _an empty array_.
**Example 1:**
**Input:** message = "this is really a very awesome message ", limit = 9
**Output:** \[ "thi<1/14> ", "s i<2/14> ", "s r<3/14> ", "eal<4/14> ", "ly <5/14> ", "a v<6/14> ", "ery<7/14> ", " aw<8/14> ", "eso<9/14> ", "me<10/14> ", " m<11/14> ", "es<12/14> ", "sa<13/14> ", "ge<14/14> "\]
**Explanation:**
The first 9 parts take 3 characters each from the beginning of message.
The next 5 parts take 2 characters each to finish splitting message.
In this example, each part, including the last, has length 9.
It can be shown it is not possible to split message into less than 14 parts.
**Example 2:**
**Input:** message = "short message ", limit = 15
**Output:** \[ "short mess<1/2> ", "age<2/2> "\]
**Explanation:**
Under the given constraints, the string can be split into two parts:
- The first part comprises of the first 10 characters, and has a length 15.
- The next part comprises of the last 3 characters, and has a length 8.
**Constraints:**
* `1 <= message.length <= 104`
* `message` consists only of lowercase English letters and `' '`.
* `1 <= limit <= 104` | null |
O(n) solution with O(1) space with "simple" math and problem constraints analysis | split-message-based-on-limit | 0 | 1 | # Intuition\nThe intuition is that no matter how many parts you have, they will always follow a pattern of digits. Any number between 100 and 999, for example, will always have 3 digits. So instead of combining everything and seeing if it magically works (I\'m looking at you brute-forcers), we can calculate how many parts we actually need by trying all possible max part **digits** combinations, which are constrained by the problem.\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n$$O(n)$$\n\n- Space complexity:\n$$O(1)$$, as the output does not count as space\n\n# Code\n```\nfrom typing import Optional, List, Tuple\nimport math\n\nclass Solution:\n\n # O(n)\n def splitMessage(self, message: str, limit: int) -> List[str]:\n # Consider the smallest possible suffix: <1/1>\n # It\'s already 5 digits. Can\'t work with that...\n if limit <= 5:\n return []\n \n # O(1)\n def find_max_part() -> Optional[int]:\n # Consider that at most we\'ll have 5 digits for the\n # total part, as the problem is constrained to:\n #\n # 1 <= limit <= 10**4\n # 1 <= message.length <= 10**4\n #\n # In the worst case scenario, for the biggest message\n # possible and the smallest (viable) limit, we can\n # partition it in less than or equal to:\n #\n # 10 ** 4 = 10_000 parts (which has 5 digits) [1]\n #\n # [1] This was revealed to me in a dream.\n for total_digits in range(1, 5 + 1):\n remaining = len(message)\n parts = 0\n\n # Start reducing the message. First we start by the\n # 1 digit numbers, then 2 digit numbers, until we reach\n # the current total_digits maximum.\n for part_digits in range(1, total_digits + 1):\n # The maximum part can be calculated as such.\n #\n # This wields the following values:\n # [1, 9 ] => 10 - 1 = 9 items\n # [10, 99 ] => 100 - 10 = 90 items\n # [100, 999] => 1000 - 100 = 900 items\n max_part = 10 ** part_digits - 10 ** (part_digits - 1)\n\n suffix_len = 3 + part_digits + total_digits\n message_len = limit - suffix_len\n\n # No possible combination satisfies this. And \n # no possible >future< combination will neither, as\n # we\'re always increasing the number of digits.\n if message_len <= 0:\n return None\n\n # Calculate how many items we can fit here.\n # Be careful with the maximum this chunk can hold\n items_for_part = min(math.ceil(remaining / message_len), max_part)\n remaining -= items_for_part * message_len\n parts += items_for_part\n\n if remaining <= 0:\n return parts\n \n return None\n\n max_part = find_max_part()\n if not max_part:\n return []\n\n max_parts_str = str(max_part)\n \n i = 0\n partitions = []\n \n # Simply build the partitions.\n # O(n)\n for part in range(1, max_part + 1):\n part_str = str(part)\n message_chunk_len = limit - (3 + len(part_str) + len(max_parts_str))\n message_chunk = message[i:i+message_chunk_len]\n i += message_chunk_len\n\n partitions.append(f"{message_chunk}<{part_str}/{max_parts_str}>")\n \n return partitions\n\n``` | 0 | You are given a string, `message`, and a positive integer, `limit`.
You must **split** `message` into one or more **parts** based on `limit`. Each resulting part should have the suffix `" "`, where `"b "` is to be **replaced** with the total number of parts and `"a "` is to be **replaced** with the index of the part, starting from `1` and going up to `b`. Additionally, the length of each resulting part (including its suffix) should be **equal** to `limit`, except for the last part whose length can be **at most** `limit`.
The resulting parts should be formed such that when their suffixes are removed and they are all concatenated **in order**, they should be equal to `message`. Also, the result should contain as few parts as possible.
Return _the parts_ `message` _would be split into as an array of strings_. If it is impossible to split `message` as required, return _an empty array_.
**Example 1:**
**Input:** message = "this is really a very awesome message ", limit = 9
**Output:** \[ "thi<1/14> ", "s i<2/14> ", "s r<3/14> ", "eal<4/14> ", "ly <5/14> ", "a v<6/14> ", "ery<7/14> ", " aw<8/14> ", "eso<9/14> ", "me<10/14> ", " m<11/14> ", "es<12/14> ", "sa<13/14> ", "ge<14/14> "\]
**Explanation:**
The first 9 parts take 3 characters each from the beginning of message.
The next 5 parts take 2 characters each to finish splitting message.
In this example, each part, including the last, has length 9.
It can be shown it is not possible to split message into less than 14 parts.
**Example 2:**
**Input:** message = "short message ", limit = 15
**Output:** \[ "short mess<1/2> ", "age<2/2> "\]
**Explanation:**
Under the given constraints, the string can be split into two parts:
- The first part comprises of the first 10 characters, and has a length 15.
- The next part comprises of the last 3 characters, and has a length 8.
**Constraints:**
* `1 <= message.length <= 104`
* `message` consists only of lowercase English letters and `' '`.
* `1 <= limit <= 104` | null |
just impliment of task | split-message-based-on-limit | 0 | 1 | \n# Approach\njust impliment of task\n\n# Code\n```\nclass Solution:\n def splitMessage(self, m: str, l: int) -> List[str]:\n d=len(m)\n for i in range(1,5):\n t=d\n for j in range(1,i):t-=(10**j-1)\n if(k:=l-3-2*i)<=0:return []\n if (p:=(t-1+k)//k)>(10**i-1):continue\n o,x=[],0\n for i in range(p):\n z=len(s:=f\'<{i+1}/{p}>\')\n o.append(m[x:x+(l-z)]+s)\n x+=(l-z)\n return o\n \n``` | 0 | You are given a string, `message`, and a positive integer, `limit`.
You must **split** `message` into one or more **parts** based on `limit`. Each resulting part should have the suffix `" "`, where `"b "` is to be **replaced** with the total number of parts and `"a "` is to be **replaced** with the index of the part, starting from `1` and going up to `b`. Additionally, the length of each resulting part (including its suffix) should be **equal** to `limit`, except for the last part whose length can be **at most** `limit`.
The resulting parts should be formed such that when their suffixes are removed and they are all concatenated **in order**, they should be equal to `message`. Also, the result should contain as few parts as possible.
Return _the parts_ `message` _would be split into as an array of strings_. If it is impossible to split `message` as required, return _an empty array_.
**Example 1:**
**Input:** message = "this is really a very awesome message ", limit = 9
**Output:** \[ "thi<1/14> ", "s i<2/14> ", "s r<3/14> ", "eal<4/14> ", "ly <5/14> ", "a v<6/14> ", "ery<7/14> ", " aw<8/14> ", "eso<9/14> ", "me<10/14> ", " m<11/14> ", "es<12/14> ", "sa<13/14> ", "ge<14/14> "\]
**Explanation:**
The first 9 parts take 3 characters each from the beginning of message.
The next 5 parts take 2 characters each to finish splitting message.
In this example, each part, including the last, has length 9.
It can be shown it is not possible to split message into less than 14 parts.
**Example 2:**
**Input:** message = "short message ", limit = 15
**Output:** \[ "short mess<1/2> ", "age<2/2> "\]
**Explanation:**
Under the given constraints, the string can be split into two parts:
- The first part comprises of the first 10 characters, and has a length 15.
- The next part comprises of the last 3 characters, and has a length 8.
**Constraints:**
* `1 <= message.length <= 104`
* `message` consists only of lowercase English letters and `' '`.
* `1 <= limit <= 104` | null |
Python binary search solution beats 99.25% | split-message-based-on-limit | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def splitMessage(self, message: str, limit: int) -> List[str]:\n ln=len(message)\n maxSuffix=(limit-3-1)//2\n if maxSuffix<1:\n return []\n \n left=1\n right=maxSuffix+1\n allParts=0\n while left<right:\n mid=(left+right)//2\n space=limit-3-mid-1\n if mid>maxSuffix:\n break\n digits=1\n parts=0\n words=ln\n while digits<=mid:\n part=ceil(words/space)\n carry=(9*(10**(digits-1)))\n if part>carry:\n words-=carry*space\n parts+=carry\n digits+=1\n space-=1\n else:\n parts+=part\n break\n if digits>mid:\n left=mid+1\n else:\n right=mid\n allParts=parts\n\n if right>maxSuffix:\n return []\n res=[]\n id=0\n space=limit-3-right-1\n parts=1\n digits=1\n while id<ln:\n part=ceil((ln-id)/space)\n for _ in range(min(part,9*(10**(digits-1)))):\n res.append(message[id:id+space]+f"<{parts}/{allParts}>")\n id+=space\n parts+=1\n digits+=1\n space-=1\n return res\n``` | 0 | You are given a string, `message`, and a positive integer, `limit`.
You must **split** `message` into one or more **parts** based on `limit`. Each resulting part should have the suffix `" "`, where `"b "` is to be **replaced** with the total number of parts and `"a "` is to be **replaced** with the index of the part, starting from `1` and going up to `b`. Additionally, the length of each resulting part (including its suffix) should be **equal** to `limit`, except for the last part whose length can be **at most** `limit`.
The resulting parts should be formed such that when their suffixes are removed and they are all concatenated **in order**, they should be equal to `message`. Also, the result should contain as few parts as possible.
Return _the parts_ `message` _would be split into as an array of strings_. If it is impossible to split `message` as required, return _an empty array_.
**Example 1:**
**Input:** message = "this is really a very awesome message ", limit = 9
**Output:** \[ "thi<1/14> ", "s i<2/14> ", "s r<3/14> ", "eal<4/14> ", "ly <5/14> ", "a v<6/14> ", "ery<7/14> ", " aw<8/14> ", "eso<9/14> ", "me<10/14> ", " m<11/14> ", "es<12/14> ", "sa<13/14> ", "ge<14/14> "\]
**Explanation:**
The first 9 parts take 3 characters each from the beginning of message.
The next 5 parts take 2 characters each to finish splitting message.
In this example, each part, including the last, has length 9.
It can be shown it is not possible to split message into less than 14 parts.
**Example 2:**
**Input:** message = "short message ", limit = 15
**Output:** \[ "short mess<1/2> ", "age<2/2> "\]
**Explanation:**
Under the given constraints, the string can be split into two parts:
- The first part comprises of the first 10 characters, and has a length 15.
- The next part comprises of the last 3 characters, and has a length 8.
**Constraints:**
* `1 <= message.length <= 104`
* `message` consists only of lowercase English letters and `' '`.
* `1 <= limit <= 104` | null |
No Binary Search | Purely Mathematical | Beats 99.04% | split-message-based-on-limit | 0 | 1 | \n\n\n# Intuition\nFollowing formula can be deduced for this problem\n$$ \\sum_{i=1}^{d} 9 * 10^{ (i-1)} * ( limit - 3 -(i+d)) $$\n\nwhere limit is the given parameter in the program, d is maximum number of digits there can be in split, for eg. for 14 it is 2. and i is {1, ... , d}. This provides us total length of string that can be constructed if we want d digits in size of split.\n\nUsing this finding d suitable for our case, Since max limit here is 10**4, hence a total of 5 digits, therefore searching from 1..5 for best len for our split value or d.\n\n\n# Code\n```\nfrom math import ceil\n\nclass Solution:\n def splitMessage(self, message: str, limit: int) -> List[str]:\n def func(limit, dig_num):\n s = 0\n for i in range(1, dig_num+1):\n mid_term = 9 * (10 ** (i - 1 )) *(limit - 3 - (i + dig_num ))\n s+=mid_term\n return s, mid_term\n\n proposed_chars = 0\n\n for i in range(1, 6): #Because max chars can be 5 as limit is 10**4\n s, mid_term = func(limit, i)\n if s >= len(message):\n proposed_chars = i\n break\n if proposed_chars == 0: return []\n\n n = len(message)\n n -= (s - mid_term)\n extras = ceil(n / (limit - (3 + 2*proposed_chars) ))\n total = 10 ** (proposed_chars - 1) + ( extras - 1)\n res = []\n prev = 0\n for i in range(total):\n num_chars = limit - 3 - proposed_chars - len(str(i+1))\n mid_str = message[ prev : min(len(message), prev + num_chars) ]\n mid_str = mid_str + "<{}/{}>".format(i+1, total)\n res.append(mid_str)\n prev+=num_chars\n\n return res\n \n``` | 0 | You are given a string, `message`, and a positive integer, `limit`.
You must **split** `message` into one or more **parts** based on `limit`. Each resulting part should have the suffix `" "`, where `"b "` is to be **replaced** with the total number of parts and `"a "` is to be **replaced** with the index of the part, starting from `1` and going up to `b`. Additionally, the length of each resulting part (including its suffix) should be **equal** to `limit`, except for the last part whose length can be **at most** `limit`.
The resulting parts should be formed such that when their suffixes are removed and they are all concatenated **in order**, they should be equal to `message`. Also, the result should contain as few parts as possible.
Return _the parts_ `message` _would be split into as an array of strings_. If it is impossible to split `message` as required, return _an empty array_.
**Example 1:**
**Input:** message = "this is really a very awesome message ", limit = 9
**Output:** \[ "thi<1/14> ", "s i<2/14> ", "s r<3/14> ", "eal<4/14> ", "ly <5/14> ", "a v<6/14> ", "ery<7/14> ", " aw<8/14> ", "eso<9/14> ", "me<10/14> ", " m<11/14> ", "es<12/14> ", "sa<13/14> ", "ge<14/14> "\]
**Explanation:**
The first 9 parts take 3 characters each from the beginning of message.
The next 5 parts take 2 characters each to finish splitting message.
In this example, each part, including the last, has length 9.
It can be shown it is not possible to split message into less than 14 parts.
**Example 2:**
**Input:** message = "short message ", limit = 15
**Output:** \[ "short mess<1/2> ", "age<2/2> "\]
**Explanation:**
Under the given constraints, the string can be split into two parts:
- The first part comprises of the first 10 characters, and has a length 15.
- The next part comprises of the last 3 characters, and has a length 8.
**Constraints:**
* `1 <= message.length <= 104`
* `message` consists only of lowercase English letters and `' '`.
* `1 <= limit <= 104` | null |
Beginner Understanding | split-message-based-on-limit | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\nBest in all the way to make the beginners understand good and the flow is user-friendly.\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def splitMessage(self, message: str, limit: int) -> List[str]:\n dig,cum_sum=[0]*10001,[0]*10001\n ret=0\n \n for i in range(1,10001):\n dig[i] = len(str(i)) \n cum_sum[i] = cum_sum[i-1]+dig[i]\n \n actual_size = len(message)\n ret=[]\n\n for N in range(1,actual_size+1):\n newSize=actual_size+3*N+dig[N]*N+cum_sum[N]\n\n if ((newSize+limit-1)//limit==N):\n print("in",N)\n s=""\n cur = 1\n\n extra = dig[N]+3\n for c in message:\n s+=c\n if len(s)+dig[cur]+extra==limit:\n s+="<"+str(cur)+"/"+str(N)+">"\n ret.append(s)\n s=""\n cur+=1\n print("s is:",s)\n if len(s):\n s+="<"+str(cur)+"/"+str(N)+">"\n ret.append(s)\n s=""\n return ret\n return ret\n``` | 0 | You are given a string, `message`, and a positive integer, `limit`.
You must **split** `message` into one or more **parts** based on `limit`. Each resulting part should have the suffix `" "`, where `"b "` is to be **replaced** with the total number of parts and `"a "` is to be **replaced** with the index of the part, starting from `1` and going up to `b`. Additionally, the length of each resulting part (including its suffix) should be **equal** to `limit`, except for the last part whose length can be **at most** `limit`.
The resulting parts should be formed such that when their suffixes are removed and they are all concatenated **in order**, they should be equal to `message`. Also, the result should contain as few parts as possible.
Return _the parts_ `message` _would be split into as an array of strings_. If it is impossible to split `message` as required, return _an empty array_.
**Example 1:**
**Input:** message = "this is really a very awesome message ", limit = 9
**Output:** \[ "thi<1/14> ", "s i<2/14> ", "s r<3/14> ", "eal<4/14> ", "ly <5/14> ", "a v<6/14> ", "ery<7/14> ", " aw<8/14> ", "eso<9/14> ", "me<10/14> ", " m<11/14> ", "es<12/14> ", "sa<13/14> ", "ge<14/14> "\]
**Explanation:**
The first 9 parts take 3 characters each from the beginning of message.
The next 5 parts take 2 characters each to finish splitting message.
In this example, each part, including the last, has length 9.
It can be shown it is not possible to split message into less than 14 parts.
**Example 2:**
**Input:** message = "short message ", limit = 15
**Output:** \[ "short mess<1/2> ", "age<2/2> "\]
**Explanation:**
Under the given constraints, the string can be split into two parts:
- The first part comprises of the first 10 characters, and has a length 15.
- The next part comprises of the last 3 characters, and has a length 8.
**Constraints:**
* `1 <= message.length <= 104`
* `message` consists only of lowercase English letters and `' '`.
* `1 <= limit <= 104` | null |
Two phases python solution | split-message-based-on-limit | 0 | 1 | # Intuition\n\nFirst one need to find the number of parts. After that the splitting is obvious.\n\n# Complexity\n- Time complexity: $$O(n\\log(n))$$ where $n$ is the length of the message. \n\nThe $\\log(n)$ comes from the `tags_total_len` function which is called at most $n$ times, but this is a very rough estimation. This is true since if the `message` can be split into parts then the number of parts is at most the length of the message, and at each iteration either we stop or increase the number of parts by at least one. \n\n# Code\n```\ndef tags_total_len(n):\n """returns sum(len("<"+str(i)+"/"+str(n)+">") for i in range(1, n+1))"""\n total = 0 \n n_digit = 1\n bottom = 0\n top = 9\n while top < n:\n total += (top-bottom)*n_digit\n bottom = top\n top = 10*top+9\n n_digit += 1\n total += (n-bottom)*n_digit + (3+n_digit)*n\n return total\n \ndef tags_max_len(n):\n return len(str(n))*2+3\n\nclass Solution:\n def splitMessage(self, message: str, limit: int) -> List[str]:\n required = len(message)\n n, r = divmod(required, limit)\n n = n + int(r > 0)\n while True:\n if tags_max_len(n)>=limit:\n return []\n required = len(message) + tags_total_len(n)\n n0, r0 = divmod(required, limit)\n n0 = n0 + int(r0 > 0)\n if n0 == n:\n break\n else:\n n = n0\n result = []\n start = 0\n for i in range(1, n+1):\n tag = f"<{i}/{n}>"\n mlen = limit-len(tag)\n result.append(message[start:start+mlen]+tag)\n start += mlen\n return result\n\n``` | 0 | You are given a string, `message`, and a positive integer, `limit`.
You must **split** `message` into one or more **parts** based on `limit`. Each resulting part should have the suffix `" "`, where `"b "` is to be **replaced** with the total number of parts and `"a "` is to be **replaced** with the index of the part, starting from `1` and going up to `b`. Additionally, the length of each resulting part (including its suffix) should be **equal** to `limit`, except for the last part whose length can be **at most** `limit`.
The resulting parts should be formed such that when their suffixes are removed and they are all concatenated **in order**, they should be equal to `message`. Also, the result should contain as few parts as possible.
Return _the parts_ `message` _would be split into as an array of strings_. If it is impossible to split `message` as required, return _an empty array_.
**Example 1:**
**Input:** message = "this is really a very awesome message ", limit = 9
**Output:** \[ "thi<1/14> ", "s i<2/14> ", "s r<3/14> ", "eal<4/14> ", "ly <5/14> ", "a v<6/14> ", "ery<7/14> ", " aw<8/14> ", "eso<9/14> ", "me<10/14> ", " m<11/14> ", "es<12/14> ", "sa<13/14> ", "ge<14/14> "\]
**Explanation:**
The first 9 parts take 3 characters each from the beginning of message.
The next 5 parts take 2 characters each to finish splitting message.
In this example, each part, including the last, has length 9.
It can be shown it is not possible to split message into less than 14 parts.
**Example 2:**
**Input:** message = "short message ", limit = 15
**Output:** \[ "short mess<1/2> ", "age<2/2> "\]
**Explanation:**
Under the given constraints, the string can be split into two parts:
- The first part comprises of the first 10 characters, and has a length 15.
- The next part comprises of the last 3 characters, and has a length 8.
**Constraints:**
* `1 <= message.length <= 104`
* `message` consists only of lowercase English letters and `' '`.
* `1 <= limit <= 104` | null |
Python (Simple Maths) | split-message-based-on-limit | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def splitMessage(self, message, limit):\n p = a = 1\n\n while p*(len(str(p))+3) + a + len(message) > p*limit:\n if 3 + len(str(p))*2 >= limit: return []\n p += 1\n a += len(str(p)) \n\n res = []\n\n for i in range(1,p+1):\n j = limit - (len(str(i))+len(str(p))+3)\n part, message = message[:j], message[j:]\n res.append(part + "<"+ str(i) + "/" + str(p) + ">") \n\n return res\n\n``` | 0 | You are given a string, `message`, and a positive integer, `limit`.
You must **split** `message` into one or more **parts** based on `limit`. Each resulting part should have the suffix `" "`, where `"b "` is to be **replaced** with the total number of parts and `"a "` is to be **replaced** with the index of the part, starting from `1` and going up to `b`. Additionally, the length of each resulting part (including its suffix) should be **equal** to `limit`, except for the last part whose length can be **at most** `limit`.
The resulting parts should be formed such that when their suffixes are removed and they are all concatenated **in order**, they should be equal to `message`. Also, the result should contain as few parts as possible.
Return _the parts_ `message` _would be split into as an array of strings_. If it is impossible to split `message` as required, return _an empty array_.
**Example 1:**
**Input:** message = "this is really a very awesome message ", limit = 9
**Output:** \[ "thi<1/14> ", "s i<2/14> ", "s r<3/14> ", "eal<4/14> ", "ly <5/14> ", "a v<6/14> ", "ery<7/14> ", " aw<8/14> ", "eso<9/14> ", "me<10/14> ", " m<11/14> ", "es<12/14> ", "sa<13/14> ", "ge<14/14> "\]
**Explanation:**
The first 9 parts take 3 characters each from the beginning of message.
The next 5 parts take 2 characters each to finish splitting message.
In this example, each part, including the last, has length 9.
It can be shown it is not possible to split message into less than 14 parts.
**Example 2:**
**Input:** message = "short message ", limit = 15
**Output:** \[ "short mess<1/2> ", "age<2/2> "\]
**Explanation:**
Under the given constraints, the string can be split into two parts:
- The first part comprises of the first 10 characters, and has a length 15.
- The next part comprises of the last 3 characters, and has a length 8.
**Constraints:**
* `1 <= message.length <= 104`
* `message` consists only of lowercase English letters and `' '`.
* `1 <= limit <= 104` | null |
Python || One line Code || 99.6% beats | convert-the-temperature | 0 | 1 | **Plz Upvote ..if you got help from this..**\n# Code\n```\nclass Solution:\n def convertTemperature(self, celsius: float) -> List[float]:\n return [celsius + 273.15, celsius * 1.80 + 32.00 ]\n # or\n kelvin = celsius + 273.15\n fahrenheit = celsius * 1.80 + 32.00\n\n return [kelvin, fahrenheit]\n\n\n\n\n``` | 2 | You are given a non-negative floating point number rounded to two decimal places `celsius`, that denotes the **temperature in Celsius**.
You should convert Celsius into **Kelvin** and **Fahrenheit** and return it as an array `ans = [kelvin, fahrenheit]`.
Return _the array `ans`._ Answers within `10-5` of the actual answer will be accepted.
**Note that:**
* `Kelvin = Celsius + 273.15`
* `Fahrenheit = Celsius * 1.80 + 32.00`
**Example 1:**
**Input:** celsius = 36.50
**Output:** \[309.65000,97.70000\]
**Explanation:** Temperature at 36.50 Celsius converted in Kelvin is 309.65 and converted in Fahrenheit is 97.70.
**Example 2:**
**Input:** celsius = 122.11
**Output:** \[395.26000,251.79800\]
**Explanation:** Temperature at 122.11 Celsius converted in Kelvin is 395.26 and converted in Fahrenheit is 251.798.
**Constraints:**
* `0 <= celsius <= 1000` | null |
🔥🔥1 Liner🔥🔥 and that's it | convert-the-temperature | 0 | 1 | \n# Code\n```\nclass Solution:\n def convertTemperature(self, celsius: float) -> List[float]:\n return celsius + 273.15 , celsius * 1.80+32.00\n``` | 4 | You are given a non-negative floating point number rounded to two decimal places `celsius`, that denotes the **temperature in Celsius**.
You should convert Celsius into **Kelvin** and **Fahrenheit** and return it as an array `ans = [kelvin, fahrenheit]`.
Return _the array `ans`._ Answers within `10-5` of the actual answer will be accepted.
**Note that:**
* `Kelvin = Celsius + 273.15`
* `Fahrenheit = Celsius * 1.80 + 32.00`
**Example 1:**
**Input:** celsius = 36.50
**Output:** \[309.65000,97.70000\]
**Explanation:** Temperature at 36.50 Celsius converted in Kelvin is 309.65 and converted in Fahrenheit is 97.70.
**Example 2:**
**Input:** celsius = 122.11
**Output:** \[395.26000,251.79800\]
**Explanation:** Temperature at 122.11 Celsius converted in Kelvin is 395.26 and converted in Fahrenheit is 251.798.
**Constraints:**
* `0 <= celsius <= 1000` | null |
Easy O(1): Create a new language from scratch, a register-based VM and calculate the result in it | convert-the-temperature | 0 | 1 | # Intuition\nSelf explanatory.\n\n# Approach\nCreate an x86 assembly-like language, a parser and an "infinite" (8 is good enough) register-based VM to execute the program.\n\n# Complexity\n- Time complexity:\n$$O(who\\:cares)$$\n\n- Space complexity:\n$$O(1)$$\n\n\n# Code\n```\nfrom enum import Enum\nfrom typing import List\nfrom dataclasses import dataclass\nimport re\n\nclass Opcode(Enum):\n MOV = 0\n MOVC = 1\n ADD = 2\n MUL = 3\n RET = 4\n\n@dataclass\nclass Instruction:\n opcode: Opcode\n constant: float = 0\n register_a: int = 0\n register_b: int = 0\n\nclass Parser:\n\n @staticmethod\n def parse(s: str) -> List[Instruction]:\n lines = s.split("\\n")\n lines = map(lambda l: l.strip(), lines)\n lines = filter(lambda l: not l.startswith(";"), lines)\n lines = filter(None, lines)\n\n instructions = []\n for line in lines:\n instruction = Instruction(Opcode.RET)\n\n parts = re.split(r\'\\s*,\\s*|\\s+\', line)\n match parts[0]:\n case "mov": \n instruction.opcode = Opcode.MOV\n instruction.register_a = Parser.parse_register(parts[1])\n instruction.register_b = Parser.parse_register(parts[2])\n\n case "movc": \n instruction.opcode = Opcode.MOVC\n instruction.register_a = Parser.parse_register(parts[1])\n instruction.constant = Parser.parse_constant(parts[2])\n\n case "add":\n instruction.opcode = Opcode.ADD\n instruction.register_a = Parser.parse_register(parts[1])\n instruction.register_b = Parser.parse_register(parts[2])\n\n case "mul": \n instruction.opcode = Opcode.MUL\n instruction.register_a = Parser.parse_register(parts[1])\n instruction.register_b = Parser.parse_register(parts[2])\n \n case "ret":\n instruction.opcode = Opcode.RET\n\n case _:\n raise Exception(f"unknown instruction {parts[0]}")\n \n instructions.append(instruction)\n\n return instructions\n\n @staticmethod\n def parse_register(s: str) -> int:\n return int(s[1:])\n \n @staticmethod\n def parse_constant(s: str) -> float:\n return float(s)\n\nclass VM:\n MAX_REGISTERS = 8\n\n def __init__(self, s: str):\n self.registers = [0.0] * VM.MAX_REGISTERS\n self.program = Parser.parse(s)\n \n def set_register(self, i: int, v: float):\n self.registers[i] = v\n\n def get_register(self, i: int) -> float:\n return self.registers[i]\n \n def execute(self):\n for instruction in self.program:\n match instruction.opcode:\n case Opcode.MOV:\n self.registers[instruction.register_a] = self.registers[instruction.register_b]\n case Opcode.MOVC:\n self.registers[instruction.register_a] = instruction.constant\n case Opcode.ADD:\n self.registers[instruction.register_a] += self.registers[instruction.register_b]\n case Opcode.MUL:\n self.registers[instruction.register_a] *= self.registers[instruction.register_b]\n case Opcode.RET:\n return\n\nclass Solution:\n def convertTemperature(self, celsius: float) -> List[float]:\n source = """\n ; calculate in kelvin\n mov r1, r0\n movc r2, 273.15\n add r1, r2\n\n ; calculate in farenheit\n mov r2, r0\n movc r3, 1.80\n movc r4, 32.0\n mul r2, r3\n add r2, r4\n\n ; results in r1 and r2\n ret\n """\n\n vm = VM(source)\n vm.set_register(0, celsius)\n vm.execute()\n\n kelvin = vm.get_register(1)\n farenheit = vm.get_register(2)\n\n return [kelvin, farenheit]\n``` | 1 | You are given a non-negative floating point number rounded to two decimal places `celsius`, that denotes the **temperature in Celsius**.
You should convert Celsius into **Kelvin** and **Fahrenheit** and return it as an array `ans = [kelvin, fahrenheit]`.
Return _the array `ans`._ Answers within `10-5` of the actual answer will be accepted.
**Note that:**
* `Kelvin = Celsius + 273.15`
* `Fahrenheit = Celsius * 1.80 + 32.00`
**Example 1:**
**Input:** celsius = 36.50
**Output:** \[309.65000,97.70000\]
**Explanation:** Temperature at 36.50 Celsius converted in Kelvin is 309.65 and converted in Fahrenheit is 97.70.
**Example 2:**
**Input:** celsius = 122.11
**Output:** \[395.26000,251.79800\]
**Explanation:** Temperature at 122.11 Celsius converted in Kelvin is 395.26 and converted in Fahrenheit is 251.798.
**Constraints:**
* `0 <= celsius <= 1000` | null |
Python short and easy | number-of-subarrays-with-lcm-equal-to-k | 0 | 1 | This question is just a variant of [2447](https://leetcode.com/problems/number-of-subarrays-with-gcd-equal-to-k/description/) asked in recent contest\n```\nfrom math import gcd\nclass Solution:\n \n def lcm(self,a, b):\n return abs(a*b) // math.gcd(a, b)\n\n def subarrayLCM(self, nums: List[int], k: int) -> int:\n \n cnt = 0\n size = len(nums)\n for ind in range(size) :\n\n curLcm = nums[ind]\n\n for ind2 in range(ind, size) :\n curLcm = self.lcm(curLcm, nums[ind2])\n cnt += (curLcm == k)\n\n return cnt\n```\n\nMy solution for 2447 for reference :\n```\nfrom math import gcd\nclass Solution:\n def subarrayGCD(self, nums: List[int], k: int) -> int:\n \n cnt = 0\n size = len(nums)\n for ind in range(size) :\n\n curGcd = 0\n\n for ind2 in range(ind, size) :\n curGcd = gcd(curGcd , nums[ind2])\n\n cnt += (curGcd == k)\n\n return cnt\n``` | 2 | Given an integer array `nums` and an integer `k`, return _the number of **subarrays** of_ `nums` _where the least common multiple of the subarray's elements is_ `k`.
A **subarray** is a contiguous non-empty sequence of elements within an array.
The **least common multiple of an array** is the smallest positive integer that is divisible by all the array elements.
**Example 1:**
**Input:** nums = \[3,6,2,7,1\], k = 6
**Output:** 4
**Explanation:** The subarrays of nums where 6 is the least common multiple of all the subarray's elements are:
- \[**3**,**6**,2,7,1\]
- \[**3**,**6**,**2**,7,1\]
- \[3,**6**,2,7,1\]
- \[3,**6**,**2**,7,1\]
**Example 2:**
**Input:** nums = \[3\], k = 2
**Output:** 0
**Explanation:** There are no subarrays of nums where 2 is the least common multiple of all the subarray's elements.
**Constraints:**
* `1 <= nums.length <= 1000`
* `1 <= nums[i], k <= 1000` | null |
Beginners Approach : | number-of-subarrays-with-lcm-equal-to-k | 0 | 1 | ```\nclass Solution:\n def subarrayLCM(self, nums: List[int], k: int) -> int:\n def find_lcm(num1, num2):\n if(num1>num2):\n num = num1\n den = num2\n else:\n num = num2\n den = num1\n rem = num % den\n while(rem != 0):\n num = den\n den = rem\n rem = num % den\n gcd = den\n lcm = int(int(num1 * num2)/int(gcd))\n return lcm\n count=0\n for i in range(len(nums)):\n lcm=1\n for j in range(i,len(nums)):\n lcm=find_lcm(nums[j],lcm)\n \n if lcm==k:\n count+=1\n if lcm>k:\n break\n return count\n``` | 1 | Given an integer array `nums` and an integer `k`, return _the number of **subarrays** of_ `nums` _where the least common multiple of the subarray's elements is_ `k`.
A **subarray** is a contiguous non-empty sequence of elements within an array.
The **least common multiple of an array** is the smallest positive integer that is divisible by all the array elements.
**Example 1:**
**Input:** nums = \[3,6,2,7,1\], k = 6
**Output:** 4
**Explanation:** The subarrays of nums where 6 is the least common multiple of all the subarray's elements are:
- \[**3**,**6**,2,7,1\]
- \[**3**,**6**,**2**,7,1\]
- \[3,**6**,2,7,1\]
- \[3,**6**,**2**,7,1\]
**Example 2:**
**Input:** nums = \[3\], k = 2
**Output:** 0
**Explanation:** There are no subarrays of nums where 2 is the least common multiple of all the subarray's elements.
**Constraints:**
* `1 <= nums.length <= 1000`
* `1 <= nums[i], k <= 1000` | null |
Python||Very Easy Implementation|| | number-of-subarrays-with-lcm-equal-to-k | 0 | 1 | \n def gcd(a,b):\n while b:\n a,b = b, a%b\n return abs(a)\n def lcm(a,b):\n return a*b / gcd(a,b)\n def subarrayLCM(self, nums: List[int], k: int) -> int:\n c=0\n l=len(nums)\n for i in range(l):\n x=nums[i]\n for j in range(i,l):\n x=lcm(x,nums[j])\n if x==k:\n c+=1\n return c | 4 | Given an integer array `nums` and an integer `k`, return _the number of **subarrays** of_ `nums` _where the least common multiple of the subarray's elements is_ `k`.
A **subarray** is a contiguous non-empty sequence of elements within an array.
The **least common multiple of an array** is the smallest positive integer that is divisible by all the array elements.
**Example 1:**
**Input:** nums = \[3,6,2,7,1\], k = 6
**Output:** 4
**Explanation:** The subarrays of nums where 6 is the least common multiple of all the subarray's elements are:
- \[**3**,**6**,2,7,1\]
- \[**3**,**6**,**2**,7,1\]
- \[3,**6**,2,7,1\]
- \[3,**6**,**2**,7,1\]
**Example 2:**
**Input:** nums = \[3\], k = 2
**Output:** 0
**Explanation:** There are no subarrays of nums where 2 is the least common multiple of all the subarray's elements.
**Constraints:**
* `1 <= nums.length <= 1000`
* `1 <= nums[i], k <= 1000` | null |
python solution | number-of-subarrays-with-lcm-equal-to-k | 0 | 1 | ```\nimport math\nclass Solution:\n def subarrayLCM(self, nums: List[int], k: int) -> int:\n def gcd(a,b):\n return math.gcd(a,b)\n def lcm(n1,n2):\n return (n1*n2)//gcd(n1,n2)\n n=len(nums)\n ans=0\n for i in range(n):\n lcmi=nums[i]\n for j in range(i,n):\n lcmi=lcm(lcmi,nums[j])\n if(lcmi==k):\n ans+=1\n return ans\n``` | 4 | Given an integer array `nums` and an integer `k`, return _the number of **subarrays** of_ `nums` _where the least common multiple of the subarray's elements is_ `k`.
A **subarray** is a contiguous non-empty sequence of elements within an array.
The **least common multiple of an array** is the smallest positive integer that is divisible by all the array elements.
**Example 1:**
**Input:** nums = \[3,6,2,7,1\], k = 6
**Output:** 4
**Explanation:** The subarrays of nums where 6 is the least common multiple of all the subarray's elements are:
- \[**3**,**6**,2,7,1\]
- \[**3**,**6**,**2**,7,1\]
- \[3,**6**,2,7,1\]
- \[3,**6**,**2**,7,1\]
**Example 2:**
**Input:** nums = \[3\], k = 2
**Output:** 0
**Explanation:** There are no subarrays of nums where 2 is the least common multiple of all the subarray's elements.
**Constraints:**
* `1 <= nums.length <= 1000`
* `1 <= nums[i], k <= 1000` | null |
Recursive solution in python | number-of-subarrays-with-lcm-equal-to-k | 0 | 1 | # Approach\nConsider every index in the list, if it\'s lcm <= k, expand it in both sides until lcm >= k. And keep the subarray if its lcm = k. Avoid redundant computing with the memo dict. \n\n\n# Code\n```\nimport math\nclass Solution:\n def subarrayLCM(self, nums, k: int) -> int:\n if len(set(nums)) == 1:\n if nums[0] == k:\n return int(len(nums)*(len(nums)+1)//2)\n elif nums[0] < k:\n return 0\n memo = {}\n n = len(nums)\n def aux(i,j,nuevo,mcm):\n def MCM(a,b):\n return a*int(b)/math.gcd(a,int(b))\n if i > j or i < 0 or j == len(nums):\n return 0\n if (i,j) in memo:\n if memo[(i,j)] > k:\n return 0\n elif memo[(i,j)] <= k:\n return aux(i-1,j,nums[(i-1)%n],mcm) + aux(i,j+1,nums[(j+1)%n],mcm)\n else:\n mcm = MCM(nuevo,mcm)\n memo[(i,j)] = mcm\n if mcm > k:\n return 0\n elif mcm == k:\n return 1 + aux(i-1,j,nums[(i-1)%n],mcm) + aux(i,j+1,nums[(j+1)%n],mcm)\n else:\n return aux(i-1,j,nums[(i-1)%n],mcm) + aux(i,j+1,nums[(j+1)%n],mcm)\n total = 0\n for x in range(len(nums)):\n total += aux(x,x,nums[x],nums[x])\n return total\n``` | 0 | Given an integer array `nums` and an integer `k`, return _the number of **subarrays** of_ `nums` _where the least common multiple of the subarray's elements is_ `k`.
A **subarray** is a contiguous non-empty sequence of elements within an array.
The **least common multiple of an array** is the smallest positive integer that is divisible by all the array elements.
**Example 1:**
**Input:** nums = \[3,6,2,7,1\], k = 6
**Output:** 4
**Explanation:** The subarrays of nums where 6 is the least common multiple of all the subarray's elements are:
- \[**3**,**6**,2,7,1\]
- \[**3**,**6**,**2**,7,1\]
- \[3,**6**,2,7,1\]
- \[3,**6**,**2**,7,1\]
**Example 2:**
**Input:** nums = \[3\], k = 2
**Output:** 0
**Explanation:** There are no subarrays of nums where 2 is the least common multiple of all the subarray's elements.
**Constraints:**
* `1 <= nums.length <= 1000`
* `1 <= nums[i], k <= 1000` | null |
Python Straightforward | number-of-subarrays-with-lcm-equal-to-k | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nimport math\nclass Solution:\n def subarrayLCM(self, nums: List[int], k: int) -> int:\n n = len(nums)\n res = 0\n for i in range(n):\n lcm = nums[i]\n if lcm == k:\n res += 1\n for j in range(i+1,n):\n \n if lcm > k:\n break\n lcm = (nums[j]*lcm)//(math.gcd(lcm,nums[j]))\n if lcm == k:\n res += 1\n return res\n \n\n\n``` | 0 | Given an integer array `nums` and an integer `k`, return _the number of **subarrays** of_ `nums` _where the least common multiple of the subarray's elements is_ `k`.
A **subarray** is a contiguous non-empty sequence of elements within an array.
The **least common multiple of an array** is the smallest positive integer that is divisible by all the array elements.
**Example 1:**
**Input:** nums = \[3,6,2,7,1\], k = 6
**Output:** 4
**Explanation:** The subarrays of nums where 6 is the least common multiple of all the subarray's elements are:
- \[**3**,**6**,2,7,1\]
- \[**3**,**6**,**2**,7,1\]
- \[3,**6**,2,7,1\]
- \[3,**6**,**2**,7,1\]
**Example 2:**
**Input:** nums = \[3\], k = 2
**Output:** 0
**Explanation:** There are no subarrays of nums where 2 is the least common multiple of all the subarray's elements.
**Constraints:**
* `1 <= nums.length <= 1000`
* `1 <= nums[i], k <= 1000` | null |
Python3 | Easy Solution | minimum-number-of-operations-to-sort-a-binary-tree-by-level | 0 | 1 | # Code\n```\n# Definition for a binary tree node.\n# class TreeNode:\n# def __init__(self, val=0, left=None, right=None):\n# self.val = val\n# self.left = left\n# self.right = right\nclass Solution:\n def minimumOperations(self, root: Optional[TreeNode]) -> int:\n queue = deque([root])\n ans = 0\n while len(queue)!=0:\n levelNode = []\n for k in range(len(queue)):\n nowNode = queue.popleft()\n levelNode.append(nowNode.val)\n if nowNode.left!=None:\n queue.append(nowNode.left)\n if nowNode.right!=None:\n queue.append(nowNode.right)\n \n levelNodeSort = sorted(levelNode)\n posDict = defaultdict(int)\n for i in range(len(levelNode)):\n posDict[levelNode[i]] = i\n for i in range(len(levelNode)):\n if(levelNode[i]!=levelNodeSort[i]):\n replace = posDict[levelNodeSort[i]]\n levelNode[replace] = levelNode[i]\n posDict[levelNode[i]] = replace\n levelNode[i] = levelNodeSort[i]\n ans+=1\n\n return ans\n \n \n \n``` | 2 | You are given the `root` of a binary tree with **unique values**.
In one operation, you can choose any two nodes **at the same level** and swap their values.
Return _the minimum number of operations needed to make the values at each level sorted in a **strictly increasing order**_.
The **level** of a node is the number of edges along the path between it and the root node_._
**Example 1:**
**Input:** root = \[1,4,3,7,6,8,5,null,null,null,null,9,null,10\]
**Output:** 3
**Explanation:**
- Swap 4 and 3. The 2nd level becomes \[3,4\].
- Swap 7 and 5. The 3rd level becomes \[5,6,8,7\].
- Swap 8 and 7. The 3rd level becomes \[5,6,7,8\].
We used 3 operations so return 3.
It can be proven that 3 is the minimum number of operations needed.
**Example 2:**
**Input:** root = \[1,3,2,7,6,5,4\]
**Output:** 3
**Explanation:**
- Swap 3 and 2. The 2nd level becomes \[2,3\].
- Swap 7 and 4. The 3rd level becomes \[4,6,5,7\].
- Swap 6 and 5. The 3rd level becomes \[4,5,6,7\].
We used 3 operations so return 3.
It can be proven that 3 is the minimum number of operations needed.
**Example 3:**
**Input:** root = \[1,2,3,4,5,6\]
**Output:** 0
**Explanation:** Each level is already sorted in increasing order so return 0.
**Constraints:**
* The number of nodes in the tree is in the range `[1, 105]`.
* `1 <= Node.val <= 105`
* All the values of the tree are **unique**. | null |
level order + swap sort | minimum-number-of-operations-to-sort-a-binary-tree-by-level | 0 | 1 | class Solution:\n def minimumOperations(self, root: Optional[TreeNode]) -> int:\n \n def swp(arr):\n n = len(arr)\n ans = 0\n temp = arr.copy()\n h = {}\n temp.sort()\n\n for i in range(n):\n\n h[arr[i]] = i\n\n init = 0\n\n for i in range(n):\n\n if (arr[i] != temp[i]):\n ans += 1\n init = arr[i]\n arr[i], arr[h[temp[i]]] = arr[h[temp[i]]], arr[i]\n h[init] = h[temp[i]]\n h[temp[i]] = i\n\n return ans\n \n count = 0\n if not root:\n return 0\n queue = [root]\n \n while queue:\n size = len(queue)\n temp = []\n for _ in range(size):\n node = queue.pop(0)\n temp.append(node.val)\n if node.left:\n queue.append(node.left)\n if node.right:\n queue.append(node.right)\n count += swp(temp)\n return count | 2 | You are given the `root` of a binary tree with **unique values**.
In one operation, you can choose any two nodes **at the same level** and swap their values.
Return _the minimum number of operations needed to make the values at each level sorted in a **strictly increasing order**_.
The **level** of a node is the number of edges along the path between it and the root node_._
**Example 1:**
**Input:** root = \[1,4,3,7,6,8,5,null,null,null,null,9,null,10\]
**Output:** 3
**Explanation:**
- Swap 4 and 3. The 2nd level becomes \[3,4\].
- Swap 7 and 5. The 3rd level becomes \[5,6,8,7\].
- Swap 8 and 7. The 3rd level becomes \[5,6,7,8\].
We used 3 operations so return 3.
It can be proven that 3 is the minimum number of operations needed.
**Example 2:**
**Input:** root = \[1,3,2,7,6,5,4\]
**Output:** 3
**Explanation:**
- Swap 3 and 2. The 2nd level becomes \[2,3\].
- Swap 7 and 4. The 3rd level becomes \[4,6,5,7\].
- Swap 6 and 5. The 3rd level becomes \[4,5,6,7\].
We used 3 operations so return 3.
It can be proven that 3 is the minimum number of operations needed.
**Example 3:**
**Input:** root = \[1,2,3,4,5,6\]
**Output:** 0
**Explanation:** Each level is already sorted in increasing order so return 0.
**Constraints:**
* The number of nodes in the tree is in the range `[1, 105]`.
* `1 <= Node.val <= 105`
* All the values of the tree are **unique**. | null |
[Python3] BFS | minimum-number-of-operations-to-sort-a-binary-tree-by-level | 0 | 1 | \n```\nclass Solution:\n def minimumOperations(self, root: Optional[TreeNode]) -> int:\n ans = 0 \n queue = deque([root])\n while queue: \n vals = []\n for _ in range(len(queue)): \n node = queue.popleft()\n vals.append(node.val)\n if node.left: queue.append(node.left)\n if node.right: queue.append(node.right)\n mp = {x : i for i, x in enumerate(sorted(vals))}\n visited = [0]*len(vals)\n for i in range(len(vals)): \n cnt = 0 \n while not visited[i] and i != mp[vals[i]]: \n visited[i] = 1\n cnt += 1\n i = mp[vals[i]]\n ans += max(0, cnt-1)\n return ans \n``` | 8 | You are given the `root` of a binary tree with **unique values**.
In one operation, you can choose any two nodes **at the same level** and swap their values.
Return _the minimum number of operations needed to make the values at each level sorted in a **strictly increasing order**_.
The **level** of a node is the number of edges along the path between it and the root node_._
**Example 1:**
**Input:** root = \[1,4,3,7,6,8,5,null,null,null,null,9,null,10\]
**Output:** 3
**Explanation:**
- Swap 4 and 3. The 2nd level becomes \[3,4\].
- Swap 7 and 5. The 3rd level becomes \[5,6,8,7\].
- Swap 8 and 7. The 3rd level becomes \[5,6,7,8\].
We used 3 operations so return 3.
It can be proven that 3 is the minimum number of operations needed.
**Example 2:**
**Input:** root = \[1,3,2,7,6,5,4\]
**Output:** 3
**Explanation:**
- Swap 3 and 2. The 2nd level becomes \[2,3\].
- Swap 7 and 4. The 3rd level becomes \[4,6,5,7\].
- Swap 6 and 5. The 3rd level becomes \[4,5,6,7\].
We used 3 operations so return 3.
It can be proven that 3 is the minimum number of operations needed.
**Example 3:**
**Input:** root = \[1,2,3,4,5,6\]
**Output:** 0
**Explanation:** Each level is already sorted in increasing order so return 0.
**Constraints:**
* The number of nodes in the tree is in the range `[1, 105]`.
* `1 <= Node.val <= 105`
* All the values of the tree are **unique**. | null |
Easy Level order traversal with comments || Python | minimum-number-of-operations-to-sort-a-binary-tree-by-level | 0 | 1 | ```\nclass Solution:\n def cntSwap(self,arr):\n \n n = len(arr)\n cnt = 0\n a = sorted(arr)\n index = {}\n \n\t\t# store index of elements in arr\n for ind in range(n):\n index[arr[ind]] = ind\n\n for ind in range(n):\n\t\t\t\n\t\t\t# If element in arr is out of position we need to swap hence increase cnt\n if (arr[ind] != a[ind]):\n cnt += 1\n pos = arr[ind]\n \n\t\t\t\t# Replace it with element that should be at this "ind" in arr using a[ind]\n arr[ind], arr[index[a[ind]]] = arr[index[a[ind]]], arr[ind]\n\t\t\t\t\n\t\t\t\t# Update the indexes for swaped elements in "index"\n index[pos] = index[a[ind]]\n index[a[ind]] = ind\n\n return cnt\n \n def minimumOperations(self, root: Optional[TreeNode]) -> int:\n \n res = 0\n q = [root]\n # Level order traversal\n while q :\n \n level = []\n values = []\n for n in q:\n \n if n.left :\n level.append(n.left)\n values.append(n.left.val)\n \n if n.right :\n level.append(n.right)\n values.append(n.right.val)\n \n res += self.cntSwap(values)\n q = level\n \n return res\n``` | 5 | You are given the `root` of a binary tree with **unique values**.
In one operation, you can choose any two nodes **at the same level** and swap their values.
Return _the minimum number of operations needed to make the values at each level sorted in a **strictly increasing order**_.
The **level** of a node is the number of edges along the path between it and the root node_._
**Example 1:**
**Input:** root = \[1,4,3,7,6,8,5,null,null,null,null,9,null,10\]
**Output:** 3
**Explanation:**
- Swap 4 and 3. The 2nd level becomes \[3,4\].
- Swap 7 and 5. The 3rd level becomes \[5,6,8,7\].
- Swap 8 and 7. The 3rd level becomes \[5,6,7,8\].
We used 3 operations so return 3.
It can be proven that 3 is the minimum number of operations needed.
**Example 2:**
**Input:** root = \[1,3,2,7,6,5,4\]
**Output:** 3
**Explanation:**
- Swap 3 and 2. The 2nd level becomes \[2,3\].
- Swap 7 and 4. The 3rd level becomes \[4,6,5,7\].
- Swap 6 and 5. The 3rd level becomes \[4,5,6,7\].
We used 3 operations so return 3.
It can be proven that 3 is the minimum number of operations needed.
**Example 3:**
**Input:** root = \[1,2,3,4,5,6\]
**Output:** 0
**Explanation:** Each level is already sorted in increasing order so return 0.
**Constraints:**
* The number of nodes in the tree is in the range `[1, 105]`.
* `1 <= Node.val <= 105`
* All the values of the tree are **unique**. | null |
[Python 3] BFS | Union-Find | minimum-number-of-operations-to-sort-a-binary-tree-by-level | 0 | 1 | ```\nclass Solution:\n def minimumOperations(self, root: Optional[TreeNode]) -> int:\n par = {}\n rank = {}\n \n def ins(n1):\n if n1 in par:\n return\n par[n1] = n1\n rank[n1] = 1\n \n def find(n1):\n while n1 != par[n1]:\n par[n1] = par[par[n1]]\n n1 = par[n1]\n return n1\n \n def union(n1, n2):\n p1, p2 = find(n1), find(n2)\n if p1 == p2:\n return 0\n if rank[p1] < rank[p2]:\n p1, p2 = p2, p1\n par[p2] = p1\n rank[p1] += rank[p2]\n return 1\n \n def helper(nums):\n t = sorted(nums)\n \n for i, j in zip(nums, t):\n ins(i)\n ins(j)\n union(i, j)\n \n return sum(rank[i] - 1 for i in nums if i == find(i))\n \n \n q = deque( [root] )\n res = 0\n \n while q:\n level = []\n \n for _ in range(len(q)):\n cur = q.popleft()\n level.append(cur.val)\n \n if cur.left: q.append(cur.left)\n if cur.right: q.append(cur.right)\n \n res += helper(level)\n \n return res\n``` | 2 | You are given the `root` of a binary tree with **unique values**.
In one operation, you can choose any two nodes **at the same level** and swap their values.
Return _the minimum number of operations needed to make the values at each level sorted in a **strictly increasing order**_.
The **level** of a node is the number of edges along the path between it and the root node_._
**Example 1:**
**Input:** root = \[1,4,3,7,6,8,5,null,null,null,null,9,null,10\]
**Output:** 3
**Explanation:**
- Swap 4 and 3. The 2nd level becomes \[3,4\].
- Swap 7 and 5. The 3rd level becomes \[5,6,8,7\].
- Swap 8 and 7. The 3rd level becomes \[5,6,7,8\].
We used 3 operations so return 3.
It can be proven that 3 is the minimum number of operations needed.
**Example 2:**
**Input:** root = \[1,3,2,7,6,5,4\]
**Output:** 3
**Explanation:**
- Swap 3 and 2. The 2nd level becomes \[2,3\].
- Swap 7 and 4. The 3rd level becomes \[4,6,5,7\].
- Swap 6 and 5. The 3rd level becomes \[4,5,6,7\].
We used 3 operations so return 3.
It can be proven that 3 is the minimum number of operations needed.
**Example 3:**
**Input:** root = \[1,2,3,4,5,6\]
**Output:** 0
**Explanation:** Each level is already sorted in increasing order so return 0.
**Constraints:**
* The number of nodes in the tree is in the range `[1, 105]`.
* `1 <= Node.val <= 105`
* All the values of the tree are **unique**. | null |
Python [Explained] | O(nlogn) | minimum-number-of-operations-to-sort-a-binary-tree-by-level | 0 | 1 | * We will find all the nodes at current level and then count minimum number of swaps required to make them sorted.\n\n* How to calculate minimum swaps required to make array sorted :\n\nmake a new array (called temp), which is the sorted form of the input array. We know that we need to transform the input array to the new array (temp) in the minimum number of swaps. Make a map that stores the elements and their corresponding index, of the input array.\n\n* So at each i starting from 0 to N in the given array, where N is the size of the array:\n\n1. If i is not in its correct position according to the sorted array, then\n2. We will fill this position with the correct element from the hashmap we built earlier. We know the correct element which should come here is temp[i], so we look up the index of this element from the hashmap. \n3. After swapping the required elements, we update the content of the hashmap accordingly, as temp[i] to the ith position, and arr[i] to where temp[i] was earlier.\n\n**complexity of above approach :**\n* Time Complexity: O(n Log n) \n* Auxiliary Space: O(n)\n----------------------\n**Level order traversal :**\n* Time Complexity: O(n) \n* Auxiliary Space: O(n)\n------------------------\n**Overall complexity :**\n* Time Complexity: O(n log n) \n* Auxiliary Space: O(n)\n---------------\n**Code**\n```\n# Definition for a binary tree node.\n# class TreeNode:\n# def __init__(self, val=0, left=None, right=None):\n# self.val = val\n# self.left = left\n# self.right = right\nclass Solution:\n def minimumOperations(self, root: Optional[TreeNode]) -> int:\n def minSwap(arr, n): #function to count minimum number of swaps to make array sorted\n ans = 0\n temp = arr.copy()\n h = {}\n temp.sort()\n\n for i in range(n):\n h[arr[i]] = i\n init = 0\n\n for i in range(n):\n if (arr[i] != temp[i]):\n ans += 1\n init = arr[i]\n arr[i], arr[h[temp[i]]] = arr[h[temp[i]]], arr[i]\n h[init] = h[temp[i]]\n h[temp[i]] = i\n\n return ans\n \n def levelorder(root, ans):\n if root:\n q = []\n q.append(root)\n \n while q:\n sublist = [] #sublist will store all the nodes at current level in sequense.\n q_len = len(q)\n for node in q:\n sublist.append(node.val)\n ans += minSwap(sublist, len(sublist)) #count swaps required to sort current level\n \n while q_len > 0:\n node = q.pop(0)\n if node.left:\n q.append(node.left)\n if node.right:\n q.append(node.right)\n q_len -= 1\n return ans\n \n return levelorder(root, 0)\n```\n-------------------\n\n**Upvote the post if you find it helpful.\nHappy coding.** | 1 | You are given the `root` of a binary tree with **unique values**.
In one operation, you can choose any two nodes **at the same level** and swap their values.
Return _the minimum number of operations needed to make the values at each level sorted in a **strictly increasing order**_.
The **level** of a node is the number of edges along the path between it and the root node_._
**Example 1:**
**Input:** root = \[1,4,3,7,6,8,5,null,null,null,null,9,null,10\]
**Output:** 3
**Explanation:**
- Swap 4 and 3. The 2nd level becomes \[3,4\].
- Swap 7 and 5. The 3rd level becomes \[5,6,8,7\].
- Swap 8 and 7. The 3rd level becomes \[5,6,7,8\].
We used 3 operations so return 3.
It can be proven that 3 is the minimum number of operations needed.
**Example 2:**
**Input:** root = \[1,3,2,7,6,5,4\]
**Output:** 3
**Explanation:**
- Swap 3 and 2. The 2nd level becomes \[2,3\].
- Swap 7 and 4. The 3rd level becomes \[4,6,5,7\].
- Swap 6 and 5. The 3rd level becomes \[4,5,6,7\].
We used 3 operations so return 3.
It can be proven that 3 is the minimum number of operations needed.
**Example 3:**
**Input:** root = \[1,2,3,4,5,6\]
**Output:** 0
**Explanation:** Each level is already sorted in increasing order so return 0.
**Constraints:**
* The number of nodes in the tree is in the range `[1, 105]`.
* `1 <= Node.val <= 105`
* All the values of the tree are **unique**. | null |
[C++, Java, Python3] Palindromic Substrings + Non-overlapping Intervals | maximum-number-of-non-overlapping-palindrome-substrings | 1 | 1 | \n**Explanation**\nThis question is a combination of Palindromic Substring and Non-overlapping intervals\nhttps://leetcode.com/problems/palindromic-substrings/\nhttps://leetcode.com/problems/non-overlapping-intervals/\n* First find all palindromic substrings with length >= k in O(n*k) and store their start and end in an `intervals` list\n\n* Then find minumum number of intervals you need to remove to make the `intervals` array non overlapping in O(n) (`intervals` is already added in sorted order.)\n\n**Solution1:**\n<iframe src="https://leetcode.com/playground/FZexChvv/shared" frameBorder="0" width="1000" height="510"></iframe>\n\n*Time complexity = O(nk)*\n\n**Solution2:**\nNo need to find non overlapping intervals just record the end of the last found palindromic substring. \n\n<iframe src="https://leetcode.com/playground/V2R8VVPx/shared" frameBorder="0" width="1000" height="345"></iframe>\n\n*Time complexity = O(nk)* | 97 | You are given a string `s` and a **positive** integer `k`.
Select a set of **non-overlapping** substrings from the string `s` that satisfy the following conditions:
* The **length** of each substring is **at least** `k`.
* Each substring is a **palindrome**.
Return _the **maximum** number of substrings in an optimal selection_.
A **substring** is a contiguous sequence of characters within a string.
**Example 1:**
**Input:** s = "abaccdbbd ", k = 3
**Output:** 2
**Explanation:** We can select the substrings underlined in s = "**aba**cc**dbbd** ". Both "aba " and "dbbd " are palindromes and have a length of at least k = 3.
It can be shown that we cannot find a selection with more than two valid substrings.
**Example 2:**
**Input:** s = "adbcda ", k = 2
**Output:** 0
**Explanation:** There is no palindrome substring of length at least 2 in the string.
**Constraints:**
* `1 <= k <= s.length <= 2000`
* `s` consists of lowercase English letters. | null |
[Python3] DP with Explanations | Only Check Substrings of Length k and k + 1 | maximum-number-of-non-overlapping-palindrome-substrings | 0 | 1 | **Observation**\nGiven the constraints, a solution with quadratic time complexity suffices for this problem. The key to this problem is to note that, if a given palindromic substring has length greater or equal to `k + 2`, then we can always remove the first and last character of the substring without losing the optimality. As such, we only need to check if a substring is a palindrome that has length `k` or `k + 1`.\n \n**Implementation**\nWe define a `dp` array where `dp[i]` represents the maximum number of substrings in the first `i` characters. For each possible `i`, we check whether the substring with length `k` or `k + 1` ending at the `i`-th character (1-indexed) is a valid palindrome, and update the value of `dp[i]`.\n \n**Complexity**\nTime Complexity: `O(nk)`\nSpace Complexity: `O(n)`, for the use of `dp`\n \n**Solution**\n```\nclass Solution:\n def maxPalindromes(self, s: str, k: int) -> int:\n n = len(s)\n dp = [0] * (n + 1)\n for i in range(k, n + 1):\n dp[i] = dp[i - 1]\n for length in range(k, k + 2):\n j = i - length\n if j < 0:\n break\n if self.isPalindrome(s, j, i):\n dp[i] = max(dp[i], 1 + dp[j])\n return dp[-1]\n \n \n def isPalindrome(self, s, j, i):\n left, right = j, i - 1\n while left < right:\n if s[left] != s[right]:\n return False\n left += 1\n right -= 1\n return True\n```\n \n **Follow-up (open question)** Can we improve the time complexity from `O(nk)` to `O(n)` by using the Manacher\'s algorithm? | 12 | You are given a string `s` and a **positive** integer `k`.
Select a set of **non-overlapping** substrings from the string `s` that satisfy the following conditions:
* The **length** of each substring is **at least** `k`.
* Each substring is a **palindrome**.
Return _the **maximum** number of substrings in an optimal selection_.
A **substring** is a contiguous sequence of characters within a string.
**Example 1:**
**Input:** s = "abaccdbbd ", k = 3
**Output:** 2
**Explanation:** We can select the substrings underlined in s = "**aba**cc**dbbd** ". Both "aba " and "dbbd " are palindromes and have a length of at least k = 3.
It can be shown that we cannot find a selection with more than two valid substrings.
**Example 2:**
**Input:** s = "adbcda ", k = 2
**Output:** 0
**Explanation:** There is no palindrome substring of length at least 2 in the string.
**Constraints:**
* `1 <= k <= s.length <= 2000`
* `s` consists of lowercase English letters. | null |
✅ [Python] Very simple solution - O(n) || no DP | maximum-number-of-non-overlapping-palindrome-substrings | 0 | 1 | ## Intuition\nSimilar to 647 https://leetcode.com/problems/palindromic-substrings/\nJust need to add the constraint - non-overlapping\n\n## Approach\nExpand from centers to make the time complexity O(N), space complexity O(1)\nOnly count the palindrome with shortest length (>=k)\nKeep track of the last end position to avoid overlapping\n\n## Code\n```\nclass Solution:\n def maxPalindromes(self, s: str, k: int) -> int:\n res = 0\n lastp = -1\n\n def helper(l, r):\n nonlocal lastp, res\n while l >= 0 and r < len(s) and s[l] == s[r] and l > lastp:\n if r-l+1 >= k:\n res += 1\n lastp = r\n break # find the shortest palindrome\n else:\n l -= 1\n r += 1\n \n for i in range(len(s)):\n helper(i, i) # odd length\n helper(i, i+1) # even length\n return res \n``` | 2 | You are given a string `s` and a **positive** integer `k`.
Select a set of **non-overlapping** substrings from the string `s` that satisfy the following conditions:
* The **length** of each substring is **at least** `k`.
* Each substring is a **palindrome**.
Return _the **maximum** number of substrings in an optimal selection_.
A **substring** is a contiguous sequence of characters within a string.
**Example 1:**
**Input:** s = "abaccdbbd ", k = 3
**Output:** 2
**Explanation:** We can select the substrings underlined in s = "**aba**cc**dbbd** ". Both "aba " and "dbbd " are palindromes and have a length of at least k = 3.
It can be shown that we cannot find a selection with more than two valid substrings.
**Example 2:**
**Input:** s = "adbcda ", k = 2
**Output:** 0
**Explanation:** There is no palindrome substring of length at least 2 in the string.
**Constraints:**
* `1 <= k <= s.length <= 2000`
* `s` consists of lowercase English letters. | null |
Subsets and Splits